Why Am I Getting a ‘Couldn’t Convert String to Float’ Error in Python?
In the world of programming, few errors can be as perplexing as the dreaded “Couldn’t Convert String to Float” message in Python. For both novice and seasoned developers, this error can halt progress and spark frustration, especially when dealing with data manipulation or numerical computations. Understanding the nuances of data types in Python is crucial, as this language’s dynamic typing can lead to unexpected challenges when converting between types. In this article, we will unravel the mystery behind this common error, explore its causes, and equip you with the knowledge to troubleshoot and resolve it effectively.
At its core, the “Couldn’t Convert String to Float” error arises when Python encounters a string that it cannot interpret as a floating-point number. This can happen for a variety of reasons, such as the presence of non-numeric characters, improper formatting, or even whitespace issues. As data scientists and developers increasingly rely on data input from various sources, the importance of ensuring data integrity cannot be overstated. A solid grasp of how to handle and convert data types will not only enhance your coding skills but also improve the reliability of your applications.
Throughout this article, we will delve into the common pitfalls that lead to this error, provide practical examples, and share best practices for converting strings to floats seamlessly. Whether you’re cleaning up datasets
Understanding the Error
When you encounter the error message “couldn’t convert string to float” in Python, it typically indicates that the code is attempting to convert a string into a floating-point number, but the string is not formatted correctly for this conversion. This often occurs when the string contains characters that are not numbers, such as letters or symbols.
Common causes of this error include:
- Inconsistent number formats (e.g., using commas instead of periods).
- Presence of non-numeric characters (e.g., alphabetic characters, currency symbols).
- Leading or trailing spaces in the string.
To diagnose the issue, you can print the string before the conversion attempt to ensure it is in the expected format.
Handling the Error
To effectively handle this error, consider employing techniques to sanitize and validate your input before attempting the conversion. Here are some strategies:
- Trim whitespace: Use the `strip()` method to remove any leading or trailing spaces from the string.
- Check for numeric content: Use the `str.isdigit()` method or regular expressions to ensure the string contains only valid numeric characters.
- Replace invalid characters: If the string contains commas or currency symbols, replace them with appropriate characters or remove them entirely.
Here’s an example of how to implement these strategies:
“`python
input_string = “$1,234.56”
clean_string = input_string.replace(“$”, “”).replace(“,”, “”).strip()
try:
float_value = float(clean_string)
except ValueError as e:
print(f”Error: {e}”)
“`
Common Scenarios
The error can arise in several contexts. Understanding these scenarios can help mitigate the risk of encountering the error:
Scenario | Description |
---|---|
User Input | When accepting input from users, they may enter invalid data. |
File Reading | Reading data from files that are not properly formatted. |
Data Parsing | Parsing data from APIs or web scraping where formats vary. |
For instance, when processing user input, consider implementing input validation to check whether the input is numeric before attempting conversion. This can significantly reduce the likelihood of encountering the error.
Debugging Techniques
If you encounter the error, use the following debugging techniques:
- Print Statements: Add print statements before the conversion to display the string being processed.
- Try-Except Blocks: Enclose your conversion logic within a try-except block to catch and handle exceptions gracefully.
Example:
“`python
input_string = “123abc”
try:
float_value = float(input_string)
except ValueError as e:
print(f”Conversion failed for ‘{input_string}’: {e}”)
“`
By implementing these practices, you can minimize the chances of running into conversion errors and make your code more robust.
Understanding the Error
The error message “Couldn’t convert string to float” in Python typically arises when you attempt to convert a non-numeric string into a float. This can occur in various scenarios, such as when reading data from files, user input, or when processing data structures like lists and dictionaries. The root cause is often an unexpected character or format within the string.
Common reasons for this error include:
- Presence of non-numeric characters (e.g., letters, punctuation).
- Whitespace or leading/trailing spaces.
- Use of commas instead of decimal points (e.g., “1,234.56” instead of “1234.56”).
- Empty strings or `None` values.
Identifying the Problematic Data
To troubleshoot the issue, you should identify which string is causing the conversion failure. This can be achieved by employing a try-except block around your conversion attempts:
“`python
data = [“12.5”, “not_a_number”, “3.14”, ” “, “42”]
for item in data:
try:
value = float(item)
print(f”Converted ‘{item}’ to {value}”)
except ValueError as e:
print(f”Error converting ‘{item}’: {e}”)
“`
This code snippet will output the problematic strings, allowing you to pinpoint the source of the error.
Data Cleaning Techniques
To handle strings that cannot be converted directly to floats, you can implement several data cleaning techniques:
- Stripping Whitespace: Use the `.strip()` method to remove leading and trailing spaces.
- Replacing Commas: Use `.replace(‘,’, ”)` to eliminate commas from the string.
