What Is Scripting in Python and How Can It Enhance Your Programming Skills?
What Is Scripting In Python?
In the ever-evolving landscape of programming, Python stands out as a versatile and powerful language that has captured the hearts of developers around the globe. One of its most compelling features is its ability to facilitate scripting—a process that allows programmers to automate tasks, manipulate data, and create dynamic applications with ease. Whether you’re a seasoned developer or a curious beginner, understanding scripting in Python opens up a world of possibilities, enabling you to streamline workflows and enhance productivity.
At its core, scripting in Python involves writing small, simple programs, or scripts, that perform specific tasks. These scripts can range from automating mundane file management processes to developing complex web applications. The beauty of Python scripting lies in its readability and simplicity, making it an accessible choice for those looking to dive into the world of programming. With a rich ecosystem of libraries and frameworks, Python empowers users to tackle a wide variety of challenges, from data analysis to web scraping, all while maintaining a clean and efficient codebase.
As we delve deeper into the nuances of Python scripting, we will explore its fundamental principles, practical applications, and the tools that make it an essential skill for any aspiring programmer. Whether you aim to optimize your daily tasks or embark on ambitious projects, mastering Python scripting can
Understanding Python Scripting
Python scripting refers to the process of writing a series of commands in a file that Python can execute sequentially. This method allows for the automation of tasks, manipulation of data, and implementation of complex algorithms. Python scripts are typically saved with a `.py` extension, making them easy to identify and execute.
The flexibility of Python as a scripting language makes it popular for various applications, including:
- Web development
- Data analysis and visualization
- Automation of system tasks
- Game development
- Machine learning and artificial intelligence
Advantages of Python Scripting
Python scripting offers several benefits that enhance productivity and simplify coding tasks:
- Simplicity and Readability: Python’s syntax closely resembles the English language, which makes it accessible for beginners and enhances code readability.
- Extensive Libraries: Python boasts a rich ecosystem of libraries and frameworks, such as NumPy for numerical computations and Flask for web applications, which accelerate development.
- Cross-Platform Compatibility: Python scripts can run on various operating systems, including Windows, macOS, and Linux, without modification.
- Interactivity: Python can be executed in an interactive mode, allowing for quick testing and iteration during script development.
Common Uses of Python Scripts
Python scripts are versatile and can be utilized in numerous domains. Some common use cases include:
- Data Analysis: Automating data extraction, transformation, and loading (ETL) processes.
- Web Scraping: Collecting data from websites using libraries like Beautiful Soup and Scrapy.
- System Administration: Automating routine tasks such as file management and user account creation.
- Game Development: Creating simple games or prototypes using libraries like Pygame.
Python Scripting Basics
To create a Python script, follow these fundamental steps:
- Set Up the Environment: Ensure Python is installed on your machine. Use a text editor or an Integrated Development Environment (IDE) for coding.
- Write the Script: Begin coding by defining functions, importing necessary libraries, and writing the main logic.
- Save the File: Save your work with a `.py` extension.
- Run the Script: Execute the script from the command line or terminal using the command `python script_name.py`.
Sample Python Script
Here’s a simple example of a Python script that prints “Hello, World!” and calculates the sum of two numbers:
python
# Hello World Script
print(“Hello, World!”)
# Sum Function
def add_numbers(a, b):
return a + b
# Example of using the function
result = add_numbers(5, 10)
print(“The sum is:”, result)
Python Scripting Tools
Various tools can enhance the Python scripting experience. Below is a comparison of popular IDEs:
IDE | Features | Pros | Cons |
---|---|---|---|
PyCharm | Smart code assistance, debugging, testing tools | Robust features, great for professional development | Heavy resource usage |
Visual Studio Code | Extensible with plugins, integrated terminal | Lightweight, customizable, free | May require setup for Python support |
Jupyter Notebook | Interactive coding, inline visualizations | Great for data analysis and presentation | Not ideal for large projects |
By leveraging the advantages and tools associated with Python scripting, developers can create efficient, maintainable, and scalable scripts tailored to their specific needs.
Understanding Scripting in Python
Scripting in Python refers to the process of writing small programs or scripts that automate tasks, perform data manipulation, or manage system operations. Python’s simplicity and readability make it an ideal choice for scripting, allowing developers to write efficient code with minimal overhead.
Key Characteristics of Python Scripting
- Interpreted Language: Python is an interpreted language, meaning scripts are executed line-by-line at runtime. This facilitates quick testing and debugging.
- High-Level Language: Python abstracts many complex details of the computer’s operation, allowing developers to focus on problem-solving rather than low-level coding.
- Dynamic Typing: Variables in Python do not require explicit declaration, making the code more flexible and easier to write.
- Rich Libraries: Python offers a wide range of libraries and frameworks that simplify common scripting tasks, such as file manipulation, web scraping, and data analysis.
