How Can You Efficiently Remove an Item from an Array in PowerShell?
PowerShell, a powerful scripting language and command-line shell, has become an indispensable tool for system administrators and developers alike. One of its many capabilities is the efficient manipulation of data structures, including arrays. While arrays provide a convenient way to store collections of items, there are times when you might need to remove specific elements to streamline your data or adjust your scripts. Whether you’re cleaning up a list of user accounts, filtering out unwanted values, or simply refining your data set, mastering the art of removing items from an array in PowerShell is a skill that can significantly enhance your scripting prowess.
In this article, we will explore the various methods available for removing items from arrays in PowerShell. Understanding how to effectively manage array contents is crucial for anyone looking to optimize their scripts and improve performance. We will delve into the different techniques, including the use of built-in cmdlets and array manipulation methods, providing you with the knowledge to handle arrays with confidence and precision.
From simple removals to more complex filtering scenarios, this guide will equip you with the tools you need to manipulate arrays effectively. As we journey through the intricacies of PowerShell array management, you’ll discover best practices and tips that can elevate your scripting capabilities, making your workflows more efficient and your code cleaner.
Using the Remove-Item Cmdlet
The `Remove-Item` cmdlet in PowerShell is commonly used for deleting files, folders, or other items from the filesystem. However, when it comes to removing items from an array, `Remove-Item` is not directly applicable. Instead, PowerShell provides other methods to achieve this.
To remove an item from an array, you can utilize the `Where-Object` cmdlet or leverage array slicing. Here is how you can effectively remove an item from an array:
- Using Where-Object: This method filters the array by excluding the specified item.
“`powershell
$array = 1, 2, 3, 4, 5
$array = $array | Where-Object { $_ -ne 3 }
“`
- Using Array Slicing: You can create a new array that excludes the item you want to remove.
“`powershell
$array = 1, 2, 3, 4, 5
$array = $array[0..1] + $array[3..4]
“`
Example of Removing an Item
Consider an example where you have an array of numbers, and you want to remove the number `3`.
“`powershell
$array = 1, 2, 3, 4, 5
$array = $array | Where-Object { $_ -ne 3 }
“`
After executing the command, the contents of `$array` will be:
“`
1
2
4
5
“`
Removing Multiple Items
If you need to remove multiple items from an array, you can still use the `Where-Object` cmdlet by providing an array of items to exclude:
“`powershell
$array = 1, 2, 3, 4, 5
$itemsToRemove = 2, 4
$array = $array | Where-Object { $_ -notin $itemsToRemove }
“`
This results in:
“`
1
3
5
“`
Performance Considerations
When dealing with large arrays, the method of removing items can affect performance. Here’s a brief comparison of methods:
Method | Performance | Complexity |
---|---|---|
Where-Object | Good for small to medium arrays | Simple |
Array Slicing | Faster for large arrays | More complex |
In scenarios where performance is critical, consider the size of your array and the frequency of operations. For large datasets, array slicing may yield better performance compared to filtering through `Where-Object`.
PowerShell provides versatile methods for manipulating arrays, including removing items. Understanding the nuances of these methods can enhance your scripting efficiency and performance when working with collections in PowerShell.
Understanding Array Manipulation in PowerShell
In PowerShell, arrays are versatile data structures that allow for the storage and management of multiple items. Removing an item from an array can be accomplished using various methods. Each method has its own implications and use cases, depending on the specific requirements of the task.
Method 1: Using the `Where-Object` Cmdlet
The `Where-Object` cmdlet filters objects based on specified criteria, allowing you to create a new array without the item you wish to remove.
“`powershell
$array = 1, 2, 3, 4, 5
$itemToRemove = 3
$newArray = $array | Where-Object { $_ -ne $itemToRemove }
“`
Explanation:
- The original array contains five integers.
- The variable `$itemToRemove` specifies which item to exclude from the new array.
- The output, `$newArray`, will contain `1, 2, 4, 5`.
Method 2: Using Array Slicing
Array slicing provides a straightforward way to remove an item by creating a new array that excludes the desired index.
“`powershell
$array = 1, 2, 3, 4, 5
$indexToRemove = 2
$newArray = $array[0..($indexToRemove – 1)] + $array[($indexToRemove + 1)..($array.Length – 1)]
“`
Explanation:
- In this example, the item at index `2` (which is `3`) is removed.
- The new array is created by concatenating slices of the original array before and after the specified index.
- The resulting `$newArray` will be `1, 2, 4, 5`.
Method 3: Using `ArrayList` for Dynamic Removal
Using an `ArrayList` from the .NET framework allows for more dynamic manipulation, including easy removal of items.
