How Can You Convert a String JSON to a JSON Object in Java?
In the world of software development, data interchange formats play a crucial role in enabling seamless communication between applications. Among these formats, JSON (JavaScript Object Notation) has emerged as a favorite due to its lightweight structure and human-readable format. For Java developers, the ability to convert a string representation of JSON into a usable JSON object is an essential skill that can unlock a plethora of functionalities, from API integrations to data manipulation. Whether you’re building a web application, working with microservices, or simply handling data exchanges, mastering the conversion of a string to a JSON object in Java is a vital step in your programming journey.
Understanding how to transform a string into a JSON object in Java involves familiarizing yourself with various libraries and methods that facilitate this process. Java offers several powerful libraries, such as Jackson and Gson, which simplify the parsing and handling of JSON data. These libraries not only allow you to convert strings into JSON objects but also provide tools for serializing and deserializing complex data structures, making it easier to work with data in a structured format.
As we delve deeper into this topic, we will explore the intricacies of JSON parsing in Java, highlighting the best practices and common pitfalls developers may encounter. Armed with this knowledge, you will be better equipped to manage JSON
Converting String to JSON Object
To convert a JSON string into a JSON object in Java, you typically use libraries such as Gson or Jackson. These libraries provide straightforward methods to parse JSON strings and create corresponding Java objects. Below are step-by-step guidelines for both libraries.
Using Gson
Gson is a popular Java library developed by Google for converting Java Objects into their JSON representation and vice versa. To convert a JSON string to a JSON object using Gson, you would follow these steps:
- Add Gson Dependency: Include the Gson library in your project. If you are using Maven, add the following to your `pom.xml`:
“`xml
“`
- Convert String to JSON Object:
Use the following code to convert a JSON string into a JsonObject:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
Using Jackson
Jackson is another widely used library for handling JSON in Java. It provides powerful features and is particularly well-suited for working with large JSON datasets. Here’s how to convert a JSON string to a JSON object using Jackson:
- Add Jackson Dependency: Include the Jackson library. For Maven, add the following to your `pom.xml`:
“`xml
“`
- Convert String to JSON Object:
Use the following code snippet:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) throws Exception {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
}
}
“`
Comparison of Gson and Jackson
Both Gson and Jackson offer robust capabilities for JSON processing, but they have differences in performance, features, and ease of use. The following table summarizes key aspects:
Feature | Gson | Jackson |
---|---|---|
Performance | Moderate | High |
Ease of Use | Simple API | More features, slightly complex |
Streaming API | No | Yes |
Data Binding | Yes | Yes |
In summary, both libraries can effectively convert JSON strings to JSON objects in Java. The choice between Gson and Jackson generally depends on the specific requirements of your project, such as performance needs and the complexity of JSON data structures being handled.
Converting String JSON to JSON Object in Java
In Java, converting a JSON string to a JSON object can be efficiently handled using libraries such as `org.json`, `Gson`, or `Jackson`. Each library has its own methods and utilities for parsing JSON strings. Below are examples using these popular libraries.
Using org.json Library
The `org.json` library provides a straightforward way to convert a JSON string to a JSON object. This library is included in many Java projects and can be easily added to your dependencies.
“`java
import org.json.JSONObject;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject.getString(“name”)); // Outputs: John
System.out.println(jsonObject.getInt(“age”)); // Outputs: 30
}
}
“`
Using Gson Library
Gson, developed by Google, allows easy conversion between JSON and Java objects. To convert a JSON string to a JSON object, you can use the `JsonParser` class.
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class GsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject.get(“name”).getAsString()); // Outputs: John
System.out.println(jsonObject.get(“age”).getAsInt()); // Outputs: 30
}
}
“`
Using Jackson Library
Jackson is another powerful library for handling JSON in Java. To convert a JSON string to a JSON object, you can utilize the `ObjectMapper` class.
