Why Is There a Question Mark After a Type in C#?
In the world of C, the question mark is more than just a punctuation mark; it holds significant meaning in the realm of programming. If you’ve ever encountered a type followed by a question mark, you might have wondered what it signifies and how it can impact your code. This seemingly simple notation can unlock a wealth of functionality and flexibility in your applications, particularly when dealing with data types that can have a null value. As we delve deeper into this topic, you’ll uncover the nuances of nullable types and their practical applications in Cprogramming.
At its core, the question mark after a type in Cindicates that the type is nullable, allowing it to represent all the values of its underlying type, plus an additional null value. This feature is particularly useful when working with databases or any scenario where a value may not be present. By embracing nullable types, developers can write more robust and error-resistant code, reducing the chances of encountering null reference exceptions that can lead to runtime errors.
Understanding how to effectively use the question mark in Ccan enhance your programming skills and improve the overall quality of your applications. As we explore this topic further, we will examine the syntax, benefits, and potential pitfalls of nullable types, providing you with the knowledge to leverage this powerful feature in your own projects
Understanding Nullable Types
In C, the question mark (`?`) after a type indicates that the variable can hold a null value in addition to its usual range of values. This is particularly useful when dealing with value types, which typically cannot be null. By declaring a type as nullable, you enhance its flexibility in scenarios such as database operations where a field might not have a value.
For example:
“`csharp
int? nullableInt = null;
“`
Here, `nullableInt` can either hold an integer value or be null.
Benefits of Using Nullable Types
Nullable types offer several advantages, particularly in database applications and business logic where the absence of a value is meaningful. Some key benefits include:
- Database Compatibility: They align well with database fields that allow nulls.
- Conditional Logic: You can check if a value exists before performing operations, thus reducing the risk of exceptions.
- Improved Code Clarity: Nullable types can make your intentions clearer in the code, indicating that a value might be absent.
How to Work with Nullable Types
To work with nullable types effectively, Cprovides several methods and properties. Here are some of the primary methods:
- HasValue: A property that returns true if the nullable type has a value.
- Value: A property that retrieves the value of the nullable type, throwing an exception if it is null.
- GetValueOrDefault(): Returns the value if it exists; otherwise, it returns the default value of the underlying type.
Example usage:
“`csharp
int? number = null;
if (number.HasValue)
{
Console.WriteLine(number.Value);
}
else
{
Console.WriteLine(“No value present.”);
}
“`
Nullable Types in Collections
When using nullable types in collections, special attention is required to handle null values appropriately. For example, a list of nullable integers can be declared as follows:
“`csharp
List
“`
You can iterate through this list and handle the nulls using LINQ or traditional loops.
Value | Is Null? |
---|---|
1 | No |
null | Yes |
3 | No |
null | Yes |
5 | No |
In this table, you can see how nullable integers can be managed within a collection, and how the presence or absence of values can be tracked effectively.
Understanding the CQuestion Mark Syntax
In C, the question mark (`?`) serves multiple purposes depending on its context, primarily indicating nullable types and conditional operations. Below are the key usages of the question mark in C.
Nullable Types
A nullable type is a value type that can represent all the values of its underlying type plus an additional `null` value. This is particularly useful for database interactions where a field may not have a value.
- Syntax: The syntax for declaring a nullable type involves appending a question mark to the type name.
“`csharp
int? nullableInt = null;
“`
- Usage: Nullable types can be particularly beneficial in scenarios such as:
- Database fields that may not have a value.
- Optional parameters in methods.
- Avoiding exceptions when dealing with uninitialized value types.
Checking for Null Values
When working with nullable types, it is essential to check for null values to avoid runtime exceptions.
- HasValue Property: This property checks if the nullable type contains a value.
“`csharp
if (nullableInt.HasValue)
{
Console.WriteLine(nullableInt.Value);
}
“`
- Null Coalescing Operator: The null coalescing operator (`??`) provides a default value if the nullable type is null.
“`csharp
int value = nullableInt ?? 0; // value will be 0 if nullableInt is null
“`
Ternary Conditional Operator
The question mark is also used in the ternary conditional operator, which is a shorthand way of performing conditional checks.
