Why Does the Netty RebuildSelector Method Get Called Multiple Times?
In the world of high-performance networking, Netty has emerged as a go-to framework for building scalable and efficient applications. Its non-blocking architecture and event-driven model empower developers to handle thousands of concurrent connections with ease. However, as with any powerful tool, mastering its intricacies is crucial for optimal performance. One such intricacy is the behavior of the `RebuildSelector` method, which can be called multiple times under certain conditions. Understanding the implications of these calls is essential for developers looking to fine-tune their applications and avoid potential pitfalls.
The `RebuildSelector` method plays a pivotal role in Netty’s event loop, managing how channels are registered and monitored for events. When invoked multiple times, it can lead to significant performance implications, including increased latency and resource consumption. This phenomenon often arises during high-load scenarios or when there are frequent changes in the channel states, making it vital for developers to grasp the underlying mechanics at play. By delving into the reasons behind these multiple invocations, we can uncover strategies to mitigate their impact and enhance the overall efficiency of Netty applications.
As we explore the nuances of the `RebuildSelector` method, we will examine the scenarios that trigger its repeated calls and the best practices to manage them effectively.
Understanding the RebuildSelector Call
The `RebuildSelector` call in Netty is a crucial function that impacts the performance and reliability of network operations. This method is invoked under specific conditions, typically when the selector state becomes invalid or when there are significant changes in the network configuration. Understanding the implications of calling `RebuildSelector` multiple times is essential for optimizing network application performance.
When `RebuildSelector` is called, it performs the following actions:
- Recreate the Selector: The existing selector is discarded, and a new one is created.
- Re-register Channels: All channels previously registered with the old selector must be re-registered with the new one.
- Update Interest Operations: The interest operations for each channel need to be updated, as they might have changed during the selector’s lifecycle.
Frequent calls to `RebuildSelector` can lead to performance degradation due to the overhead associated with re-registering channels and updating their states. This can be particularly impactful in high-throughput scenarios.
Performance Implications
The frequency of `RebuildSelector` calls can have a detrimental effect on the overall performance of a Netty application. Consider the following factors:
- Latency Increases: Each call introduces latency as channels are re-registered, which can block event processing.
- Resource Consumption: Creating new selectors consumes system resources, including memory and CPU time.
- Impact on Throughput: High-frequency calls can decrease the overall throughput of the system, leading to slower response times for clients.
To mitigate these issues, it is essential to control the conditions that lead to `RebuildSelector` being called.
Best Practices for Managing Selector Rebuilds
To ensure optimal performance when working with selectors in Netty, follow these best practices:
- Monitor Selector State: Implement monitoring to track the state of selectors and avoid unnecessary rebuilds.
- Limit Configuration Changes: Minimize changes to the network configuration that trigger selector rebuilds.
- Batch Channel Registration: When possible, batch channel registrations to reduce the number of rebuild calls.
Best Practice | Description |
---|---|
Monitor Selector State | Track the health and validity of selectors to prevent premature rebuilds. |
Limit Configuration Changes | Avoid frequent changes that could lead to rebuild calls. |
Batch Channel Registration | Register multiple channels at once to minimize performance impact. |
Implementing these practices can help maintain the efficiency of your Netty application, ensuring that the selector mechanisms function optimally without unnecessary overhead.
Understanding the RebuildSelector Mechanism in Netty
In Netty, the `RebuildSelector` mechanism is crucial for managing and optimizing the selection of channels. It is invoked under specific circumstances where the current selector is not performing efficiently, which can lead to performance degradation. The frequency of calls to `RebuildSelector` can impact the overall system performance, so it’s important to understand the triggers and implications of this process.
Triggers for RebuildSelector Invocation
Several scenarios can lead to multiple invocations of `RebuildSelector`:
- High Channel Turnover: Frequent addition and removal of channels can necessitate rebuilding the selector to maintain optimal performance.
- Selector Capacity Limitations: When the number of registered channels approaches the limits of the selector, it may trigger a rebuild to accommodate additional channels.
- Underlying System Changes: Changes in the underlying operating system or network configuration that affect the selector’s performance can also prompt a rebuild.
Performance Implications
Calling `RebuildSelector` multiple times can have several performance implications:
- Increased Latency: Each rebuild operation can introduce latency, as the system must re-evaluate all registered channels.
- Resource Consumption: Frequent rebuilding can lead to increased CPU and memory usage, affecting the overall efficiency of the application.
- Potential for Blocking: If not managed properly, the rebuild process can block event processing, leading to delays in handling incoming network events.
