How Do You Handle Unknown C Struct Types in Objective-C?
In the world of Objective-C programming, developers often encounter a variety of challenges, particularly when dealing with complex data structures. One such challenge arises when working with unknown C struct types, which can lead to confusion and frustration. Understanding how to navigate these intricacies is essential for any programmer looking to harness the full power of Objective-C and its interoperability with C. This article delves into the nuances of handling unknown C struct types, equipping you with the knowledge to tackle these situations with confidence.
When faced with an unknown C struct type in Objective-C, developers may find themselves at a crossroads, unsure of how to proceed. This scenario often occurs when interfacing with third-party libraries or legacy code, where the definitions of certain structs may be obscured or poorly documented. The lack of clarity can hinder the development process, making it crucial to grasp the underlying concepts and techniques for effectively managing these unknown types.
In this article, we will explore the common pitfalls associated with unknown C struct types in Objective-C, providing insights into best practices for defining, using, and debugging these structures. By understanding the principles of C structs and their integration within Objective-C, you will be better equipped to enhance your coding skills and streamline your development workflow. Prepare to unlock the potential of your programming
Understanding Unknown C Struct Types
When working with Objective-C, developers may encounter the term “Unknown C Struct Type.” This typically arises when interfacing with C libraries or frameworks that define complex data structures not explicitly recognized by the Objective-C compiler. Understanding how to manage these unknown struct types is essential for effective data handling and interoperability.
Identifying Unknown Structs
An unknown C struct type can manifest in several scenarios, such as when:
- The struct is defined in a C header file that hasn’t been included.
- The struct type is part of an opaque type, meaning its definition is hidden from the user.
- The struct is defined in a library that has not been properly linked.
To address these issues, you can use the following strategies:
- Ensure that all relevant header files are properly included in your Objective-C implementation.
- When working with opaque types, refer to the library documentation for the necessary interfaces to interact with the struct.
- Use forward declarations when appropriate to inform the compiler of the existence of the struct without revealing its details.
Working with Opaque Structs
When dealing with opaque structs, the implementation details are hidden, which means you can only use pointers to these structs. For example, consider a C library that provides a struct defined as follows:
“`c
typedef struct MyStruct *MyStructRef;
“`
To work with this struct in Objective-C, you might interact with it like so:
“`objc
MyStructRef myStruct = CreateMyStruct();
UseMyStruct(myStruct);
DestroyMyStruct(myStruct);
“`
This approach allows you to maintain encapsulation while still utilizing the struct’s functionality.
Common Strategies for Handling Unknown Struct Types
To effectively manage unknown struct types, consider the following strategies:
- Encapsulation: Use functions provided by the library to manipulate struct data instead of accessing struct members directly.
- Type Casting: When necessary, perform type casting carefully to avoid runtime errors and ensure type safety.
- Documentation Review: Always review the documentation of the library you are interfacing with to understand the expected usage patterns for unknown structs.
Example Table of Struct Management Techniques
Technique | Description | Use Case |
---|---|---|
Encapsulation | Utilize library functions to interact with struct data. | When structs are opaque and details are hidden. |
Type Casting | Cast pointers to the appropriate struct type. | When interfacing with C functions that expect specific types. |
Forward Declaration | Declare struct types before use without providing definitions. | To inform the compiler of a type’s existence. |
By employing these techniques, developers can effectively navigate the challenges posed by unknown C struct types in Objective-C, ensuring robust and maintainable code.
Understanding Unknown C Struct Types in Objective-C
When dealing with C struct types in Objective-C, encountering the error “Unknown C Struct Type” often indicates that the compiler cannot recognize a specific struct definition. This issue typically arises due to a few common reasons, which can be addressed systematically.
Common Causes of the Error
- Missing Header File: The struct may be defined in a header file that has not been imported into your Objective-C file.
- Incorrect Order of Declarations: Structs must be declared before they are used. If you reference a struct before its declaration, the compiler will raise an error.
- Namespace Conflicts: If there are naming conflicts between Objective-C and C types, the compiler may fail to resolve the struct type correctly.
- Typedef Misuse: Using a typedef incorrectly can lead to confusion about the struct type. Ensure that the typedef is properly defined.
- Compiler Settings: Ensure that your project settings allow for mixing C and Objective-C code, particularly in file types and compilation flags.
Resolution Strategies
To resolve the “Unknown C Struct Type” error, consider the following strategies:
- Check Header Imports:
- Ensure that you include the correct header file at the top of your Objective-C file:
“`objc
import “YourStructHeader.h”
“`
- Verify Declaration Order:
- Ensure that the struct is declared before any usage in the code:
“`c
typedef struct {
int field1;
float field2;
} MyStruct;
MyStruct myStructInstance;
“`
- Avoid Naming Conflicts:
- Rename your struct or the conflicting type to avoid ambiguity.
