Is Golang Memory Safe? Exploring the Safety Features and Pitfalls of Go Programming
In the ever-evolving landscape of programming languages, Go, commonly known as Golang, has emerged as a powerful contender, particularly in the realms of cloud computing, microservices, and concurrent programming. With its clean syntax and robust performance, Go has garnered a loyal following among developers seeking efficiency and simplicity. However, as software systems grow increasingly complex, the question of memory safety becomes paramount. Is Golang truly memory safe? This inquiry delves into the heart of Go’s design philosophy, examining how its features contribute to or detract from memory safety, and what developers need to consider when choosing Go for their projects.
Memory safety is a critical aspect of programming that prevents common vulnerabilities such as buffer overflows, use-after-free errors, and memory leaks. In Go, the language’s design incorporates garbage collection and strict typing, which are intended to mitigate many of these issues. However, the nuances of Go’s memory management and its approach to pointers and concurrency introduce unique challenges. Understanding how Go handles memory allocation and deallocation is essential for developers aiming to write secure and efficient applications.
As we explore the intricacies of Golang’s memory safety, we will uncover the mechanisms that help protect against memory-related errors, while also addressing the limitations that developers must navigate. By
Understanding Memory Safety in Go
Memory safety refers to the prevention of bugs and vulnerabilities that arise from improper memory access. In programming languages, this often involves ensuring that a program does not read or write memory that it shouldn’t, which can lead to behavior, crashes, or security vulnerabilities. Go, also known as Golang, incorporates several features aimed at enhancing memory safety.
One of the primary mechanisms that Go employs for memory safety is its garbage collection system. This automatic memory management helps to prevent memory leaks and dangling pointers, which are common issues in languages that require manual memory management, such as C or C++. By automatically reclaiming memory that is no longer in use, Go reduces the programmer’s burden and minimizes the risk of memory-related errors.
Key Features Contributing to Memory Safety
Go includes several important features that contribute to its overall memory safety:
- Garbage Collection: Automatically manages memory allocation and deallocation, reducing the risk of memory leaks.
- Strong Typing: Go enforces strict type checks at compile time, which helps prevent unintended operations on incompatible data types.
- Concurrency Model: Go’s goroutines and channels provide safe communication between concurrent processes, minimizing the risk of race conditions.
- Immutable Data Structures: Although Go does not enforce immutability, it encourages the use of immutable data structures, which can help maintain state integrity.
Feature | Description | Benefit |
---|---|---|
Garbage Collection | Automatic memory management | Reduces memory leaks and dangling pointers |
Strong Typing | Compile-time type checks | Prevents operations on incompatible types |
Concurrency Model | Goroutines and channels | Minimizes race conditions and unsafe access |
Immutable Data Structures | Encourages use of immutability | Maintains state integrity |
Limitations and Considerations
Despite these safety features, Go does have some limitations that developers should be aware of. For instance, while Go’s garbage collector greatly simplifies memory management, it may introduce latency during program execution. Garbage collection pauses can affect performance-sensitive applications, particularly in real-time systems.
Additionally, while Go provides strong typing, it does allow for type assertions and reflection, which can lead to unsafe behaviors if not used carefully. Developers need to ensure that they validate types appropriately to avoid runtime panics.
Moreover, Go does not provide built-in protections against buffer overflows or out-of-bounds array access. While the language’s design minimizes these risks, developers must still adhere to best practices when handling slices and arrays to ensure safe access.
In summary, while Go is designed with memory safety in mind, it is essential for developers to understand both its strengths and limitations. By leveraging Go’s features effectively and adhering to best practices, programmers can minimize memory-related issues and enhance the safety of their applications.
Understanding Memory Safety in Golang
Memory safety refers to the prevention of bugs that can lead to memory corruption, such as buffer overflows, dangling pointers, and memory leaks. Go (Golang) has been designed with specific features that enhance its memory safety compared to languages like C or C++.
Key Features of Go That Enhance Memory Safety
Go incorporates several features aimed at ensuring memory safety:
- Garbage Collection: Go utilizes an automatic garbage collection mechanism, which manages memory allocation and deallocation. This reduces the likelihood of memory leaks and dangling pointers.
- Strongly Typed Language: Variables in Go are strongly typed, which helps prevent type-related errors and enforces correct usage of memory allocations.
- Slices and Maps: Go’s slice and map data structures provide bounds checking, which helps avoid buffer overflows by ensuring that accesses are within allocated memory limits.
- Concurrency Model: Go’s goroutines and channels enable safe concurrent programming by providing a model that minimizes shared state, thus reducing potential race conditions.
