How Can You Pass a Variable from a Userform to a Module in VBA?
In the world of Excel VBA, userforms serve as powerful tools that enhance user interaction and streamline data entry processes. However, one of the common challenges developers face is effectively passing variables from these userforms to modules. This crucial skill can significantly improve the functionality of your applications, allowing for smoother data manipulation and more dynamic programming. Whether you’re building a simple data entry form or a complex application, understanding how to transfer information seamlessly between userforms and modules is essential for any VBA developer looking to elevate their projects.
At its core, passing variables from a userform to a module involves understanding the relationship between the two components within the VBA environment. Userforms are designed to capture user input, while modules contain the logic that processes this data. By mastering the techniques for transferring values, you can ensure that the data collected in your userform can be utilized effectively in your code, enabling you to create more robust applications. This process not only enhances the functionality of your projects but also opens the door to more sophisticated programming techniques.
As we delve deeper into this topic, we will explore various methods and best practices for passing variables, including the use of public variables, properties, and functions. With practical examples and clear explanations, you’ll gain the confidence to implement these strategies in your own VBA projects, transforming
Understanding UserForm and Module Interaction
When working with UserForms in VBA (Visual Basic for Applications), it is essential to understand how to efficiently pass variables from the UserForm to a standard module. This allows for better organization of your code and enhances reusability. Variables can be passed in several ways, including through public variables, properties, or function parameters.
Using Public Variables
One common method to share data between a UserForm and a module is to declare a public variable in a standard module. This variable can then be accessed from both the UserForm and the module.
- Declare a Public Variable: In a standard module, declare a variable as Public.
“`vba
‘ In a standard module
Public userInput As String
“`
- Set the Variable in UserForm: In your UserForm, assign a value to the public variable.
“`vba
‘ In UserForm code
Private Sub btnSubmit_Click()
userInput = txtInput.Text
Call ProcessInput
End Sub
“`
- Access the Variable in Module: You can then access `userInput` in any procedure within the same or another module.
“`vba
‘ In a standard module
Sub ProcessInput()
MsgBox “User Input: ” & userInput
End Sub
“`
Using Properties in UserForms
Another approach is to define properties in the UserForm that can be accessed from the module. This encapsulates the data within the UserForm.
- Define a Property: Add a property to the UserForm.
“`vba
‘ In UserForm code
Public Property Get UserInput() As String
UserInput = txtInput.Text
End Property
“`
- Access the Property in Module: You can call this property from the module after creating an instance of the UserForm.
“`vba
‘ In a standard module
Sub ShowForm()
Dim myForm As UserForm1
Set myForm = New UserForm1
myForm.Show
MsgBox “User Input: ” & myForm.UserInput
Set myForm = Nothing
End Sub
“`
Passing Variables via Function Parameters
Alternatively, you can pass variables directly as parameters when calling functions or subroutines.
- Define a Function: Create a function in the module that accepts a parameter.
“`vba
‘ In a standard module
Sub DisplayInput(userInput As String)
MsgBox “User Input: ” & userInput
End Sub
“`
- Call the Function from UserForm: Pass the UserForm’s input to the function.
“`vba
‘ In UserForm code
Private Sub btnSubmit_Click()
DisplayInput txtInput.Text
End Sub
“`
Comparison of Methods
Each method has its advantages and disadvantages. The table below summarizes these approaches:
Method | Advantages | Disadvantages |
---|---|---|
Public Variables | Easy to implement; accessible globally. | Can lead to code that is harder to maintain; potential for variable name conflicts. |
Properties | Encapsulates data; enhances code readability. | Requires more code; can be less straightforward for beginners. |
Function Parameters | Clear data flow; promotes modular design. | Requires more calls; may be cumbersome with multiple variables. |
Understanding these methods allows developers to choose the most effective way to pass variables from UserForms to modules in their VBA applications.
Understanding UserForms in VBA
UserForms in VBA are custom dialog boxes that allow users to interact with applications. They can capture user input, display messages, and provide a more user-friendly interface. To effectively pass variables from a UserForm to a module, it’s essential to understand the structure of UserForms and how they communicate with other components of your VBA project.
Key Components of UserForms
- Controls: Objects like text boxes, combo boxes, and buttons that allow user interaction.
- Properties: Attributes of controls, such as `Value`, `Visible`, and `Enabled`.
- Events: Actions triggered by user interactions, such as clicking a button or changing a selection.
Passing Variables from UserForm to Module
To pass variables from a UserForm to a standard module in VBA, follow these steps:
Step-by-Step Process
- Declare Variables in the UserForm: Define your variables within the UserForm.
- Create a Public Subroutine in the Module: This subroutine will receive the variables.
- Call the Module’s Subroutine from the UserForm: Use the UserForm’s controls to pass the values.
