Can We Use an Else Clause with a While Loop in Python?
When diving into the world of Python programming, many developers find themselves enchanted by the language’s simplicity and versatility. One of the more intriguing features that Python offers is the ability to use an `else` clause in conjunction with a `while` loop. While this may seem unconventional at first—especially for those accustomed to other programming languages—understanding this unique construct can enhance your coding skills and improve the readability of your programs. In this article, we will explore the nuances of using `else` with `while` loops, unraveling its purpose and demonstrating how it can be effectively employed in your code.
In Python, the `else` clause can be a powerful addition to your looping constructs, providing a way to execute a block of code after the loop has completed its iterations. This behavior is distinct from what many might expect, as it does not serve as a fallback for loop termination due to an error or break statement. Instead, it signifies that the loop has finished executing normally, allowing developers to include additional logic that should only run when the loop concludes without interruption.
As we delve deeper into this topic, we will clarify the mechanics behind the `else` clause in `while` loops, discuss scenarios where it can be particularly useful, and provide examples that
Understanding the Else Clause in While Loops
In Python, the `else` clause can be utilized with `while` loops, which might seem unconventional to programmers coming from other languages. The `else` block will execute after the `while` loop concludes, provided that the loop was not terminated by a `break` statement. This feature allows for cleaner and more readable code by grouping the loop’s completion logic with the associated conditional logic.
How the Else Clause Works
When a `while` loop is executed, it checks the condition and continues to execute as long as the condition remains `True`. Once the condition becomes “, the loop will exit, and the code in the `else` block will run. However, if the loop is exited prematurely due to a `break` statement, the `else` block will not execute.
For example:
“`python
count = 0
while count < 5:
print(count)
count += 1
else:
print("Loop completed successfully!")
```
In this code:
- The loop prints numbers from 0 to 4.
- After the loop completes, “Loop completed successfully!” is printed.
If you were to include a `break` statement:
“`python
count = 0
while count < 5:
if count == 3:
break
print(count)
count += 1
else:
print("Loop completed successfully!")
```
Here, the output would be:
```
0
1
2
```
The `else` block will not execute because the loop was terminated by the `break` statement.
Use Cases for Else with While Loops
Using `else` with a `while` loop can enhance the clarity of your code in certain scenarios:
- Search Operations: When searching through a collection, the `else` block can indicate that the search was unsuccessful if the loop completes without finding the desired element.
- Resource Management: In cases where resources are allocated within the loop, the `else` can handle cleanup operations if the loop completes normally.
Example Use Case
Consider a scenario where you are searching for an item in a list:
“`python
items = [1, 2, 3, 4, 5]
target = 6
found =
index = 0
while index < len(items): if items[index] == target: found = True break index += 1 else: print("Item not found.") if found: print("Item found.") ``` In this example:
- If the target item (6) is not in the list, the `else` block executes, printing “Item not found.”
- If the item is found, the `else` block is skipped.
Table of Key Points
Condition | Else Execution |
---|---|
Loop completes normally | Else block executes |
Loop is exited via break | Else block does not execute |
The `else` clause can be a powerful tool in structuring your `while` loops, allowing for elegant handling of scenarios where further actions depend on the loop’s execution path.
Understanding the Else Clause in While Loops
In Python, the `else` clause can indeed be used with a `while` loop. This feature might be unfamiliar to many programmers coming from other languages, as it is not a common practice. The `else` block is executed when the `while` loop terminates naturally, that is, when the loop condition becomes . However, if the loop is exited prematurely with a `break` statement, the `else` block will not be executed.
Syntax of While Loop with Else
The syntax for using an `else` clause with a `while` loop is straightforward:
“`python
while condition:
Loop body
Code to execute repeatedly
else:
Code to execute when loop finishes normally
“`
Example of While Loop with Else
Consider the following example that demonstrates this feature:
“`python
count = 0
while count < 5: print(count) count += 1 else: print("Loop finished normally.") ``` In this example, the numbers 0 to 4 will be printed, followed by the message "Loop finished normally." The `else` block is executed because the loop condition `count < 5` becomes without interruption.
