Why Am I Facing ‘ImportError: Cannot Import Name UserProfile’ in My Python Project?
In the world of programming, encountering errors is an inevitable part of the journey, often serving as a rite of passage for developers at all levels. One such common yet perplexing error is the `ImportError: Cannot Import Name UserProfile`. This seemingly cryptic message can halt progress and leave even seasoned coders scratching their heads. Understanding the nuances behind this error not only aids in troubleshooting but also enhances your overall coding proficiency. In this article, we will delve into the intricacies of this import error, exploring its causes, implications, and effective solutions to get you back on track.
Overview
The `ImportError: Cannot Import Name UserProfile` typically arises in Python projects when the interpreter is unable to locate the specified class or function within a module. This can happen for a variety of reasons, including circular imports, misnamed files, or incorrect module paths. As developers navigate through their code, recognizing these potential pitfalls is crucial for maintaining a smooth workflow and ensuring that applications run as intended.
Moreover, this error highlights the importance of understanding Python’s import system and module organization. By dissecting the underlying issues that lead to this error, developers can not only resolve immediate challenges but also cultivate a deeper comprehension of best practices in code structure and modularity. Whether
Understanding the ImportError
When encountering the error message “ImportError: Cannot Import Name Userprofile”, it indicates that the Python interpreter is unable to locate or import the specified name from the module. This situation can arise due to several reasons, and understanding the underlying causes can assist in troubleshooting effectively.
Common reasons for this error include:
- Circular Imports: If two modules depend on each other, this can create a circular reference that prevents the proper loading of one or both modules.
- Incorrect Module Path: If the module from which you are trying to import does not exist in the specified path or is misspelled, the import will fail.
- Name Conflicts: If the name you are trying to import conflicts with existing names in the same namespace, it may lead to import errors.
- Version Issues: Sometimes, different versions of a library may change the structure, leading to missing classes or functions.
Troubleshooting Steps
To resolve the “ImportError: Cannot Import Name Userprofile”, follow these troubleshooting steps:
- Check for Typos: Verify that the name ‘Userprofile’ is spelled correctly and matches the case exactly as defined in the module.
- Inspect Module Structure: Ensure that the module you are trying to import from actually contains the ‘Userprofile’ class or function.
- Review Import Statements: Look at how the import statements are structured in your code. Changing the order of imports or restructuring them can sometimes resolve circular dependencies.
- Use Absolute Imports: If you are using relative imports, consider switching to absolute imports to avoid ambiguity.
- Update Libraries: If the import is from an external library, ensure that you are using the latest version of that library.
Example of Circular Import
Consider two modules, `module_a.py` and `module_b.py`, each attempting to import from each other:
module_a.py
“`python
from module_b import Userprofile
class ModuleA:
pass
“`
module_b.py
“`python
from module_a import ModuleA
class Userprofile:
pass
“`
This will lead to an ImportError since neither module can fully load before the other.
Best Practices to Avoid Import Errors
To minimize the risk of encountering import errors in your projects, consider the following best practices:
- Organize Code Structure: Keep your modules organized and avoid circular dependencies by minimizing inter-module dependencies.
- Use Virtual Environments: This helps manage dependencies effectively and prevents version conflicts.
- Document Dependencies: Maintain clear documentation of module dependencies and their expected versions.
Example Table of Potential Causes and Solutions
Potential Cause | Solution |
---|---|
Circular Import | Refactor code to avoid circular dependencies |
Incorrect Module Path | Check and correct the module path or name |
Name Conflicts | Rename conflicting classes or functions |
Version Issues | Update or downgrade the library as necessary |
By following these steps and practices, developers can effectively address the ImportError and ensure smoother development processes.
Common Causes of ImportError
The `ImportError: Cannot Import Name Userprofile` typically arises due to several common issues within Python projects. Understanding these causes can aid developers in troubleshooting effectively.
- Incorrect Module Path: The most frequent reason for this error is specifying the wrong path or module name in the import statement. Ensure that the module from which you are trying to import the `Userprofile` class or function exists in the specified location.
- Circular Imports: If two or more modules are importing each other, it may lead to an incomplete initialization of one of the modules, resulting in an `ImportError`.
- Name Conflicts: If there is a naming conflict, such as a local file having the same name as the module you are trying to import from, Python may mistakenly try to import from the local file instead of the intended module.
- Module Not Installed: If the module containing `Userprofile` is not installed, or if it is installed in a different Python environment, it will lead to this error.
Troubleshooting Steps
To resolve the `ImportError`, consider the following troubleshooting steps:
- Verify Module Installation:
- Check if the required module is installed using pip:
“`bash
pip show module_name
“`
- Check Import Statements:
- Review the import statements to ensure they are correct:
“`python
from module_name import Userprofile
“`
- Inspect for Circular Imports:
- Examine your project structure for circular dependencies. Refactor the code to avoid these situations.
