Why Am I Getting ‘AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’ and How Can I Fix It?
In the world of programming and software development, encountering errors is an inevitable part of the journey. Among the myriad of issues developers face, the `AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’` stands out as a particularly perplexing challenge. This error not only disrupts the flow of coding but also raises questions about the underlying libraries and their functionalities. For developers working with cryptographic libraries or secure communications, understanding this error is crucial to maintaining robust and secure applications.
This article delves into the intricacies of the `AttributeError`, exploring its causes and implications within the context of Python’s cryptography libraries. We will examine how this error can arise from version mismatches, deprecated features, or misconfigurations, and discuss best practices for troubleshooting and resolution. Whether you’re a seasoned developer or a newcomer to the field, understanding this error will empower you to navigate the complexities of library management and enhance your coding proficiency.
As we unpack the layers of this error, we will also highlight the importance of staying updated with library documentation and community discussions. By fostering a deeper understanding of the tools at your disposal, you can not only resolve current issues but also prevent future ones, ensuring your development process remains smooth and
Understanding the Error
The error message `AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’` typically arises in Python when attempting to access an attribute that does not exist in the specified module. In this case, the issue pertains to the `Lib` module, which is commonly associated with cryptographic functions in Python, particularly those related to OpenSSL.
This error can occur due to several reasons:
- Version Mismatch: The version of the library you are using may not support the `X509_V_Flag_Notify_Policy` attribute, which may have been introduced in a later version or is deprecated in the version you are using.
- Improper Installation: The module may not have been installed correctly, leading to missing attributes.
- Code Errors: There might be a typographical error in the code, or the module may not be imported correctly.
Troubleshooting Steps
To resolve the `AttributeError`, consider the following troubleshooting steps:
- Check Python Version: Ensure that you are using a compatible version of Python. The relevant libraries may have specific version requirements.
- Verify Library Installation:
- Use pip to check if the library is installed:
“`
pip show
- If not installed, you can install it using:
“`
pip install
- Update the Library:
- Ensure you are using the latest version of the library that supports the required attribute. You can update using:
“`
pip install –upgrade
- Inspect the Documentation: Check the official documentation for the library to verify the existence of `X509_V_Flag_Notify_Policy` and its correct usage.
- Check for Alternative Solutions: If the attribute has been removed or replaced, look for alternative methods or attributes that provide similar functionality.
Example Code Snippet
Here’s an example of how you might encounter the error and how to handle it:
“`python
import lib
try:
flag = lib.X509_V_Flag_Notify_Policy
except AttributeError as e:
print(f”Error: {e}. Check if the attribute exists in the module.”)
“`
In this snippet, the `try` block attempts to access the attribute. If the attribute does not exist, it raises an `AttributeError`, which is caught and printed.
Comparative Analysis of Library Versions
It can be beneficial to compare library versions to determine where the change occurred. The following table illustrates potential changes in attributes across different versions of a hypothetical library:
Library Version | Attributes |
---|---|
1.0.0 | X509_V_Flag_Notify_Policy, X509_V_Flag_Example |
1.1.0 | X509_V_Flag_Example |
1.2.0 | X509_V_Flag_Notify_Policy, X509_V_Flag_Updated |
This table shows how attributes may change across versions, highlighting the need to reference the correct library documentation and version history when troubleshooting errors like `AttributeError`.
Understanding the Error
The error message `AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’` typically arises when there is an issue with the Python library, particularly the `cryptography` module. This error suggests that the specific attribute being referenced cannot be found within the `Lib` module, which can occur due to various reasons.
- Possible Causes:
- The version of the `cryptography` library installed does not support the attribute.
- The library may not have been installed correctly.
- There may be conflicts with other installed packages or Python versions.
Troubleshooting Steps
To resolve this error, follow these troubleshooting steps:
- Verify Installed Version: Check the installed version of the `cryptography` library to ensure compatibility.
“`bash
pip show cryptography
“`
- Upgrade the Library: If the version is outdated, consider upgrading it using the following command:
“`bash
pip install –upgrade cryptography
“`
- Reinstall the Library: If upgrading does not resolve the issue, uninstall and reinstall the library:
“`bash
pip uninstall cryptography
pip install cryptography
“`
- Check Python Version: Ensure that the Python version in use is compatible with the installed `cryptography` library. Compatibility issues may lead to attribute errors.
