How Can You Code a Website Using Python?
Creating a website can seem like a daunting task, especially if you’re new to the world of programming. However, with the right tools and guidance, you can harness the power of Python to build a dynamic and engaging web presence. Python, known for its simplicity and versatility, has emerged as a popular choice among developers for web development. Whether you’re looking to create a personal blog, a portfolio site, or a full-fledged web application, Python offers a range of frameworks and libraries that make the process not only manageable but also enjoyable.
In this article, we will explore the fundamentals of coding a website using Python. We’ll delve into the various frameworks available, such as Flask and Django, which provide robust structures for building web applications. You’ll learn how Python’s clean syntax and extensive libraries can streamline your development process, allowing you to focus on creativity and functionality rather than getting bogged down in complex code.
As we guide you through the essential steps of web development with Python, you’ll discover how to set up your environment, create interactive features, and deploy your site for the world to see. Whether you’re a complete beginner or looking to expand your programming skills, this article will equip you with the knowledge and confidence to embark on your web development journey. Get ready to unlock the
Choosing a Framework
When coding a website in Python, selecting the right framework is crucial as it provides the structure and tools necessary for development. The most popular frameworks include Flask and Django.
Flask is a micro-framework that is lightweight and modular, making it an excellent choice for small to medium-sized applications. Its simplicity allows developers to add only the components they need.
Django, on the other hand, is a high-level framework that follows the “batteries included” philosophy, providing numerous features out of the box, such as an ORM (Object-Relational Mapping), authentication, and an admin interface. This makes it ideal for larger projects that require scalability and security.
Comparison Table of Frameworks
Feature | Flask | Django |
---|---|---|
Type | Micro-framework | Full-stack framework |
Flexibility | Very High | Moderate |
Built-in Tools | Limited | Extensive |
Learning Curve | Easy | Moderate |
Setting Up the Development Environment
After selecting a framework, the next step is to set up the development environment. This typically involves the following steps:
- Install Python: Ensure that Python is installed on your machine. You can download it from the official Python website.
- Create a Virtual Environment: Using `venv` or `virtualenv`, create a virtual environment to manage dependencies.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use: myenv\Scripts\activate
“`
- Install Framework: Use `pip` to install your chosen framework. For instance, to install Flask or Django, you would run:
“`bash
pip install Flask
or
pip install Django
“`
Creating a Simple Web Application
To illustrate how to code a website in Python, here’s a brief example of creating a simple web application using Flask.
- Create the Application File: Start by creating a file named `app.py`.
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Welcome to My Website!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the Application: Execute the application by running:
“`bash
python app.py
“`
- Access the Web Application: Open your web browser and navigate to `http://127.0.0.1:5000/` to see your website in action.
Adding Templates and Static Files
Enhancing your web application with templates and static files is essential for creating a user-friendly interface. Flask uses Jinja2 as its templating engine, allowing you to create HTML templates that can include dynamic content.
- Create a Templates Folder: Inside your project directory, create a folder named `templates`.
- Create an HTML File: Inside the `templates` folder, create a file named `index.html`.
“`html
Welcome to My Website!
“`
- Render the Template in the Application: Modify your `app.py` to render the template:
“`python
from flask import render_template
@app.route(‘/’)
def home():
return render_template(‘index.html’)
“`
Static files such as CSS or JavaScript should be placed in a folder named `static`, which Flask will serve automatically.
By following these steps, you can establish a solid foundation for coding a website in Python, allowing for further enhancement and expansion of your application.
Choosing the Right Framework
When coding a website in Python, selecting the appropriate web framework is crucial. Each framework has its strengths and is suited for different types of projects. The most popular Python web frameworks include:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. Ideal for complex, data-driven websites.
- Flask: A micro-framework that is lightweight and modular. It is suitable for simpler applications where flexibility is a priority.
- FastAPI: Known for its speed and support for asynchronous programming. Best for building APIs quickly and efficiently.
Framework | Strengths | Best Use Cases |
---|---|---|
Django | Feature-rich, secure | E-commerce, content management |
Flask | Lightweight, flexible | Prototyping, small applications |
FastAPI | Performance, async support | RESTful APIs, microservices |
Setting Up Your Development Environment
To begin coding a website in Python, set up your development environment properly. Follow these steps:
- Install Python: Ensure you have Python installed on your machine. You can download it from the [official Python website](https://www.python.org/downloads/).
- Choose a Code Editor: Popular editors include Visual Studio Code, PyCharm, or Sublime Text.
- Set Up a Virtual Environment:
- Use `venv` or `virtualenv` to create isolated environments for your projects.
- Command: `python -m venv myprojectenv`
- Install Required Packages: Use `pip` to install the chosen framework.
