How Can You Effectively Implement A/B Testing in Python?
In the fast-paced world of digital marketing and product development, making informed decisions is crucial to success. Enter A/B testing, a powerful method that allows businesses to compare two versions of a webpage, app feature, or marketing campaign to determine which one performs better. With the rise of data-driven strategies, mastering A/B testing is no longer just an option; it’s a necessity. This article will delve into the intricacies of A/B testing in Python, equipping you with the knowledge and tools to harness this technique effectively.
A/B testing, also known as split testing, is a systematic approach that enables teams to optimize their offerings by analyzing user behavior and preferences. By presenting two variations to different segments of users and measuring their responses, businesses can gain valuable insights into what resonates with their audience. Python, with its robust libraries and user-friendly syntax, provides an ideal platform for implementing A/B testing, making it accessible for both seasoned developers and newcomers alike.
In this article, we will explore the fundamental concepts of A/B testing, the importance of statistical significance, and how to leverage Python’s capabilities to streamline the process. Whether you’re looking to enhance user engagement, boost conversion rates, or refine your product features, understanding A/B testing in Python will empower you to make data
A/B Testing Frameworks in Python
When implementing A/B testing in Python, several frameworks can simplify the process, allowing for efficient design, execution, and analysis of experiments. Popular libraries include:
- SciPy: Useful for statistical tests and data analysis.
- Statsmodels: Provides classes and functions for estimating statistical models and conducting hypothesis tests.
- PyAB: Specifically designed for A/B testing, offering a simple interface to run tests and analyze results.
Setting Up an A/B Test
Setting up an A/B test requires careful planning and execution. The following steps outline the process:
- Define Goals: Establish what you want to achieve (e.g., increase conversion rates).
- Select Variables: Identify the elements to test (e.g., button color, layout).
- Segment Audience: Randomly divide your audience into control (A) and test (B) groups.
- Run the Experiment: Implement the changes for the test group while keeping the control group unchanged.
- Collect Data: Monitor user interactions and gather data for analysis.
Statistical Analysis of A/B Test Results
After running the A/B test, analyzing the results is crucial to determine if the changes led to significant improvements. Common statistical measures include:
- Conversion Rate: The percentage of users who completed the desired action.
- P-Value: Indicates the probability of observing the results if the null hypothesis is true.
- Confidence Interval: A range of values that likely contains the population parameter.
To interpret the results, use the following table:
Metric | Control Group (A) | Test Group (B) |
---|---|---|
Conversion Rate | 5% | 7% |
P-Value | – | 0.03 |
Confidence Interval | – | [0.01, 0.05] |
This table summarizes the key metrics of the experiment, allowing for a straightforward comparison between groups.
Common Pitfalls in A/B Testing
While A/B testing can provide valuable insights, certain pitfalls should be avoided:
- Insufficient Sample Size: Running tests with too few participants can lead to inconclusive results.
- Testing Too Many Variants: Multiple simultaneous tests can cause confusion and dilute the results.
- Ignoring External Factors: Changes in user behavior due to seasonality or marketing campaigns can skew results.
- Lack of Clear Hypothesis: Without a well-defined hypothesis, it becomes difficult to interpret the results meaningfully.
By following best practices and avoiding common mistakes, you can maximize the effectiveness of your A/B testing efforts in Python.
A/B Testing in Python: Concepts and Tools
A/B testing, also known as split testing, is a method used to compare two versions of a webpage or product to determine which one performs better. In Python, several libraries facilitate A/B testing, making it easier to implement and analyze the results.
Key Libraries for A/B Testing in Python
- SciPy: Useful for statistical tests, such as t-tests, which are critical in determining the significance of differences observed in A/B tests.
- Statsmodels: Offers a wide array of statistical models and tests, including linear regression and hypothesis tests that are essential for A/B testing analysis.
- Pandas: Provides data manipulation and analysis tools, allowing for efficient handling of datasets generated from A/B tests.
- Matplotlib/Seaborn: Visualization libraries that help in plotting results, making it easier to interpret and communicate findings.
Setting Up an A/B Test in Python
To set up an A/B test, follow these steps:
- Define the Objective: Clearly specify what you are testing, such as conversion rates, click-through rates, or user engagement.
- Randomly Assign Users: Split your audience randomly into two groups:
- Group A (Control): Sees the original version.
- Group B (Variant): Sees the modified version.
- Collect Data: Track relevant metrics, such as clicks, conversions, or other user interactions.
- Analyze Results: Use statistical methods to compare the performance of both groups.
Example Code for A/B Testing
The following code snippet demonstrates a basic A/B test using Python:
“`python
import numpy as np
import pandas as pd
from scipy import stats
Simulated data
np.random.seed(0)
control = np.random.binomial(n=1, p=0.1, size=1000)
variant = np.random.binomial(n=1, p=0.12, size=1000)
Create a DataFrame
data = pd.DataFrame({
‘Control’: control,
‘Variant’: variant
})
Calculate conversion rates
conversion_rates = data.mean()
print(conversion_rates)
Perform a t-test
t_stat, p_value = stats.ttest_ind(data[‘Control’], data[‘Variant’])
print(f’T-statistic: {t_stat}, P-value: {p_value}’)
“`
This code simulates user interactions for both control and variant groups, calculates conversion rates, and performs a t-test to determine if the differences are statistically significant.