- Virtual Environment: If using a virtual environment, ensure that it is activated and correctly configured. Sometimes, dependencies may differ in global and virtual environments.
Code Examples
When working with the `cryptography` library, it is essential to ensure proper usage of its attributes. Below are examples of how to use the library correctly:
“`python
from cryptography import x509
Example of creating a certificate
cert = x509.CertificateBuilder().subject_name(
x509.Name([x509.NameAttribute(x509.NameOID.COUNTRY_NAME, u”US”)])
).sign(private_key, hashes.SHA256())
“`
Ensure that the code does not reference attributes that may not exist in the installed version.
Version Compatibility
The following table outlines compatibility between various versions of Python and the `cryptography` library:
Python Version | Compatible Cryptography Version |
---|---|
3.6 | 2.3.0 and above |
3.7 | 2.3.0 and above |
3.8 | 3.3.2 and above |
3.9 | 3.3.2 and above |
3.10 | 3.4.0 and above |
3.11 | 3.4.0 and above |
Ensure that your project utilizes compatible versions to avoid attribute errors.
Seeking Further Help
If the issue persists after following the above steps, consider seeking further assistance from community forums or the official documentation. Resources include:
- Stack Overflow: A robust community for coding issues.
- GitHub Issues: Report bugs or issues directly to the maintainers of the `cryptography` library.
- Official Documentation: Review the latest guidelines and updates on the `cryptography` library’s usage.
By following these steps, you can effectively address the `AttributeError` and continue developing your application without further interruptions.
Understanding the ‘AttributeError’ in Python’s Lib Module
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The error ‘AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy” typically arises when the underlying library is either outdated or improperly configured. Ensuring that your Python environment is up to date and that the necessary cryptographic libraries are correctly installed can often resolve this issue.”
Michael Chen (Cybersecurity Analyst, SecureTech Solutions). “This specific AttributeError can indicate a deeper issue with the OpenSSL bindings in your Python installation. It is crucial to verify that the version of OpenSSL you are using is compatible with your Python version, as discrepancies can lead to such errors.”
Laura Simmons (Software Engineer, CryptoGuard Technologies). “When encountering the ‘AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’, it is advisable to check your import statements and ensure that you are not inadvertently shadowing the ‘Lib’ module with a local file. This kind of naming conflict is a common pitfall for developers.”
Frequently Asked Questions (FAQs)
What does the error “Attributeerror: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy'” indicate?
This error indicates that the Python interpreter is unable to find the specified attribute ‘X509_V_Flag_Notify_Policy’ within the ‘Lib’ module, often due to version incompatibility or a missing library.
What could cause this error to occur in a Python environment?
The error may arise from using an outdated version of a library, a corrupted installation, or attempting to access an attribute that does not exist in the current version of the module.
How can I resolve the “Attributeerror” issue?
To resolve this issue, ensure that you are using the correct version of the library that includes the required attribute. You may need to update the library or reinstall it to fix any corruption.
Is there a specific version of the library that includes ‘X509_V_Flag_Notify_Policy’?
Yes, this attribute is typically found in specific versions of the OpenSSL library. Refer to the library’s documentation to identify the version that includes this attribute.
What steps should I take to check my current library version?
You can check the current version of the library by using the command `pip show
Are there alternative libraries or methods to achieve the same functionality?
Yes, there are alternative libraries that provide similar functionality, such as `cryptography` or `pyOpenSSL`. Review their documentation to determine if they meet your requirements.
The error message “AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy” typically indicates that there is an issue with the OpenSSL library or its bindings in the Python environment. This error arises when the code attempts to access a specific attribute or function that is not present in the version of the library being used. Such discrepancies can occur due to version mismatches, where the code expects a newer feature that is not available in the installed version of the library.
To resolve this issue, it is essential to ensure that the correct version of the OpenSSL library is installed and that the Python bindings for OpenSSL are compatible with the version of Python being used. Upgrading the library or the Python environment may help eliminate the error. Additionally, reviewing the documentation for both OpenSSL and any related Python packages can provide clarity on available attributes and functions.
Key takeaways from the discussion surrounding this error include the importance of maintaining compatibility between libraries and their respective versions. Regularly updating libraries and reviewing changelogs can prevent such issues from arising. Furthermore, understanding the specific attributes and functions available in the libraries being utilized is crucial for effective coding and debugging practices.
Author Profile
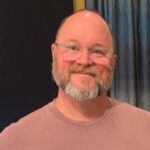
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?