How Can You Create a PR to Update the Value of a File in Golang?
In the world of software development, managing and updating files efficiently is a cornerstone of maintaining robust applications. For developers working with Go, or Golang, the need to create pull requests (PRs) to update file values is a common yet crucial task. Whether you’re collaborating on a team project or refining your own codebase, understanding how to effectively implement these changes through a PR can streamline your workflow and enhance your coding practices. This article delves into the intricacies of creating a PR to update file values in Go, equipping you with the knowledge to navigate this essential process with confidence.
Creating a pull request in Go not only involves modifying the file you wish to update but also understanding the broader context of version control systems like Git. A PR serves as a formal request to merge your changes into the main codebase, allowing for code review and collaboration. This process ensures that any modifications are scrutinized for quality and compatibility before they become part of the project, fostering a culture of accountability and teamwork.
In this article, we will explore the step-by-step approach to crafting a PR that updates file values in a Go project. From setting up your local development environment to pushing your changes and submitting your PR, we will cover the essential practices that can help you contribute effectively to any
Create a Pull Request to Update a Value of a File in Golang
Creating a pull request (PR) to update a file in a Golang project involves several steps, including making changes to the code, committing those changes, and then pushing them to a remote repository. This process allows for collaborative development and code review, ensuring that modifications are vetted before merging into the main codebase.
To initiate this process, follow these steps:
- Clone the Repository: If you haven’t already, clone the repository to your local machine using the `git clone` command.
“`bash
git clone https://github.com/username/repository.git
cd repository
“`
- Create a New Branch: Before making changes, create a new branch to work on. This keeps your changes isolated from the main codebase.
“`bash
git checkout -b update-file-value
“`
- Modify the File: Open the file you intend to update in your preferred text editor or IDE. Make the necessary changes to the file. For instance, if you need to change a configuration value:
“`go
// Original value
const MaxRetries = 3
// Updated value
const MaxRetries = 5
“`
- Stage Your Changes: After editing the file, stage the changes for commit.
“`bash
git add path/to/your/file.go
“`
- Commit Your Changes: Commit the changes with a descriptive message that summarizes what was modified.
“`bash
git commit -m “Update MaxRetries value from 3 to 5”
“`
- Push Your Branch: Push your branch to the remote repository.
“`bash
git push origin update-file-value
“`
- Create the Pull Request: Navigate to the repository on GitHub (or your version control platform), and you will typically see an option to create a pull request for your newly pushed branch. Click on it and fill out the necessary details.
- Title: Clearly describe the changes made.
- Description: Provide additional context, such as why the change was necessary and any implications it may have.
Here’s an example of a PR description:
“`
This PR updates the MaxRetries constant in the config file from 3 to 5 to allow for more retries in case of transient errors.
“`
- Review and Merge: Once the PR is created, team members can review the changes. They may provide feedback or request further modifications. After approval, the PR can be merged into the main branch.
Best Practices for Creating a Pull Request
To ensure your PR is effective and facilitates a smooth review process, adhere to the following best practices:
- Keep Changes Small: Limit the scope of your PR to a single change or feature to simplify the review process.
- Write Clear Commit Messages: Clearly explain what each commit does to provide context for reviewers.
- Include Tests: If applicable, include tests that cover the changes made to verify that the functionality works as expected.
- Follow Code Style Guidelines: Ensure that your code adheres to the project’s coding standards for consistency.
Step | Command | Description |
---|---|---|
Clone Repository | git clone | Clone the remote repository to local |
Create Branch | git checkout -b | Create a new branch for changes |
Stage Changes | git add | Stage modified files for commit |
Commit Changes | git commit -m | Commit changes with a message |
Push Branch | git push origin | Push changes to remote repository |
Creating a Pull Request to Update a File in Go
To create a pull request (PR) for updating a file in a Go project, you need to follow several steps. This process involves modifying the file, committing your changes, pushing them to your branch, and finally creating the pull request on your version control platform (e.g., GitHub, GitLab).
Step-by-Step Guide
- Clone the Repository
If you haven’t already, clone the repository to your local machine using the following command:
“`bash
git clone https://github.com/username/repo.git
cd repo
“`
- Create a New Branch
It’s best practice to create a new branch for your changes:
“`bash
git checkout -b update-file
“`
- Modify the File
Open the file you wish to update in your preferred text editor. Make the necessary changes. For example, if you’re updating a configuration file or a source file, ensure your changes align with Go’s syntax and conventions.
