What Does ‘Expression Must Be A Modifiable Lvalue’ Mean in Programming?
### Introduction
In the world of programming, encountering errors is a rite of passage that every developer faces. Among the myriad of cryptic messages that can appear during code compilation or execution, few are as perplexing as the “Expression Must Be A Modifiable Lvalue” error. This seemingly innocuous phrase can halt your progress, leaving you scratching your head and questioning the very structure of your code. But fear not! Understanding this error is not just about fixing a bug; it’s an opportunity to deepen your grasp of variable handling, memory management, and the nuances of the programming language you are using.
This article delves into the intricacies of what constitutes a modifiable lvalue and why this error arises. We will explore the fundamental concepts behind lvalues and rvalues, shedding light on how they interact within the context of assignments and expressions. By dissecting common scenarios that trigger this error, we aim to equip you with the knowledge to not only resolve the issue but also to prevent it from cropping up in the future. Whether you are a novice programmer or a seasoned developer, understanding this concept will enhance your coding prowess and bolster your confidence in tackling similar challenges.
Join us as we navigate the labyrinth of variable types and expressions, illuminating the path to clearer, more effective
Understanding the Error
The error message “Expression must be a modifiable lvalue” typically arises in programming languages like C, C++, and Java when an attempt is made to assign a value to a non-modifiable or immutable object. An lvalue (locator value) refers to an object that occupies some identifiable location in memory (i.e., it has an address). In contrast, an rvalue (read value) refers to a temporary value that does not persist beyond the expression that uses it.
This error most commonly occurs in the following scenarios:
- Attempting to assign a value to a constant.
- Trying to modify a temporary object or literal.
- Using the result of a function that returns a non-modifiable type.
Examples of Non-Modifiable Lvalues
Consider the following examples to illustrate situations that lead to the “Expression must be a modifiable lvalue” error:
c
const int x = 10;
x = 20; // Error: x is a constant and cannot be modified.
int* p = NULL;
*p = 5; // Error: p is a null pointer and dereferencing it is invalid.
int getValue() {
return 10; // Returns a temporary value
}
getValue() = 20; // Error: Cannot assign to a temporary rvalue.
Common Scenarios Leading to the Error
To effectively troubleshoot the “Expression must be a modifiable lvalue” error, it’s important to recognize common coding patterns that can trigger it. Below are a few of those patterns:
- Constants: Attempting to assign values to constants declared with `const`.
- Function Return Values: Trying to assign a value to the return of a function that returns a value rather than a reference.
- Array Elements: Incorrectly trying to modify the size or type of an array during assignment.
- Dereferencing Null or Invalid Pointers: Attempting to assign a value through a pointer that points to `NULL` or an invalid memory address.
Scenario | Example Code | Explanation |
---|---|---|
Assigning to a constant | `const int a = 5; a = 10;` | `a` cannot be modified as it is declared with `const`. |
Modifying a temporary value | `getValue() = 15;` | The function returns a temporary rvalue, which cannot be modified. |
Dereferencing a null pointer | `int* ptr = NULL; *ptr = 5;` | `ptr` is null; dereferencing it results in an error. |
Resolving the Error
To resolve the “Expression must be a modifiable lvalue” error, consider the following steps:
- Check for Constants: Ensure that the variable you are trying to modify is not declared with `const`.
- Return References: If a function needs to allow modification, consider returning a reference or a pointer instead of a value.
- Validate Pointers: Always check pointers for validity before dereferencing and modifying their pointed value.
- Use Valid Lvalues: Ensure that the left side of the assignment is a valid lvalue that can be modified.
By understanding and applying these concepts, developers can effectively troubleshoot and resolve the “Expression must be a modifiable lvalue” error in their code.
Understanding Modifiable Lvalues
A modifiable lvalue refers to an expression that can be assigned a new value. In programming languages such as C and C++, understanding the concept of lvalues and rvalues is crucial for managing memory and data effectively. The error message “Expression must be a modifiable lvalue” typically occurs when an attempt is made to assign a value to a non-modifiable expression.
### Characteristics of Modifiable Lvalues
- Memory Addressable: Modifiable lvalues are variables that can be stored in a specific memory location.
- Assignable: They can receive new values through assignment operations.
- Examples: Variables, array elements, dereferenced pointers.
### Non-Modifiable Lvalues
Certain expressions cannot be assigned new values, leading to the aforementioned error. Examples include:
- Constants: Numeric literals (e.g., `5`, `3.14`) cannot be assigned values.
- Temporary Objects: Results of expressions such as `x + y` are not stored in a memory location that can be modified.
- Function Returns: If a function returns a value by value instead of by reference, the returned value cannot be modified.