- No Pointer Arithmetic: Unlike C/C++, Go does not allow pointer arithmetic, which can lead to out-of-bounds memory access. This design choice contributes to safer memory management.
Common Memory Safety Issues in Golang
While Go improves memory safety, certain issues can still arise:
- Nil Pointer Dereference: Attempting to dereference a nil pointer can lead to runtime panics.
- Race Conditions: Concurrent access to shared data without proper synchronization can lead to race conditions, although Go provides tools like the `sync` package to mitigate these issues.
- Memory Leaks: While garbage collection reduces the risk, developers must still be cautious about maintaining references to unused objects, which can prevent them from being collected.
Best Practices for Ensuring Memory Safety in Go
To further enhance memory safety in Go applications, consider the following best practices:
- Use the `go vet` Tool: This static analysis tool helps identify potential issues in code, including memory-related problems.
- Implement Proper Error Handling: Always check for errors and handle them appropriately to prevent unexpected behavior.
- Leverage Built-in Data Structures: Use Go’s built-in slices and maps instead of raw arrays and custom data structures, as they provide safety features.
- Conduct Code Reviews: Regular code reviews can help spot potential memory safety issues that automated tools might miss.
- Utilize the Race Detector: Go provides a race detector that can identify race conditions during testing, helping developers ensure thread safety.
Conclusion on Go’s Memory Safety
Golang’s design incorporates various features and best practices that significantly improve memory safety compared to many traditional programming languages. By leveraging these tools and adhering to best practices, developers can create robust applications with reduced memory-related issues.
Evaluating Memory Safety in Golang
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Golang incorporates several features that enhance memory safety, such as garbage collection and strict typing. However, developers must still be vigilant, as unsafe code can lead to vulnerabilities if not managed properly.”
Michael Chen (Lead Systems Architect, Cloud Solutions Group). “While Golang is designed with memory safety in mind, it is essential to understand that no language can guarantee complete safety. The responsibility lies with developers to adhere to best practices and utilize the language’s features effectively.”
Sarah Patel (Cybersecurity Analyst, SecureTech Labs). “Golang’s memory model provides a solid foundation for building secure applications, but developers must remain aware of potential pitfalls, such as race conditions and improper use of pointers, which can compromise memory safety.”
Frequently Asked Questions (FAQs)
Is Golang memory safe?
Golang provides a level of memory safety through its garbage collection and strong typing system. However, it does not eliminate all memory-related issues, such as data races in concurrent programming.
What features of Golang contribute to memory safety?
Golang includes features such as automatic garbage collection, bounds checking on arrays and slices, and a strong type system that helps prevent common memory errors like buffer overflows and null pointer dereferences.
Can developers introduce memory safety issues in Golang?
Yes, developers can introduce memory safety issues if they use unsafe packages or manipulate pointers incorrectly. Careful coding practices are essential to maintain memory safety.
How does Golang handle memory allocation?
Golang uses a garbage collector to manage memory allocation and deallocation automatically. This reduces the burden on developers to manually manage memory, minimizing the risk of memory leaks.
Are there any tools available for checking memory safety in Golang?
Yes, tools such as the `go vet` command and static analysis tools can help identify potential memory safety issues in Golang code. Additionally, the race detector can help find data races in concurrent programs.
What are the common memory-related issues developers face in Golang?
Common memory-related issues include data races in concurrent programming, improper use of the `unsafe` package, and memory leaks due to references held longer than necessary. Developers should be vigilant to avoid these pitfalls.
In summary, Golang, or Go, is designed with several features that contribute to its memory safety. The language employs garbage collection, which automatically manages memory allocation and deallocation, thereby reducing the risk of memory leaks and dangling pointers. Additionally, Go’s type system and strong emphasis on concurrency help prevent common programming errors that can lead to memory safety issues, such as race conditions and buffer overflows.
Moreover, Go’s approach to handling pointers is more restrictive compared to languages like C or C++. While pointers are still a part of the language, Go encourages the use of value semantics and provides built-in mechanisms to avoid unsafe operations. This design philosophy enhances the overall safety of memory management in Go applications.
Key takeaways from the discussion on Go’s memory safety include the importance of its garbage collection mechanism, the advantages of a strong type system, and the language’s focus on concurrency safety. Developers can leverage these features to build robust applications that minimize the risk of memory-related vulnerabilities. Overall, while no programming language can guarantee complete memory safety, Go provides a framework that significantly mitigates many common risks associated with memory management.
Author Profile
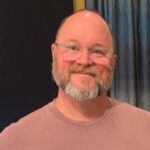
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?