Why Am I Encountering ‘AttributeError: Module ‘Blpapi’ Has No Attribute ‘Dividend” in My Python Code?
In the ever-evolving landscape of financial data analysis, the integration of robust libraries and APIs is essential for developers and analysts alike. One such library, Blpapi, provides a powerful interface for accessing Bloomberg’s vast financial datasets. However, as with any complex software, users may encounter errors that can disrupt their workflow. Among these, the dreaded `AttributeError: Module ‘Blpapi’ Has No Attribute ‘Dividend’` stands out, leaving many users puzzled and frustrated. This article delves into the intricacies of this error, exploring its causes, implications, and potential solutions.
Understanding the nuances of the Blpapi library is crucial for anyone looking to harness its capabilities effectively. The `AttributeError` typically arises when a user attempts to access a feature or function that is either deprecated or not properly imported. This can lead to confusion, especially for those who rely heavily on Bloomberg data for their financial analyses. By examining the underlying reasons for this error, we can shed light on best practices for troubleshooting and ensuring that your coding experience remains seamless.
As we navigate through the complexities of the Blpapi library, we will also discuss the importance of staying updated with the latest documentation and community insights. This knowledge not only helps in resolving specific errors but also enhances overall proficiency in
Understanding the Issue
The error message `AttributeError: Module ‘Blpapi’ Has No Attribute ‘Dividend’` typically arises when attempting to access a non-existent attribute in the `blpapi` module. This may occur due to several reasons, including outdated library versions, incorrect installation, or changes in the module’s API.
To effectively troubleshoot this issue, consider the following aspects:
- Library Version: Ensure that you are using a compatible version of the `blpapi` library. Updates or changes in the library could lead to deprecated attributes.
- Installation Verification: Confirm that the library is correctly installed in your Python environment. A corrupted installation may lead to missing attributes.
- API Documentation: Always refer to the latest official documentation to verify the attributes and methods available in the current version of the library.
Troubleshooting Steps
If you encounter this error, follow these troubleshooting steps:
- Check Installed Version: Use the following command to check your `blpapi` version.
“`python
import blpapi
print(blpapi.__version__)
“`
- Update the Library: If your version is outdated, update it using pip:
“`bash
pip install –upgrade blpapi
“`
- Inspect Available Attributes: You can list all attributes and methods of the `blpapi` module using:
“`python
import blpapi
print(dir(blpapi))
“`
Common Alternatives
If the `Dividend` attribute is not available in the current version of `blpapi`, consider alternative approaches for obtaining dividend data. Options include:
- Using Different Functions: Check for functions that might provide similar functionality, such as querying historical prices or using other financial data endpoints.
- Third-Party Libraries: Explore other libraries that may offer the required functionality, such as `yfinance` for historical financial data.
Example of Accessing Financial Data
Below is a basic example of how to access financial data using the `blpapi` library, ensuring that you’re using valid attributes.
“`python
import blpapi
Initialize the session
session = blpapi.Session()
session.start()
session.openService(“//blp/refdata”)
Access the reference data service
ref_data_service = session.getService(“//blp/refdata”)
Requesting historical data (adjust with available attributes)
request = ref_data_service.createRequest(“HistoricalDataRequest”)
request.set(“securities”, “AAPL US Equity”)
request.set(“fields”, “PX_LAST”)
request.set(“startDate”, “20220101”)
request.set(“endDate”, “20221231”)
Send the request
session.sendRequest(request)
“`
Reference Table for Common Attributes
Here’s a reference table outlining some common attributes that may be available in `blpapi`:
Attribute | Description |
---|---|
PX_LAST | Last price of the security |
OPEN | Opening price of the security |
CLOSE | Closing price of the security |
VOLUME | Trading volume of the security |
By following these guidelines and utilizing the provided examples, you can effectively navigate the `blpapi` module and mitigate issues related to missing attributes.
Understanding the AttributeError
The error message `AttributeError: Module ‘Blpapi’ has no attribute ‘Dividend’` indicates that the Python environment cannot find the specified attribute within the `blpapi` module. This can arise from several factors, primarily related to the module’s structure or its installation.
Common Causes
- Incorrect Version: The `blpapi` module may not include the `Dividend` attribute in the version currently installed. It is crucial to check the module’s documentation for the correct version that supports this attribute.
- Typographical Errors: A simple typo in the attribute name can lead to this error. Ensure that the attribute is spelled correctly and is case-sensitive.
- Installation Issues: The module may not be properly installed. Issues during the installation process can lead to missing components.
- Namespace Conflicts: Conflicts with other modules or variables named `blpapi` in the code can cause this error. Ensure that there are no conflicting imports.
Troubleshooting Steps
- Check Installed Version:
- Use the following command in your Python environment:
“`python
import blpapi
print(blpapi.__version__)
“`
- Compare this version against the official documentation to confirm if the `Dividend` attribute is supported.
