How Can You Effectively Multiply Numbers in Python?
In the world of programming, mastering the fundamentals is essential for unlocking the full potential of any language. Python, known for its simplicity and versatility, is no exception. Whether you’re a beginner taking your first steps into coding or an experienced developer looking to refine your skills, understanding how to perform multiplication in Python is a foundational concept that can elevate your programming prowess. This article will guide you through the various methods and nuances of multiplication in Python, ensuring you grasp not just the “how,” but also the “why” behind these techniques.
Multiplication in Python is straightforward, yet it offers a wealth of possibilities, from basic arithmetic operations to more complex applications in data analysis and scientific computing. At its core, Python provides several built-in ways to multiply numbers, whether they are integers, floats, or even arrays. As you delve deeper into the language, you’ll discover how these multiplication techniques can be applied in different contexts, enhancing your ability to solve problems efficiently.
Moreover, understanding multiplication in Python sets the stage for exploring more advanced topics, such as vectorized operations with libraries like NumPy, which can significantly speed up computations. As we embark on this journey through the mechanics of multiplication, prepare to uncover the simplicity and power of Python as a tool for both novice and seasoned
Using the Multiplication Operator
In Python, the most straightforward way to perform multiplication is by using the asterisk (`*`) operator. This operator can be applied to integers, floats, and even complex numbers.
For example:
“`python
result_int = 5 * 3 Result: 15
result_float = 2.5 * 4.0 Result: 10.0
result_complex = 1 + 2j * 3 + 4j Result: (-5+10j)
“`
The multiplication operator is versatile and can be used in various contexts, such as multiplying variables, literals, or even results from functions.
Multiplying Lists and Tuples
When dealing with sequences like lists and tuples, the multiplication operator can be used to repeat these sequences. This operation does not perform element-wise multiplication; instead, it concatenates the sequence multiple times.
For example:
“`python
list_example = [1, 2, 3] * 3 Result: [1, 2, 3, 1, 2, 3, 1, 2, 3]
tuple_example = (1, 2) * 2 Result: (1, 2, 1, 2)
“`
This feature can be particularly useful for initializing data structures or creating repeated patterns in data.
Using NumPy for Element-wise Multiplication
For more complex multiplication operations, especially with arrays, the NumPy library is an excellent choice. NumPy allows for element-wise multiplication of arrays, making it easy to handle mathematical operations on large datasets.
To perform element-wise multiplication with NumPy, you first need to install the library (if not already installed):
“`bash
pip install numpy
“`
Once installed, you can use it as follows:
“`python
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result = array1 * array2 Result: array([ 4, 10, 18])
“`
Multiplication Table Example
To illustrate multiplication in Python, consider creating a multiplication table. This can be achieved with nested loops. Below is a simple implementation that generates a multiplication table from 1 to 10.
“`python
def multiplication_table(n):
print(“Multiplication Table”)
print(“———————“)
print(“
{i * j} |
“)
multiplication_table(10)
“`
This function creates an HTML-style multiplication table that can be rendered in a web browser, presenting the results in a structured format.
Summary of Multiplication Techniques
The following table summarizes the various multiplication techniques in Python:
Method | Description | Example |
---|---|---|
Operator (`*`) | Basic multiplication for numbers | `5 * 3` |
List/Tuple Multiplication | Repeats lists/tuples | `[1, 2] * 3` |
NumPy | Element-wise multiplication of arrays | `np.array([1, 2]) * np.array([3, 4])` |
Each of these methods serves different use cases, allowing for flexibility depending on the data types and structures used in your Python programming.
Multiplying Numbers in Python
In Python, multiplication can be performed using the asterisk `*` operator. This operator is straightforward and can be used with various numerical types, including integers, floats, and complex numbers.
Basic Multiplication
To multiply two numbers, simply use the `*` operator between them. Here are some examples:
“`python
Multiplying integers
result_int = 3 * 4 result_int is 12
Multiplying floats
result_float = 2.5 * 4.0 result_float is 10.0
Multiplying a float and an integer
result_mixed = 5 * 2.0 result_mixed is 10.0
“`
Multiplying Lists and Other Sequences
Python also allows multiplication of sequences, such as lists and strings, which will repeat the sequence a specified number of times.
“`python
Multiplying a list
list_example = [1, 2, 3] * 3 list_example is [1, 2, 3, 1, 2, 3, 1, 2, 3]
Multiplying a string
string_example = “Hello ” * 2 string_example is “Hello Hello ”
“`
Using the `numpy` Library for Arrays
For more complex mathematical operations involving arrays, the `numpy` library is highly effective. It provides an efficient way to perform element-wise multiplication on arrays.