- Syntax: The ternary operator has the following syntax:
“`csharp
condition ? valueIfTrue : valueIf;
“`
- Example:
“`csharp
int result = (nullableInt.HasValue) ? nullableInt.Value : -1;
“`
This example assigns `nullableInt.Value` to `result` if `nullableInt` has a value; otherwise, it assigns `-1`.
Combining Nullable Types and Ternary Operator
Using both nullable types and the ternary operator can streamline code that involves conditional logic based on the presence of values.
- Example:
“`csharp
string message = nullableInt.HasValue ? $”Value: {nullableInt.Value}” : “No value provided”;
“`
This approach enhances code readability while efficiently handling potential null values.
Practical Considerations
When utilizing the question mark in C, consider the following best practices:
- Readability: Ensure that the use of nullable types and ternary operations enhances code clarity.
- Error Handling: Always check for null values before accessing the properties of nullable types to prevent exceptions.
- Performance: While nullable types can simplify code, be mindful of potential performance implications in scenarios with extensive use.
Summary of Key Points
Usage | Description | Example |
---|---|---|
Nullable Types | Represents value types that can be null | `int? nullableInt = null;` |
HasValue Property | Checks if a nullable type has a value | `nullableInt.HasValue` |
Null Coalescing Operator | Provides a default value for null types | `int value = nullableInt ?? 0;` |
Ternary Operator | Shorthand for conditional logic | `result = condition ? trueVal : Val;` |
Utilizing the question mark in Cenhances the flexibility and robustness of code while managing nullability and conditional logic effectively.
Understanding the CQuestion Mark Syntax
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The question mark in Cindicates a nullable type, allowing developers to assign null values to value types. This feature significantly enhances the flexibility of data handling in applications, especially when dealing with databases where null values are common.
Mark Thompson (CLanguage Specialist, CodeCraft Academy). Utilizing the question mark after a type not only simplifies the code but also improves readability. It signals to other developers that the variable can hold a null value, which is crucial for preventing runtime exceptions related to null references.
Linda Garcia (Technical Author, Programming Insights). The of nullable types in Chas transformed how developers approach data validation. By explicitly using the question mark, programmers can implement more robust error handling strategies, ultimately leading to more stable applications.
Frequently Asked Questions (FAQs)
What does the question mark after a type in Csignify?
The question mark indicates that the type is a nullable type, allowing it to represent all the values of its underlying type plus an additional null value.
How do I declare a nullable type in C?
You can declare a nullable type by appending a question mark to the type name, for example, `int? myNullableInt = null;`.
What are the benefits of using nullable types in C?
Nullable types provide a way to represent the absence of a value, which is particularly useful in database operations, optional parameters, and when dealing with data that may not always be present.
Can I use nullable types with value types only?
Yes, nullable types can only be used with value types, such as `int`, `double`, `bool`, etc. Reference types inherently support null values.
How do I check if a nullable type has a value in C?
You can check if a nullable type has a value by using the `HasValue` property or by comparing it to `null`, for example, `if (myNullableInt.HasValue)` or `if (myNullableInt != null)`.
What happens if I try to access the value of a nullable type that is null?
Accessing the value of a nullable type that is null will result in an `InvalidOperationException`. It is essential to check for null before accessing the value using the `Value` property.
The question mark in Cserves as a vital component in defining nullable types, enhancing the language’s flexibility in handling data. By appending a question mark to a value type, such as `int?` or `bool?`, developers can indicate that the variable may hold a null value. This feature is particularly useful in scenarios where a variable may not have a value assigned, allowing for more robust error handling and data validation in applications.
Additionally, the question mark operator is instrumental in simplifying null checks and conditional operations. The null-conditional operator (`?.`) allows developers to safely access members of an object that might be null, thereby reducing the risk of null reference exceptions. This operator streamlines code and improves readability, making it easier to manage complex data structures and relationships.
In summary, the use of the question mark in Cfor nullable types and null-conditional operations represents a significant advancement in type safety and code clarity. By leveraging these features, developers can write more resilient and maintainable code, ultimately leading to improved application performance and user experience. Understanding and effectively utilizing the question mark in Cis essential for modern software development practices.
Author Profile
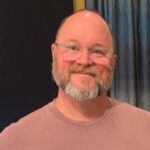
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?