How Can You Implement A Star Algorithm in Python for Optimal Pathfinding?
In the realm of computer science and artificial intelligence, finding the most efficient path from one point to another is a challenge that has fascinated researchers and developers alike. Enter the A* (A-star) algorithm, a powerful and versatile pathfinding and graph traversal technique that has become a cornerstone in various applications, from video games to robotics. With its ability to intelligently navigate complex networks, the A* algorithm not only optimizes routes but also enhances the overall performance of systems that rely on spatial reasoning. This article delves into the intricacies of implementing the A* algorithm in Python, showcasing its elegance and efficiency.
At its core, the A* algorithm combines the strengths of Dijkstra’s algorithm and heuristic-based approaches, allowing it to find the shortest path while minimizing computational overhead. By evaluating the cost of each potential path and leveraging heuristics to guide its search, A* effectively balances exploration and exploitation. This makes it an ideal choice for scenarios where speed and accuracy are paramount, such as in real-time strategy games or navigation systems.
In this exploration, we will break down the fundamental concepts behind the A* algorithm, demonstrating how it can be implemented in Python. As we navigate through the algorithm’s mechanics and its practical applications, readers will gain a deeper understanding of not
A Star Algorithm Overview
The A* (A-star) algorithm is a popular pathfinding and graph traversal algorithm used in computer science and artificial intelligence. It is particularly well-suited for finding the shortest path between nodes in a weighted graph. The A* algorithm combines the benefits of Dijkstra’s algorithm and the Greedy Best-First Search, making it both efficient and effective in many scenarios.
At its core, the A* algorithm maintains a priority queue of nodes to explore. Each node has an associated cost, which is a sum of two main components:
- g(n): The cost from the start node to the current node n.
- h(n): A heuristic estimate of the cost from the current node n to the goal.
The A* algorithm chooses the next node to explore based on the lowest total cost function:
\[ f(n) = g(n) + h(n) \]
To implement the A* algorithm in Python, one must define the graph, the heuristic function, and the open and closed lists to track explored and unexplored nodes.
Python Implementation of A Star Algorithm
Below is a basic implementation of the A* algorithm in Python. This example assumes a grid-based graph, where each cell can be traversed or blocked.
“`python
import heapq
class Node:
def __init__(self, position, g=0, h=0):
self.position = position Node position (x, y)
self.g = g Cost from start to current node
self.h = h Heuristic cost to goal
self.f = g + h Total cost
self.parent = None Parent node for path reconstruction
def __lt__(self, other):
return self.f < other.f
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1]) Manhattan distance
def astar(start, goal, grid):
open_list = []
closed_list = set()
start_node = Node(start, 0, heuristic(start, goal))
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
if current_node.position == goal:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1] Return reversed path
closed_list.add(current_node.position)
for new_position in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
node_position = (current_node.position[0] + new_position[0],
current_node.position[1] + new_position[1])
if (node_position[0] < 0 or node_position[0] >= len(grid) or
node_position[1] < 0 or node_position[1] >= len(grid[0]) or
grid[node_position[0]][node_position[1]] != 0 or
node_position in closed_list):
continue
g = current_node.g + 1
h = heuristic(node_position, goal)
new_node = Node(node_position, g, h)
new_node.parent = current_node
if add_to_open(open_list, new_node):
heapq.heappush(open_list, new_node)
return None Return None if no path is found
def add_to_open(open_list, neighbor):
for node in open_list:
if neighbor.position == node.position and neighbor.g >= node.g:
return
return True
“`
Understanding the Components
The A* algorithm involves several key components:
Component | Description |
---|---|
Node | An individual point in the graph with a position, costs (g, h, f), and a parent. |
Open List | A priority queue of nodes that need to be evaluated. |
Closed List | A set of nodes that have already been evaluated. |
Heuristic Function | A function that estimates the cost to reach the goal from a given node. |
Utilizing the A* algorithm effectively requires careful selection of the heuristic function, as it significantly affects the algorithm’s performance and efficiency.
A* Algorithm Overview
The A* (A-star) algorithm is a popular pathfinding and graph traversal algorithm used in various applications, such as robotics and video games. It efficiently finds the shortest path from a starting node to a target node by utilizing a cost function that combines the actual distance traveled and an estimated distance to the goal.
The key components of the A* algorithm are:
- g(n): The cost from the start node to node n.
- h(n): The heuristic estimated cost from node n to the goal.
- f(n): The total cost function, defined as f(n) = g(n) + h(n).
A* employs a priority queue to explore nodes with the lowest f(n) value first, ensuring an optimal path is found.
Implementing A* Algorithm in Python
To implement the A* algorithm in Python, we can use the following structure:
- Node Class: Represents each point in the search space.
- Priority Queue: Manages the nodes to explore based on their f(n) values.
- Heuristic Function: Estimates the cost to reach the goal from a given node.
