How Can You Create Text Box Graphics in Python?
### Introduction
In the ever-evolving world of Python programming, creating visually appealing applications is just as important as writing efficient code. Whether you’re developing a desktop application, a game, or a data visualization tool, the ability to integrate graphics seamlessly can elevate your project to new heights. One of the most effective ways to enhance user interaction and display information is through the use of text box graphics. If you’re looking to add a touch of creativity and functionality to your Python projects, mastering text box graphics is a skill worth acquiring.
Text box graphics serve as a powerful tool for developers, allowing them to present information in a clear and engaging manner. These graphical elements can be customized to suit various themes and styles, making them versatile for any application. From simple text inputs to complex data displays, text boxes can enhance user experience by providing intuitive ways to interact with your program. Understanding how to implement and manipulate these graphics can open up new avenues for creativity and functionality in your projects.
As we delve deeper into the world of text box graphics in Python, you’ll discover a range of libraries and techniques that can help you create stunning visual interfaces. Whether you’re a novice programmer or an experienced developer, this guide will equip you with the knowledge and tools necessary to bring your ideas to life. Get ready to transform
Understanding Text Box Graphics in Python
To create text box graphics in Python, one of the most commonly used libraries is `Tkinter`. It provides a simple way to create graphical user interfaces (GUIs) and allows for the integration of text boxes that can enhance user interaction. Text boxes serve as input fields or display areas for text, and customizing them can significantly improve the usability of your application.
Creating a Basic Text Box
To start, you need to import the `Tkinter` library and initialize the main application window. Here’s a basic example of how to create a text box:
python
import tkinter as tk
root = tk.Tk()
root.title(“Text Box Example”)
text_box = tk.Text(root, height=10, width=30)
text_box.pack()
root.mainloop()
In this example:
- The `Text` widget creates a multi-line text box.
- The `height` and `width` parameters define the size of the text box.
Customizing Text Box Appearance
Customization options in `Tkinter` allow you to enhance the visual appeal and functionality of the text box. Here are some key properties you can modify:
- Font: Change the font style and size.
- Background and foreground colors: Alter the text and background colors for better readability.
- Borders: Adjust the border width for aesthetic purposes.
Here’s how you can customize a text box:
python
text_box.config(font=(“Arial”, 12), bg=”light yellow”, fg=”dark blue”, bd=5)
Handling Text Input
To manipulate the text input and retrieve data from the text box, you can use various methods available in the `Text` widget. Key methods include:
- get(start, end): Retrieves text from the specified range.
- insert(index, text): Inserts text at the given index.
- delete(start, end): Deletes text from the specified range.
For example, you can retrieve text from the text box like this:
python
input_text = text_box.get(“1.0”, “end-1c”) # Gets all text
Table of Common Text Box Methods
Method | Description |
---|---|
get(start, end) | Fetches text from the specified start to end index. |
insert(index, text) | Inserts text at the specified index. |
delete(start, end) | Removes text from the specified start to end index. |
index(pos) | Returns the character index for the given position. |
Event Handling with Text Boxes
To enhance interactivity, you can bind events to the text box. For instance, you might want to perform an action whenever the text changes or when the user presses a specific key.
You can use the `bind` method to link an event to a function:
python
def on_text_change(event):
print(“Text changed!”)
text_box.bind(“
This code snippet will print a message to the console each time a key is released while typing in the text box.
Incorporating text box graphics in Python applications using `Tkinter` allows for a richer user interface. By following the guidelines provided, developers can create functional and visually appealing text boxes that enhance user engagement and interaction.
Understanding Text Box Graphics in Python
Text box graphics in Python can be effectively implemented using various libraries, such as Tkinter, Pygame, and Matplotlib. Each library offers unique functionalities to create and manipulate text boxes for different applications.
Using Tkinter for Text Boxes
Tkinter is the standard GUI toolkit for Python, making it an excellent choice for creating text box graphics. The `Entry` widget is commonly used for single-line text input, while the `Text` widget handles multi-line text input.
Key Features:
- Entry Widget:
- Use for single-line text input.
- Configurable background and foreground colors.
- Text Widget:
- Supports multi-line text.
- Allows text formatting (font, size, color).
Example Code:
python
import tkinter as tk
def submit():
print(entry.get())
text_box.insert(tk.END, “Submitted: ” + entry.get() + “\n”)
root = tk.Tk()
root.title(“Text Box Example”)
entry = tk.Entry(root, width=50)
entry.pack()
submit_button = tk.Button(root, text=”Submit”, command=submit)
submit_button.pack()
text_box = tk.Text(root, height=10, width=50)
text_box.pack()
root.mainloop()
Creating Text Boxes with Pygame
Pygame is a versatile library for game development in Python. It allows for the creation of text boxes that can handle user input effectively in a graphical window.
Implementation Steps:
- Initialize Pygame and create a display surface.
- Render the text box using `pygame.draw.rect`.
- Capture user input using event handling.
