How Can You Easily Install Third-Party Libraries in Python?
In the ever-evolving world of programming, Python stands out as a versatile and powerful language, beloved by developers for its simplicity and rich ecosystem. One of the key features that enhance Python’s appeal is its ability to integrate third-party libraries, which can significantly extend its functionality and streamline development processes. Whether you’re building web applications, data analysis tools, or machine learning models, knowing how to install and manage these libraries is crucial for unleashing Python’s full potential. In this article, we’ll explore the ins and outs of installing third-party libraries in Python, empowering you to enhance your projects with ease.
To get started, it’s important to understand what third-party libraries are and why they are essential in Python development. These libraries are collections of pre-written code that provide additional functionality, allowing developers to avoid reinventing the wheel. From popular libraries like NumPy and Pandas for data manipulation to Flask and Django for web development, the Python Package Index (PyPI) offers a treasure trove of resources that can simplify complex tasks. However, navigating the installation process can be daunting for newcomers, which is why a clear understanding of the methods available is vital.
In this article, we will delve into various ways to install third-party libraries in Python, covering everything from
Using pip to Install Third-Party Libraries
The most common method for installing third-party libraries in Python is through the package manager called pip. Pip is included by default with Python versions 3.4 and later. To use pip, you will need to open your command line interface (CLI) and follow these steps:
- Ensure that pip is installed by running:
“`
pip –version
“`
- To install a library, use the command:
“`
pip install library_name
“`
- Replace `library_name` with the actual name of the library you wish to install.
For example, to install the popular Requests library, you would type:
“`
pip install requests
“`
You can also specify a version of the library using:
“`
pip install library_name==version_number
“`
Installing from a Requirements File
In many projects, you may encounter a `requirements.txt` file that lists all the dependencies needed for that project. You can install all the libraries listed in this file using:
“`
pip install -r requirements.txt
“`
This command reads each line in the `requirements.txt` file and installs the listed libraries. An example of a `requirements.txt` file might look like this:
“`
requests==2.25.1
numpy==1.19.5
pandas==1.2.1
“`
Using Virtual Environments
Creating a virtual environment is highly recommended when working with third-party libraries to avoid conflicts between packages. Virtual environments allow you to manage dependencies for different projects separately. You can create a virtual environment using the following commands:
- Install the `virtualenv` package if you don’t have it:
“`
pip install virtualenv
“`
- Create a new virtual environment:
“`
virtualenv myenv
“`
- Activate the virtual environment:
- On Windows:
“`
myenv\Scripts\activate
“`
- On macOS/Linux:
“`
source myenv/bin/activate
“`
Once the virtual environment is activated, you can install libraries as usual using pip, and they will be contained within that environment.
Using Conda for Package Management
An alternative to pip is Conda, which is particularly useful for data science and scientific computing. If you are using Anaconda or Miniconda, you can manage packages using the conda command. Installation of libraries can be done with:
“`
conda install library_name
“`
For example, to install the NumPy library, you would use:
“`
conda install numpy
“`
Conda also allows you to create isolated environments similar to virtualenv. You can create a new environment with:
“`
conda create –name myenv
“`
And activate it using:
“`
conda activate myenv
“`
Common Installation Issues
While installing third-party libraries, you may encounter several common issues:
Issue | Solution |
---|---|
Permission Denied | Use `sudo` on Linux/macOS or run as Admin on Windows. |
Library Not Found | Ensure the library name is spelled correctly. |
Incompatible Python Version | Check the library documentation for compatible Python versions. |
Missing Dependencies | Install any required dependencies listed in the library documentation. |
By following these guidelines, you can effectively manage and install third-party libraries in Python, ensuring a smoother development experience.
Installing Third-Party Libraries Using pip
The most common method for installing third-party libraries in Python is through the package manager `pip`. This tool simplifies the process, allowing users to easily download and manage libraries from the Python Package Index (PyPI).
To install a library using pip, follow these steps:
- Open your command line interface (CLI):
- On Windows, you can use Command Prompt or PowerShell.
- On macOS or Linux, use the Terminal.
