How Can You Make an ASPX Checkbox Not Visible?
### Introduction
In the world of web development, user experience is paramount, and sometimes, less is more. When designing interactive web applications using ASP.NET, developers often encounter scenarios where certain elements, such as checkboxes, should be hidden from view. This can be crucial for maintaining a clean interface, guiding user interactions, or managing conditional logic in forms. If you’ve ever wondered how to make an ASP.NET checkbox not visible, you’re in the right place. This article will explore various methods to achieve this, ensuring your web application remains intuitive and user-friendly.
### Overview
Making an ASP.NET checkbox invisible is a straightforward yet essential task that can enhance the overall functionality of your web application. By understanding the different techniques available, developers can choose the most suitable approach based on their specific requirements. Whether you prefer to manipulate the checkbox’s visibility through server-side code or leverage client-side scripting, there are multiple strategies to ensure that unnecessary elements do not clutter your user interface.
In this article, we will delve into the various methods to hide an ASPX checkbox, discussing the implications of each approach and providing practical examples. From using the `Visible` property in the code-behind to employing CSS for dynamic visibility changes, we will equip you with the knowledge needed to streamline your forms and improve user
Understanding the Visibility of ASP.NET CheckBox Controls
ASP.NET CheckBox controls can be made invisible for various reasons, such as improving user interface design or controlling user interactions based on certain conditions. When a CheckBox is not visible, it does not render on the page, preventing users from interacting with it.
There are several methods to achieve this, each with its own use cases and implications.
Using the Visible Property
One of the simplest methods to hide an ASP.NET CheckBox is by setting its `Visible` property to “. This property determines whether the control is rendered on the page.
aspx
In this example, the CheckBox will not be visible to the user. The control will not be included in the rendered HTML, which can be beneficial for preventing user interaction.
CSS Display Property
Another approach is to use CSS to control visibility. This method allows you to hide the CheckBox while still keeping it in the page’s DOM. You can apply CSS styles directly to the CheckBox or use a class.
aspx
css
.hidden-checkbox {
display: none;
}
By using the CSS `display: none;` rule, the CheckBox will not be displayed, but it remains part of the page structure, allowing for potential future manipulation.
Conditional Visibility with Code-Behind
You might want to conditionally hide a CheckBox based on user input or other criteria. This can be done in the code-behind file.
csharp
protected void Page_Load(object sender, EventArgs e)
{
if (someCondition)
{
CheckBox1.Visible = ;
}
}
This allows for dynamic control of the CheckBox’s visibility based on runtime conditions, enhancing the interactivity of your application.
Table of Visibility Methods
Method | Description | Impact on DOM |
---|---|---|
Visible Property | Sets the CheckBox not to render on the page. | Not included in DOM |
CSS Display Property | Hides the CheckBox using CSS styles. | Included in DOM |
Conditional Code-Behind | Dynamically controls visibility during page load. | Depends on conditions |
Considerations for Hiding CheckBoxes
When deciding to hide CheckBox controls, consider the following:
- User Experience: Ensure that hiding a CheckBox does not confuse users. If a CheckBox is hidden as part of a conditional workflow, provide clear feedback.
- Accessibility: Consider how hiding elements affects users relying on assistive technologies. Ensure that any hidden CheckBox still conveys necessary information.
- Validation: If a CheckBox is hidden, ensure that it does not interfere with validation requirements when submitting forms.
By carefully managing the visibility of CheckBox controls, you can create a more streamlined and user-friendly interface in your ASP.NET applications.
Methods to Hide an ASPX Checkbox
To make an ASPX Checkbox control (CheckBox) not visible on a web page, several approaches can be employed. Below are the most common methods.
Using CSS to Hide the Checkbox
One effective way to hide an ASPX CheckBox is through CSS. This approach ensures that the checkbox is not rendered visually on the page while keeping it in the DOM.
css
.hidden-checkbox {
display: none; /* Hides the checkbox completely */
}
This CSS rule will hide any checkbox with the class `hidden-checkbox`.
Setting the Visible Property
ASP.NET controls, including CheckBox, have a `Visible` property that can be set to “ to remove the control from the page rendering.
csharp
CheckBox1.Visible = ; // Hides the checkbox from rendering
When set to “, the checkbox will not appear on the page at all.
Using JavaScript for Dynamic Visibility
JavaScript can be used to hide or show the checkbox dynamically based on user actions or other conditions. This method allows for greater interactivity.
In this example, clicking the button will toggle the visibility of the checkbox.
