Why Am I Seeing the Error ‘Cannot Find Module Or Its Corresponding Type Declarations’ in My TypeScript Project?
In the fast-paced world of software development, encountering errors can be both frustrating and enlightening. One common issue that developers, especially those using TypeScript, often face is the dreaded error message: “Cannot find module or its corresponding type declarations.” This seemingly cryptic message can halt progress and lead to hours of debugging. However, understanding the root causes and solutions to this problem is essential for maintaining productivity and ensuring a smooth development experience. In this article, we will delve into the intricacies of this error, exploring its implications and providing insights on how to effectively resolve it.
Overview
The “Cannot find module or its corresponding type declarations” error typically arises when TypeScript is unable to locate the necessary type definitions for a module you are trying to import. This can happen for various reasons, such as missing type declaration files, incorrect import paths, or even the absence of the module itself. As TypeScript enforces strict typing, it requires clear definitions to ensure that the code behaves as expected, making this error particularly significant for developers aiming for type safety.
Navigating this issue involves understanding both the TypeScript configuration and the structure of your project. By addressing the underlying causes, developers can not only resolve the immediate error but also enhance their overall coding practices. Whether you
Understanding the Error
The error message “Cannot find module or its corresponding type declarations” typically occurs in TypeScript projects when the compiler is unable to locate a module that is being imported. This can arise due to several reasons such as missing dependencies, incorrect paths, or misconfigured TypeScript settings.
Common scenarios that lead to this error include:
- The module has not been installed.
- The module’s TypeScript definitions are not available or are not installed.
- The path used in the import statement is incorrect.
- The TypeScript configuration (`tsconfig.json`) does not include the necessary paths.
Resolving the Error
To address this error, consider the following steps:
- Install Missing Dependencies: Ensure that the required module is installed in your project. You can do this using npm or yarn:
“`bash
npm install module-name
“`
or
“`bash
yarn add module-name
“`
- Check Type Definitions: If the module does not come with its own TypeScript definitions, you might need to install them separately. For many popular libraries, you can find type definitions in the DefinitelyTyped repository. Install them using:
“`bash
npm install @types/module-name –save-dev
“`
- Verify Import Paths: Check that the import statement references the correct module path. Ensure that any relative paths are correctly pointing to the files or modules.
Example of a correct import statement:
“`typescript
import { MyComponent } from ‘./components/MyComponent’;
“`
- Update tsconfig.json: Ensure your TypeScript configuration file is set up to include all necessary files and modules. The `include` and `exclude` properties should be reviewed to make sure they allow the necessary files.
Example `tsconfig.json` settings:
“`json
{
“compilerOptions”: {
“target”: “es5”,
“module”: “commonjs”,
“strict”: true,
“esModuleInterop”: true
},
“include”: [“src/**/*”],
“exclude”: [“node_modules”, “**/*.spec.ts”]
}
“`
Common Troubleshooting Steps
If the error persists after following the above steps, consider the following additional troubleshooting methods:
- Clear Node Modules: Sometimes, clearing out the `node_modules` folder and reinstalling all dependencies can resolve hidden issues.
“`bash
rm -rf node_modules
npm install
“`
- Check for Typos: Review your import statements for any typographical errors, as even a small mistake can lead to this error.
- Use Correct Module Syntax: Ensure that you are using the correct import syntax based on the module’s export type (CommonJS vs. ES Modules).
- Rebuild the Project: Sometimes, rebuilding the TypeScript project can help clear out any lingering issues.
“`bash
tsc –build
“`
Example Table of Solutions
Problem | Solution |
---|---|
Module not found | Install the module using npm or yarn. |
Missing type definitions | Install type definitions with @types/module-name. |
Incorrect import path | Double-check and correct the import statement. |
tsconfig.json misconfiguration | Update include/exclude settings in tsconfig.json. |
By following these guidelines, you can effectively troubleshoot and resolve the “Cannot find module or its corresponding type declarations” error in your TypeScript projects.
Common Causes of Module Not Found Errors
Several factors can lead to the error message “Cannot find module or its corresponding type declarations.” Understanding these causes is crucial for troubleshooting effectively.
- Missing Dependencies: The module might not be installed. Check your `package.json` file to confirm that the dependency is listed.
- Incorrect Path: The import statement may contain a typo or incorrect relative path.
- Type Declaration Files: If you are using TypeScript and the module lacks type definitions, TypeScript will throw this error.
- Node Module Resolution: Issues with how Node.js resolves modules can arise, especially if the module is located in a non-standard directory.
- Version Compatibility: The installed version of the module may not be compatible with your application or TypeScript settings.
Resolving the Error
To fix the “Cannot find module or its corresponding type declarations” error, consider the following steps:
- Install the Missing Module:
- Use npm or yarn to install the missing package.