- For Django: `pip install django`
- For Flask: `pip install flask`
- For FastAPI: `pip install fastapi uvicorn`
Creating Your First Web Application
Regardless of the framework chosen, the process of creating a basic web application is fairly similar. Here’s a quick guide for each framework:
Django:
- Create a new project:
“`bash
django-admin startproject myproject
“`
- Run the development server:
“`bash
python manage.py runserver
“`
Flask:
- Create a new file, `app.py`, and add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the application:
“`bash
python app.py
“`
FastAPI:
- Create a new file, `main.py`, and add:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
def read_root():
return {“Hello”: “World”}
“`
- Run the application:
“`bash
uvicorn main:app –reload
“`
Routing and Handling Requests
Routing is essential for managing URLs and directing requests to the appropriate functions. Each framework has its own way of handling this.
- Django: Define URLs in `urls.py`:
“`python
from django.urls import path
from .views import home
urlpatterns = [
path(”, home, name=’home’),
]
“`
- Flask: Use decorators to define routes directly in your application code:
“`python
@app.route(‘/about’)
def about():
return “About Page”
“`
- FastAPI: Use Python function annotations to define routes:
“`python
@app.get(“/items/{item_id}”)
def read_item(item_id: int):
return {“item_id”: item_id}
“`
Database Integration
Integrating a database is vital for dynamic applications. Common choices include SQLite, PostgreSQL, and MySQL. Here’s a brief overview of how to connect to a database in each framework:
- Django: Django comes with an ORM and supports various databases. Configure your database settings in `settings.py`.
- Flask: Use SQLAlchemy or Flask-SQLAlchemy for ORM support. Example configuration:
“`python
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy(app)
“`
- FastAPI: Use SQLAlchemy or databases for async support. An example connection might look like:
“`python
from sqlalchemy import create_engine
engine = create_engine(“sqlite:///./test.db”)
“`
These frameworks provide extensive documentation and communities that can support you as you build your website.
Expert Insights on Coding a Website in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When coding a website in Python, it is essential to choose the right framework. Django and Flask are two of the most popular options, each offering unique advantages. Django is excellent for larger applications due to its built-in features, while Flask provides more flexibility for smaller projects.”
Michael Chen (Full-Stack Developer, CodeCraft Solutions). “Understanding the fundamentals of web development, including HTML, CSS, and JavaScript, is crucial before diving into Python. This foundational knowledge will enhance your ability to integrate Python with front-end technologies effectively.”
Lisa Patel (Web Development Instructor, Future Coders Academy). “For beginners, I recommend starting with Flask for its simplicity and ease of learning. As you become more comfortable with Python, transitioning to Django can provide a more structured approach to building complex web applications.”
Frequently Asked Questions (FAQs)
How can I start coding a website using Python?
To start coding a website using Python, you should choose a web framework such as Flask or Django. Install the framework, set up a virtual environment, and create a basic project structure. Begin by defining routes and views to handle user requests.
What are the best Python frameworks for web development?
The best Python frameworks for web development include Django, Flask, FastAPI, and Pyramid. Django is ideal for large applications with built-in features, while Flask is suited for smaller, more flexible projects. FastAPI is excellent for building APIs quickly.
Do I need to know HTML and CSS to code a website in Python?
Yes, knowledge of HTML and CSS is essential for coding a website in Python. HTML structures the content, while CSS styles it. Understanding these languages allows you to create visually appealing and functional web applications.
Can I use Python for front-end development?
Python is primarily a back-end language; however, frameworks like Brython and PyScript allow you to write front-end code in Python. These tools enable Python to interact with the browser, but traditional front-end languages like JavaScript are still more widely used.
How do I deploy a Python web application?
To deploy a Python web application, choose a hosting service that supports Python, such as Heroku, AWS, or DigitalOcean. Prepare your application by configuring the environment, setting up a database, and ensuring all dependencies are installed. Finally, upload your code and start the application.
What databases can I use with Python web applications?
You can use various databases with Python web applications, including PostgreSQL, MySQL, SQLite, and MongoDB. The choice of database depends on your application requirements and the framework you are using, as most frameworks provide ORM support for these databases.
In summary, coding a website in Python involves understanding various frameworks and tools that facilitate web development. Popular frameworks such as Flask and Django provide robust structures for building web applications, allowing developers to focus on functionality and user experience. Flask is known for its simplicity and flexibility, making it ideal for smaller projects, while Django offers a more comprehensive solution with built-in features suitable for larger applications.
Additionally, mastering HTML, CSS, and JavaScript is essential for front-end development, as these technologies work in conjunction with Python to create interactive and visually appealing websites. Integrating Python with front-end technologies can be achieved through various methods, such as using RESTful APIs or WebSocket for real-time communication, enhancing the overall user experience.
Furthermore, understanding deployment strategies is crucial for making a website accessible to users. Utilizing cloud services like Heroku or AWS can simplify the process of deploying Python web applications, ensuring scalability and reliability. Testing and debugging are also integral parts of the development process, ensuring that the website functions as intended across different environments and devices.
Ultimately, coding a website in Python is a multifaceted endeavor that requires a blend of programming skills, knowledge of web technologies, and an understanding of deployment practices. By leveraging the right
Author Profile
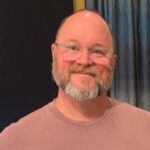
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?