How Can You Use Regular Expressions to Effectively Validate Email Addresses?
In today’s digital age, email remains one of the most vital means of communication, bridging distances and connecting people across the globe. However, with the convenience of sending messages comes the challenge of ensuring that the email addresses we collect and utilize are valid. Enter regular expressions, a powerful tool that can help developers and businesses alike streamline their processes by validating email formats efficiently. Understanding how to construct and implement a regular expression for email validation can save time, reduce errors, and enhance the overall user experience.
Regular expressions, often abbreviated as regex, are sequences of characters that define search patterns. When applied to email validation, they serve as a gatekeeper, ensuring that only properly formatted email addresses are accepted. This not only helps in maintaining a clean database but also minimizes the chances of communication failures due to incorrect addresses. As we delve deeper into the intricacies of regex for email validation, we will explore the essential components that make up a valid email address and the common pitfalls to avoid in crafting an effective regex pattern.
In this article, we will break down the elements of a well-structured regular expression specifically designed for email validation. We will discuss the reasoning behind the rules of email formatting, the significance of each character in a regex pattern, and provide practical examples that can be readily implemented in various
Understanding the Components of Email Validation
Email validation using regular expressions (regex) involves assessing various components of an email address to ensure its correctness. An email address typically consists of two primary parts: the local part and the domain part, separated by an “@” symbol.
- Local Part: This is the portion before the “@” symbol, which can include letters, numbers, and special characters.
- Domain Part: This follows the “@” symbol and usually consists of a domain name and a top-level domain (TLD).
A robust regex pattern must encapsulate these components while also adhering to specific rules dictated by email standards.
Common Patterns in Email Regex
When constructing a regex for email validation, several common patterns are observed:
- Allowed Characters: The local part can include:
- Letters (a-z, A-Z)
- Digits (0-9)
- Special characters such as `.`, `-`, `_`, and `+`
- Domain Structure: The domain part typically includes:
- Letters (a-z, A-Z)
- Digits (0-9)
- Hyphens (`-`), but not at the beginning or end of the domain
- A dot (`.`) followed by a TLD (e.g., `.com`, `.org`)
The regex pattern can thus be structured to accommodate these rules.
Example Regex for Email Validation
A widely accepted regex pattern for validating email addresses is:
“`
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
“`
This regex breaks down as follows:
Pattern Component | Description |
---|---|
^ | Asserts the start of the string |
[a-zA-Z0-9._%+-]+ | Matches one or more allowed characters in the local part |
@ | Matches the “@” symbol |
[a-zA-Z0-9.-]+ | Matches one or more allowed characters in the domain part |
\. | Matches the dot before the TLD |
[a-zA-Z]{2,} | Matches the TLD with a minimum of two letters |
$ | Asserts the end of the string |
Limitations and Considerations
While regex provides a powerful tool for email validation, it is essential to recognize its limitations:
- Complexity of Valid Emails: Email standards (RFC 5321 and RFC 5322) permit a variety of characters and formats that may not be fully captured by a single regex pattern.
- Positives/Negatives: A regex may incorrectly validate an invalid email or reject a valid one due to its strictness.
- Internationalization: With the advent of internationalized email addresses, additional considerations must be made for non-ASCII characters.
In practice, it is advisable to combine regex validation with other validation methods, such as sending confirmation emails, to ensure the validity of email addresses comprehensively.
Understanding Email Validation Requirements
Validating an email address is crucial for ensuring that user inputs are reliable and correctly formatted. Various components make up a valid email address, including:
- Local Part: The segment before the “@” symbol.
- Domain Part: The segment after the “@” symbol, which includes:
- Domain Name: The name of the organization or service.
- Top-Level Domain (TLD): The suffix indicating the nature of the organization (e.g., .com, .org).
A valid email must adhere to specific syntax rules defined by standards such as RFC 5321 and RFC 5322.
Basic Structure of an Email Address
The general format of an email address can be represented as:
“`
local-part@domain
“`
Here’s a breakdown of valid characters:
Component | Valid Characters |
---|---|
Local Part | Letters (a-z, A-Z), digits (0-9), special characters (._%+-) |
Domain Part | Letters (a-z, A-Z), digits (0-9), hyphens (-), dots (.) |
TLD | Letters only (2 to 63 characters) |
Regular Expression for Email Validation
To effectively validate email addresses, a robust regular expression (regex) can be employed. Below is a commonly used regex pattern for validating standard email addresses:
“`regex
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
“`
Explanation of the Regex Components:
- `^`: Asserts the start of the string.
