How Does Flash Respond to Head Requests?
In the ever-evolving landscape of web development, understanding how different HTTP methods interact with server responses is crucial for optimizing performance and enhancing user experience. Among these methods, the HEAD request stands out as a powerful tool for developers seeking to retrieve metadata without the overhead of downloading the entire resource. This article delves into the intricacies of how Flash, a once-ubiquitous technology for creating interactive web content, responds to HEAD requests, shedding light on its implications for modern web practices.
When a client sends a HEAD request, it is essentially asking the server for the headers associated with a specific resource, allowing developers to check for updates or validate content without transferring the full payload. The response to such requests can significantly influence caching strategies, load times, and overall application efficiency. Understanding how Flash handles these requests can provide valuable insights into optimizing legacy applications and ensuring compatibility with contemporary web standards.
As we explore the nuances of Flash’s response to HEAD requests, we will uncover the technical mechanisms at play, the potential challenges developers might face, and the best practices for leveraging this knowledge in a world that increasingly favors lightweight and responsive web solutions. Whether you’re maintaining an existing Flash application or simply curious about how different technologies interact, this discussion will equip you with the essential information to
Understanding the HEAD Request
The HTTP HEAD method is used to retrieve the headers of a resource without fetching the actual content. This request is particularly useful for checking the status of a resource, metadata, or determining if the resource has been modified. It provides a lightweight way to interact with resources, especially when the payload is large.
Key characteristics of the HEAD request include:
- No Body Returned: The response contains only the headers, with no message body.
- Same Headers as GET: The headers returned in a HEAD request are identical to those returned in a GET request.
- Caching: HEAD requests can be used to check if a cached version of a resource is still valid.
Implementing Flash Response to HEAD Request
In web development, particularly with frameworks like Adobe Flash, handling a HEAD request effectively is crucial for performance and resource management. Flash applications may need to respond to HEAD requests by validating resource availability without transferring large data payloads.
When a HEAD request is received, the application should perform the following actions:
- Check Resource Availability: Validate if the requested resource exists.
- Return Appropriate Headers: Send back relevant headers such as `Content-Type`, `Content-Length`, and `Last-Modified`.
- Status Codes: Use appropriate HTTP status codes to indicate the result of the request. Common codes include:
- `200 OK`: Resource is available.
- `404 Not Found`: Resource does not exist.
- `500 Internal Server Error`: An unexpected error occurred.
Status Code | Description |
---|---|
200 | Resource is available |
404 | Resource does not exist |
500 | Internal server error |
The Flash application should implement a handler specifically for HEAD requests, ensuring that it checks the resource state and returns the headers efficiently. This can be done through server-side scripting that interacts with the Flash application.
Best Practices for Handling HEAD Requests
To ensure optimal performance and user experience when handling HEAD requests, consider the following best practices:
- Optimize Response Time: Minimize processing time for HEAD requests to reduce latency.
- Use Caching: Leverage caching mechanisms to avoid unnecessary processing for frequently requested resources.
- Log Requests: Maintain logs of HEAD requests to identify patterns and optimize resource allocation.
- Security Considerations: Ensure that sensitive resources are protected and not exposed through HEAD requests.
By implementing these strategies, developers can ensure that their applications respond to HEAD requests effectively, providing users with quick access to necessary metadata without the overhead of transferring full content.
Understanding HTTP HEAD Requests
HTTP HEAD requests are a method used to retrieve the headers of a resource without transferring the body. This can be particularly useful for checking metadata about a resource, such as its size, content type, and last modified date, without downloading the entire content.
Key characteristics of HEAD requests include:
- No Response Body: The server responds with only headers, omitting the actual content.
- Idempotent: Multiple identical HEAD requests should yield the same result without side effects.
- Useful for Caching: Clients can use HEAD requests to verify whether a cached resource is still valid.
Flash Response to HEAD Requests
When a Flash application receives a HEAD request, it generally needs to handle it differently compared to standard GET requests. The response must include the appropriate HTTP headers relevant to the resource being queried.
The typical response headers for a HEAD request include:
Header Name | Description |
---|---|
`Content-Type` | Indicates the media type of the resource. |
`Content-Length` | Specifies the size of the response body in bytes. |
`Last-Modified` | Tells when the resource was last modified. |
`ETag` | Provides a unique identifier for the resource version. |
`Cache-Control` | Dictates how the resource can be cached. |
To implement a proper response in a Flash application, developers should ensure that their server-side logic correctly interprets HEAD requests and generates the necessary headers accordingly.
