How Can You Speed Up Jest Tests Using All Async Techniques?
In the fast-paced world of software development, the need for efficient testing has never been more critical. As teams strive to deliver high-quality applications at an accelerated pace, the performance of testing frameworks can make or break a project. Enter Jest, a powerful testing library that has gained immense popularity for its simplicity and speed. However, as test suites grow in size and complexity, developers often find themselves grappling with slow test execution times. This is where mastering the art of asynchronous testing can be a game-changer.
In this article, we will explore how to leverage Jest’s asynchronous capabilities to significantly enhance the speed of your tests. By understanding the underlying principles of asynchronous operations and how they interact with Jest’s execution model, you can optimize your test runs and streamline your development process. We will delve into practical strategies that not only reduce waiting times but also improve the overall efficiency of your testing workflow, allowing you to focus more on writing code and less on waiting for tests to complete.
Whether you are a seasoned developer or just starting out, optimizing your Jest tests for asynchronous operations can lead to faster feedback loops and a smoother development experience. Join us as we uncover the best practices and techniques that will transform your testing approach, enabling you to harness the full potential of Jest while keeping your test
Understanding Asynchronous Tests in Jest
Jest allows for writing tests that can handle asynchronous code, which is essential when dealing with promises, callbacks, or any function that may not complete immediately. This is particularly useful in scenarios involving API calls or database interactions. Understanding how to effectively manage these asynchronous tests can significantly enhance the performance and efficiency of your testing suite.
When writing asynchronous tests, Jest provides several methods that can be used, including the use of `async/await`, returning promises, and callbacks. Each of these methods has its own advantages and can be selected based on the specific needs of your test case.
Optimizing Async Tests
To speed up asynchronous tests in Jest, consider the following strategies:
- Use `async/await`: This modern syntax allows for writing cleaner and more readable asynchronous code. It simplifies the chaining of promises and makes error handling straightforward.
- Mocking External Calls: Use Jest’s mocking capabilities to avoid hitting real endpoints during testing. This reduces the time taken by tests and allows for more controlled environments.
- Parallel Testing: Jest runs tests in parallel by default. Ensure that you take full advantage of this feature by structuring your tests to avoid shared state, which can lead to slowdowns and flakiness.
- Limit Test Scope: Focus on only the necessary parts of your application. Use `describe.only` and `it.only` to run specific tests when debugging or iterating quickly.
Example of Asynchronous Tests
Here’s an example illustrating the use of `async/await` in a Jest test:
“`javascript
test(‘fetches data from API’, async () => {
const data = await fetchData();
expect(data).toEqual(expectedData);
});
“`
In this example, `fetchData` is an asynchronous function that returns a promise. The test waits for the promise to resolve before making assertions, ensuring that the test does not proceed until the data is available.
Table of Jest Async Methods
Method | Description | Example Usage |
---|---|---|
async/await | Allows the use of asynchronous code in a synchronous manner. | await fetchData() |
Return Promise | Directly return a promise from the test function. | return fetchData().then(data => expect(data).toEqual(expectedData)); |
done Callback | Use a callback to signal the completion of asynchronous tests. | test(done => { fetchData().then(data => { expect(data).toEqual(expectedData); done(); }); }); |
By implementing these strategies and understanding the tools Jest provides for asynchronous testing, developers can significantly reduce the execution time of their test suites while maintaining robust test coverage.
Understanding Async in Jest
Jest is designed to handle asynchronous code seamlessly, allowing developers to test functions that return promises or use async/await syntax. This capability is essential for ensuring that tests do not complete until asynchronous operations are finished, maintaining the integrity of the test results.
- Promises: Jest automatically waits for promises to resolve. If a test function returns a promise, Jest knows to wait for it to resolve before completing the test.
- Async/Await: When using async functions, Jest will pause until the async function resolves, simplifying the syntax and improving readability.
Optimizing Async Tests
Speeding up tests that involve asynchronous operations can significantly enhance the development workflow. Here are several strategies to improve the performance of async tests in Jest:
- Use `jest.setTimeout`: Adjust the default timeout for tests to a lower value if the operations are expected to finish quickly. This can be done globally or per test.
“`javascript
jest.setTimeout(500); // Set timeout to 500ms
“`
- Parallel Testing: Leverage Jest’s ability to run tests in parallel. This can be especially beneficial for tests that involve independent async operations.
- Mocking External APIs: Instead of making actual API calls, use Jest’s mocking capabilities. This reduces latency and avoids dependency on external services.
“`javascript
jest.mock(‘axios’); // Mocking axios for API calls
“`
- Batching Operations: If multiple async operations can be batched together, this reduces the number of awaits and overall execution time.
Using `Promise.all` for Concurrent Operations
When testing functions that execute multiple asynchronous operations, consider using `Promise.all` to run them concurrently. This approach minimizes waiting time, significantly improving test speed.