Interpreting A/B Test Results
After conducting an A/B test, it is crucial to interpret the results accurately. The following metrics are vital:
- Conversion Rate: Measures the percentage of users who took the desired action.
Group | Conversions | Total Users | Conversion Rate |
---|---|---|---|
Control | 100 | 1000 | 10% |
Variant | 120 | 1000 | 12% |
- P-Value: Indicates the probability that the observed results occurred by chance. A p-value less than 0.05 typically suggests statistical significance.
- Confidence Intervals: Provide a range of values within which the true conversion rate likely falls.
Best Practices for A/B Testing
- Test One Variable at a Time: Isolate the impact of each change.
- Ensure Adequate Sample Size: Larger samples yield more reliable results.
- Run Tests for Sufficient Duration: Avoid premature conclusions by allowing tests to run long enough to capture variability.
- Monitor External Factors: Be aware of any external influences that might affect the results.
By adhering to these practices, A/B testing can yield meaningful insights into user behavior and preferences, driving informed decision-making in product development and marketing strategies.
Expert Insights on A/B Testing in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “A/B testing in Python allows for robust statistical analysis and the ability to handle large datasets efficiently. Utilizing libraries such as SciPy and StatsModels can significantly enhance the accuracy of your test results, enabling data-driven decision-making.”
Mark Thompson (Senior Software Engineer, Analytics Solutions Group). “Implementing A/B testing in Python is not just about running tests; it’s crucial to understand the underlying principles of experimental design. Using frameworks like Flask or Django for web applications can streamline the process of serving different variants to users.”
Lisa Nguyen (Marketing Analyst, Digital Strategies Corp.). “Incorporating A/B testing into your marketing strategy using Python can lead to significant improvements in conversion rates. Leveraging libraries such as Pandas for data manipulation and visualization tools like Matplotlib can provide clear insights into user behavior.”
Frequently Asked Questions (FAQs)
What is A/B testing in Python?
A/B testing in Python is a statistical method used to compare two versions of a variable to determine which one performs better. It involves splitting users into two groups, exposing each group to a different version, and analyzing the results to identify the more effective option.
How do I implement A/B testing in Python?
To implement A/B testing in Python, you can use libraries such as `scipy` for statistical analysis and `pandas` for data manipulation. You would typically define your hypotheses, collect data, perform the test, and analyze the results using appropriate statistical tests like t-tests or chi-squared tests.
What libraries are commonly used for A/B testing in Python?
Common libraries for A/B testing in Python include `pandas` for data handling, `scipy` for statistical functions, `statsmodels` for hypothesis testing, and `matplotlib` or `seaborn` for data visualization.
How do I interpret the results of an A/B test?
Interpreting A/B test results involves analyzing metrics such as conversion rates, statistical significance (p-values), and confidence intervals. A statistically significant result indicates that the observed difference is unlikely to have occurred by chance, guiding decision-making.
What are common pitfalls to avoid in A/B testing?
Common pitfalls include insufficient sample size, running tests for too short a duration, not defining clear objectives, and failing to account for external factors that may influence results. These issues can lead to inaccurate conclusions.
Can A/B testing be automated in Python?
Yes, A/B testing can be automated in Python using frameworks like `Airflow` for scheduling tests and `Flask` or `Django` for web applications. Automation can streamline the process of data collection, analysis, and reporting, enhancing efficiency and accuracy.
A/B testing in Python is a powerful method for comparing two versions of a variable to determine which one performs better. This technique is widely used in various fields, including marketing, product development, and user experience design. By leveraging Python’s robust libraries and frameworks, practitioners can efficiently implement A/B tests, analyze results, and draw meaningful conclusions from their data. The process typically involves defining a hypothesis, segmenting the audience, and using statistical methods to evaluate the performance of the variants.
One of the key insights from the discussion on A/B testing in Python is the importance of proper experimental design. A well-structured A/B test ensures that the results are statistically significant and not influenced by external factors. Utilizing libraries such as SciPy, Statsmodels, or even dedicated A/B testing frameworks like PyAB can streamline the analysis process. Additionally, understanding the metrics that matter, such as conversion rates or user engagement, is crucial for interpreting the results accurately.
Another takeaway is the necessity of continuous iteration and learning. A/B testing is not a one-time event but rather a cycle of testing, learning, and optimizing. By systematically running tests and analyzing their outcomes, organizations can make informed decisions that enhance user experience and drive business growth. Furthermore, documenting
Author Profile
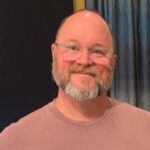
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?