How Can You Effectively Push Your Node.js Project to GitHub?
In the ever-evolving world of software development, sharing your code and collaborating with others has never been more vital. For Node.js developers, pushing your project to GitHub not only showcases your work but also opens the door to a vibrant community of developers eager to contribute and learn. Whether you’re a seasoned programmer or just starting your journey, understanding how to effectively manage your code on GitHub can elevate your projects and enhance your professional portfolio.
Pushing a Node.js project to GitHub involves more than just a few simple commands; it’s about creating a seamless workflow that allows you to track changes, collaborate with others, and maintain a clean codebase. From initializing your repository to managing branches and handling pull requests, the process is designed to empower developers to work together efficiently. Moreover, leveraging GitHub’s features, such as issue tracking and project boards, can significantly improve your project’s organization and visibility.
As you embark on this journey, you’ll discover the essential tools and best practices that will not only help you push your Node.js project to GitHub but also ensure that your code is well-structured and accessible to others. So, whether you’re looking to share your latest app, contribute to open-source projects, or simply refine your skills, mastering the art of pushing your Node.js project to Git
Setting Up Your GitHub Repository
Before pushing your Node.js project to GitHub, you need to create a repository on the GitHub platform. Follow these steps to set up your repository:
- Log into your GitHub account.
- Click on the “+” icon in the upper right corner and select “New repository.”
- Fill in the repository details:
- Repository Name: Choose a descriptive name for your project.
- Description: (Optional) Provide a brief overview of your project.
- Visibility: Choose between Public or Private.
- Initialize this repository with a README: It’s often helpful to include a README file.
- Click on “Create repository.”
Once the repository is created, you’ll be directed to a page with instructions on how to push your existing project.
Preparing Your Local Project
Ensure that your Node.js project is ready for deployment to GitHub. You should have a `.gitignore` file in your project directory to exclude files that don’t need to be pushed, such as `node_modules` and environment variable files.
Here’s an example of what to include in a `.gitignore` file for a Node.js project:
“`
node_modules/
.env
.DS_Store
npm-debug.log
“`
Initializing Git in Your Project
To start version control with Git, navigate to your project directory in the command line and execute the following commands:
“`bash
cd /path/to/your/project
git init
“`
This command initializes a new Git repository in your project folder.
Adding Your Files to Git
After initializing Git, you need to add your project files to the staging area. You can do this by using the following command:
“`bash
git add .
“`
This command stages all changes in your project. To check which files have been staged, you can use:
“`bash
git status
“`
Committing Your Changes
Once your files are staged, the next step is to commit the changes. This command creates a snapshot of your project at this point in time. Use the following command to commit:
“`bash
git commit -m “Initial commit”
“`
Replace “Initial commit” with a meaningful message that describes the changes being made.
Pushing to GitHub
After committing your changes, you need to link your local repository to the remote repository you created on GitHub. Use the following command, replacing `USERNAME` with your GitHub username and `REPOSITORY_NAME` with the name of your repository:
“`bash
git remote add origin https://github.com/USERNAME/REPOSITORY_NAME.git
“`
Now, push your committed changes to the remote repository:
“`bash
git push -u origin master
“`
If you are using a different branch name (like `main`), substitute `master` with your branch name.
Verifying Your Push
To ensure that your project has been successfully pushed to GitHub, navigate to your repository in a web browser. You should see your project files listed there. If you included a README file, it will also be displayed prominently on the repository page.
Command | Description |
---|---|
git init | Initializes a new Git repository. |
git add . | Stages all changes in the project. |
git commit -m “message” | Commits staged changes with a message. |
git remote add origin | Links the local repository to the remote repository. |
git push -u origin master | Pushing changes to the master branch of the remote repository. |
This process will allow you to efficiently manage your Node.js project using Git and keep it updated on GitHub.
Setting Up Your Local Repository
To push a Node.js project to GitHub, you first need to ensure that your local repository is correctly initialized. Follow these steps:
- Navigate to your project directory using the terminal:
“`bash
cd path/to/your/nodejs-project
“`
- Initialize a Git repository if it hasn’t been done already:
“`bash
git init
“`
- Add your project files to the staging area:
“`bash
git add .
“`
- Commit your changes with a meaningful message:
“`bash
git commit -m “Initial commit”
“`
Creating a New Repository on GitHub
Before you can push your project, you need to create a new repository on GitHub:
- Log in to your GitHub account.
- Click on the “+” icon in the upper right corner, then select “New repository.”