### Common Causes of the Error
- Assigning to a Constant
c
const int x = 10;
x = 20; // Error: Expression must be a modifiable lvalue
- Using a Temporary Value
c
int a = 5;
(a + 2) = 10; // Error: Expression must be a modifiable lvalue
- Function Return Values
c
int getValue() {
return 42;
}
getValue() = 100; // Error: Expression must be a modifiable lvalue
### How to Resolve the Error
To resolve this error, ensure that any assignment is made to a modifiable lvalue. Here are some strategies:
- Use Variables Instead of Constants
Always assign values to variables that are declared as modifiable.
- Store Results in Variables
Instead of assigning directly to a temporary result, store the result in a variable first.
c
int result = a + 2;
result = 10; // Correct usage
- Return by Reference
If a function needs to provide a modifiable value, consider returning a reference instead.
c
int& getRefValue(int& ref) {
return ref;
}
int x = 5;
getRefValue(x) = 10; // Correct usage
### Summary Table of Modifiable vs Non-Modifiable Lvalues
Type | Modifiable Lvalue | Non-Modifiable Lvalue |
---|---|---|
Variables | `int x; x = 5;` | `const int y = 10; y = 20;` |
Array Elements | `arr[0] = 5;` | `int arr[5]; 5 = arr[0];` |
Pointers | `int* ptr; *ptr = 20;` | `int* p = &x; 10 = *p;` |
Function Return Values | `int& getRef(); getRef() = 100;` | `int getValue(); getValue() = 100;` |
By adhering to the guidelines for proper assignment and understanding the principles of lvalues and rvalues, developers can prevent the “Expression must be a modifiable lvalue” error and write more robust code.
Understanding the ‘Expression Must Be A Modifiable Lvalue’ Error
Dr. Emily Carter (Senior Software Engineer, CodeSafe Technologies). “The ‘Expression Must Be A Modifiable Lvalue’ error typically arises in programming languages like C and C++. It indicates that the expression you are trying to modify is not a variable that can be changed, such as a constant or a temporary value. Understanding the context of lvalues and rvalues is crucial for debugging this issue effectively.”
Michael Chen (Lead Developer, Tech Innovations Inc.). “This error serves as a reminder to developers about the importance of variable types and their mutability. When you encounter this message, it is essential to review your code to ensure that you are not attempting to assign a new value to an immutable expression, such as a literal or a function return that does not yield a modifiable reference.”
Sarah Thompson (Programming Language Researcher, Future Code Labs). “In many cases, resolving the ‘Expression Must Be A Modifiable Lvalue’ error involves re-evaluating how you structure your expressions. By ensuring that your assignments target valid lvalues, you can maintain code integrity and avoid common pitfalls associated with improper value assignments.”
Frequently Asked Questions (FAQs)
What does “Expression Must Be A Modifiable Lvalue” mean?
This error indicates that the expression you are trying to assign a value to is not a modifiable lvalue, meaning it cannot be assigned a new value. An lvalue refers to an object that occupies identifiable location in memory, while an rvalue is a temporary value that does not have a persistent memory location.
What are common causes of this error?
Common causes include attempting to assign a value to a constant, using a literal value on the left side of an assignment, or trying to modify the return value of a function that returns a non-reference type.
How can I fix this error in my code?
To resolve this error, ensure that you are assigning a value to a valid lvalue, such as a variable that is not declared as `const`. Check that you are not trying to assign values to literals or temporary results.
Can this error occur in both C and C++?
Yes, this error can occur in both C and C++. The rules regarding lvalues and rvalues are similar in both languages, and the error will arise when attempting to assign to a non-modifiable expression.
What is an example of an expression that would trigger this error?
An example would be attempting to execute the following code: `5 = x;`. Here, `5` is a literal and cannot be modified, leading to the “Expression Must Be A Modifiable Lvalue” error.
Are there any tools or methods to help identify this error in my code?
Using modern Integrated Development Environments (IDEs) or compilers with static analysis tools can help identify this error. Enabling compiler warnings can also provide insights into potential issues related to lvalues and rvalues.
The phrase “Expression Must Be A Modifiable Lvalue” primarily pertains to programming languages, particularly in the context of C and C++. An lvalue refers to an expression that points to a memory location and allows us to take the address of that location. In contrast, an rvalue is a temporary value that does not persist beyond the expression that uses it. When a compiler generates an error stating that an expression must be a modifiable lvalue, it indicates that the code is attempting to assign a value to an expression that cannot be modified, such as a constant or a temporary result.
This error often arises in scenarios where developers mistakenly attempt to assign a value to a literal, a constant, or the result of a function that returns a non-reference type. Understanding the distinction between lvalues and rvalues is crucial for effective programming and debugging. It is essential for developers to ensure that they are working with modifiable lvalues when performing assignments to avoid this common pitfall.
In summary, the key takeaway is that recognizing and correctly utilizing lvalues is fundamental in programming. Developers should be vigilant about the types of expressions they are working with and ensure that assignments are made to valid, modifiable lvalues. By doing so, they can prevent
Author Profile
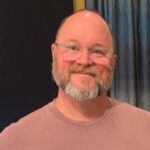
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?