How Can You Redirect Users in React When Their Token Has Expired?
In the fast-paced world of web development, ensuring a seamless user experience is paramount. One of the challenges developers face is managing user authentication effectively, especially when it comes to handling expired tokens. In a React application, an expired token can lead to confusion and frustration for users, as they may find themselves unexpectedly logged out or unable to access certain features. This article delves into the critical process of redirecting users when their authentication token has expired, ensuring that they remain informed and engaged with your application.
When a user logs into a React application, they receive an authentication token that allows them to access protected routes and resources. However, tokens have a finite lifespan, and when they expire, it’s crucial to have a strategy in place to manage this scenario gracefully. A well-implemented redirect mechanism not only enhances user experience but also reinforces security by preventing unauthorized access to sensitive areas of the application.
In this article, we will explore various methods for detecting expired tokens, the importance of implementing redirects, and best practices for maintaining a smooth user journey. By understanding these concepts, developers can create robust applications that not only protect user data but also keep users informed and engaged, even when their session has ended.
Understanding Token Expiration
Token expiration is a crucial aspect of securing applications that utilize authentication tokens. When a user logs in, the server generates a token that is used to authenticate the user on subsequent requests. However, these tokens often have an expiration time to enhance security. This ensures that even if a token is intercepted, it cannot be used indefinitely.
The expiration time for tokens can vary based on application requirements. Common practices include:
- Short-lived tokens: Typically valid for a few minutes to an hour.
- Long-lived tokens: May last for days or even weeks, often requiring refresh tokens for continued access.
Understanding how to handle expired tokens is essential for maintaining a seamless user experience.
Detecting Expired Tokens in React
To manage expired tokens in a React application, developers can implement a function that checks the validity of the token before making requests to the server. This can be achieved by decoding the token and examining its expiration payload.
A common structure of a JWT (JSON Web Token) includes:
– **Header**: Contains metadata about the token.
– **Payload**: Holds the claims, including the expiration time (`exp`).
– **Signature**: Used to verify the token’s authenticity.
To decode a JWT and check its expiration, you can use the `jwt-decode` library. Here’s a basic implementation:
“`javascript
import jwtDecode from ‘jwt-decode’;
const isTokenExpired = (token) => {
if (!token) return true;
const decoded = jwtDecode(token);
return decoded.exp < Date.now() / 1000;
};
```
This function returns `true` if the token is expired.
Redirecting Users Upon Expiration
Once you detect that a token has expired, it is vital to redirect users to the login page or another appropriate location. This can be done using React Router’s `useHistory` or `useNavigate` hooks. Below is an example of how to implement this functionality:
“`javascript
import { useEffect } from ‘react’;
import { useNavigate } from ‘react-router-dom’;
const App = () => {
const navigate = useNavigate();
const token = localStorage.getItem(‘token’);
useEffect(() => {
if (isTokenExpired(token)) {
localStorage.removeItem(‘token’); // Clear the expired token
navigate(‘/login’); // Redirect to login page
}
}, [token, navigate]);
return (
);
};
“`
This implementation checks the token’s validity whenever the component mounts and redirects the user if necessary.
Best Practices for Token Management
When dealing with token management, consider the following best practices:
- Use Secure Storage: Store tokens in secure locations such as `httpOnly` cookies or secure storage mechanisms, rather than local storage, to mitigate XSS attacks.
- Implement Refresh Tokens: Use refresh tokens to maintain user sessions without requiring them to log in frequently.
- Monitor Token Lifetimes: Adjust the lifetime of access tokens based on the sensitivity of the application and user needs.
Token Management Table
Token Type | Expiration Duration | Usage |
---|---|---|
Access Token | Short-lived (e.g., 15 min) | Authenticates user requests |
Refresh Token | Long-lived (e.g., 30 days) | Used to obtain new access tokens |
Handling Token Expiration in React
When developing applications with React, it is crucial to manage user authentication effectively, particularly when dealing with token expiration. An expired token can lead to unauthorized access and a poor user experience. Therefore, implementing redirects when a token expires is essential.
Detecting Token Expiration
To ensure that your application responds appropriately to an expired token, you first need to establish a method for detecting it. This can be achieved by:
– **Storing the Expiration Time**: When a token is issued, record its expiration time in local storage or state.
– **Checking the Token Before Requests**: Create a utility function to check the token’s validity before making API calls.
Example function to check token expiration:
“`javascript
const isTokenExpired = (token) => {
const decodedToken = jwt.decode(token);
return decodedToken.exp < Date.now() / 1000;
};
```
Redirecting Users on Expiration
Once you have established a mechanism to detect token expiration, the next step is to redirect users to a login page or another appropriate component when the token is invalid.
- **Using React Router**: Integrate with React Router to manage redirects.
- **Implementing a Higher-Order Component (HOC)**: Create an HOC that checks for token validity and redirects if necessary.
