How Can You Implement Sleep Functionality in JavaScript?
How To Sleep In JavaScript: A Guide to Managing Asynchronous Code
In the fast-paced world of web development, mastering asynchronous programming is essential for creating smooth, responsive applications. One common challenge developers face is managing time delays or pauses in code execution, often referred to as “sleeping.” While JavaScript doesn’t have a built-in sleep function like some other programming languages, there are effective techniques to achieve similar results. In this article, we will explore various methods to implement sleep functionality in JavaScript, allowing you to control the flow of your code with precision.
Understanding how to effectively pause execution can significantly enhance your ability to handle asynchronous tasks, such as API calls, animations, or timed events. We’ll delve into the nuances of using promises and async/await syntax to create delays in a way that keeps your code clean and readable. Additionally, we’ll discuss the implications of using timers and how they can impact your application’s performance, ensuring you make informed decisions when implementing sleep functionality.
Whether you’re a seasoned developer looking to refine your skills or a newcomer eager to learn the ropes of JavaScript, mastering the concept of sleeping in your code will empower you to build more dynamic and user-friendly applications. Get ready to unlock the secrets of asynchronous programming and take your JavaScript capabilities to the next
Understanding the Set Timeout Function
The `setTimeout` function is a fundamental method in JavaScript that allows you to execute a specified function after a defined delay. This function takes two primary arguments: the function you wish to execute and the delay duration in milliseconds. It is crucial to understand that `setTimeout` does not pause the execution of the code; it merely schedules a function to run after the specified time.
Example of using `setTimeout`:
“`javascript
console.log(“Start”);
setTimeout(() => {
console.log(“Executed after 2 seconds”);
}, 2000);
console.log(“End”);
“`
In the example above, “Start” is logged immediately, then “End” is logged before the message from the `setTimeout`, which appears after a 2-second delay.
Using Promises for Asynchronous Sleep
To create a more modern and cleaner approach for sleeping in JavaScript, you can utilize Promises combined with `async/await`. This technique allows you to pause execution in an asynchronous function.
Here’s how you can implement a sleep function using Promises:
“`javascript
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function demoSleep() {
console.log(“Sleeping for 3 seconds…”);
await sleep(3000);
console.log(“Awake!”);
}
demoSleep();
“`
In this example, `sleep` returns a Promise that resolves after the specified milliseconds, allowing `demoSleep` to pause execution until the Promise is resolved.
Comparison of Sleep Techniques
The following table illustrates the differences between the traditional `setTimeout` and the `Promise`-based sleep method.
Feature | setTimeout | Promise-based Sleep |
---|---|---|
Syntax | setTimeout(func, delay) | await sleep(ms) |
Blocking | Non-blocking | Non-blocking (with async/await) |
Readability | Less readable | More readable |
Return Value | ID of the timer | Promise |
Utilizing the Promise-based method enhances code readability and maintainability, particularly in complex asynchronous workflows.
Considerations When Using Sleep
When implementing sleep functionality in your applications, consider the following:
- Performance: Excessive use of sleep can lead to performance issues, especially in UI-heavy applications.
- User Experience: Avoid blocking the main thread for extended periods, as this can lead to a poor user experience.
- Functionality: Use sleep judiciously, primarily for delaying operations in a controlled manner, such as animations or timed operations.
By understanding these techniques and their implications, developers can effectively manage asynchronous tasks in JavaScript.
Understanding the Concept of Sleep in JavaScript
In JavaScript, the concept of “sleep” is not natively supported as it is in some other programming languages. However, developers often seek a way to pause execution temporarily, particularly in asynchronous code. This is typically achieved using `setTimeout`, Promises, or async/await syntax.
Using setTimeout for Delayed Execution
The `setTimeout` function allows you to execute a block of code after a specified delay. While it does not pause execution, it schedules code to run after a set period.
“`javascript
console.log(“Start”);
setTimeout(() => {
console.log(“Executed after 2 seconds”);
}, 2000);
console.log(“End”);
“`
In this example:
- The first console log (“Start”) is executed immediately.
- After 2 seconds, the second console log (“Executed after 2 seconds”) runs.
- The third console log (“End”) runs right after “Start”.
Implementing Sleep with Promises
To create a sleep-like function using Promises, you can define a function that returns a Promise which resolves after a specified timeout:
“`javascript
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
// Usage
async function demo() {
console.log(“Sleeping for 2 seconds”);
await sleep(2000);
console.log(“Awake now!”);
}
demo();
“`
This code allows you to pause execution in an asynchronous function, providing a more intuitive flow, especially in complex workflows.