- `[a-zA-Z0-9._%+-]+`: Matches one or more characters that are letters, digits, or any of the specified special characters.
- `@`: Matches the “@” symbol.
- `[a-zA-Z0-9.-]+`: Matches one or more characters in the domain name, which can include letters, digits, dots, and hyphens.
- `\.`: Escapes the dot, indicating a literal dot in the domain.
- `[a-zA-Z]{2,}`: Matches the top-level domain, ensuring it consists of at least two letters.
- `$`: Asserts the end of the string.
Considerations for Email Validation
When implementing email validation using regex, consider the following:
- Internationalization: The regex provided does not account for internationalized domain names (IDNs) or email addresses. For comprehensive validation, consider additional patterns or libraries supporting IDNs.
- Length Restrictions: The total length of an email address should not exceed 254 characters.
- Complexity of Email Formats: Some valid email formats may include quoted strings and comments, which are not covered by basic regex patterns.
Testing Your Regex
To ensure your regex works effectively, utilize testing tools available online. Some recommended tools include:
- Regex101: Offers real-time regex testing with explanations.
- RegExr: A community-driven platform for regex testing and sharing.
- Regex Pal: A straightforward tool for testing regex patterns.
By validating against a diverse set of email formats, you can refine your regex pattern for accuracy and efficiency.
Expert Insights on Regular Expressions for Email Validation
Dr. Emily Carter (Senior Software Engineer, CodeSecure Inc.). “A well-constructed regular expression for email validation is crucial for ensuring data integrity in applications. It should account for various valid email formats while avoiding overly complex patterns that may lead to negatives.”
Michael Tran (Lead Data Scientist, DataGuard Solutions). “When designing a regular expression for email validation, it is essential to balance strictness and flexibility. The regex must accommodate common email variations, including those with subdomains and special characters, without compromising security.”
Linda Zhao (Cybersecurity Analyst, SecureTech Labs). “While regular expressions are powerful for email validation, they should not be the sole method of verification. Combining regex with additional validation techniques, such as domain checks, enhances the reliability of email inputs.”
Frequently Asked Questions (FAQs)
What is a regular expression for validating email addresses?
A regular expression (regex) for validating email addresses typically follows the pattern: `^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$`. This pattern checks for a valid format, including local parts, domain names, and top-level domains.
Why is it important to validate email addresses using regular expressions?
Validating email addresses helps ensure that the input conforms to a standard format, reducing errors in data entry and improving communication reliability. It also helps prevent spam and malicious activities.
Can a regular expression fully guarantee a valid email address?
No, while a regular expression can check the format of an email address, it cannot guarantee that the address exists or is currently in use. Additional verification methods, such as sending a confirmation email, are necessary.
What are some common pitfalls when using regular expressions for email validation?
Common pitfalls include overly complex patterns that may reject valid addresses, ignoring internationalized email formats, and failing to account for all valid domain extensions. Simplicity and adherence to standards are key.
How can I test a regular expression for email validation?
You can test a regular expression using online regex testers, programming language tools, or integrated development environments (IDEs) that support regex functionality. Input various email formats to evaluate the accuracy of the regex.
Are there any alternatives to using regular expressions for email validation?
Yes, alternatives include using built-in validation libraries in programming languages or frameworks, which often provide more comprehensive and user-friendly validation methods, including checks for existing email addresses.
In summary, the use of regular expressions (regex) to validate email addresses is a common practice in programming and data validation. Regular expressions provide a powerful tool for matching patterns in strings, allowing developers to ensure that email addresses conform to specified formats. A well-constructed regex can help filter out invalid email addresses, thus enhancing data integrity and reducing errors in applications that rely on user input.
Key takeaways from the discussion include the importance of understanding the structure of valid email addresses, which typically consist of a local part, an “@” symbol, and a domain part. A robust regex for email validation should account for various factors, including the presence of special characters, domain extensions, and overall length constraints. However, it is crucial to recognize that while regex can effectively filter many invalid formats, it cannot guarantee that an email address is deliverable or exists.
Additionally, developers should be cautious about overly complex regex patterns that may lead to positives or negatives. Simplicity and clarity in regex design are essential for maintainability and readability. Ultimately, while regex serves as a valuable first line of defense in email validation, it is advisable to complement it with additional verification methods, such as sending confirmation emails, to ensure the accuracy and validity of
Author Profile
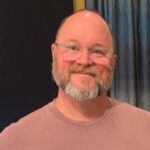
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?