How Can You Effectively Store API Keys in a Docker Container?
In the fast-evolving world of software development, securing sensitive information is more crucial than ever. Among the various types of sensitive data, API keys stand out as vital credentials that grant access to external services and resources. When deploying applications within Docker containers, the challenge of managing these keys securely becomes even more pronounced. The convenience of containerization should not come at the expense of security, and understanding how to best store API keys in Docker containers is essential for any developer looking to safeguard their applications.
Storing API keys in Docker containers requires a careful balance between accessibility and security. Developers must consider various strategies to ensure that these keys are not hard-coded into their applications or exposed in plain text. Instead, best practices involve utilizing Docker’s built-in features, such as environment variables and Docker secrets, which provide a more secure way to manage sensitive information. Additionally, understanding the implications of image layers and container orchestration can further enhance the security posture of your applications.
As we delve deeper into the topic, we will explore practical approaches to securely storing API keys in Docker containers, highlighting common pitfalls and offering solutions that align with industry standards. By implementing these strategies, developers can confidently deploy their applications, knowing that their API keys are protected from unauthorized access and potential breaches.
Environment Variables
Storing API keys as environment variables is one of the most common and secure methods when working within Docker containers. By using environment variables, sensitive information is kept out of your codebase, which reduces the risk of accidental exposure.
To use environment variables in a Docker container, you can define them in your `Dockerfile` or pass them at runtime using the `docker run` command. Here’s how you can do it:
- Defining in Dockerfile:
“`Dockerfile
ENV API_KEY=your_api_key_here
“`
- Passing at Runtime:
“`bash
docker run -e API_KEY=your_api_key_here your_image
“`
This method allows you to access the API key within your application using the standard environment variable access methods provided by your programming language.
Docker Secrets
For applications that require enhanced security, particularly in production environments, Docker Secrets is an excellent choice. Docker Secrets are specifically designed to manage sensitive data, providing a more secure alternative to environment variables.
To utilize Docker Secrets, follow these steps:
- Create a Secret:
“`bash
echo “your_api_key_here” | docker secret create api_key –
“`
- Deploy a Service with the Secret:
“`bash
docker service create –name your_service –secret api_key your_image
“`
- Access the Secret in the Container:
Secrets are stored in `/run/secrets/` by default. Your application can read the key as follows:
“`bash
cat /run/secrets/api_key
“`
The use of Docker Secrets ensures that your API keys are only accessible to the services that need them, minimizing the risk of exposure.
Configuration Files
Another method to store API keys is by using configuration files. This approach can be useful when managing multiple keys or related configurations. However, care must be taken to ensure that these files are not included in version control systems like Git.
To implement this method:
- Create a configuration file (e.g., `config.env`):
“`plaintext
API_KEY=your_api_key_here
“`
- Mount the configuration file during container runtime:
“`bash
docker run –env-file=config.env your_image
“`
This allows your application to load the API key from the configuration file while keeping it out of the codebase.
Best Practices
When storing API keys in Docker containers, adhere to the following best practices:
- Avoid Hardcoding: Never hardcode API keys directly in your application code.
- Use .gitignore: Ensure that configuration files containing sensitive information are listed in `.gitignore`.
- Regularly Rotate Keys: Implement a process for regularly updating and rotating your API keys to limit exposure.
- Limit Permissions: Apply the principle of least privilege by limiting access to secrets and environment variables only to the services that require them.
Method | Security Level | Ease of Use |
---|---|---|
Environment Variables | Moderate | Easy |
Docker Secrets | High | Moderate |
Configuration Files | Moderate | Easy |
By following these guidelines, you can effectively manage and secure your API keys while using Docker containers.
Environment Variables
Storing API keys as environment variables is one of the most common practices when working with Docker containers. This method allows you to separate sensitive data from your codebase, reducing the risk of accidental exposure.
- Setting Environment Variables:
- You can define environment variables directly in the Dockerfile:
“`Dockerfile
ENV API_KEY=your_api_key_here
“`
- Alternatively, you can pass them at runtime using the `-e` flag:
“`bash
docker run -e API_KEY=your_api_key_here your_image
“`
- Using an `.env` File:
- You can create a `.env` file that contains your environment variables:
“`
API_KEY=your_api_key_here
“`
- Reference the `.env` file in your Docker Compose file:
“`yaml
version: ‘3’
services:
app:
image: your_image
env_file:
- .env
“`
Docker Secrets
Docker Secrets is a more secure way to handle sensitive information, particularly in swarm mode. This allows you to manage API keys without exposing them in your code or environment variables.