Example Code:
python
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 300))
font = pygame.font.Font(None, 36)
input_box = pygame.Rect(100, 100, 200, 32)
color_inactive = pygame.Color(‘lightskyblue3’)
color_active = pygame.Color(‘dodgerblue2’)
color = color_inactive
active =
text = ”
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.MOUSEBUTTONDOWN:
if input_box.collidepoint(event.pos):
active = not active
else:
active =
color = color_active if active else color_inactive
if event.type == pygame.KEYDOWN:
if active:
if event.key == pygame.K_RETURN:
print(text)
text = ”
elif event.key == pygame.K_BACKSPACE:
text = text[:-1]
else:
text += event.unicode
screen.fill((30, 30, 30))
txt_surface = font.render(text, True, color)
width = max(200, txt_surface.get_width()+10)
input_box.w = width
screen.blit(txt_surface, (input_box.x+5, input_box.y+5))
pygame.draw.rect(screen, color, input_box, 2)
pygame.display.flip()
Employing Matplotlib for Text Box Annotations
Matplotlib is primarily used for plotting but can also create text boxes within plots using annotations.
Text Box Creation:
- Use `plt.text` or `plt.annotate` to display text within the plot area.
- Customize the text box with a background color and border.
Example Code:
python
import matplotlib.pyplot as plt
plt.figure()
plt.plot([1, 2, 3], [1, 4, 9])
plt.text(1.5, 5, ‘Sample Text Box’, bbox=dict(facecolor=’white’, alpha=0.5))
plt.show()
Comparison of Libraries for Text Box Graphics
Feature | Tkinter | Pygame | Matplotlib |
---|---|---|---|
GUI Support | Yes | Limited | No |
Multi-line Input | Yes | No | No |
Game Development | No | Yes | No |
Plot Integration | No | No | Yes |
By leveraging these libraries, developers can effectively integrate text box graphics into their Python applications, tailoring functionality to meet specific user interface or data visualization needs.
Expert Insights on Creating Text Box Graphics in Python
Dr. Emily Carter (Senior Data Visualization Specialist, Tech Insights). “To effectively create text box graphics in Python, leveraging libraries such as Matplotlib or Seaborn is essential. These libraries provide built-in functionalities that simplify the process of adding annotations and text boxes to visualizations, enhancing clarity and engagement.”
James Liu (Lead Software Engineer, DataCraft Solutions). “Utilizing the Tkinter library for GUI applications can significantly streamline the creation of text box graphics. By combining Tkinter with matplotlib, developers can create interactive plots that include text boxes for user input or information display, making applications more user-friendly.”
Sarah Mitchell (Python Developer and Educator, Code Academy). “For beginners, I recommend starting with simple text box graphics using the Pygame library. It allows for easy manipulation of graphics and text, providing a solid foundation for understanding how to integrate text boxes into more complex projects down the line.”
Frequently Asked Questions (FAQs)
How can I create a text box in Py?
To create a text box in Python, you can use libraries such as Tkinter or PyQt. In Tkinter, you can use the `Text` widget to create a multi-line text box, while in PyQt, you can use the `QTextEdit` class.
What libraries are commonly used for graphics in Python?
Common libraries for graphics in Python include Tkinter, Pygame, Matplotlib, and PyQt. Each library has its own strengths and is suited for different types of applications, such as GUI development or game design.
How do I style a text box in Tkinter?
You can style a text box in Tkinter by using options such as `bg` for background color, `fg` for text color, `font` for font style and size, and `width` and `height` for dimensions. These options can be set when initializing the `Text` widget.
Can I add graphics to a text box in PyQt?
Yes, you can add graphics to a text box in PyQt by using the `QTextEdit` class, which supports rich text formatting. You can insert images, formatted text, and other graphical elements using HTML and CSS within the text box.
Is it possible to get user input from a text box in Python?
Yes, user input can be retrieved from a text box in Python. In Tkinter, you can use the `get` method of the `Text` widget, while in PyQt, you can use the `toPlainText` method of the `QTextEdit` class to obtain the text entered by the user.
How do I handle events in a text box in Python?
Event handling in a text box can be achieved by binding events to the widget. In Tkinter, you can use the `bind` method to associate functions with events, while in PyQt, you can connect signals to slots using the `connect` method for specific user interactions.
In summary, creating text box graphics in Python can be achieved through various libraries, each offering unique features and functionalities. Popular choices include Tkinter for simple GUI applications, Matplotlib for data visualization, and Pygame for more interactive graphics. Each library provides different methods for rendering text boxes, allowing developers to choose the most suitable option based on their specific project requirements.
Moreover, understanding the fundamentals of each library is crucial for effectively implementing text box graphics. For instance, Tkinter’s `Text` widget allows for easy text manipulation, while Matplotlib’s annotation capabilities enable the incorporation of text boxes within plots. Pygame, on the other hand, offers flexibility for game development, making it ideal for creating dynamic text displays. Familiarity with these tools can significantly enhance a developer’s ability to create visually appealing applications.
Finally, it is essential to consider the overall design and usability when integrating text box graphics into applications. Properly styled and positioned text boxes can improve user experience and engagement. By leveraging the appropriate library and adhering to best practices in design, developers can effectively communicate information and enhance the functionality of their Python applications.
Author Profile
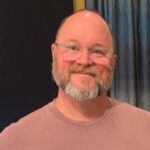
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?