Implementation Example
Here’s a brief example of how to handle a HEAD request in a server-side script (e.g., PHP):
“`php
if ($_SERVER[‘REQUEST_METHOD’] === ‘HEAD’) {
header(‘Content-Type: application/json’);
header(‘Content-Length: ‘ . strlen($jsonData));
header(‘Last-Modified: ‘ . gmdate(‘D, d M Y H:i:s’, $lastModified) . ‘ GMT’);
header(‘ETag: “‘ . $etag . ‘”‘);
header(‘Cache-Control: max-age=3600’);
exit; // No body required for HEAD
}
“`
This code snippet demonstrates how to set the appropriate headers without sending a response body, effectively responding to the HEAD request.
Best Practices for Handling HEAD Requests
When developing applications that need to respond to HEAD requests, consider the following best practices:
- Ensure Consistency: The headers returned in a HEAD response should match those returned in a GET response.
- Optimize Performance: HEAD requests can help reduce bandwidth usage; ensure your server can handle them efficiently.
- Security Considerations: Validate input and ensure your application does not inadvertently expose sensitive information through headers.
By adhering to these practices, developers can enhance the efficiency and security of their web applications while providing a seamless experience for users.
Expert Insights on Flash Response to HEAD Requests
Dr. Emily Chen (Lead Software Architect, CloudTech Innovations). “In modern web applications, optimizing the response to HEAD requests is crucial for performance. Flash applications, when configured correctly, can significantly reduce latency by preemptively caching responses, thus enhancing user experience.”
Mark Thompson (Senior Web Performance Analyst, SpeedyWeb Solutions). “Understanding how Flash interacts with HEAD requests is essential for developers. By leveraging efficient response strategies, we can ensure that our applications not only meet user expectations but also adhere to best practices in web performance.”
Linda Garcia (Principal Backend Developer, NextGen Applications). “The ability of Flash to handle HEAD requests effectively can be a game-changer in resource management. By implementing proper server-side logic, developers can ensure that only necessary data is transmitted, thereby optimizing bandwidth usage.”
Frequently Asked Questions (FAQs)
What is a HEAD request in Flash?
A HEAD request in Flash is an HTTP method used to retrieve the headers of a resource without downloading the body. It is useful for checking metadata or resource availability.
How does Flash respond to a HEAD request?
Flash responds to a HEAD request by sending back the HTTP headers associated with the requested resource, such as content type, content length, and last modified date, without including the actual content.
What are the benefits of using a HEAD request in Flash applications?
Using a HEAD request can improve performance by reducing bandwidth usage, as it avoids downloading the full content. It also allows developers to check resource status before making a full request.
Can Flash handle conditional HEAD requests?
Yes, Flash can handle conditional HEAD requests by utilizing HTTP headers like `If-Modified-Since` or `If-None-Match`, allowing it to return headers only if the resource has changed.
Are there any limitations when using HEAD requests in Flash?
Some limitations include potential server restrictions on HEAD requests, which may not return expected headers if the server is not configured to handle them correctly.
How can developers implement HEAD requests in Flash?
Developers can implement HEAD requests in Flash using the URLLoader class, setting the request method to “HEAD” when creating the URLRequest object. This allows them to receive only the headers.
In summary, the handling of HEAD requests in Flash applications is a crucial aspect of web communication, particularly for optimizing performance and resource management. HEAD requests are designed to retrieve the headers of a resource without transferring the entire content, thereby allowing clients to obtain metadata such as content type, content length, and modification dates. This functionality is essential for applications that require efficient data handling, especially when dealing with large files or limited bandwidth scenarios.
Moreover, implementing a robust response mechanism for HEAD requests can significantly enhance the user experience by reducing load times and improving the overall responsiveness of the application. By ensuring that the server correctly processes HEAD requests, developers can facilitate better caching strategies and provide clients with the necessary information to make informed decisions about resource management. This not only optimizes server performance but also contributes to a more efficient use of network resources.
Key takeaways from the discussion include the importance of understanding the nuances of HTTP methods, particularly HEAD requests, and their implications for web application development. Developers should prioritize implementing proper response handling to maximize the benefits of these requests. Furthermore, leveraging HEAD requests can lead to improved application performance, making it a vital consideration in the design and architecture of modern web applications.
Author Profile
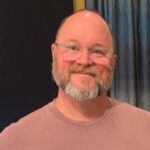
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?