How Can You Effectively Fix JavaScript Errors in Your Code?
How Do You Fix JavaScript Errors?
JavaScript is the backbone of modern web development, powering everything from interactive web applications to dynamic content updates. However, as any developer knows, the journey to a flawless user experience is often riddled with errors. Whether you’re a seasoned coder or a novice just starting out, encountering JavaScript errors can be frustrating and time-consuming. But fear not! Understanding how to effectively troubleshoot and fix these errors is a crucial skill that can elevate your coding prowess and enhance your projects.
In this article, we will explore the common types of JavaScript errors you might encounter, from syntax mistakes to runtime issues. We’ll delve into the tools and techniques available for diagnosing these problems, including the use of browser developer tools and debugging practices. By learning how to systematically identify and resolve errors, you will not only improve your coding efficiency but also gain confidence in your ability to tackle challenges head-on.
Moreover, we will discuss best practices for writing cleaner, more maintainable code that minimizes the risk of errors in the first place. With the right strategies in place, you can transform error handling from a daunting task into a straightforward process, allowing you to focus on what you do best: creating amazing web experiences. Join us as we unravel the mysteries of JavaScript errors
Common JavaScript Errors
JavaScript errors can arise from various sources, including syntax mistakes, runtime errors, and logical errors. Understanding the different types of errors is crucial for effective debugging. Here are some common JavaScript errors:
- Syntax Errors: These occur when the JavaScript engine encounters code that doesn’t conform to the language’s syntax rules. For example, forgetting a closing bracket or using an incorrect keyword can lead to syntax errors.
- Reference Errors: These happen when the code attempts to access a variable that has not been declared. For example, using a variable outside its scope can trigger this type of error.
- Type Errors: This type of error occurs when a value is not of the expected type. For instance, calling a method on an variable can result in a type error.
Using the Browser Console
The browser console is a powerful tool for debugging JavaScript. It allows developers to view error messages and trace issues in real-time. To access the console:
- Right-click on the webpage and select “Inspect” or “Inspect Element.”
- Navigate to the “Console” tab.
The console will display error messages with line numbers and descriptions, making it easier to identify the source of the error.
Debugging Techniques
Employing effective debugging techniques can help isolate and fix JavaScript errors. Here are some methods:
- Use Breakpoints: Set breakpoints in your code to pause execution and inspect the values of variables at specific points.
- Console Logging: Use `console.log()` to output variable values and track the flow of execution. This can help identify where things may be going wrong.
- Error Handling: Implement try-catch blocks to gracefully handle errors and provide informative messages.
Best Practices to Avoid Errors
Following best practices in JavaScript can minimize the occurrence of errors. Consider the following guidelines:
- Consistent Naming Conventions: Use clear and consistent naming conventions for variables and functions to enhance code readability.
- Modular Code: Break code into smaller, manageable functions or modules to isolate functionality and reduce complexity.
- Code Reviews: Regular code reviews can help catch potential errors before they become problematic.
Common Error Messages and Solutions
Understanding common error messages can expedite the debugging process. Below is a table summarizing typical error messages and their solutions:
Error Message | Possible Cause | Solution |
---|---|---|
Uncaught SyntaxError | Incorrect syntax in the code | Check for missing brackets or incorrect punctuation |
Uncaught ReferenceError | Using a variable that is not defined | Ensure the variable is declared and in scope |
Uncaught TypeError | Calling a function on a non-function object | Verify the object type before calling the method |
By implementing these strategies and understanding common errors, developers can effectively troubleshoot and enhance their JavaScript applications.
Common JavaScript Errors
JavaScript errors can generally be categorized into several types. Understanding these errors can significantly aid in troubleshooting.
- Syntax Errors: These occur when the code violates the language’s grammar rules.
- Runtime Errors: These happen during the execution of the script, often due to issues like variables or functions.
- Logical Errors: The code runs without crashing, but produces incorrect results due to flawed logic.
Utilizing Browser Developer Tools
Most modern browsers come equipped with developer tools that can help diagnose and fix JavaScript errors.
- Accessing Developer Tools:
- In Chrome, right-click on the page and select “Inspect” or press `Ctrl + Shift + I`.
- In Firefox, the process is similar; use `Ctrl + Shift + I`.
- Features to Use:
- Console: Displays errors and messages; use `console.log()` to debug.
- Debugger: Set breakpoints to pause code execution and inspect variables.
- Network Tab: Monitor requests and responses to identify issues with API calls.
Reading Error Messages
Error messages provide valuable information on what went wrong. Key components include:
Component | Description |
---|---|
Error Type | Indicates whether it’s a syntax, runtime, or logical error. |
File Name | Shows the file in which the error occurred. |
Line Number | Points to the specific line, facilitating quick navigation. |
Message | Provides a brief description of the error. |
Common Fixes for JavaScript Errors
Addressing errors often involves specific fixes depending on the type of error encountered.