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JacksonExample {
public static void main(String[] args) throws Exception {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode.get(“name”).asText()); // Outputs: John
System.out.println(jsonNode.get(“age”).asInt()); // Outputs: 30
}
}
“`
Comparative Overview of Libraries
Feature | org.json | Gson | Jackson |
---|---|---|---|
Ease of Use | Simple API | Moderate | Moderate |
Performance | Good | Good | Excellent |
Dependency Size | Small | Moderate | Moderate |
Mapping to Java Objects | Limited | Excellent | Excellent |
Streaming Support | No | Yes | Yes |
Common Issues and Troubleshooting
When converting JSON strings to JSON objects, several common issues may arise:
- Malformed JSON: Ensure that the JSON string is correctly formatted. Use online validators to check the syntax.
- Data Type Mismatches: Confirm that the expected data types in your Java code match those in the JSON string.
- Library Dependency Conflicts: Ensure that there are no conflicting versions of JSON libraries in your project.
Utilizing the above methods allows for effective handling of JSON data in Java applications, enabling developers to seamlessly integrate JSON parsing capabilities.
Expert Insights on Converting String JSON to JSON Object in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Converting a String representation of JSON into a JSON object in Java is a fundamental skill for developers. Utilizing libraries such as Jackson or Gson can streamline this process significantly, allowing for efficient parsing and manipulation of JSON data.”
Michael Thompson (Lead Java Developer, CodeCraft Solutions). “When handling String JSON in Java, it’s crucial to ensure that the JSON format is valid before conversion. Tools like JSONLint can be invaluable for debugging. Once validated, libraries like org.json provide straightforward methods to convert Strings into JSON objects seamlessly.”
Sarah Lee (Java Architect, Enterprise Systems Group). “Performance considerations are key when converting String JSON to JSON objects in Java. For large datasets, utilizing streaming APIs offered by Jackson can enhance performance and reduce memory overhead, making it a preferred choice for enterprise applications.”
Frequently Asked Questions (FAQs)
What is the process to convert a String JSON to a JSON Object in Java?
To convert a String JSON to a JSON Object in Java, you can use libraries like `org.json` or `Gson`. For `org.json`, use `new JSONObject(jsonString)`; for Gson, use `new Gson().fromJson(jsonString, JsonObject.class)`.
What libraries are commonly used for JSON manipulation in Java?
Common libraries for JSON manipulation in Java include `org.json`, `Gson`, `Jackson`, and `JsonPath`. Each library has its own methods and features for parsing and generating JSON.
Can I convert a JSON Array String to a JSON Array Object in Java?
Yes, you can convert a JSON Array String to a JSON Array Object. For `org.json`, use `new JSONArray(jsonArrayString)`. For Gson, use `new Gson().fromJson(jsonArrayString, JsonArray.class)`.
What exceptions should I handle when converting String JSON to JSON Object?
You should handle `JSONException` for `org.json` and `JsonSyntaxException` for Gson. These exceptions indicate issues with the JSON format or parsing errors.
Is it possible to convert a JSON Object back to a String in Java?
Yes, you can convert a JSON Object back to a String. For `org.json`, use `jsonObject.toString()`. For Gson, use `new Gson().toJson(jsonObject)`.
What is the difference between JSONObject and JsonObject in Java?
`JSONObject` is part of the `org.json` library, while `JsonObject` is part of the Gson library. They serve similar purposes but have different methods and functionalities based on their respective libraries.
In Java, converting a string in JSON format to a JSON object is a common task that developers encounter when working with APIs or data interchange formats. The primary libraries used for this purpose include the popular Gson and Jackson libraries. Both libraries provide straightforward methods to parse JSON strings and convert them into Java objects, facilitating easier data manipulation and access.
Utilizing these libraries, developers can efficiently handle JSON data by leveraging their built-in functionalities. For instance, Gson offers the `fromJson` method, which allows for direct conversion from a JSON string to a specified Java class. Similarly, Jackson provides the `ObjectMapper` class, which can deserialize JSON strings into Java objects with ease. These tools not only streamline the conversion process but also enhance code readability and maintainability.
In summary, understanding how to convert a string in JSON format to a JSON object in Java is essential for effective data handling. By employing libraries like Gson and Jackson, developers can simplify this process, ensuring that their applications can easily interact with JSON data structures. This knowledge is invaluable for building robust applications that rely on data exchange and integration with various services.
Author Profile
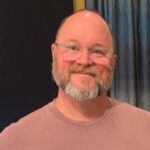
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?