Conditional Rendering in ASPX
You can also conditionally render the checkbox based on some server-side logic using the `Visible` property in the code-behind.
csharp
if (someCondition) {
CheckBox1.Visible = true; // Checkbox will be visible
} else {
CheckBox1.Visible = ; // Checkbox will be hidden
}
This method is particularly useful for scenarios where the visibility of the checkbox depends on specific conditions evaluated during page load.
Utilizing Panel Control
Wrapping the checkbox within a Panel control can also facilitate visibility management. You can control the visibility of the entire Panel, which will include the checkbox.
csharp
Panel1.Visible = ; // Hides the entire panel, including the checkbox
This method is helpful when you want to group multiple controls together.
Summary of Methods
Method | Description |
---|---|
CSS | Use `display: none;` to hide the checkbox visually. |
Visible Property | Set `CheckBox1.Visible = ;` in code-behind. |
JavaScript | Dynamically toggle visibility using JavaScript. |
Conditional Rendering | Use conditions to set visibility in the code-behind. |
Panel Control | Wrap the checkbox in a Panel and control its visibility. |
These methods provide various ways to manage the visibility of ASPX CheckBox controls effectively, depending on the requirements of your application.
Strategies for Hiding AspxCheckbox in Web Applications
Dr. Emily Carter (Senior Web Developer, Tech Innovations Inc.). “To make an AspxCheckbox not visible, developers can set the ‘Visible’ property to in the code-behind file. This approach ensures that the checkbox is not rendered in the HTML, effectively removing it from the user interface.”
Mark Thompson (UI/UX Designer, Creative Solutions). “Hiding an AspxCheckbox can also be achieved through CSS by applying ‘display: none;’ to the checkbox’s ID. This method allows for more dynamic control, especially when toggling visibility based on user interactions.”
Linda Garcia (Software Engineer, Web Dynamics). “In scenarios where the checkbox needs to be conditionally hidden, leveraging JavaScript to manipulate the DOM can be highly effective. By using JavaScript to set the checkbox’s style to ‘display: none;’, developers can create a responsive user experience.”
Frequently Asked Questions (FAQs)
How can I make an ASPX checkbox not visible on the page?
You can set the `Visible` property of the checkbox to “ in your ASPX code. For example: `
Is it possible to hide an ASPX checkbox using CSS?
Yes, you can use CSS to hide the checkbox by applying a style like `display: none;` to the checkbox. For instance: ` `.
Can I control the visibility of an ASPX checkbox dynamically with C#?
Yes, you can control the visibility in the code-behind file by setting the `Visible` property based on certain conditions. For example: `CheckBox1.Visible = ;` within an event handler.
What is the difference between setting Visible to and using CSS to hide the checkbox?
Setting `Visible` to removes the checkbox from the page’s control tree, while using CSS only hides it visually but keeps it in the control tree, which may affect postbacks and events.
Are there any accessibility concerns when hiding an ASPX checkbox?
Yes, hiding elements can affect accessibility. Ensure that hidden checkboxes do not disrupt the user experience for assistive technologies. Consider using `aria-hidden=”true”` for better accessibility practices.
Can I make an ASPX checkbox visible again after hiding it?
Yes, you can set the `Visible` property back to `true` in your code-behind or change the CSS style to make it visible again. For example: `CheckBox1.Visible = true;` or changing the CSS to `display: block;`.
In summary, making an ASP.NET CheckBox control invisible can be accomplished through various methods, primarily focusing on the properties and visibility settings of the control. The most straightforward approach is to set the CheckBox’s `Visible` property to “, which effectively removes it from the rendered page. Additionally, developers may consider using CSS to hide the CheckBox by applying a style that sets its display to `none`, providing more flexibility in managing the control’s visibility without altering its server-side state.
It is essential to understand the implications of hiding a CheckBox, especially in terms of user experience and accessibility. While hiding a control may be necessary for certain application logic, it is crucial to ensure that users are still able to interact with the necessary elements of the interface. Developers should consider alternative approaches, such as disabling the CheckBox or providing clear visual cues, to maintain a seamless user experience.
Ultimately, the choice of method for making an ASP.NET CheckBox invisible should align with the overall design and functionality of the web application. By carefully evaluating the context in which the CheckBox is used, developers can make informed decisions that enhance usability while achieving the desired visibility outcomes. This strategic approach contributes to a more effective and user-friendly application design.
Author Profile
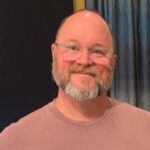
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?