How Can You Run a Bat File in PowerShell Effectively?
In the world of Windows operating systems, automation and efficiency are paramount, especially for developers and system administrators. One of the most versatile tools at your disposal is the batch file, a simple yet powerful script that can execute a series of commands in a sequential manner. However, as technology evolves, so do the methods we use to run these scripts. Enter PowerShell, a more advanced command-line shell and scripting language that offers enhanced capabilities for managing and automating tasks. In this article, we will explore how to seamlessly run batch files within PowerShell, unlocking a new level of functionality and control over your scripts.
PowerShell has become the go-to solution for many users seeking to streamline their workflows. While batch files have been a staple for automation in Windows for decades, integrating them with PowerShell can elevate your scripting game. By leveraging PowerShell’s robust features, you can not only execute batch files but also harness the power of PowerShell’s advanced scripting capabilities, error handling, and output management. This combination allows for a more sophisticated approach to task automation, making it easier to manage complex processes.
As we delve deeper into the topic, we will discuss the various methods to execute batch files in PowerShell, highlighting the benefits and potential pitfalls of each approach. Whether
Executing a Batch File in PowerShell
To run a batch file in PowerShell, you can use several methods, each with its own advantages depending on your specific requirements. Below are the primary methods for executing a batch file.
Using the Call Command
The `call` command is a straightforward way to execute a batch file from PowerShell. This method is particularly useful when you want to run a batch file and then return to the PowerShell prompt without terminating the session.
“`powershell
call “C:\path\to\your\script.bat”
“`
Ensure that the path to the batch file is enclosed in double quotes, especially if it contains spaces.
Using the Start-Process Cmdlet
The `Start-Process` cmdlet allows you to run a batch file in a new process. This is beneficial when you want to run the batch file independently of the PowerShell session.
“`powershell
Start-Process “C:\path\to\your\script.bat”
“`
You can also specify additional parameters, such as `-NoNewWindow` to run it in the same window or `-Wait` to wait for the process to complete before returning control to PowerShell.
Using the & (Call Operator)
The call operator `&` can also be employed to execute batch files. This is a flexible method that allows you to run any executable file, including batch files.
“`powershell
& “C:\path\to\your\script.bat”
“`
This approach is particularly useful for running scripts that may require parameters or when you want to execute them in the context of the current PowerShell session.
Handling Execution Policies
When running scripts in PowerShell, you may encounter execution policies that restrict script execution for security reasons. To check the current execution policy, use:
“`powershell
Get-ExecutionPolicy
“`
If needed, you can change the execution policy using:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
This command allows the execution of scripts downloaded from the internet, provided they are signed by a trusted publisher.
Common Issues and Troubleshooting
When executing batch files in PowerShell, you may face some common issues:
- File Not Found: Ensure the file path is correct and that the batch file exists at the specified location.
- Permission Denied: You may need to run PowerShell as an administrator if the batch file requires elevated privileges.
- Execution Policy Restrictions: If scripts are blocked, adjust the execution policy as mentioned earlier.
Batch File Execution Summary
The following table summarizes the different methods for executing batch files in PowerShell:
Method | Syntax | Notes |
---|---|---|
Call Command | call “C:\path\to\your\script.bat” | Returns to PowerShell prompt after execution. |
Start-Process Cmdlet | Start-Process “C:\path\to\your\script.bat” | Runs in a new process; can specify parameters. |
Call Operator | & “C:\path\to\your\script.bat” | Runs in the current session; can accept parameters. |
By utilizing these methods, you can effectively run batch files within PowerShell, adapting the approach to suit your operational needs.
Running a Batch File in PowerShell
To execute a batch file within PowerShell, you can use a straightforward command that allows the script to run seamlessly. Here are the methods you can utilize:
Method 1: Direct Execution
Simply navigate to the directory containing the batch file and call it directly. Follow these steps:
- Open PowerShell.
- Use the `cd` command to change to the directory where the batch file is located:
“`powershell
cd “C:\Path\To\Your\BatchFile”
“`
- Execute the batch file by typing its name:
“`powershell
.\YourBatchFile.bat
“`
Method 2: Using the Start-Process Cmdlet
The `Start-Process` cmdlet provides enhanced control over the execution of batch files. This method is particularly useful when you want to run the batch file in a new window or with specific parameters.