- Use the pip install command:
The syntax is straightforward:
“`bash
pip install
- Example:
To install the popular requests library, you would run:
“`bash
pip install requests
“`
- Installing Specific Versions:
If you need a specific version of a library, specify it as follows:
“`bash
pip install
“`
For example:
“`bash
pip install requests==2.25.1
“`
- Upgrading Libraries:
To upgrade an existing library to the latest version, use:
“`bash
pip install –upgrade
- Installing from Requirements File:
If you have multiple libraries to install, you can create a `requirements.txt` file listing all libraries and their versions, then run:
“`bash
pip install -r requirements.txt
“`
Using Virtual Environments
It is highly recommended to use virtual environments to manage project dependencies. This prevents conflicts between libraries required by different projects.
- Creating a Virtual Environment:
- Navigate to your project directory:
“`bash
cd /path/to/your/project
“`
- Create a virtual environment:
“`bash
python -m venv venv
“`
- Activating the Virtual Environment:
- On Windows:
“`bash
venv\Scripts\activate
“`
- On macOS and Linux:
“`bash
source venv/bin/activate
“`
- Installing Libraries in the Virtual Environment:
Once activated, any `pip install` commands will be confined to this environment, keeping your global Python environment clean.
Common Issues and Troubleshooting
While installing third-party libraries, users may encounter common issues. Here are some solutions:
Issue | Solution |
---|---|
`pip` not recognized | Ensure Python is added to your system PATH. |
Permission denied | Use `sudo pip install |
Outdated `pip` version | Upgrade `pip` using `pip install –upgrade pip`. |
Incompatible library version | Check library documentation for compatible versions. Use `pip install |
By adhering to these methods and practices, you can efficiently install and manage third-party libraries in your Python projects.
Expert Insights on Installing Third-Party Libraries in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Installing third-party libraries in Python is a straightforward process, primarily facilitated by package managers like pip. However, it’s crucial to ensure that you are using a virtual environment to avoid dependency conflicts and maintain a clean workspace.”
Michael Thompson (Python Developer Advocate, CodeCraft). “While pip is the go-to tool for installing libraries, understanding the requirements.txt file and how to manage it effectively can significantly enhance your project management. This practice allows for easier collaboration and deployment.”
Sarah Lee (Open Source Contributor and Educator, Python Community Forum). “When installing third-party libraries, always review the library’s documentation and check for compatibility with your Python version. This diligence can save you from potential issues down the line.”
Frequently Asked Questions (FAQs)
How do I install third-party libraries in Python?
You can install third-party libraries in Python using the package manager `pip`. Open your command line interface and run the command `pip install library_name`, replacing `library_name` with the name of the library you wish to install.
What is pip and how does it work?
`pip` is the package installer for Python, allowing users to install and manage additional libraries and dependencies that are not part of the standard Python library. It connects to the Python Package Index (PyPI) to download and install packages.
Can I install multiple libraries at once using pip?
Yes, you can install multiple libraries simultaneously by listing them in a single command. For example, `pip install library1 library2 library3` will install all specified libraries at once.
What should I do if I encounter a permissions error while installing a library?
If you encounter a permissions error, you can try running the command with elevated privileges by using `sudo pip install library_name` on Unix-based systems or running the command prompt as an administrator on Windows.
How can I verify if a library has been installed successfully?
You can verify the installation of a library by running the command `pip show library_name`. This command will display details about the installed library, including its version and location.
Is it possible to uninstall a third-party library in Python?
Yes, you can uninstall a library using the command `pip uninstall library_name`. This command will remove the specified library from your Python environment.
In summary, installing third-party libraries in Python is a straightforward process that significantly enhances the functionality of your projects. The most common method for installation is through the Python Package Index (PyPI) using the package manager pip. By executing a simple command in the terminal or command prompt, users can easily download and install the desired libraries, making it an efficient way to extend Python’s capabilities.
Additionally, it is important to manage dependencies effectively. Using virtual environments, such as venv or conda, allows developers to create isolated spaces for different projects. This practice prevents conflicts between library versions and ensures that each project maintains its own set of dependencies, leading to a more organized and manageable development process.
Furthermore, understanding how to search for and verify libraries is crucial. Utilizing resources like PyPI’s website or GitHub can help users find reliable and well-maintained libraries. Reading documentation and checking user reviews can also guide developers in selecting the most suitable libraries for their needs, ensuring a smoother integration into their projects.
Author Profile
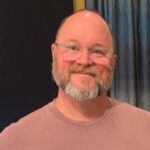
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?