Example with Break Statement
To illustrate the behavior when using a `break` statement, consider the following code:
“`python
count = 0
while count < 5: if count == 3: break print(count) count += 1 else: print("Loop finished normally.") ``` Here, the output will be: ``` 0 1 2 ``` The message "Loop finished normally." will not be printed because the loop is exited with a `break` when `count` equals 3.
Use Cases for Else in While Loops
Using `else` with `while` loops can be beneficial in specific scenarios:
- Search Operations: When searching for an item in a collection, the `else` can indicate that the search was unsuccessful if the loop completes without finding the item.
- Confirmation of Conditions: It can provide a clear path for executing code when a loop completes without interruptions, enhancing code readability.
Comparison with Other Loop Constructs
Feature | While Loop with Else | For Loop with Else |
---|---|---|
Executes on natural termination | Yes | Yes |
Executes on break statement | No | No |
Common use case | Iterative conditions | Iterating over collections |
In summary, the `else` clause in Python’s `while` loops serves a unique purpose that can enhance clarity and control flow in your code. By understanding its behavior, you can leverage this feature effectively in your programming practices.
Understanding the Use of Else with While Loops in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, the else clause can indeed be used with while loops. This feature allows developers to execute a block of code when the loop terminates normally, which can be particularly useful for handling scenarios where a loop is expected to run until a certain condition is met.”
Michael Chang (Lead Software Engineer, CodeCraft Solutions). “Utilizing an else statement with a while loop enhances code readability and can simplify logic. When the while loop exits without hitting a break statement, the else block executes, providing a clear way to handle post-loop operations.”
Sarah Thompson (Python Educator, LearnPythonNow). “For beginners, the else clause in a while loop can be a source of confusion. However, it serves as an elegant way to differentiate between normal termination and premature exits due to break statements, thus improving the overall flow of control in the program.”
Frequently Asked Questions (FAQs)
Can we use an else clause with a while loop in Python?
Yes, Python allows the use of an else clause with a while loop. The else block executes when the while loop terminates normally, meaning it did not encounter a break statement.
What is the purpose of the else clause in a while loop?
The else clause provides a way to execute a block of code after the while loop finishes its iterations, allowing for additional processing or cleanup without needing a separate condition check.
When does the else block execute in a while loop?
The else block executes after the while loop completes all its iterations without hitting a break statement. If the loop is exited via a break, the else block is skipped.
Can you provide an example of a while loop with an else clause?
Certainly. Here is an example:
“`python
count = 0
while count < 5:
print(count)
count += 1
else:
print("Loop finished without a break.")
```
In this example, "Loop finished without a break." will be printed after the loop completes.
Is using an else clause with a while loop common in Python programming?
Using an else clause with a while loop is less common than with a for loop, but it can be useful for specific scenarios where you want to distinguish between normal termination and termination due to a break.
Are there any performance implications of using an else clause with a while loop?
There are no significant performance implications of using an else clause with a while loop. The presence of the else block does not affect the loop’s performance; it merely provides additional functionality.
In Python, the use of the `else` clause with a `while` loop is a unique feature that distinguishes it from many other programming languages. The `else` block is executed after the `while` loop completes normally, meaning it runs when the loop condition evaluates to . This feature allows for cleaner code and can be particularly useful for scenarios where you need to execute a block of code after the loop has finished iterating, provided that the loop was not terminated by a `break` statement.
One of the key takeaways is that the `else` clause can enhance the readability and maintainability of your code. By using `else`, you can clearly separate the logic that should occur after the loop from the loop’s body itself. This can help prevent errors and make the code easier to follow, especially in complex algorithms where multiple exit points might exist.
Moreover, the `else` clause in a `while` loop can be particularly beneficial in search operations. For instance, if you are searching for an item and want to execute specific logic if the item is not found, the `else` clause allows you to handle that case cleanly without needing additional flags or conditions. Overall, understanding and utilizing the `else`
Author Profile
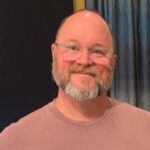
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?