How Can You Use SQL CASE in a WHERE Clause with an IN Statement?
In the world of relational databases, SQL (Structured Query Language) reigns supreme as the go-to tool for managing and manipulating data. As developers and data analysts delve deeper into the intricacies of SQL, they often encounter scenarios that require a nuanced approach to querying data. One such scenario involves the use of the `CASE` statement within a `WHERE` clause, particularly when combined with the `IN` statement. This powerful combination allows for more dynamic and conditional filtering of data, enabling users to craft sophisticated queries that can adapt to varying requirements.
Understanding how to effectively utilize `CASE` in conjunction with `IN` can significantly enhance the flexibility of your SQL queries. This approach not only streamlines the process of conditional logic but also opens up new avenues for data analysis. By incorporating these elements, you can create queries that respond intelligently to different criteria, making your data retrieval more efficient and tailored to specific needs. Whether you’re looking to categorize data on the fly or filter results based on multiple conditions, mastering this technique is essential for any SQL practitioner.
As we delve deeper into this topic, we will explore practical examples and best practices for implementing `CASE` statements within `WHERE` clauses, particularly when paired with the `IN` statement. This exploration will provide you with the tools
Using SQL CASE in WHERE Clause with IN Statement
The SQL `CASE` statement is a powerful tool that allows for conditional logic within SQL queries. When combined with the `WHERE` clause and the `IN` statement, it can significantly enhance query flexibility by enabling complex filtering conditions.
You can use the `CASE` statement to evaluate conditions and return specific values, which can then be utilized in the `WHERE` clause. This approach is particularly useful when dealing with varying criteria for filtering results based on different columns or values.
For example, the following SQL query demonstrates how to use the `CASE` statement within a `WHERE` clause that includes an `IN` condition:
“`sql
SELECT *
FROM Employees
WHERE DepartmentID IN (
CASE
WHEN JobTitle = ‘Manager’ THEN (1, 2)
WHEN JobTitle = ‘Engineer’ THEN (3, 4)
ELSE (5, 6)
END
);
“`
In this example, the `WHERE` clause filters employees based on their `DepartmentID`, which is determined by their `JobTitle`. Depending on the `JobTitle`, different sets of `DepartmentID`s are specified.
It’s important to note that the syntax may vary depending on the SQL database system you are using. Some databases, like SQL Server, support direct use of `CASE` in the `IN` clause, while others may require a different approach, such as using a subquery.
Alternative Approach Using Subqueries
If the direct approach using `CASE` in the `IN` clause is not supported, you can achieve similar results through subqueries. This method involves creating a separate query that computes the desired values before using them in the main query. Here’s how you can structure it:
“`sql
SELECT *
FROM Employees
WHERE DepartmentID IN (
SELECT DepartmentID
FROM Departments
WHERE DepartmentID IN (
SELECT CASE
WHEN JobTitle = ‘Manager’ THEN DepartmentID
WHEN JobTitle = ‘Engineer’ THEN DepartmentID
ELSE NULL
END
FROM Employees
)
);
“`
In this structure:
- The inner `SELECT CASE` statement returns `DepartmentID`s based on the `JobTitle`.
- The outer query then uses these results to filter employees.
Considerations When Using CASE with IN
When implementing the `CASE` statement within the `WHERE` clause, keep the following considerations in mind:
- Performance: Complex queries can lead to performance degradation. Always analyze query execution plans.
- Readability: While powerful, overusing `CASE` can make SQL statements difficult to read. Aim for clarity.
- Null Handling: Be cautious with null values, as they can affect the outcome of `IN` comparisons.
- Database Compatibility: Ensure that your SQL syntax is compatible with the specific database system.
Job Title | Department IDs |
---|---|
Manager | 1, 2 |
Engineer | 3, 4 |
Other | 5, 6 |
Utilizing the `CASE` statement effectively in conjunction with the `WHERE` clause and `IN` statement can lead to more dynamic and efficient SQL queries, allowing for tailored data retrieval based on varying conditions.
Understanding SQL CASE in WHERE Clause
The SQL `CASE` statement allows for conditional logic within SQL queries. When used in a `WHERE` clause, it provides the ability to create complex filtering criteria based on multiple conditions.
Syntax of CASE in WHERE Clause
The basic syntax of using `CASE` within a `WHERE` clause follows this structure:
“`sql
SELECT column1, column2
FROM table_name
WHERE
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE result_default
END IN (value1, value2, …);
“`
Practical Use Cases
Using `CASE` within a `WHERE` clause can be particularly useful in scenarios where the filtering criteria depend on varying conditions. Here are some examples:
– **Filtering based on categorical data**: Apply different filters based on the category of the data.
– **Conditional logic for date ranges**: Select records from specific date ranges based on varying conditions.