Example Implementation
UserForm Code
“`vba
Private Sub CommandButton1_Click()
Dim userInput As String
userInput = TextBox1.Value
Call Module1.ReceiveInput(userInput)
End Sub
“`
Module Code
“`vba
Public Sub ReceiveInput(inputString As String)
MsgBox “Received input: ” & inputString
End Sub
“`
Explanation of the Code
- In the UserForm, when `CommandButton1` is clicked, it retrieves the value from `TextBox1`.
- The value is then passed to the `ReceiveInput` subroutine in `Module1`.
- `ReceiveInput` displays the received input using a message box.
Best Practices for Variable Passing
- Use Public Variables: If the variable needs to be accessed across multiple UserForms or modules, declare it as public in a standard module.
- Data Validation: Always validate user input before passing it to ensure data integrity.
- Encapsulation: Limit the exposure of variables by using properties within UserForms to control access.
Example of Public Variable
Standard Module Code
“`vba
Public userInput As String
Public Sub ReceiveInput()
MsgBox “Received input: ” & userInput
End Sub
“`
UserForm Code
“`vba
Private Sub CommandButton1_Click()
userInput = TextBox1.Value
Call ReceiveInput
End Sub
“`
Advantages of Using Public Variables
- Simplifies variable management.
- Reduces the need for multiple parameter passing.
- Enhances code readability and maintainability.
Common Issues and Troubleshooting
While passing variables from UserForms to modules, you may encounter several issues:
Issue | Solution |
---|---|
Variable not recognized | Ensure the variable is declared as Public. |
Incorrect data type | Validate input types before passing. |
Event not triggering | Check the event handler and control names. |
By following these guidelines and examples, you can efficiently manage variable passing between UserForms and modules in VBA, enhancing your application’s functionality and user experience.
Expert Insights on Passing Variables from Userform to Module in VBA
Linda Carter (Senior VBA Developer, Tech Solutions Inc.). “Passing variables from a userform to a module in VBA is crucial for maintaining clean and efficient code. It allows developers to utilize user inputs dynamically, enhancing the interactivity of applications. I recommend using public variables or properties within the userform to facilitate this process.”
James Thompson (Lead Software Engineer, CodeCraft Labs). “One effective method to pass variables from a userform to a module is by employing the ‘Call’ statement. This approach not only simplifies the transfer of data but also ensures that the module can perform operations based on user inputs seamlessly. Properly structuring your code is essential for scalability.”
Maria Gonzalez (VBA Programming Consultant, Excel Experts). “I have found that using a dedicated class module can significantly streamline the process of passing variables. By encapsulating userform data within a class, developers can manage and manipulate data more effectively, leading to cleaner and more maintainable code.”
Frequently Asked Questions (FAQs)
How can I pass a variable from a UserForm to a module in VBA?
To pass a variable from a UserForm to a module in VBA, you can declare the variable as a public variable in the module. Then, assign the value from the UserForm to this variable when the UserForm is submitted or closed.
What is the difference between public and private variables in VBA?
Public variables are accessible from any module or UserForm within the same project, while private variables can only be accessed within the module or UserForm where they are declared. Use public variables to share data across different parts of your application.
Can I pass multiple variables from a UserForm to a module?
Yes, you can pass multiple variables by declaring each variable as public in the module or by using a custom data type or class to encapsulate the variables together and pass that single object.
What is the best practice for passing data from a UserForm to a module?
The best practice is to use public variables for simple data types or to create a dedicated class or structure for more complex data. This approach enhances code readability and maintainability.
How do I retrieve the value of a variable in a module after passing it from a UserForm?
To retrieve the value, simply reference the public variable in the module where it was declared. Ensure that the UserForm has been closed or the value has been assigned before attempting to access it.
Is it possible to pass variables back from a module to a UserForm?
Yes, you can pass variables back to a UserForm by either setting properties of the UserForm or by using public variables in the UserForm that can be accessed from the module.
In summary, passing variables from a UserForm to a module in VBA is a fundamental aspect of enhancing the functionality and interactivity of Excel applications. This process typically involves defining public variables within the module, which can then be accessed and modified by the UserForm. Properly structuring the code ensures that data flows seamlessly between the UserForm and the module, allowing for dynamic user inputs to influence the program’s behavior.
Key insights include the importance of understanding the scope of variables in VBA. Public variables declared in a module are accessible throughout the project, making them ideal for sharing data between different components. Additionally, utilizing properties in the UserForm can encapsulate data and provide a more organized approach to managing user inputs. This encapsulation not only improves code readability but also enhances maintainability.
Moreover, leveraging event-driven programming within UserForms allows for real-time data handling, which can significantly improve user experience. By implementing appropriate event procedures, developers can ensure that user actions trigger the necessary updates to the module, thereby creating a responsive interface. This integration between UserForms and modules is essential for building robust and user-friendly applications in VBA.
Author Profile
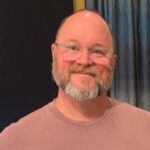
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?