Common Use Cases for Python Scripting
Python scripting is utilized across various domains, including:
- Automation: Automate repetitive tasks such as file organization, data entry, or web scraping.
- Data Analysis: Perform data manipulation and analysis using libraries like Pandas and NumPy.
- Web Development: Generate dynamic web content or automate web service interactions.
- System Administration: Manage system operations, such as monitoring server performance or automating backup processes.
Basic Structure of a Python Script
A typical Python script consists of the following components:
Component | Description |
---|---|
Shebang | `#!/usr/bin/env python3` to specify the interpreter. |
Imports | Import necessary libraries using `import` statements. |
Main Function | Define the main function using `def main():` to encapsulate logic. |
Code Logic | Implement the core functionality of the script. |
Execution Block | Use `if __name__ == “__main__”:` to execute the main function. |
Example of a simple Python script:
python
#!/usr/bin/env python3
import os
def main():
print(“Current directory contents:”)
for file in os.listdir(‘.’):
print(file)
if __name__ == “__main__”:
main()
Advantages of Using Python for Scripting
- Ease of Learning: Python’s syntax is straightforward, making it accessible to beginners.
- Versatility: Suitable for various tasks from simple scripts to complex applications.
- Community Support: A strong community provides extensive documentation and third-party modules.
- Cross-Platform: Python scripts can run on different operating systems without modification.
Best Practices in Python Scripting
- Code Readability: Follow PEP 8 style guidelines to ensure code is clean and understandable.
- Modular Design: Break scripts into functions or modules for better organization and reusability.
- Error Handling: Use try-except blocks to gracefully handle exceptions and errors.
- Documentation: Comment code adequately and provide docstrings for functions to enhance clarity.
By leveraging these characteristics and practices, developers can harness the full potential of Python scripting to create efficient, maintainable, and powerful scripts tailored to their needs.
Understanding the Role of Scripting in Python Development
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Scripting in Python is essential for automating repetitive tasks and simplifying complex workflows. It enables developers to write concise code that can be executed without the need for compilation, making it a powerful tool for rapid prototyping and development.”
Michael Chen (Lead Python Developer, Data Solutions Group). “The versatility of Python scripting allows it to be used across various domains, from web development to data analysis. This flexibility is what makes Python a preferred choice for many developers looking to streamline their processes and enhance productivity.”
Sarah Thompson (Technical Instructor, Python Academy). “Understanding scripting in Python is crucial for new programmers. It not only helps them grasp fundamental programming concepts but also prepares them for more complex software development challenges in the future.”
Frequently Asked Questions (FAQs)
What is scripting in Python?
Scripting in Python refers to writing small programs or scripts that automate tasks, manipulate data, or perform specific functions without the need for compilation. Python scripts are typically executed by the Python interpreter.
How does Python differ from other programming languages in scripting?
Python is known for its simplicity and readability, which makes it an excellent choice for scripting. Unlike some languages that require extensive setup or complex syntax, Python allows developers to write concise and clear scripts quickly.
What are common use cases for Python scripting?
Common use cases include automating repetitive tasks, data analysis, web scraping, system administration, and developing simple web applications. Python’s extensive libraries enhance its capabilities in these areas.
Can Python scripts be used for web development?
Yes, Python scripts can be used in web development. Frameworks like Flask and Django allow developers to create dynamic web applications, handling backend logic through Python scripts.
Is Python scripting suitable for beginners?
Absolutely. Python’s straightforward syntax and extensive documentation make it an ideal language for beginners to learn scripting and programming concepts effectively.
What tools are available for writing and executing Python scripts?
Popular tools include integrated development environments (IDEs) like PyCharm and Visual Studio Code, as well as text editors like Sublime Text and Atom. The Python interpreter itself can also be used to execute scripts directly from the command line.
Scripting in Python refers to the process of writing small programs or scripts that automate tasks, manipulate data, or perform specific functions. Python’s simplicity and readability make it an ideal choice for scripting, allowing developers to write code quickly and efficiently. The language supports various libraries and frameworks that enhance its scripting capabilities, enabling users to execute complex tasks with minimal code.
One of the key advantages of using Python for scripting is its versatility. Python scripts can be used for web development, data analysis, automation of repetitive tasks, and even in scientific computing. The extensive standard library and third-party modules available in Python further empower users to extend their scripts’ functionality, making it a powerful tool for both beginners and experienced programmers.
Another important aspect of Python scripting is its cross-platform compatibility. Scripts written in Python can run on different operating systems without modification, which is particularly beneficial for developers working in diverse environments. This feature, combined with Python’s strong community support and extensive documentation, makes it an accessible and effective choice for scripting tasks.
scripting in Python offers a robust solution for automating tasks and enhancing productivity. Its ease of use, versatility, and cross-platform capabilities make it a preferred language for many developers. As Python continues
Author Profile
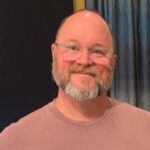
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?