Example Scenarios
Consider a `sales` table containing `salesperson`, `region`, and `sales_amount`. You can filter based on the region and specific conditions for the sales amount:
“`sql
SELECT salesperson, sales_amount
FROM sales
WHERE
CASE
WHEN region = ‘North’ THEN sales_amount
WHEN region = ‘South’ THEN sales_amount
ELSE NULL
END IN (1000, 2000);
“`
This query retrieves `salesperson` records whose sales amounts match either 1000 or 2000, but only for the North and South regions.
Combining CASE with IN Statement
The `IN` statement can also be combined with `CASE` for more granular control. This approach ensures that specific conditions match defined criteria for filtering:
“`sql
SELECT salesperson, region
FROM sales
WHERE
salesperson_id IN (
SELECT salesperson_id
FROM sales
WHERE
CASE
WHEN region = ‘East’ AND sales_amount > 1500 THEN ‘Valid’
WHEN region = ‘West’ AND sales_amount < 500 THEN 'Valid'
ELSE 'Invalid'
END = 'Valid'
);
```
In this case, the inner `CASE` statement filters `salesperson_id` based on conditions for different regions, allowing the outer query to select valid entries.
Considerations
- Performance: Using `CASE` in the `WHERE` clause can impact query performance. Always test for efficiency.
- Readability: Complex `CASE` statements can reduce the readability of queries. Consider using comments or breaking down complex logic into simpler components.
- Database Compatibility: Ensure that the SQL syntax is compatible with the specific database system being used, as implementations may vary.
Conclusion
Integrating `CASE` within a `WHERE` clause provides flexibility and power in SQL queries, allowing for sophisticated data filtering based on multiple conditions. By understanding the syntax and practical applications, you can enhance your SQL querying capabilities significantly.
Expert Insights on Using SQL Case in Where Clause with In Statement
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Utilizing a SQL CASE statement within a WHERE clause combined with an IN statement can significantly enhance query flexibility. It allows for conditional logic that can simplify complex filtering scenarios, making it easier to manage data retrieval based on varying criteria.”
Michael Tran (Senior Data Analyst, Data Insights Group). “Incorporating a CASE statement in conjunction with an IN clause can optimize performance by reducing the need for multiple queries. This approach can streamline the logic, allowing for more efficient execution of SQL statements, especially in large datasets.”
Lisa Patel (SQL Developer, Cloud Solutions Ltd.). “The combination of CASE and IN in a WHERE clause is particularly useful for creating dynamic filters based on user input. This technique not only enhances the readability of the SQL code but also improves maintainability, as changes to filtering logic can be managed within a single statement.”
Frequently Asked Questions (FAQs)
What is the purpose of using SQL CASE in a WHERE clause?
The SQL CASE statement allows for conditional logic within a query, enabling different criteria to be applied in the WHERE clause based on specific conditions.
Can I use the SQL CASE statement with an IN clause?
Yes, you can use the SQL CASE statement in conjunction with the IN clause to dynamically evaluate multiple conditions and filter results based on those evaluations.
How do I structure a SQL query with CASE in the WHERE clause using IN?
To structure such a query, you can use a syntax like: `WHERE column_name IN (CASE WHEN condition1 THEN value1 WHEN condition2 THEN value2 END)`. This allows for conditional evaluation of the column values.
Are there performance considerations when using CASE in a WHERE clause?
Yes, using CASE in a WHERE clause can impact performance, especially if the dataset is large. It is advisable to analyze the execution plan and consider indexing strategies to optimize query performance.
Can I combine multiple conditions in a CASE statement within a WHERE clause?
Yes, you can combine multiple conditions using logical operators (AND, OR) within the CASE statement to create more complex filtering criteria in the WHERE clause.
What are some common use cases for using SQL CASE in a WHERE clause with IN?
Common use cases include dynamically filtering results based on user input, applying different business rules for data retrieval, and implementing role-based access controls in data queries.
The use of the SQL CASE statement within a WHERE clause, particularly in conjunction with the IN statement, serves as a powerful tool for conditional filtering of query results. This approach allows developers to implement complex logic directly in their SQL queries, enabling them to evaluate multiple conditions and return specific values based on those evaluations. By integrating CASE with IN, users can streamline their queries, making them more efficient and easier to read while still achieving the desired outcome based on varying conditions.
One of the key advantages of utilizing a CASE statement in the WHERE clause is its flexibility. It allows for dynamic comparisons and can simplify queries that would otherwise require multiple OR conditions. This not only improves the clarity of the SQL code but also enhances maintainability, as changes to the logic can be made in one place rather than scattered throughout the query. Furthermore, using CASE with IN can lead to more concise code, reducing redundancy and the potential for errors.
leveraging the SQL CASE statement within a WHERE clause in combination with the IN statement is an effective strategy for executing conditional logic in queries. It empowers developers to craft more sophisticated and readable SQL statements, ultimately leading to improved performance and easier maintenance of database interactions. As SQL continues to evolve, mastering such techniques will be essential
Author Profile
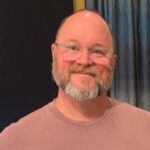
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?