“`javascript
test(‘fetch data from multiple endpoints’, async () => {
const [data1, data2] = await Promise.all([
fetchDataFromAPI1(),
fetchDataFromAPI2(),
]);
expect(data1).toBeDefined();
expect(data2).toBeDefined();
});
“`
Best Practices for Async Tests
To ensure efficient and maintainable async tests, adhere to the following best practices:
- Keep Tests Isolated: Ensure that each test does not rely on the results of another. Use `beforeEach` to set up necessary states.
- Limit the Use of `setTimeout`: Avoid using `setTimeout` to control the flow of tests. Instead, use proper async handling techniques.
- Clean Up After Tests: Use `afterEach` or `afterAll` to clean up any resources, which helps avoid potential memory leaks and ensures tests run independently.
Best Practice | Description |
---|---|
Isolated Tests | Tests should not depend on one another. |
Avoid setTimeout | Rely on async/await for flow control instead. |
Resource Cleanup | Always clean up resources to maintain independence. |
Measuring Test Performance
To identify bottlenecks in your async tests, utilize Jest’s built-in performance tracking features. You can run tests with the `–verbose` or `–detectOpenHandles` flags to gather insights into performance.
- Verbose Mode: Displays detailed information about each test’s execution time.
- Open Handles Detection: Helps identify open handles that prevent Jest from exiting cleanly, which can indicate issues with async operations.
By applying these strategies and best practices, developers can effectively speed up their async tests in Jest, leading to a more efficient testing process and a smoother development experience.
Strategies for Accelerating Jest Tests with Asynchronous Code
Dr. Emily Carter (Software Performance Analyst, Tech Innovations Inc.). Asynchronous tests can often lead to bottlenecks if not managed properly. Utilizing Jest’s built-in features such as `jest.setTimeout()` can help optimize the execution time of your tests. Additionally, leveraging `Promise.all()` for concurrent operations can significantly reduce the overall test duration.
Mark Thompson (Senior Software Engineer, Agile Solutions). One effective approach to speed up Jest tests that involve asynchronous operations is to mock external API calls. By using Jest’s mocking capabilities, you can simulate responses and eliminate the latency associated with real network requests, thus allowing your tests to run much faster.
Linda Zhang (Test Automation Specialist, Quality Assurance Experts). It is crucial to minimize the number of async calls in your tests. Refactoring your code to reduce dependencies on asynchronous operations can lead to faster test execution. Additionally, consider using `async/await` syntax effectively to improve readability and maintainability while ensuring that tests complete in a timely manner.
Frequently Asked Questions (FAQs)
What are the common reasons for slow Jest tests?
Slow Jest tests often result from excessive asynchronous operations, unoptimized test setups, or heavy reliance on external resources like databases or APIs. Inefficient code and large test suites can also contribute to longer execution times.
How can I identify slow tests in my Jest suite?
You can identify slow tests by using the `–detectOpenHandles` flag when running Jest. Additionally, the `–verbose` flag provides detailed information about each test’s execution time, allowing you to pinpoint which tests are taking longer than expected.
What strategies can I use to speed up Jest tests with async operations?
To speed up Jest tests with async operations, consider using `Promise.all` to run multiple asynchronous tasks concurrently. Additionally, mock external dependencies to eliminate network delays and use `jest.runAllTimers()` to control timers in tests.
Is it beneficial to run tests in parallel with Jest?
Yes, running tests in parallel can significantly reduce overall test execution time. Jest automatically runs tests in parallel by default, but ensure that your tests are isolated and do not share state to avoid flaky results.
How can I optimize my Jest configuration for faster test execution?
Optimize your Jest configuration by enabling the `maxWorkers` option to control the number of worker threads, using `testPathIgnorePatterns` to exclude unnecessary files, and leveraging `setupFilesAfterEnv` to streamline your test environment setup.
What role do mocks play in speeding up Jest tests?
Mocks play a crucial role in speeding up Jest tests by simulating external dependencies, which reduces the time spent on I/O operations. By using mocks, you can focus on testing your code’s logic without the overhead of real API calls or database queries.
In summary, speeding up tests in Jest, particularly when dealing with asynchronous operations, is crucial for maintaining an efficient development workflow. By leveraging Jest’s built-in capabilities and adopting best practices, developers can significantly reduce test execution time. Key strategies include optimizing test structure, utilizing mocks and spies effectively, and ensuring that asynchronous code is handled properly. This approach not only enhances performance but also improves the overall reliability of the test suite.
One of the most valuable insights is the importance of minimizing the use of unnecessary asynchronous operations within tests. By ensuring that tests only include essential async calls, developers can prevent delays caused by excessive waiting times. Additionally, using Jest’s `jest.runAllTimers()` and `jest.advanceTimersByTime()` functions can help control the flow of time in tests, allowing for faster execution of timed operations.
Another critical takeaway is the effective use of parallel test execution. Jest supports running tests concurrently, which can lead to significant time savings, especially in large projects. By configuring Jest to run tests in parallel and optimizing the test environment, developers can take full advantage of available system resources, further enhancing test performance.
Ultimately, by focusing on these strategies and insights, developers can streamline their testing processes in Jest, leading
Author Profile
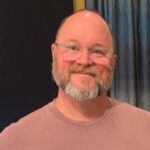
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?