Using Async/Await for Cleaner Code
Combining async/await with the sleep function leads to cleaner and more readable code. The `async` keyword is used to declare an asynchronous function, while `await` pauses the execution of the function until the Promise resolves.
“`javascript
async function runTasks() {
console.log(“Task 1”);
await sleep(1000);
console.log(“Task 2 after 1 second”);
await sleep(1500);
console.log(“Task 3 after additional 1.5 seconds”);
}
runTasks();
“`
This allows for a linear execution flow that is easy to follow.
Performance Considerations
When implementing sleep-like functionality in JavaScript, consider the following:
- Event Loop Impact: Sleeping does not block the main thread but can delay subsequent operations, affecting user experience.
- Long Sleeps: Avoid excessively long sleep times, as they can lead to unresponsive applications.
- Use Cases: Ideal for animations, polling, and waiting for asynchronous operations rather than for heavy computation.
Common Pitfalls
- Blocking the Main Thread: Using a synchronous sleep (e.g., while loop) to simulate sleep will block the main thread, causing the UI to freeze.
- Error Handling: Ensure proper error handling when using async/await to manage potential rejections in Promises.
Issue | Description | Solution |
---|---|---|
UI Freezing | Using synchronous sleep can freeze the UI. | Use async/await with Promises. |
Unhandled Promise Rejection | Failing to catch errors in async functions. | Implement try/catch blocks. |
By understanding these concepts, developers can effectively manage timing and execution flow in JavaScript applications.
Expert Insights on Implementing Sleep in JavaScript
Dr. Emily Carter (Senior Software Engineer, Code Innovations). “In JavaScript, the concept of sleeping or pausing execution can be effectively managed using asynchronous programming techniques. Utilizing Promises and async/await syntax allows developers to create non-blocking code that enhances user experience without freezing the main thread.”
James Liu (JavaScript Framework Specialist, Tech Solutions Inc.). “Implementing a sleep function in JavaScript can be achieved through setTimeout or by creating a custom sleep function using Promises. This approach is essential for controlling the flow of asynchronous operations, especially in scenarios where timing is critical.”
Maria Gonzalez (Front-End Developer, Creative Web Agency). “While JavaScript does not have a built-in sleep function like some other languages, developers can simulate this behavior using async/await with a Promise-based delay. This technique is particularly useful in scenarios such as animations or when waiting for user inputs.”
Frequently Asked Questions (FAQs)
What does “sleep” mean in JavaScript?
In JavaScript, “sleep” refers to pausing the execution of code for a specified duration, allowing for asynchronous operations without blocking the main thread.
How can I implement a sleep function in JavaScript?
You can implement a sleep function using `setTimeout` or by creating a Promise that resolves after a specified time, allowing you to use `async/await` for a more readable approach.
Is there a built-in sleep function in JavaScript?
JavaScript does not have a built-in sleep function. Developers typically create custom sleep functions using `setTimeout` or Promises to achieve similar behavior.
Can sleep be used in asynchronous functions?
Yes, sleep can be effectively used in asynchronous functions. By using `async/await` with a custom sleep function, you can pause execution without blocking the event loop.
What is the impact of using sleep on performance?
Using sleep can impact performance if overused, as it may lead to delays in code execution. It is essential to use it judiciously to maintain responsiveness in applications.
Are there alternatives to sleep in JavaScript?
Alternatives to sleep include using `setTimeout` for callbacks, Promises for chaining asynchronous operations, or leveraging libraries like `rxjs` for more complex timing and scheduling needs.
In JavaScript, the concept of “sleeping” refers to pausing the execution of code for a specified duration. Unlike some other programming languages that have built-in sleep functions, JavaScript relies on asynchronous programming techniques to achieve similar functionality. The most common methods to simulate sleep in JavaScript involve using `setTimeout` or `Promises` with `async/await` syntax. These methods allow developers to create delays in code execution without blocking the main thread, which is crucial for maintaining a responsive user interface.
One of the key takeaways is the importance of understanding the non-blocking nature of JavaScript. By using `setTimeout`, developers can schedule a function to run after a specified delay, effectively creating a pause in execution. However, this does not halt the execution of the entire script, allowing other operations to continue running. On the other hand, the `async/await` approach provides a more readable and structured way to handle asynchronous code, making it easier to implement sleep-like behavior in modern JavaScript applications.
Additionally, it is essential to consider the context in which sleeping is applied. While it can be useful in scenarios such as animations, API call retries, or timed events, excessive use of sleep-like functions can
Author Profile
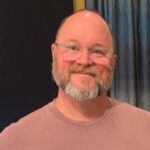
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?