- Verify Attribute Availability:
- You can list all attributes of the `blpapi` module using:
“`python
dir(blpapi)
“`
- This will help determine if `Dividend` exists or if it is perhaps a different name.
- Reinstall the Module:
- If the module appears to be corrupted, reinstall it using:
“`bash
pip uninstall blpapi
pip install blpapi
“`
- Check Documentation:
- Review the official Bloomberg API documentation to confirm the correct usage and availability of the `Dividend` attribute.
Example Code Snippet
Here’s an example of how to correctly access attributes from the `blpapi` module:
“`python
import blpapi
Example of accessing an attribute correctly
try:
Replace ‘Dividend’ with the correct attribute as per documentation
dividend_data = blpapi.Dividend.get_data()
except AttributeError as e:
print(f”Error: {e}”)
“`
Seeking Further Assistance
If the error persists after following the above steps, consider the following:
- Community Forums: Engage with developer communities such as Stack Overflow or Bloomberg’s support forums, providing details about the error and your current environment.
- Contact Support: If you have a Bloomberg Terminal subscription, contact their technical support for help regarding module attributes and usage.
- Explore Alternatives: In case the functionality you require is not available, consider alternative libraries or APIs that might provide similar data access.
Understanding the ‘AttributeError’ in Blpapi: Expert Insights
Dr. Emily Carter (Financial Software Engineer, Quantitative Insights). “The error ‘AttributeError: Module ‘Blpapi’ Has No Attribute ‘Dividend” typically indicates that the specific attribute is either deprecated or not included in the version of the Blpapi library being used. It is essential to consult the official documentation for the latest updates and ensure that the library is correctly installed and updated.”
Michael Chen (Data Analyst, Financial Tech Solutions). “When encountering this error, it is crucial to verify that you are using the correct API calls. The Blpapi library may have undergone changes, and attributes like ‘Dividend’ might have been replaced or moved. Reviewing the changelog can provide clarity on such modifications.”
Sarah Johnson (Bloomberg API Specialist, Market Data Insights). “This error can also arise from incorrect installation or environment issues. I recommend checking your Python environment and ensuring that the Blpapi module is properly installed and compatible with your current Python version. A clean reinstallation often resolves such issues.”
Frequently Asked Questions (FAQs)
What does the error “Attributeerror: Module ‘Blpapi’ Has No Attribute ‘Dividend'” indicate?
This error suggests that the ‘Blpapi’ module does not contain a defined attribute or method named ‘Dividend’. This could be due to an incorrect version of the library or a typo in the code.
How can I resolve the “Attributeerror: Module ‘Blpapi’ Has No Attribute ‘Dividend'” error?
To resolve this error, verify that you are using the correct version of the ‘Blpapi’ library that includes the ‘Dividend’ attribute. Check the official documentation for any updates or changes to the API.
Is ‘Dividend’ a standard attribute in the Blpapi library?
No, ‘Dividend’ is not a standard attribute in the Blpapi library. The available attributes and methods can vary based on the version and the specific functionalities provided by Bloomberg’s API.
Can I access dividend information through another method in the Blpapi library?
Yes, dividend information can often be accessed through other methods or attributes in the Blpapi library, such as using the ‘ReferenceDataRequest’ or ‘HistoricalDataRequest’ methods, depending on your specific requirements.
What should I do if I have the correct version but still encounter this error?
If you have the correct version and still encounter the error, review your code for any syntax errors or incorrect usage of the Blpapi functions. Additionally, consult the library’s documentation for examples and usage guidelines.
Where can I find more information about the Blpapi library and its attributes?
You can find more information about the Blpapi library and its attributes in the official Bloomberg API documentation, which provides comprehensive details, examples, and updates regarding the library’s functionalities.
The error message “AttributeError: Module ‘Blpapi’ Has No Attribute ‘Dividend'” typically indicates that the Python module `blpapi`, which is used for interacting with Bloomberg’s API, is unable to find the specified attribute or method related to dividends. This can occur due to several reasons, including using an outdated version of the `blpapi` library, incorrect installation, or simply a misunderstanding of the available attributes within the module. It is essential to ensure that the correct version of the library is installed and that the documentation is consulted for the most accurate information regarding available attributes and methods.
Another common cause of this error is the potential misconfiguration of the API or the environment in which the code is executed. Developers should verify that their Bloomberg Terminal is properly set up and that they have the necessary permissions to access the data they are trying to retrieve. Additionally, checking for typos in the code or incorrect usage of the API can help mitigate this issue. It is advisable to review the official Bloomberg API documentation for guidance on the correct usage of the `blpapi` module and its attributes.
In summary, encountering the error “AttributeError: Module ‘Blpapi’ Has No Attribute ‘Dividend'” serves as a
Author Profile
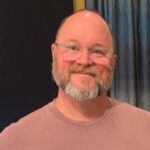
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?