- Filtering Non-Numeric Characters: Create a function to retain only valid numeric characters.
Example of a cleaning function:
“`python
def clean_string(s):
s = s.strip() Remove whitespace
s = s.replace(‘,’, ”) Remove commas
try:
return float(s) Attempt to convert to float
except ValueError:
return None Return None for non-convertible strings
“`
Practical Example
Consider a scenario where you read numeric values from a CSV file. You may encounter strings that need cleaning before conversion. Here’s an example using the `pandas` library:
“`python
import pandas as pd
Sample DataFrame
data = {‘numbers’: [‘100’, ‘200.5’, ‘not_a_number’, ‘300’, ”]}
df = pd.DataFrame(data)
Clean and convert
df[‘cleaned_numbers’] = df[‘numbers’].apply(clean_string)
print(df)
“`
This will result in a DataFrame where the `cleaned_numbers` column contains floats or `None` for non-convertible entries.
Original | Cleaned |
---|---|
100 | 100.0 |
200.5 | 200.5 |
not_a_number | None |
300 | 300.0 |
None |
Best Practices
To avoid encountering the “Couldn’t convert string to float” error, consider the following best practices:
- Validate input data before processing.
- Use exception handling to manage conversion errors gracefully.
- Employ data cleaning functions to preprocess strings before conversion.
- Utilize libraries like `pandas` for more robust data handling and conversion capabilities.
By adhering to these practices, you can significantly reduce the likelihood of encountering this error in your Python applications.
Expert Insights on Handling String to Float Conversion Errors in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The error ‘Couldn’t convert string to float’ typically arises when the string contains non-numeric characters. It is crucial to sanitize input data before conversion, ensuring that only valid numeric strings are processed. Implementing robust error handling can significantly reduce the occurrence of this issue.”
Michael Chen (Software Engineer, CodeCraft Solutions). “When dealing with user input, it’s essential to validate and clean the data. Utilizing functions like `str.replace()` to remove unwanted characters, or employing regular expressions, can help in converting strings to floats more reliably. A proactive approach to data validation can prevent runtime errors.”
Sarah Thompson (Python Developer, DataFlow Analytics). “Understanding the context of the data is vital. Sometimes, the string may represent a number in a different format, such as currency or scientific notation. Using the `locale` module or custom parsing functions can facilitate the correct conversion of such strings to floats without raising errors.”
Frequently Asked Questions (FAQs)
What does the error “Couldn’t convert string to float” mean in Python?
This error occurs when you attempt to convert a string that does not represent a valid floating-point number into a float type. It indicates that the string contains invalid characters or formatting.
How can I troubleshoot the “Couldn’t convert string to float” error?
To troubleshoot this error, inspect the string you are trying to convert. Ensure it contains only numeric characters, a decimal point, and possibly a leading minus sign for negative numbers. Remove any extraneous characters or whitespace.
Can I convert a string with commas to a float in Python?
No, a string containing commas cannot be directly converted to a float. You must first remove the commas using the `replace()` method before conversion, e.g., `float(string.replace(‘,’, ”))`.
What should I do if my string contains spaces?
If your string contains spaces, use the `strip()` method to remove leading and trailing whitespace before attempting to convert it to a float, e.g., `float(string.strip())`.
Is it possible to handle this error using exception handling?
Yes, you can use a try-except block to handle this error gracefully. This allows you to catch the `ValueError` and take appropriate action, such as prompting the user for valid input.
What are some common causes of this error in data processing?
Common causes include non-numeric characters in the string, incorrect formatting (e.g., using commas or currency symbols), and unexpected whitespace. Always validate and clean your data before conversion.
The error message “Couldn’t convert string to float” in Python typically arises when attempting to convert a string that does not represent a valid floating-point number into a float type. This conversion issue is commonly encountered when dealing with user input, data files, or external data sources where the format may not be as expected. Understanding the root causes of this error is crucial for effective debugging and data handling in Python applications.
One of the primary reasons for this error is the presence of non-numeric characters in the string. For instance, strings that contain letters, special characters, or even whitespace can lead to this conversion failure. It is essential to sanitize and validate the input data before attempting the conversion. Techniques such as using regular expressions or built-in string methods can help clean the data to ensure it is in a proper format for conversion.
Another important takeaway is the significance of exception handling in Python. Utilizing try-except blocks allows developers to gracefully manage conversion errors and implement fallback mechanisms or user notifications. This practice not only improves the robustness of the code but also enhances the user experience by providing clear feedback when errors occur.
effectively managing the “Couldn’t convert string to float” error involves a combination of input validation, data sanit
Author Profile
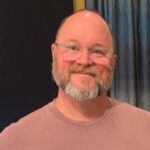
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?