- Correct Typedef Usage:
- Make sure the typedef is set up correctly. For example:
“`c
typedef struct {
int id;
char name[50];
} Person;
“`
- Check Project Settings:
- Ensure that the project settings allow for C files to be compiled with Objective-C. This may involve checking the file types and ensuring proper compatibility.
Example of Struct Declaration and Usage
“`c
// ExampleStruct.h
ifndef ExampleStruct_h
define ExampleStruct_h
typedef struct {
int x;
int y;
} Point;
endif /* ExampleStruct_h */
“`
“`objc
// ExampleUsage.m
import “ExampleStruct.h”
@implementation ExampleUsage
- (void)useStruct {
Point point;
point.x = 10;
point.y = 20;
NSLog(@”Point coordinates: (%d, %d)”, point.x, point.y);
}
@end
“`
Best Practices for Structs in Objective-C
- Always Include Header Files: Make it a habit to include necessary header files where structs are defined.
- Maintain Clean Code: Keep struct definitions organized and avoid placing them in implementation files.
- Use Namespaces: To prevent naming conflicts, consider using namespaces or unique prefixes for struct names.
- Comment Your Code: Document the purpose of each struct for better maintainability.
By following these guidelines and addressing the common pitfalls, you can effectively manage C structs within your Objective-C projects, ensuring smoother compilation and fewer errors.
Understanding Objective-C and the Challenge of Unknown C Struct Types
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When dealing with Objective-C, encountering an unknown C struct type can be particularly challenging. It is crucial to have a clear understanding of the data structure’s definition in C, as Objective-C relies heavily on these types for its interoperability with C libraries. Without proper documentation or type definitions, developers may face significant hurdles in debugging and maintaining their code.”
Michael Chen (Lead iOS Developer, App Solutions LLC). “The integration of C structs in Objective-C is often a source of confusion, especially when the struct types are not explicitly defined. Developers should utilize the ‘typedef’ keyword to create more readable and maintainable code. Additionally, leveraging tools like Clang can help in identifying and resolving issues related to unknown struct types during the compilation process.”
Sarah Patel (Technical Writer and C Programming Expert). “Understanding the implications of unknown C struct types in Objective-C is essential for effective memory management and type safety. Developers should prioritize creating comprehensive headers that define all structs used within their projects. This practice not only aids in clarity but also prevents runtime errors that can arise from misinterpreted data types.”
Frequently Asked Questions (FAQs)
What does “Unknown C Struct Type” mean in Objective-C?
The term “Unknown C Struct Type” refers to a situation where the compiler encounters a C structure that has not been defined or declared prior to its use in the Objective-C code. This typically results in a compilation error.
How can I resolve the “Unknown C Struct Type” error in my Objective-C code?
To resolve this error, ensure that the struct type is properly defined before its usage. Include the struct definition in the code or import the relevant header file that contains the struct definition.
Can I use C structs directly in Objective-C?
Yes, you can use C structs directly in Objective-C. Objective-C is a superset of C, allowing for seamless integration of C data types, including structs.
What are common reasons for encountering “Unknown C Struct Type”?
Common reasons include forgetting to include the header file where the struct is defined, misspelling the struct name, or using the struct in a scope where it is not visible.
Are there any best practices for using C structs in Objective-C?
Best practices include clearly defining structs in header files, using meaningful names for structs, and ensuring that struct definitions are included in the appropriate implementation files to avoid visibility issues.
Is it possible to create Objective-C classes that encapsulate C structs?
Yes, it is possible to create Objective-C classes that encapsulate C structs. This approach allows for better memory management and object-oriented design while still utilizing the performance benefits of C structs.
In Objective-C, encountering an “Unknown C Struct Type” error typically indicates that the compiler cannot recognize a specific struct type being referenced in the code. This issue often arises when the struct has not been properly defined or declared before its usage. It is crucial to ensure that any custom struct types are defined in the correct scope and that the necessary header files are included in the implementation files where they are utilized.
Additionally, developers should pay attention to the order of declarations and ensure that any dependencies between structs are resolved. If a struct is defined within a specific context, such as within a class or a category, it may not be accessible from other parts of the code without proper forward declarations. Utilizing forward declarations can help mitigate issues related to circular dependencies and improve code organization.
Moreover, it is essential to verify that the struct definitions are not being obscured by naming conflicts or scope issues. Utilizing unique names for structs and being mindful of the visibility of these types can prevent many common pitfalls. By adhering to best practices in struct definition and organization, developers can minimize the occurrence of “Unknown C Struct Type” errors and enhance the overall robustness of their Objective-C code.
Author Profile
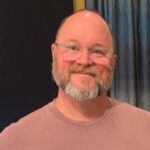
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?