- Syntax Errors:
- Check for missing semicolons, brackets, or parentheses.
- Use a linter to catch errors before runtime.
- Runtime Errors:
- Ensure all variables are defined before use.
- Validate data types and values before operations.
- Logical Errors:
- Utilize `console.log()` to track variable states and flow.
- Review algorithm steps to confirm they align with intended logic.
Testing and Debugging Strategies
Effective testing and debugging can prevent and resolve JavaScript errors. Consider the following strategies:
- Unit Testing: Write tests for individual functions to ensure they behave correctly.
- Integration Testing: Test how different modules work together.
- Error Handling: Implement `try…catch` blocks to gracefully handle exceptions.
Using Online Resources and Documentation
When in doubt, consult online resources and documentation for assistance. Recommended sources include:
- MDN Web Docs: Comprehensive documentation on JavaScript and web APIs.
- Stack Overflow: Community-driven Q&A platform for troubleshooting specific issues.
- JavaScript Console: Experiment with code snippets directly in the browser console to test hypotheses.
Best Practices to Avoid Errors
Adopting best practices can help minimize the occurrence of JavaScript errors:
- Consistent Code Style: Use a consistent naming convention and indentation.
- Modular Code: Break code into smaller, reusable functions.
- Version Control: Utilize version control systems (like Git) to track changes and revert if necessary.
Conclusion on Fixing JavaScript Errors
Addressing JavaScript errors involves a systematic approach to understanding error types, utilizing tools, and adopting best practices. This continuous learning process enhances coding proficiency and efficiency.
Expert Strategies for Resolving JavaScript Errors
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively fix JavaScript errors, developers should start by utilizing browser developer tools. These tools provide detailed error messages and stack traces, which are invaluable for pinpointing the source of the issue.”
Michael Thompson (Lead Frontend Developer, CodeCraft Solutions). “A systematic approach is essential. I recommend isolating the problematic code segment, testing it independently, and using console logging to track variable states. This method often reveals hidden issues that may not be immediately apparent.”
Sarah Lee (JavaScript Framework Specialist, DevPro Academy). “Understanding the common types of JavaScript errors, such as syntax errors and runtime errors, is crucial. By familiarizing oneself with these types, developers can quickly identify and resolve issues, leading to more efficient debugging.”
Frequently Asked Questions (FAQs)
What are common types of JavaScript errors?
Common types of JavaScript errors include syntax errors, runtime errors, and logical errors. Syntax errors occur when the code does not conform to the language rules, runtime errors happen during code execution, and logical errors produce incorrect results despite the code running without issues.
How can I identify JavaScript errors in my code?
You can identify JavaScript errors using browser developer tools, specifically the console tab, which displays error messages and their locations. Additionally, using debugging tools or adding `console.log()` statements in your code can help trace the execution flow and identify issues.
What steps should I take to fix a syntax error?
To fix a syntax error, carefully review the error message provided in the console, locate the indicated line of code, and check for common issues such as missing parentheses, brackets, or semicolons. Correct the identified mistakes and re-test the code.
How can I handle runtime errors effectively?
To handle runtime errors effectively, use try-catch blocks to catch exceptions and prevent the application from crashing. Additionally, logging error details can provide insights into what went wrong, allowing for more targeted fixes.
What tools can assist in debugging JavaScript errors?
Several tools can assist in debugging JavaScript errors, including browser developer tools (Chrome DevTools, Firefox Developer Edition), integrated development environments (IDEs) like Visual Studio Code, and debugging libraries such as Sentry and LogRocket.
How can I prevent logical errors in my JavaScript code?
To prevent logical errors, employ thorough testing practices, including unit tests and integration tests. Additionally, using clear variable names, writing modular code, and conducting code reviews can help identify potential issues before they arise.
In summary, fixing JavaScript errors requires a systematic approach that involves understanding the types of errors, utilizing debugging tools, and implementing best coding practices. JavaScript errors can be broadly categorized into syntax errors, runtime errors, and logical errors. Each type necessitates a different strategy for identification and resolution. Syntax errors are often caught during the coding process, while runtime errors may require deeper investigation through debugging tools and console logs.
Utilizing browser developer tools is essential for effectively diagnosing and fixing JavaScript errors. These tools provide insights into the code execution process, allowing developers to inspect elements, monitor network activity, and view console outputs. Additionally, leveraging debugging techniques such as breakpoints and step-through debugging can significantly enhance a developer’s ability to pinpoint issues in their code.
Moreover, adopting best practices in coding can help prevent errors from occurring in the first place. This includes writing clean, modular code, adhering to coding standards, and thoroughly testing code before deployment. Implementing error handling strategies, such as try-catch blocks, can also provide a safety net for managing unexpected issues during runtime.
effectively fixing JavaScript errors involves a combination of understanding the nature of the errors, utilizing the right tools for debugging, and
Author Profile
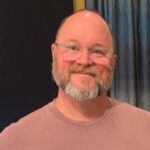
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?