Here is a basic implementation:
“`python
import heapq
class Node:
def __init__(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0 Cost from start node
self.h = 0 Heuristic cost to goal
self.f = 0 Total cost
def __eq__(self, other):
return self.position == other.position
def __lt__(self, other):
return self.f < other.f
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1]) Manhattan distance
def a_star(start, goal, grid):
open_list = []
closed_list = set()
start_node = Node(start)
goal_node = Node(goal)
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
closed_list.add(current_node.position)
if current_node == goal_node:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1] Return reversed path
children = []
for new_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]: Adjacent squares
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
if node_position[0] > (len(grid) – 1) or node_position[0] < 0 or node_position[1] > (len(grid[len(grid) – 1]) – 1) or node_position[1] < 0:
continue
if grid[node_position[0]][node_position[1]] != 0:
continue
new_node = Node(node_position, current_node)
children.append(new_node)
for child in children:
if child.position in closed_list:
continue
child.g = current_node.g + 1
child.h = heuristic(child.position, goal_node.position)
child.f = child.g + child.h
if not any(open_child == child and child.g > open_child.g for open_child in open_list):
heapq.heappush(open_list, child)
return None No path found
“`
Using the A* Algorithm
To use the A* algorithm effectively, consider the following:
- Grid Representation: Define your search space as a 2D list where 0 represents walkable cells and 1 represents obstacles.
- Heuristic Choice: Select an appropriate heuristic function based on the problem domain. Common options include:
- Manhattan Distance
- Euclidean Distance
- Diagonal Distance
- Performance Considerations:
- The efficiency of A* heavily relies on the heuristic used. A good heuristic will significantly reduce the search space.
- Ensure that the priority queue is implemented efficiently, as this impacts the algorithm’s performance.
By following the structure and implementation provided, you can adapt the A* algorithm for various applications, tailoring the heuristic and grid to suit specific needs.
Expert Insights on Implementing A Star Algorithm in Python
Dr. Emily Carter (Senior Data Scientist, AI Innovations Lab). “The A Star algorithm is a powerful pathfinding and graph traversal method that can be efficiently implemented in Python using libraries such as NumPy and NetworkX. Its heuristic approach allows for optimized performance in various applications, from robotics to game development.”
Michael Chen (Lead Software Engineer, Tech Solutions Inc.). “When implementing the A Star algorithm in Python, it is crucial to carefully select the heuristic function. A well-designed heuristic not only speeds up the search process but also ensures that the algorithm remains optimal and complete, making it highly effective for real-world scenarios.”
Dr. Sarah Patel (Professor of Computer Science, University of Technology). “In Python, the A Star algorithm can be enhanced by utilizing priority queues, which can be efficiently managed with the heapq module. This optimization significantly reduces the time complexity, making it suitable for larger datasets and complex environments.”
Frequently Asked Questions (FAQs)
What is the A* algorithm?
The A* algorithm is a popular pathfinding and graph traversal algorithm used in computer science and artificial intelligence. It efficiently finds the shortest path from a starting node to a target node by combining features of Dijkstra’s algorithm and greedy best-first search.
How does the A* algorithm work?
The A* algorithm works by maintaining a priority queue of nodes to explore, using a cost function that combines the actual cost to reach a node and an estimated cost to reach the goal. It selects the node with the lowest total cost for exploration, ensuring an optimal path is found.
What are the key components of the A* algorithm?
The key components of the A* algorithm include the open set (nodes to be evaluated), the closed set (nodes already evaluated), the cost function (g(n) + h(n)), where g(n) is the cost from the start node to the current node, and h(n) is the heuristic estimate to the goal.
Can you implement the A* algorithm in Python?
Yes, the A* algorithm can be implemented in Python using data structures like lists or priority queues. Libraries such as `heapq` can be utilized for efficient queue management, and custom heuristics can be defined based on the problem domain.
What are some common heuristics used in the A* algorithm?
Common heuristics include the Manhattan distance, Euclidean distance, and Chebyshev distance. The choice of heuristic can significantly affect the algorithm’s performance and efficiency in finding the shortest path.
What are the limitations of the A* algorithm?
The A* algorithm can be computationally expensive in terms of time and memory, especially for large search spaces. Its performance heavily relies on the quality of the heuristic used; a poor heuristic can lead to suboptimal paths and increased processing time.
The A* algorithm is a powerful pathfinding and graph traversal method widely used in various applications, including artificial intelligence, robotics, and game development. Its efficiency stems from its ability to combine the benefits of Dijkstra’s algorithm and greedy best-first search. By utilizing a heuristic to estimate the cost from the current node to the goal, A* effectively prioritizes nodes that are more likely to lead to the shortest path, making it both optimal and complete under certain conditions.
Implementing the A* algorithm in Python involves defining a suitable heuristic function, typically based on the specific problem’s requirements. Common heuristics include the Manhattan distance for grid-based maps and Euclidean distance for continuous spaces. The algorithm maintains a priority queue to explore the most promising nodes first, ensuring that it can efficiently navigate complex graphs while minimizing computational overhead.
Key takeaways from the discussion on the A* algorithm include the importance of selecting an appropriate heuristic to ensure optimal performance and the algorithm’s versatility across different domains. Additionally, understanding the trade-offs between accuracy and computational efficiency can help developers tailor the A* implementation to their specific needs. Overall, mastering the A* algorithm in Python equips developers with a robust tool for solving a wide range of pathfinding challenges.
Author Profile
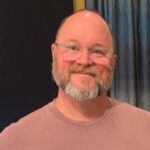
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?