“`bash
npm install
“`
or
“`bash
yarn add
“`
- Check Import Paths:
- Verify that the import paths in your files are correct. For example:
“`typescript
import { example } from ‘./path/to/module’;
“`
- Add Type Declarations:
- If the module does not have type definitions, you can create a declaration file. For instance:
“`typescript
// Declare a module if it doesn’t have type definitions
declare module ‘module-name’;
“`
- Place this declaration in a `.d.ts` file.
- Inspect `tsconfig.json`:
- Ensure that the `tsconfig.json` file is configured correctly, particularly the `include`, `exclude`, and `compilerOptions` fields.
- Node Resolution Configuration:
- Check the `NODE_PATH` environment variable, which can affect module resolution. Set it to include the directory where your modules are located.
Using TypeScript with JavaScript Libraries
When integrating JavaScript libraries into TypeScript projects, consider the following guidelines:
- Install Types: For many popular libraries, type definitions are available from DefinitelyTyped. Install them using:
“`bash
npm install @types/
- No Type Definitions Available: If no types exist for a library, you can either:
- Create a custom `.d.ts` file, or
- Use `any` type to bypass type checking:
“`typescript
declare module ‘library-name’ {
const value: any;
export default value;
}
“`
Example of a Type Declaration File
Here is a simple example of what a type declaration file might look like:
“`typescript
// my-library.d.ts
declare module ‘my-library’ {
export function myFunction(param: string): number;
export const myConstant: string;
}
“`
This file should be placed in a location where TypeScript can find it, usually alongside your source files or in a dedicated `typings` directory.
Debugging Techniques
Effective debugging can expedite the resolution of module errors:
- Console Logging: Use console logs to trace the execution flow and identify where the error occurs.
- Check Installed Packages: List all installed packages and their versions:
“`bash
npm list –depth=0
“`
- Use TypeScript Compiler: Run the TypeScript compiler with the `–noEmit` flag to catch errors without generating output files:
“`bash
tsc –noEmit
“`
- Node.js Version: Ensure your Node.js version supports the features used in your modules. Check compatibility with the libraries in use.
Expert Insights on Resolving Module Declaration Issues
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Cannot Find Module Or Its Corresponding Type Declarations’ often arises in TypeScript projects due to missing type definitions. It is crucial to ensure that all necessary type declaration files are installed and properly referenced in your tsconfig.json file.”
Michael Chen (Lead TypeScript Developer, CodeCraft Solutions). “This issue can frequently be resolved by checking your import paths and ensuring that they align with your project structure. Additionally, utilizing DefinitelyTyped can provide a wealth of type definitions for popular libraries that may not include them by default.”
Sarah Patel (Technical Consultant, DevOps Experts). “In many cases, the error indicates that the module is either not installed or not recognized by TypeScript. I recommend verifying the installation of the module and ensuring that your IDE is configured to recognize TypeScript’s module resolution strategy.”
Frequently Asked Questions (FAQs)
What does the error “Cannot Find Module Or Its Corresponding Type Declarations” mean?
This error indicates that TypeScript cannot locate a module or its type definitions, which may result from a missing package, incorrect module path, or absent type declaration files.
How can I resolve the “Cannot Find Module” error in TypeScript?
To resolve this error, ensure the module is installed via npm or yarn, check the import path for accuracy, and verify that the module’s type declarations are available or installed.
What should I do if a third-party library does not have type declarations?
If a third-party library lacks type declarations, you can create a declaration file (e.g., `*.d.ts`) in your project, or use the `declare module` syntax to define the module’s types manually.
Can I suppress the “Cannot Find Module” error in TypeScript?
You can suppress the error by using the `// @ts-ignore` comment before the import statement, but this is not recommended as it may hide other issues in your code.
How do I install type declarations for a module?
You can install type declarations for a module by using the command `npm install @types/module-name` for DefinitelyTyped packages, ensuring that the module has type definitions available.
What are the implications of ignoring type declaration errors?
Ignoring type declaration errors can lead to runtime issues, reduced code quality, and difficulties in maintaining the codebase due to the lack of type safety and autocompletion features.
The error message “Cannot find module or its corresponding type declarations” is a common issue encountered by developers, particularly when working with TypeScript. This error typically arises when the TypeScript compiler is unable to locate a module that is being imported in the code. The absence of type declarations for that module can lead to confusion, especially for developers who rely on TypeScript’s type-checking features to ensure code quality and correctness.
Several factors can contribute to this error. Firstly, it might occur due to incorrect import paths or missing modules in the project. Developers should ensure that the module is installed and correctly referenced in the import statement. Additionally, if the module does not have type definitions available, the TypeScript compiler will raise this error. In such cases, developers can either create their own type declarations or use third-party libraries that provide the necessary typings.
To mitigate this issue, it is advisable for developers to maintain an organized project structure and to utilize tools such as TypeScript’s `@types` packages, which offer type definitions for many popular libraries. Furthermore, leveraging TypeScript’s configuration options, such as `skipLibCheck` or `noImplicitAny`, can help manage type-checking behavior and reduce the likelihood of encountering this error during
Author Profile
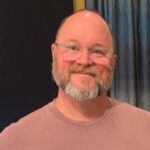
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?