- Fill out the repository details:
- Repository name: Choose a unique name for your project.
- Description: Add a brief description (optional).
- Public/Private: Select the visibility of your repository.
- Initialize this repository with a README: Leave this unchecked if you already have a README file in your project.
- Click “Create repository” to finalize the process.
Linking Local Repository to GitHub
After creating the GitHub repository, connect your local repository to GitHub:
- Copy the repository URL from GitHub (either HTTPS or SSH).
- Add the remote repository in your terminal:
“`bash
git remote add origin https://github.com/yourusername/repository-name.git
“`
Pushing Your Project to GitHub
With the local repository linked to GitHub, you can now push your changes:
- Push your commits to GitHub:
“`bash
git push -u origin master
“`
If your branch is named `main`, replace `master` with `main`.
- Enter your GitHub credentials if prompted, especially for HTTPS connections.
Verifying Your Push
To confirm that your project has been successfully pushed:
- Visit your GitHub repository in a web browser.
- Check the files to ensure all your project files are present.
- Review the commit history to verify that your commits are logged.
Managing Future Changes
For ongoing development, follow these practices for managing future changes:
- Make changes to your project files.
- Stage and commit your changes:
“`bash
git add .
git commit -m “Your descriptive message”
“`
- Push updates to GitHub:
“`bash
git push origin master
“`
Utilizing these steps will maintain synchronization between your local Node.js project and the GitHub repository, ensuring a smooth workflow.
Expert Insights on Pushing Node.js Projects to GitHub
Jessica Lin (Senior Software Engineer, Tech Innovations Inc.). “When pushing a Node.js project to GitHub, it is crucial to ensure that your .gitignore file is properly configured to exclude node_modules and other sensitive files. This practice not only keeps your repository clean but also prevents potential security issues.”
Michael Chen (DevOps Specialist, Cloud Solutions Group). “Utilizing Git branches effectively is essential when pushing Node.js projects to GitHub. It allows for better collaboration among team members and helps in managing different features or bug fixes without affecting the main codebase.”
Sarah Patel (Open Source Advocate, Code for Good). “Before pushing your Node.js project to GitHub, make sure to include a comprehensive README file. This document serves as a guide for other developers and users, detailing how to set up the project, its dependencies, and usage instructions.”
Frequently Asked Questions (FAQs)
How do I initialize a Node.js project for GitHub?
To initialize a Node.js project for GitHub, navigate to your project directory in the terminal and run `git init`. This command creates a new Git repository. Then, create a `.gitignore` file to exclude `node_modules` and other unnecessary files.
What files should I include in my GitHub repository for a Node.js project?
You should include files such as `package.json`, `index.js`, and any other source files necessary for your application. Additionally, include documentation files like `README.md` to provide information about your project.
How do I commit changes to my Node.js project before pushing to GitHub?
To commit changes, use the commands `git add .` to stage all changes and `git commit -m “Your commit message”` to commit them. Ensure your commit message is descriptive to reflect the changes made.
What is the command to push my Node.js project to GitHub?
To push your project to GitHub, use the command `git push origin main`, replacing `main` with your branch name if it differs. Ensure you have set up the remote repository with `git remote add origin
How can I check if my Node.js project has been successfully pushed to GitHub?
You can verify the push by visiting your GitHub repository in a web browser. Check for the latest commit and confirm that all intended files are present in the repository.
What should I do if I encounter an error while pushing to GitHub?
If you encounter an error, check the error message for details. Common issues include authentication errors or conflicts with the remote branch. Ensure you are authenticated correctly and resolve any merge conflicts before attempting to push again.
pushing a Node.js project to GitHub involves several key steps that ensure your code is properly version-controlled and accessible to others. First, it is essential to initialize a Git repository within your project directory using the command `git init`. This step sets up the necessary files for Git to track changes in your project. Following this, you should add your project files to the staging area with `git add .`, which prepares them for committing.
Next, committing your changes with a meaningful message using `git commit -m “Your message”` is crucial for maintaining a clear history of your project’s development. After committing, you will need to create a remote repository on GitHub and link it to your local repository using the command `git remote add origin
Key takeaways from this process include the importance of maintaining a clean commit history and using descriptive messages that reflect the changes made. Additionally, understanding how to effectively manage branches and merge requests can further enhance collaboration on your Node.js projects. By following these steps, developers can ensure their work is not only backed up but
Author Profile
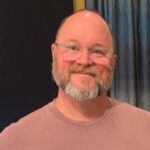
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?