- Creating a Secret:
“`bash
echo “your_api_key_here” | docker secret create api_key –
“`
- Using Secrets in a Service:
- Update your Docker Compose file to use secrets:
“`yaml
version: ‘3.1’
services:
app:
image: your_image
secrets:
- api_key
secrets:
api_key:
external: true
“`
- Accessing Secrets in Your Application:
- Secrets are available in `/run/secrets/
` within the container. For example:
“`bash
cat /run/secrets/api_key
“`
Docker Configs
Docker Configs are similar to Docker Secrets but are designed for non-sensitive data. They can be used to store configuration files or other non-sensitive keys.
- Creating a Config:
“`bash
echo “your_config_data” | docker config create config_name –
“`
- Using Configs in a Service:
- Reference the config in your Docker Compose file:
“`yaml
version: ‘3.1’
services:
app:
image: your_image
configs:
- config_name
configs:
config_name:
external: true
“`
Volume Mounts
Using volume mounts can help to manage API keys stored in files securely. This approach allows you to keep sensitive information out of the container’s filesystem.
– **Creating a Volume**:
- Create a volume that includes your API key file:
“`bash
docker volume create api_keys
“`
– **Mounting the Volume**:
- Mount the volume when running your container:
“`bash
docker run -v api_keys:/path/to/keys your_image
“`
– **Accessing the API Key**:
- Store your API key in a file within the volume:
“`bash
echo “your_api_key_here” > /path/to/keys/api_key.txt
“`
Best Practices
Implementing the following best practices can further enhance the security of your API keys in Docker containers:
- Limit Permissions: Ensure that only the necessary services have access to the API keys.
- Regularly Rotate Keys: Periodically change your API keys to minimize the risk of unauthorized access.
- Use a Secrets Management Tool: Consider using tools like HashiCorp Vault or AWS Secrets Manager for enhanced security and management capabilities.
- Avoid Hardcoding: Never hardcode sensitive information directly into your application code or Dockerfiles.
By adhering to these practices, you can effectively manage API keys within your Docker container environment.
Best Practices for Storing API Keys in Docker Containers
Dr. Emily Carter (Cloud Security Architect, SecureCloud Solutions). “The most effective way to store API keys in a Docker container is to utilize Docker secrets. This method ensures that sensitive information is encrypted and only accessible to the services that require it, minimizing the risk of exposure.”
James Liu (DevOps Engineer, Tech Innovations Inc.). “Environment variables are a common practice for storing API keys, but they can be exposed in logs or through process listings. Instead, consider using a dedicated secrets management tool, such as HashiCorp Vault, to securely manage and inject API keys into your Docker containers.”
Sarah Thompson (Software Security Consultant, CodeGuardians). “It is crucial to avoid hardcoding API keys within your Docker images. Instead, use a multi-stage build process to keep sensitive information out of the final image. This approach not only enhances security but also keeps your images clean and efficient.”
Frequently Asked Questions (FAQs)
What are API keys and why are they important?
API keys are unique identifiers used to authenticate requests made to an API. They are crucial for controlling access, monitoring usage, and securing sensitive data.
What is the best practice for storing API keys in a Docker container?
The best practice is to use environment variables or Docker secrets to store API keys. This approach prevents hardcoding sensitive information directly into the application code or Docker images.
How can I use environment variables to store API keys in Docker?
You can define environment variables in your Dockerfile using the `ENV` instruction or pass them at runtime using the `-e` flag with `docker run`. This keeps the keys separate from your codebase.
What are Docker secrets and how do they enhance security for API keys?
Docker secrets are a secure way to manage sensitive data, such as API keys, in a Docker Swarm environment. They are encrypted and only accessible to services that need them, reducing the risk of exposure.
Can I use a .env file to store API keys for Docker containers?
Yes, you can use a `.env` file to define environment variables for your Docker containers. Use the `–env-file` option with `docker run` to load the variables from the file, ensuring they are not included in your source code.
What should I avoid when storing API keys in Docker containers?
Avoid hardcoding API keys in your Dockerfile, application code, or version control systems. Also, do not expose them in logs or error messages, as this can lead to unauthorized access.
In summary, securely storing API keys in a Docker container is crucial for maintaining the integrity and security of your applications. Best practices include utilizing environment variables, Docker secrets, and external configuration management tools. Each method has its own advantages, but the choice largely depends on the specific requirements of your application and deployment environment.
Environment variables provide a straightforward way to inject sensitive information into your container at runtime, but they can be less secure if not managed properly. Docker secrets, on the other hand, offer a more secure solution by encrypting sensitive data and restricting access to it within the Docker swarm. For larger applications, integrating external configuration management tools like HashiCorp Vault can provide enhanced security and flexibility in managing API keys and other sensitive information.
Ultimately, the key takeaway is to prioritize security when handling API keys in Docker containers. Employing a combination of these methods can help mitigate risks associated with exposing sensitive information. Regularly reviewing and updating your security practices is also essential to adapt to evolving threats and ensure the ongoing protection of your applications.
Author Profile
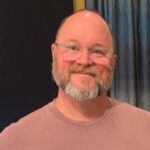
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?