- Check Your Changes
After making changes, you can view the modifications using:
“`bash
git status
git diff
“`
- Stage Your Changes
Once you are satisfied with the modifications, stage the changes:
“`bash
git add path/to/modified/file.go
“`
- Commit Your Changes
Commit the changes with a descriptive message:
“`bash
git commit -m “Update file.go to improve functionality”
“`
- Push Your Changes to GitHub
Push your changes to the remote repository:
“`bash
git push origin update-file
“`
- Create the Pull Request
Navigate to your repository on GitHub (or your chosen platform) and follow these steps:
- Click on the “Pull Requests” tab.
- Click on the “New Pull Request” button.
- Select your branch (e.g., `update-file`) from the dropdown.
- Review the changes and write a title and description for your pull request.
- Click “Create Pull Request.”
Best Practices for Pull Requests
When creating a pull request, consider the following best practices to enhance collaboration and code quality:
- Descriptive Titles: Ensure the pull request title clearly describes the changes.
- Detailed Descriptions: Provide context in the description, including:
- Purpose of the changes.
- Any relevant issues or tickets.
- Testing performed to verify functionality.
- Code Review: Encourage team members to review your code, and be open to feedback.
- Link Issues: If your PR addresses a specific issue, reference it in the description using keywords like `Fixes issue_number`.
Common Tools and Commands
Tool/Command | Description |
---|---|
`git status` | Shows the status of changes in your working directory. |
`git diff` | Displays changes between commits or working tree. |
`git log` | Shows the commit history for the repository. |
`git rebase` | Reapplies commits on top of another base tip. |
Following these steps and guidelines will streamline the process of creating a pull request in a Go project, facilitating effective collaboration and code management.
Expert Insights on Creating PRs to Update File Values in Golang
Dr. Emily Carter (Senior Software Engineer, GoLang Innovations). “When creating a pull request to update a file’s value in Golang, it is crucial to maintain clarity in your commit messages. This practice not only aids in tracking changes but also enhances collaboration among team members.”
Michael Tran (Lead Developer, Tech Solutions Inc.). “Utilizing Go’s built-in file handling capabilities is essential when updating file values. Ensure that your code adheres to Go’s idiomatic practices, as this will facilitate easier reviews and integration during the PR process.”
Sarah Patel (Open Source Contributor, Go Community). “It’s important to include tests that validate the changes made in your pull request. This not only ensures that the updated file behaves as expected but also builds trust within the community regarding the stability of your contributions.”
Frequently Asked Questions (FAQs)
What is the purpose of creating a PR to update the value of a file in Golang?
Creating a Pull Request (PR) allows developers to propose changes, such as updating a file’s value, to a codebase. This process facilitates code review, discussion, and collaboration before the changes are merged into the main branch.
How do I create a PR for a file update in a Golang project?
To create a PR, first, fork the repository, clone it locally, create a new branch, make your changes to the file, commit the changes, and then push the branch to your fork. Finally, navigate to the original repository and create a PR from your branch.
What steps should I follow to ensure my file update is properly reviewed?
Include a clear description of the changes in your PR, reference any related issues, and ensure your code adheres to the project’s coding standards. Additionally, consider adding tests if applicable and tag relevant team members for review.
How can I handle merge conflicts when creating a PR?
To resolve merge conflicts, pull the latest changes from the main branch into your feature branch. Manually edit the conflicting files to reconcile differences, then commit the resolved changes before updating your PR.
Is it necessary to run tests after updating a file in Golang?
Yes, running tests is crucial after any file update to ensure that the changes do not introduce bugs or break existing functionality. This practice maintains code integrity and reliability.
What should I do if my PR is not being reviewed in a timely manner?
If your PR is not reviewed promptly, consider politely following up with the team or project maintainers. You can also engage in discussions on the project’s communication channels to highlight the importance of your changes.
creating a pull request (PR) to update the value of a file in Golang involves a systematic approach that encompasses understanding the version control system, making the necessary code changes, and ensuring proper documentation. The process typically starts with cloning the repository, creating a new branch, and modifying the desired file. It is crucial to follow best practices for code quality and maintainability during this phase.
Furthermore, after making the changes, it is essential to commit the updates with a clear and descriptive message that reflects the purpose of the modification. This practice not only aids in tracking changes but also facilitates collaboration among team members. Once the changes are committed, pushing the branch to the remote repository and initiating a pull request allows for code review and discussion before merging into the main branch.
Key takeaways from this discussion include the importance of adhering to coding standards and ensuring thorough testing of changes before submission. Additionally, engaging with team members during the review process can lead to valuable feedback and improvements. Overall, creating a PR to update a file in Golang is a critical skill that enhances collaborative development and contributes to the overall quality of the codebase.
Author Profile
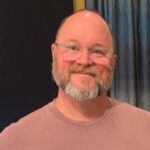
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?