“`powershell
$arrayList = [System.Collections.ArrayList]@(1, 2, 3, 4, 5)
$itemToRemove = 3
$arrayList.Remove($itemToRemove)
$newArray = @($arrayList)
“`
Explanation:
- An `ArrayList` is initialized with the same items.
- The `Remove` method directly modifies the list by removing the specified item.
- Finally, the result is cast back to a standard array.
Method 4: Using `RemoveAt` for Index-Based Removal
If you know the index of the item you wish to remove, `RemoveAt` can be utilized on an `ArrayList`.
“`powershell
$arrayList = [System.Collections.ArrayList]@(1, 2, 3, 4, 5)
$indexToRemove = 2
$arrayList.RemoveAt($indexToRemove)
$newArray = @($arrayList)
“`
Explanation:
- This method is efficient for known indices.
- It directly alters the `ArrayList`, simplifying the removal process and avoiding the need for filtering or slicing.
Considerations When Removing Items
When manipulating arrays in PowerShell, consider the following:
- Immutability of Arrays: Standard arrays are immutable in PowerShell; any modification results in a new array.
- Performance: For large datasets, using `ArrayList` may offer better performance for dynamic changes.
- Error Handling: Ensure that the item or index specified for removal exists to avoid runtime errors.
Method | Description | Performance |
---|---|---|
Where-Object | Filters items based on criteria | Moderate |
Array Slicing | Creates a new array excluding an index | Moderate |
ArrayList with Remove | Directly removes an item | High |
ArrayList with RemoveAt | Removes item by index | High |
By employing these methods, users can effectively manage and manipulate arrays in PowerShell, tailoring their approach to fit specific needs and scenarios.
Expert Insights on Powershell Remove Item From Array
Jessica Tran (Senior Systems Administrator, Tech Innovations Corp). “Using PowerShell to remove an item from an array is a fundamental skill for any systems administrator. The `Where-Object` cmdlet is particularly effective for filtering out unwanted elements, allowing for dynamic array management.”
Michael Chen (PowerShell Developer, CodeCraft Solutions). “When working with arrays in PowerShell, it is crucial to understand that arrays are fixed in size. Therefore, using the `+=` operator to remove items can lead to performance issues. Instead, leveraging the `ArrayList` class provides more flexibility and efficiency.”
Linda Patel (IT Consultant, FutureTech Strategies). “Incorporating error handling when removing items from an array is essential. Implementing try-catch blocks can prevent script failures and ensure that the user is informed of any issues during the execution of the removal process.”
Frequently Asked Questions (FAQs)
How can I remove an item from an array in PowerShell?
You can remove an item from an array in PowerShell using the `Where-Object` cmdlet or by creating a new array that excludes the item. For example, `$array = $array | Where-Object {$_ -ne $itemToRemove}`.
Is there a built-in method to remove an item from an array in PowerShell?
PowerShell does not have a built-in method to directly remove an item from an array since arrays are fixed in size. Instead, you must create a new array without the unwanted item.
Can I use the `Remove-Item` cmdlet to remove an item from an array?
No, the `Remove-Item` cmdlet is used for deleting files and folders in the file system, not for manipulating arrays. Use array manipulation techniques instead.
What is the difference between an array and an arraylist in PowerShell?
An array is a fixed-size collection of items, while an ArrayList is a dynamic collection that can grow and shrink in size. You can use the `Remove` method on an ArrayList to remove items easily.
How do I remove multiple items from an array in PowerShell?
To remove multiple items, you can use the `Where-Object` cmdlet with a condition that excludes all items you want to remove. For example, `$array = $array | Where-Object {$_ -notin $itemsToRemove}`.
What happens to the original array when I remove an item?
The original array remains unchanged because arrays in PowerShell are immutable. You must assign the result of the removal operation to a new variable or the same variable to reflect the changes.
In summary, removing an item from an array in PowerShell can be accomplished using various methods, each suited for different scenarios. The most common techniques include using the `Where-Object` cmdlet to filter out unwanted elements, employing the `ArrayList` class for more dynamic array manipulations, and leveraging the `RemoveAt` method for specific index-based removals. Understanding these methods allows users to effectively manage and manipulate arrays within their scripts.
Key takeaways include the importance of recognizing the immutability of standard arrays in PowerShell. When an item is removed from a standard array, a new array is created, which may not be immediately intuitive for users accustomed to mutable data structures. Additionally, the `ArrayList` class provides a more flexible approach, allowing for efficient additions and removals without the overhead of creating new arrays.
Ultimately, the choice of method depends on the specific requirements of the task at hand, such as performance considerations and the need for dynamic array manipulation. By mastering these techniques, PowerShell users can enhance their scripting capabilities and streamline their workflows when handling array data structures.
Author Profile
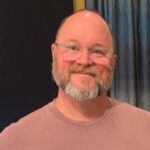
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?