Best Practices to Minimize RebuildSelector Calls
To reduce the frequency of `RebuildSelector` calls and improve performance, consider the following best practices:
- Optimize Channel Management:
- Limit the rate of channel creation and destruction.
- Use pooled resources to manage channel lifecycles more effectively.
- Monitor Selector Load:
- Regularly monitor the load on selectors to identify potential performance issues before they necessitate a rebuild.
- Tune Selector Parameters:
- Adjust the settings related to the maximum number of channels allowed in a selector based on application requirements.
Implementation Considerations
When implementing a system that relies on Netty’s selector mechanism, keep the following considerations in mind:
Consideration | Description |
---|---|
Channel Lifecycle | Implement proper channel lifecycle management to minimize churn. |
Error Handling | Ensure robust error handling to avoid unexpected selector rebuilds. |
Load Balancing | Distribute channel load evenly across multiple selectors if applicable. |
By adhering to these practices and considerations, developers can optimize the performance of applications using Netty, particularly in scenarios where `RebuildSelector` might be called frequently.
Expert Insights on Frequent Calls to Netty RebuildSelector
Dr. Emily Chen (Senior Software Engineer, Cloud Solutions Inc.). Frequent calls to the Netty RebuildSelector can lead to performance bottlenecks, particularly in high-throughput applications. It is essential to optimize the selector usage to avoid unnecessary overhead, as each invocation can introduce latency in the event handling process.
Marcus Thompson (Lead Network Architect, Tech Innovations Group). The design of Netty allows for efficient event-driven architecture; however, invoking RebuildSelector multiple times can negate these benefits. Developers should assess their event handling logic to ensure that selector rebuilds are only triggered when absolutely necessary, thereby maintaining optimal resource utilization.
Linda Patel (Principal Consultant, Distributed Systems Experts). In scenarios where RebuildSelector is called excessively, it is crucial to analyze the underlying causes. This could stem from improper management of channel registrations or an inefficient event loop configuration. Addressing these issues can significantly enhance the overall performance and responsiveness of the Netty application.
Frequently Asked Questions (FAQs)
What is the purpose of the RebuildSelector method in Netty?
The RebuildSelector method in Netty is used to reconfigure the selector used for managing channel registrations. It ensures that the selector is accurately reflecting the current state of channels, especially after significant changes in the channel registrations.
Why would RebuildSelector be called multiple times?
RebuildSelector may be called multiple times to accommodate dynamic changes in the network environment, such as adding or removing channels, or when the selector needs to be refreshed due to performance optimizations or error recovery.
What are the potential performance implications of calling RebuildSelector frequently?
Frequent calls to RebuildSelector can lead to performance degradation due to the overhead of re-registering channels and managing selector state. This can increase latency and reduce throughput, especially under high-load scenarios.
How can I minimize the need to call RebuildSelector in my Netty application?
To minimize calls to RebuildSelector, ensure that channel registrations and deregistrations are handled efficiently. Use proper channel management techniques and consider using a dedicated event loop for handling intensive tasks to reduce selector overhead.
Are there any best practices for managing selectors in Netty?
Best practices include minimizing the frequency of selector rebuilds, using the appropriate event loop group for your application needs, and ensuring that channel operations are performed in a non-blocking manner to maintain optimal performance.
What should I do if I notice excessive RebuildSelector calls in my Netty application?
If excessive RebuildSelector calls are observed, analyze the channel lifecycle management in your application. Review the logic for adding and removing channels, and consider profiling your application to identify bottlenecks or unnecessary operations that trigger frequent rebuilds.
In the context of Netty, the concept of rebuilding selectors multiple times can significantly impact the performance and efficiency of network applications. A selector in Netty is responsible for monitoring multiple channels for events, such as incoming data or connection requests. When the rebuildSelector method is invoked frequently, it can lead to increased overhead, as each rebuild process requires the allocation of resources and the reconfiguration of the selector’s state.
One of the key takeaways is that while rebuilding selectors may be necessary in certain scenarios, it should be approached with caution. Developers should assess whether the benefits of rebuilding outweigh the costs associated with the operation. Strategies such as pooling selectors or optimizing the event handling logic can help mitigate the performance penalties associated with frequent selector rebuilds.
Moreover, understanding the underlying architecture of Netty and its event-driven model is crucial for making informed decisions about selector management. By leveraging Netty’s capabilities effectively, developers can enhance the scalability and responsiveness of their applications while minimizing the need for costly selector rebuilds. This strategic approach ultimately leads to a more robust and efficient network application.
Author Profile
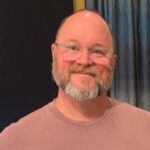
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?