How Can You Import a Class from Another Python File?
In the world of Python programming, the ability to organize code effectively is crucial for building scalable and maintainable applications. One of the key practices that developers embrace is the modularization of their code—breaking it down into separate files and classes. This not only enhances readability but also promotes reusability. However, as your projects grow in complexity, you may find yourself wondering: how do you seamlessly import a class from one Python file into another? Understanding this fundamental concept can significantly streamline your development process and empower you to create more sophisticated programs.
When working with multiple Python files, knowing how to import classes allows you to leverage existing code without redundancy. This practice not only saves time but also minimizes the risk of errors that can arise from duplicating code. By mastering the import mechanism, you can easily access functions, methods, and variables defined in other files, thus creating a more cohesive and efficient coding environment.
As we delve deeper into this topic, we will explore various methods of importing classes, the nuances of Python’s import system, and best practices to ensure your code remains clean and organized. Whether you’re a novice looking to enhance your skills or an experienced developer seeking to refine your workflow, understanding how to import classes effectively will elevate your programming game.
Understanding the Python Import System
In Python, the import system is designed to facilitate the inclusion of code from one module into another. A module is simply a file containing Python code. When you want to use functions, classes, or variables defined in one module within another, you need to import them. This promotes code reusability and organization.
To import a class from another Python file, you utilize the `import` statement along with the module’s name. The general syntax for importing a specific class from a module is:
“`python
from module_name import ClassName
“`
Where `module_name` is the name of the Python file (without the `.py` extension), and `ClassName` is the name of the class you wish to import.
File Structure and Example
Assuming you have the following file structure:
“`
project/
│
├── main.py
└── my_module.py
“`
In `my_module.py`, you may define a class as follows:
“`python
my_module.py
class MyClass:
def greet(self):
return “Hello from MyClass!”
“`
To import `MyClass` into `main.py`, you would write:
“`python
main.py
from my_module import MyClass
my_instance = MyClass()
print(my_instance.greet())
“`
This will output:
“`
Hello from MyClass!
“`
Relative vs Absolute Imports
When working with modules, it’s important to understand the difference between relative and absolute imports.
- Absolute Imports: This refers to importing a module using its full path from the project’s root directory. It is the most common and recommended way to import modules.
- Relative Imports: These imports are relative to the current module’s location. They are often used in larger projects with multiple directories.
Here is a comparison of both methods:
Type | Syntax Example | Description |
---|---|---|
Absolute Import | `from package.module import ClassName` | Full path from the project root |
Relative Import | `from .module import ClassName` | Relative path based on current module |
Common Import Scenarios
When importing classes, you may encounter various scenarios:
- Importing Multiple Classes: You can import multiple classes from the same module in one line:
“`python
from my_module import ClassOne, ClassTwo
“`
- Renaming Imports: If you want to avoid name clashes or simplify access, you can rename imports using the `as` keyword:
“`python
from my_module import MyClass as MC
“`
- Importing Everything: Although not generally recommended due to potential conflicts, you can import all classes and functions from a module:
“`python
from my_module import *
“`
This method imports everything but can lead to ambiguity in larger projects.
Utilizing the Python import system effectively allows for better project organization and code reuse. Understanding how to navigate between modules is essential for building scalable and maintainable applications.
Understanding Python Modules and Packages
In Python, files containing Python code are referred to as modules. A module can contain functions, classes, and variables, and can also include runnable code. To import a class from another Python file, you need to understand how Python modules and packages work.
- Module: A file with a `.py` extension containing Python code.
- Package: A directory containing multiple Python modules, which must include an `__init__.py` file.
Basic Importing of a Class
To import a class from another file, the syntax is straightforward. Assuming you have a file named `my_class.py` with a class called `MyClass`, you can import it using the following syntax:
“`python
from my_class import MyClass
“`
This statement enables you to use `MyClass` directly in your code. Here’s a brief example:
my_class.py
“`python
class MyClass:
def greet(self):
return “Hello from MyClass!”
“`
main.py
“`python
from my_class import MyClass
obj = MyClass()
print(obj.greet()) Output: Hello from MyClass!