“`python
import numpy as np
Creating two numpy arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
Element-wise multiplication
result_array = array1 * array2 result_array is array([4, 10, 18])
“`
Multiplying Matrices
To multiply matrices in Python, you can use the `@` operator or the `numpy.dot()` function, which performs matrix multiplication.
“`python
Using the @ operator
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
Matrix multiplication
result_matrix = matrix1 @ matrix2 result_matrix is array([[19, 22], [43, 50]])
Using numpy.dot()
result_matrix_dot = np.dot(matrix1, matrix2) same result as above
“`
Multiplying with Other Data Types
Python also supports multiplication with complex numbers, where the multiplication follows the rules of complex arithmetic.
“`python
Multiplying complex numbers
complex1 = 2 + 3j
complex2 = 1 + 2j
result_complex = complex1 * complex2 result_complex is (-4+7j)
“`
Summary of Multiplication Syntax
Operation Type | Syntax | Example | Result |
---|---|---|---|
Basic multiplication | `a * b` | `3 * 4` | `12` |
List repetition | `list * n` | `[1, 2] * 3` | `[1, 2, 1, 2, 1, 2]` |
String repetition | `string * n` | `”Hi” * 3` | `”HiHiHi”` |
Numpy element-wise | `array1 * array2` | `np.array([1,2]) * np.array([3,4])` | `array([3, 8])` |
Matrix multiplication | `matrix1 @ matrix2` | `np.array([[1, 2]]) @ np.array([[3], [4]])` | `array([[11]])` |
This concise guide outlines the different ways to perform multiplication in Python, catering to various data types and structures.
Expert Insights on Multiplication in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Multiplication in Python can be efficiently performed using the `*` operator for scalar values, but it is essential to understand how to leverage libraries like NumPy for handling arrays and matrices, which significantly optimizes performance in data-heavy applications.”
Mark Thompson (Data Scientist, Analytics Hub). “When multiplying large datasets in Python, utilizing vectorized operations through libraries such as NumPy or Pandas not only simplifies the code but also enhances computational speed, which is crucial for real-time data analysis.”
Lisa Patel (Python Developer, CodeCrafters). “Understanding the nuances of multiplication in Python, including the differences between element-wise multiplication and matrix multiplication, is vital for developers working in scientific computing or machine learning, as it can affect the outcome of algorithms significantly.”
Frequently Asked Questions (FAQs)
How do you multiply two numbers in Python?
You can multiply two numbers in Python using the asterisk (*) operator. For example, `result = a * b`, where `a` and `b` are the numbers you wish to multiply.
Can you multiply a list of numbers in Python?
Yes, you can multiply all elements in a list using a loop or a function like `reduce` from the `functools` module. For example, `from functools import reduce; result = reduce(lambda x, y: x * y, my_list)`.
What happens if you multiply a number by a string in Python?
Multiplying a number by a string in Python will result in the string being repeated that number of times. For example, `result = 3 * ‘abc’` will yield `’abcabcabc’`.
Is it possible to multiply matrices in Python?
Yes, you can multiply matrices in Python using the `numpy` library. The function `numpy.dot()` or the `@` operator can be used for matrix multiplication, e.g., `result = np.dot(matrix1, matrix2)`.
How can you handle multiplication of different data types in Python?
Python automatically handles type conversion during multiplication, but it is essential to ensure that the types are compatible. For example, multiplying an integer and a float will yield a float.
What is the output of multiplying a number by zero in Python?
The output of multiplying any number by zero in Python will always be zero. For example, `result = 5 * 0` will yield `0`.
In Python, multiplication can be performed using the asterisk (*) operator, which is the standard method for multiplying numbers. This operator can be used with various data types, including integers, floats, and even complex numbers. Additionally, Python supports multiplication of sequences, such as lists and strings, allowing for the repetition of elements or characters, respectively. Understanding these basic operations is essential for effective programming in Python.
Another important aspect of multiplication in Python is the use of libraries like NumPy, which provides advanced mathematical functions and supports operations on arrays. This is particularly useful for handling large datasets and performing element-wise multiplication efficiently. Utilizing these libraries can significantly enhance performance and streamline complex calculations, making them a valuable tool for data analysis and scientific computing.
Moreover, Python’s ability to handle multiplication in a straightforward manner, combined with its extensive libraries, makes it an ideal choice for both beginners and experienced programmers. By mastering multiplication and its applications, users can build a solid foundation for more advanced programming concepts and mathematical operations. Overall, Python’s versatility in handling multiplication reflects its strength as a programming language in various domains.
Author Profile
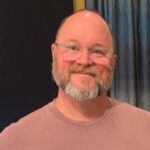
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?