Example command:
“`powershell
Start-Process “C:\Path\To\Your\BatchFile\YourBatchFile.bat”
“`
You can also pass arguments to the batch file:
“`powershell
Start-Process “C:\Path\To\Your\BatchFile\YourBatchFile.bat” -ArgumentList “arg1”, “arg2”
“`
Method 3: Using the Call Operator
The call operator (`&`) is another way to run a batch file. This method is simple and effective for executing scripts without changing the current directory context.
Example:
“`powershell
& “C:\Path\To\Your\BatchFile\YourBatchFile.bat”
“`
Handling Execution Policies
Before running scripts, ensure your PowerShell execution policy allows the running of scripts. You can check and modify the policy as follows:
- Check the current execution policy:
“`powershell
Get-ExecutionPolicy
“`
- To set the policy to allow script execution, use:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
Common Considerations
When running batch files in PowerShell, keep the following in mind:
- File Paths: Always use absolute paths for batch files to avoid ambiguity.
- Permissions: Ensure you have the necessary permissions to execute the batch file.
- Environment Variables: Be aware that the environment variables in PowerShell may differ from those in a command prompt, which can affect script execution.
Example Table: Batch File Execution Scenarios
Method | Description | Use Case |
---|---|---|
Direct Execution | Run the batch file directly from its directory | Simplest method for quick execution |
Start-Process | Execute in a new process with optional parameters | When needing a new window or specific args |
Call Operator | Run the batch file regardless of current directory | Convenient for scripts located in different directories |
Expert Insights on Running Bat Files in PowerShell
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Running batch files in PowerShell can streamline automation processes significantly. It allows users to leverage existing scripts while taking advantage of PowerShell’s advanced features, such as error handling and object manipulation.”
Michael Thompson (IT Systems Administrator, Global Tech Solutions). “Integrating batch files with PowerShell is not only efficient but also enhances compatibility across different Windows environments. By using the ‘Start-Process’ cmdlet, users can execute bat files seamlessly, ensuring a smoother workflow.”
Jessica Lin (Cybersecurity Analyst, SecureNet Corp.). “While running bat files in PowerShell can be beneficial, it is crucial to be aware of potential security risks. Ensuring that scripts are sourced from trusted locations and implementing execution policies can help mitigate these risks.”
Frequently Asked Questions (FAQs)
How do I run a .bat file in PowerShell?
To run a .bat file in PowerShell, navigate to the directory containing the file using the `cd` command, then execute the file by typing `.\filename.bat` and pressing Enter.
Can I run a .bat file with administrative privileges in PowerShell?
Yes, to run a .bat file with administrative privileges, right-click on the PowerShell icon and select “Run as administrator.” Then follow the same steps to execute the file.
What happens if I run a .bat file in PowerShell?
When you run a .bat file in PowerShell, the commands within the batch file are executed sequentially, similar to how they would run in the Command Prompt.
Are there any differences between running a .bat file in PowerShell and Command Prompt?
Yes, while both environments can execute .bat files, PowerShell supports more advanced scripting capabilities and may handle certain commands differently than Command Prompt.
Can I pass arguments to a .bat file when running it in PowerShell?
Yes, you can pass arguments to a .bat file in PowerShell by appending them after the file name, like this: `.\filename.bat arg1 arg2`.
Is it possible to schedule a .bat file to run in PowerShell?
Yes, you can schedule a .bat file to run using the Task Scheduler in Windows, and you can specify PowerShell to execute the file at designated times or events.
In summary, running a batch file in PowerShell is a straightforward process that allows users to leverage the capabilities of both scripting environments. PowerShell provides a robust and flexible platform for automating tasks, and integrating batch files can enhance the functionality of scripts by utilizing existing command-line utilities. The primary method to execute a batch file involves using the `Start-Process` cmdlet or simply calling the batch file directly, depending on the desired execution context and requirements.
Additionally, it is essential to consider the execution policy settings within PowerShell, as they can affect the ability to run scripts and batch files. Users should ensure that their execution policy is set appropriately to allow the execution of batch files without unnecessary interruptions. Furthermore, understanding how to pass arguments to batch files from PowerShell can further streamline processes and improve automation efficiency.
Overall, the integration of batch files within PowerShell scripts can significantly enhance productivity and streamline workflows. By mastering the techniques to execute batch files, users can effectively combine the strengths of both environments, leading to more powerful and efficient scripting solutions.
Author Profile
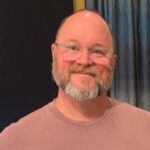
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?