Example of a HOC for token validation:
“`javascript
import { Redirect } from ‘react-router-dom’;
const withAuthRedirect = (WrappedComponent) => {
return (props) => {
const token = localStorage.getItem(‘token’);
if (!token || isTokenExpired(token)) {
return
}
return
};
};
“`
Using Context API for Global State Management
For applications that require token management across multiple components, using the Context API can be beneficial. This allows you to provide token state and expiration checks throughout your component tree.
– **Create a Context Provider**: Set up a context to manage authentication state.
– **Check for Expiration**: Use the context to check for token expiration in any component.
Example context setup:
“`javascript
import React, { createContext, useContext, useState, useEffect } from ‘react’;
const AuthContext = createContext();
const AuthProvider = ({ children }) => {
const [isAuthenticated, setIsAuthenticated] = useState();
const token = localStorage.getItem(‘token’);
useEffect(() => {
if (token && !isTokenExpired(token)) {
setIsAuthenticated(true);
} else {
setIsAuthenticated();
}
}, [token]);
return (
{children}
);
};
const useAuth = () => useContext(AuthContext);
“`
Integrating API Calls with Token Validation
When performing API calls, it’s essential to include token validation to prevent unauthorized actions. This can be executed by:
– **Using Interceptors in Axios**: If you are using Axios, set up interceptors to handle expired tokens globally.
Example Axios interceptor:
“`javascript
axios.interceptors.response.use(
response => response,
error => {
if (error.response.status === 401) {
// Redirect to login page
window.location.href = ‘/login’;
}
return Promise.reject(error);
}
);
“`
Conclusion on Redirect Mechanisms
Implementing effective redirect mechanisms for expired tokens in a React application is critical for maintaining security and user experience. By combining token expiration detection, React Router for navigation, the Context API for state management, and API call interceptors, developers can create a robust authentication flow that handles token expiration gracefully.
Strategies for Handling Token Expiration in React Applications
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Incorporating a middleware in your React application can effectively intercept API calls and check for token validity. If the token is expired, redirecting users to the login page ensures a seamless user experience while maintaining security protocols.”
Michael Chen (Lead Developer, SecureWeb Solutions). “Utilizing React Router’s `useEffect` hook to monitor authentication state can be a game-changer. By checking the token’s expiration on component mount, developers can redirect users proactively, preventing unauthorized access to protected routes.”
Sarah Patel (Cybersecurity Consultant, Guarded Networks). “It is crucial to implement a robust token management strategy that includes automatic token refresh mechanisms. This approach not only minimizes the chances of token expiration but also enhances user experience by avoiding unnecessary redirects during active sessions.”
Frequently Asked Questions (FAQs)
What happens when a token expires in a React application?
When a token expires, the application can no longer authenticate requests to protected resources. This typically results in an error response from the server, indicating that the user is unauthorized.
How can I detect an expired token in React?
You can detect an expired token by checking the token’s expiration time against the current time. If the current time exceeds the expiration time, the token is considered expired.
What is the best way to redirect users if their token is expired?
The best approach is to implement an interceptor in your API request logic that checks for token expiration. If the token is expired, redirect users to the login page or a refresh token endpoint.
Can I use React Router for handling redirects upon token expiration?
Yes, you can use React Router to manage redirects. By utilizing the `useHistory` hook or `Navigate` component, you can programmatically redirect users to the desired route when a token is detected as expired.
Should I refresh the token automatically when it expires?
Automatically refreshing the token is a common practice. However, it is essential to handle scenarios where the refresh token may also be expired or invalid, prompting a redirect to the login page.
What are some common libraries to manage token expiration in React?
Popular libraries include `axios` for API requests, which can be configured with interceptors, and `react-query` for managing server state and caching, which can help in handling token expiration effectively.
In the context of React applications, managing user authentication and session validity is crucial for ensuring a secure user experience. One common challenge developers face is handling situations where a user’s authentication token has expired. Implementing a redirect mechanism when a token is expired allows for a seamless user experience, prompting users to re-authenticate without encountering confusion or security risks. This process typically involves checking the token’s validity on protected routes and redirecting users to a login page or a refresh token flow when necessary.
Key takeaways from this discussion include the importance of implementing token validation checks within the application’s routing logic. Utilizing libraries such as React Router, developers can create higher-order components or custom hooks that encapsulate the logic for token verification. This not only streamlines the authentication process but also enhances code maintainability. Additionally, developers should consider user experience by providing informative feedback when a token expires, guiding users through the re-authentication process effectively.
Furthermore, it is essential to establish a clear strategy for token management, including expiration handling and refresh token mechanisms. By proactively managing token lifecycle events, applications can minimize disruptions for users while maintaining robust security protocols. Overall, implementing a redirect for expired tokens is a critical aspect of building secure and user-friendly React applications.
Author Profile
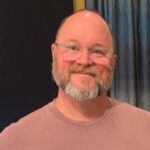
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?