How Can You Efficiently Comment Out Multiple Lines in JavaScript?
In the world of programming, clarity and organization are paramount, especially when it comes to writing and maintaining code. JavaScript, one of the most popular programming languages, offers developers a variety of tools to enhance their coding experience. Among these tools is the ability to comment out lines of code, a practice that not only aids in debugging but also improves code readability. If you’ve ever found yourself needing to temporarily disable a section of your JavaScript code or leave notes for yourself or your team, understanding how to comment out multiple lines effectively is an essential skill.
Commenting out multiple lines in JavaScript can streamline your workflow, allowing you to focus on specific parts of your code without the distraction of other lines. This technique is particularly useful during the development process, where experimenting with different code snippets can lead to better solutions. Additionally, well-placed comments can serve as documentation, guiding future developers—or even your future self—through the logic and intent behind your code.
In this article, we will explore various methods for commenting out multiple lines in JavaScript, highlighting the advantages and best practices associated with each approach. Whether you’re a seasoned developer or just starting your coding journey, mastering this skill will enhance your ability to write clean, efficient, and maintainable code. Get
Commenting Out Multiple Lines Using Block Comments
In JavaScript, one of the most efficient methods to comment out multiple lines is by using block comments. This method utilizes the `/*` and `*/` symbols to encapsulate the code you wish to comment out.
For example:
“`javascript
/*
console.log(“This line is commented out.”);
console.log(“This line is also commented out.”);
*/
“`
Using block comments is particularly useful when you need to disable a large section of code temporarily or provide detailed explanations for a block of code.
Commenting Out Multiple Lines Using Line Comments
While block comments are effective, you may also use line comments (`//`) to comment out multiple lines. However, this approach requires placing `//` at the beginning of each line you want to comment out.
For instance:
“`javascript
// console.log(“This line is commented out.”);
// console.log(“This line is also commented out.”);
“`
Although this method is straightforward, it can become cumbersome if you have many lines to comment out.
Best Practices for Commenting
When commenting out code, consider the following best practices to maintain code readability and organization:
- Clarity: Ensure that comments clearly explain the purpose of the code or why it is commented out.
- Consistency: Use a consistent commenting style throughout your codebase to enhance readability.
- Minimalism: Avoid excessive comments; only comment out code when necessary to keep the code clean and maintainable.
Comparison of Commenting Methods
The following table summarizes the differences between block comments and line comments in JavaScript:
Method | Syntax | Use Case |
---|---|---|
Block Comments | /* comment here */ | Commenting out multiple lines at once |
Line Comments | // comment here | Commenting out individual lines |
Both methods serve their purpose, and choosing one over the other often depends on the context and the specific needs of the developer.
Single-Line Comments
In JavaScript, single-line comments can be created using the double forward slash (`//`). This method is useful for commenting out a single line of code or adding brief explanations.
Example:
“`javascript
// This is a single-line comment
let x = 5; // This assigns 5 to x
“`
Multi-Line Comments
To comment out multiple lines in JavaScript, you can use multi-line comments, which are initiated with `/*` and closed with `*/`. This allows you to include longer explanations or disable blocks of code without requiring multiple single-line comments.
Example:
“`javascript
/*
This is a multi-line comment.
It can span multiple lines.
*/
let y = 10; /* This line is not commented out */
“`
Best Practices for Commenting
When using comments in your JavaScript code, consider the following best practices:
- Clarity: Write comments that clearly explain the purpose of the code.
- Relevance: Ensure comments are relevant and update them as the code changes.
- Brevity: Keep comments concise; avoid unnecessary verbosity.
- Consistency: Use a consistent commenting style throughout your codebase.
Examples of Commenting Usage
Here are several scenarios demonstrating effective use of comments:
Scenario | Comment Style | Example Code |
---|---|---|
Documenting a function | Multi-line comment | “`javascript /* This function adds two numbers */ function add(a, b) { return a + b; }“` |
Explaining complex logic | Inline comment | “`javascript let result = calculate(); // result holds the output of the calculation“` |
Temporarily disabling code | Multi-line comment | “`javascript /* console.log(‘This will not run’); */“` |
Common Mistakes to Avoid
When commenting out lines in JavaScript, avoid these common pitfalls:
- Over-commenting: Writing too many comments can clutter code and reduce readability.
- Misplaced comments: Ensure comments are placed correctly above or next to the relevant code.
- Failing to update comments: Outdated comments can lead to confusion and misinterpretation of the code’s function.
Conclusion on Commenting Techniques
Utilizing both single-line and multi-line comments effectively is crucial for maintaining clear, understandable, and maintainable JavaScript code. Following best practices and avoiding common mistakes enhances collaboration and code quality.
Expert Insights on Commenting Multiple Lines in JavaScript
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “Commenting out multiple lines in JavaScript can significantly enhance code readability and maintainability. Utilizing the block comment syntax, which is `/* comment */`, allows developers to easily disable sections of code during debugging or while writing new features.”
Michael Thompson (JavaScript Educator, TechLearn Academy). “When teaching newcomers about JavaScript, I emphasize the importance of using comments effectively. The ability to comment out multiple lines not only aids in understanding the flow of code but also encourages best practices in collaborative environments.”
Sarah Nguyen (Lead Frontend Developer, WebInnovate). “In my experience, developers often overlook the power of commenting out multiple lines. It is crucial for testing and iterating on code without the risk of permanent deletion. Mastering this technique can streamline the development process.”
Frequently Asked Questions (FAQs)
How do I comment out multiple lines in JavaScript?
You can comment out multiple lines in JavaScript by using the `/*` to start the comment and `*/` to end it. Everything between these markers will be ignored by the JavaScript engine.
Can I use single-line comments for multiple lines?
While you can use single-line comments (`//`) for multiple lines, you must place `//` at the beginning of each line. This is less efficient than using block comments.
What happens if I nest block comments in JavaScript?
Nesting block comments is not allowed in JavaScript. If you attempt to nest `/* … */` comments, it will lead to a syntax error.
Is there a shortcut to comment out multiple lines in code editors?
Many code editors and IDEs provide shortcuts to comment out multiple lines. For example, in Visual Studio Code, you can select the lines and press `Ctrl + /` (Windows) or `Cmd + /` (Mac).
Are there any best practices for commenting in JavaScript?
Best practices include using comments to explain complex logic, avoiding obvious comments, and keeping comments up to date with code changes. This enhances code readability and maintainability.
Can I use comments to temporarily disable code?
Yes, comments are often used to temporarily disable sections of code during testing or debugging. This allows developers to isolate issues without deleting code.
In JavaScript, commenting out multiple lines of code is an essential practice for developers, as it aids in debugging and enhances code readability. There are two primary methods for achieving this: using block comments and leveraging line comments. Block comments, encapsulated by `/*` and `*/`, allow developers to comment out entire sections of code seamlessly. This method is particularly useful for temporarily disabling large blocks of code during testing or when providing detailed explanations within the code itself.
On the other hand, line comments, initiated with `//`, can be used to comment out individual lines. While this method is effective for single-line comments, it can become cumbersome when dealing with multiple lines, as each line must be prefixed with the comment syntax. Therefore, for larger segments of code, block comments are generally the preferred approach due to their efficiency and clarity.
understanding how to comment out multiple lines in JavaScript is a fundamental skill that contributes to better coding practices. By utilizing block comments for larger sections and line comments for individual lines, developers can maintain clean and organized code. This not only aids in the debugging process but also facilitates collaboration among team members by providing clear explanations and annotations within the codebase.
Author Profile
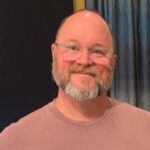
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?