- Rename Local Files:
- If there is a naming conflict, rename the local file to avoid confusion with the module name.
- Utilize Absolute Imports:
- Use absolute imports instead of relative imports to prevent ambiguity:
“`python
from package.module import Userprofile
“`
Example Scenario
Consider the following scenario to illustrate the troubleshooting process:
Project Structure:
“`
my_project/
│
├── main.py
└── user/
├── __init__.py
└── userprofile.py
“`
Contents of userprofile.py:
“`python
class Userprofile:
pass
“`
Contents of main.py:
“`python
from user.userprofile import Userprofile
“`
Potential Issues:
- If `main.py` attempts to import `Userprofile` but the import statement is incorrect or the `userprofile.py` file is not properly set up, it will raise an `ImportError`.
Solution:
- Ensure that `userprofile.py` is in the correct directory and that the import statement matches the file structure.
Useful Debugging Tools
Several tools can assist in diagnosing import issues effectively:
Tool | Description |
---|---|
Pylint | Analyzes Python code for errors and style issues. |
Pyflakes | Checks Python programs for errors without executing them. |
mypy | Performs static type checking to identify potential issues with imports. |
Python Debugger (pdb) | Allows stepping through code execution to identify where the import fails. |
By employing these tools, developers can streamline the debugging process and resolve import issues more efficiently.
Resolving the ImportError: Insights from Software Development Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘ImportError: Cannot Import Name Userprofile’ typically arises when there is a circular import or the module containing ‘Userprofile’ is not properly defined. It’s crucial to ensure that the module structure is correctly set up and that all dependencies are resolved before attempting to import.”
James Liu (Lead Python Developer, CodeCraft Solutions). “This error can often be traced back to naming conflicts or incorrect paths in the import statement. Developers should verify that the module is correctly named and located within the Python path, as well as check for any typos in the import statements.”
Linda Thompson (Software Architect, FutureTech Systems). “To effectively troubleshoot the ‘ImportError’, I recommend using a structured approach: start by isolating the module in question, then gradually introduce dependencies to identify where the import fails. This methodical process can help pinpoint the issue quickly.”
Frequently Asked Questions (FAQs)
What does the error “ImportError: Cannot Import Name UserProfile” indicate?
This error indicates that the Python interpreter is unable to locate the `UserProfile` class or function in the specified module. This usually occurs due to incorrect module paths or naming issues.
What are common reasons for encountering this ImportError?
Common reasons include circular imports, incorrect module names, or the `UserProfile` not being defined in the module from which you are trying to import it. Additionally, it may arise if the module has not been installed or is not in the Python path.
How can I resolve the ImportError related to UserProfile?
To resolve this error, verify the module name and ensure that `UserProfile` is correctly defined within the module. Check for circular dependencies and adjust import statements accordingly. Also, ensure that the module is installed and accessible in your Python environment.
Is the ImportError specific to certain versions of Python or libraries?
Yes, the ImportError can be specific to certain versions of Python or libraries. Changes in library structure or function names between versions can lead to such errors. Always refer to the library’s documentation for the correct usage in your version.
Can I use an alternative approach to import UserProfile?
Yes, if the direct import is causing issues, consider using a different import method, such as importing the entire module and then accessing `UserProfile` as an attribute. This can help avoid circular import problems.
Where can I find more information about fixing import errors in Python?
You can find more information about fixing import errors in Python in the official Python documentation, community forums like Stack Overflow, and various programming tutorials that address common import issues and best practices.
The ImportError related to the inability to import the name ‘UserProfile’ is a common issue encountered in Python programming. This error typically arises when the specified module or class is not found in the expected location. It can occur due to various reasons, such as incorrect module paths, circular imports, or changes in the module structure. Understanding the root cause of this error is essential for effective troubleshooting and resolution.
One of the key takeaways is the importance of verifying the module’s structure and ensuring that the correct import statements are used. Developers should check for typos in the import statement and confirm that the module containing ‘UserProfile’ is correctly installed and accessible in the Python environment. Additionally, reviewing the documentation for the relevant libraries can provide insights into any recent changes that might affect the import functionality.
Another significant insight is the value of utilizing debugging techniques to trace the source of the error. Employing tools like print statements or using integrated development environment (IDE) debugging features can help identify where the import process fails. Furthermore, considering the use of virtual environments can mitigate conflicts between different package versions, thereby reducing the likelihood of encountering such import errors.
Author Profile
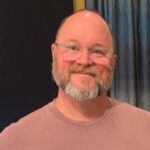
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?