“`
Importing Multiple Classes
When you need to import multiple classes from a single module, you can do so in a single import statement:
“`python
from my_class import MyClass, AnotherClass
“`
Alternatively, you can use the asterisk `*` to import all classes and functions, although this is generally discouraged due to potential name conflicts:
“`python
from my_class import *
“`
Importing from Subdirectories
If your module is located in a subdirectory, you need to ensure that the subdirectory is structured as a package. This requires an `__init__.py` file in the subdirectory. For example, if you have the following structure:
“`
project/
│
├── main.py
└── my_package/
├── __init__.py
└── my_class.py
“`
You can import `MyClass` like this:
“`python
from my_package.my_class import MyClass
“`
Using Aliases for Imports
To make your code cleaner or to avoid naming conflicts, you can use aliases when importing:
“`python
from my_class import MyClass as MC
“`
Now you can instantiate `MyClass` using the alias:
“`python
obj = MC()
“`
Handling Import Errors
When importing classes, you might encounter import errors. Common causes and solutions include:
Error Type | Description | Solution |
---|---|---|
`ModuleNotFoundError` | Python cannot find the specified module. | Ensure the module name and path are correct. |
`ImportError` | The module is found, but the class/function does not exist. | Check the spelling of the class/function name. |
Circular Import | Two modules import each other. | Refactor the code to avoid circular dependencies. |
Best Practices for Importing
- Limit imports: Only import what you need to keep the namespace clean.
- Organize modules: Keep related classes and functions together in the same module.
- Use absolute imports: Prefer absolute imports over relative imports for clarity.
By following these guidelines and utilizing the provided syntax, you can effectively manage imports across multiple Python files, enhancing code organization and reusability.
Expert Insights on Importing Classes in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When importing a class from another Python file, it is essential to ensure that the file you are importing from is in the same directory or within the Python path. Using the syntax ‘from filename import ClassName’ allows for seamless integration of classes across modules.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Understanding the nuances of Python’s import system is crucial. Utilizing relative imports can simplify the process when dealing with packages, but one must be cautious about the module’s structure to avoid import errors.”
Sarah Johnson (Python Educator, LearnPythonOnline). “For beginners, it’s vital to grasp the concept of namespaces when importing classes. This knowledge will prevent naming conflicts and enhance code readability, making it easier to manage larger projects.”
Frequently Asked Questions (FAQs)
How do I import a class from another Python file?
To import a class from another Python file, use the syntax `from filename import ClassName`, where `filename` is the name of the file (without the .py extension) and `ClassName` is the name of the class you want to import.
What should I do if the Python files are in different directories?
If the files are in different directories, you can use the `sys.path.append(‘path/to/directory’)` method to add the directory to the Python path before importing the class, or you can structure your project as a package and use relative imports.
Can I import multiple classes from the same file?
Yes, you can import multiple classes from the same file using the syntax `from filename import ClassName1, ClassName2`, separating each class name with a comma.
What if I want to import all classes from a file?
To import all classes from a file, you can use the syntax `from filename import *`. However, this practice is generally discouraged as it can lead to namespace conflicts and reduce code readability.
Is it possible to import a class using an alias?
Yes, you can import a class using an alias by using the syntax `from filename import ClassName as AliasName`. This allows you to refer to the class with a different name in your code.
What happens if the class name conflicts with an existing name?
If there is a name conflict, the imported class will overwrite the existing name in the current namespace. To avoid this, use an alias during the import or ensure unique naming conventions in your code.
In summary, importing a class from another Python file is a fundamental skill that enhances code organization and reusability. This process typically involves using the `import` statement, which allows developers to access classes, functions, and variables defined in separate modules. By structuring code into multiple files, programmers can maintain cleaner, more manageable codebases, especially in larger projects.
When importing a class, it is essential to understand the syntax and the different ways to perform the import. For instance, one can use `from module_name import ClassName` to import a specific class directly, or `import module_name` to import the entire module, accessing the class via `module_name.ClassName`. This flexibility allows developers to choose the most suitable method based on their needs and coding style.
Additionally, it is crucial to be aware of the Python module search path and how it affects the import process. The current directory and the directories listed in the `PYTHONPATH` environment variable are part of this search path. Understanding this aspect helps prevent import errors and ensures that the intended modules are correctly located and utilized.
mastering the importation of classes from other Python files not only streamlines the development process but also fosters
Author Profile
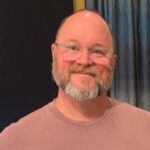
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?