How Can You Import a Python File from Another Directory?
In the world of Python programming, organizing your code efficiently is crucial for maintaining clarity and scalability. As projects grow in complexity, you may find yourself needing to access functions, classes, or variables defined in different directories. This is where the ability to import Python files from another directory becomes invaluable. Whether you’re working on a small script or a large application, mastering this skill can enhance your workflow and streamline your development process.
Importing files from different directories is not just a matter of convenience; it also promotes code reuse and modularity. By structuring your projects effectively, you can keep related functionalities together while still maintaining a clean and organized codebase. However, navigating the intricacies of Python’s import system can be daunting for newcomers and even seasoned developers alike. Understanding the various methods available for importing files from other directories is essential for leveraging Python’s full potential.
In this article, we will explore the different techniques for importing Python files across directories, shedding light on the underlying principles that govern Python’s import mechanism. From utilizing the built-in `sys` module to leveraging package structures, we’ll guide you through the steps necessary to make your code more modular and efficient. So, let’s dive in and unlock the power of cross-directory imports in Python!
Understanding the Python Module Search Path
In Python, the module search path is a crucial concept that determines how Python locates and imports modules and files. The search path is stored in the `sys.path` list, which includes directories from which Python can load modules. This list is initialized from the environment variable `PYTHONPATH`, the current directory, and the installation-dependent default paths.
To view the current module search path, you can use the following code snippet:
“`python
import sys
print(sys.path)
“`
This will display a list of directories Python checks when you attempt to import a module.
Modifying the Module Search Path
If you need to import a Python file from another directory that is not included in the default search path, you can modify `sys.path` at runtime. This allows you to add the desired directory to the search path dynamically. Here’s how to do it:
- Import the `sys` module.
- Append the path of the target directory to `sys.path`.
Example:
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import your_module
“`
This method is useful for quick imports during development or testing, but it is generally not recommended for production code due to potential maintenance issues.
Using Packages and `__init__.py`
A more structured approach to organize your Python files is to use packages. By creating a package, you can easily manage imports from various directories. To create a package, follow these steps:
- Create a directory for your package.
- Inside that directory, create a file named `__init__.py`. This file can be empty or contain initialization code for the package.
- Place your Python files (modules) in this directory.
For example, given the following structure:
“`
my_package/
__init__.py
module_a.py
module_b.py
“`
You can import `module_a` from another directory like this:
“`python
from my_package import module_a
“`
Using Relative Imports
Relative imports allow you to import modules based on the current module’s location within the package structure. This is particularly useful in larger packages. For instance, if you have the following structure:
“`
my_package/
__init__.py
module_a.py
sub_package/
__init__.py
module_b.py
“`
You can import `module_a` in `module_b.py` using:
“`python
from .. import module_a
“`
Relative imports help maintain a clean and organized import structure within packages.
Table of Import Methods
Method | Description | Use Case |
---|---|---|
sys.path.append() | Dynamically adds a directory to the module search path. | Quick imports during development. |
Packages with __init__.py | Organizes modules within a directory structure. | For larger applications to manage code. |
Relative Imports | Imports modules relative to the current module’s location. | When working within sub-packages. |
By employing these techniques, you can effectively manage and import Python files from different directories, facilitating a more organized and modular codebase.
Understanding Python’s Module System
Python’s module system allows for organized code, enabling the import of files from various directories. To successfully import a Python file from another directory, understanding the structure and syntax is crucial.
Using Absolute Paths
One method to import a Python file from another directory involves using the absolute path. An absolute path specifies the full path to the directory containing the Python file.
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import your_module
“`
- Replace `/path/to/your/directory` with the actual directory path.
- Replace `your_module` with the name of the Python file (without the `.py` extension).
Utilizing Relative Paths
Relative paths allow you to import modules based on the current working directory. This is useful for maintaining portability across different environments.
“`python
import sys
import os
sys.path.append(os.path.join(os.path.dirname(__file__), ‘../your_directory’))
import your_module
“`
- `os.path.dirname(__file__)` retrieves the directory of the current file.
- `../your_directory` navigates one level up and then into the specified directory.
Creating an `__init__.py` File
To treat a directory as a package, include an `__init__.py` file in that directory. This file can be empty or contain initialization code.
- Create an `__init__.py` file in the target directory.
- Import the desired module using a standard import statement.
Example structure:
“`
your_project/
│
├── main.py
└── your_directory/
├── __init__.py
└── your_module.py
“`
In `main.py`, you can import the module as follows:
“`python
from your_directory import your_module
“`
Using Importlib for Dynamic Imports
For scenarios where the module name is not known until runtime, the `importlib` module can be used for dynamic imports.
“`python
import importlib.util
import sys
module_name = ‘your_module’
file_path = ‘/path/to/your/directory/your_module.py’
spec = importlib.util.spec_from_file_location(module_name, file_path)
your_module = importlib.util.module_from_spec(spec)
sys.modules[module_name] = your_module
spec.loader.exec_module(your_module)
“`
- This method allows for importing modules dynamically and is useful for plugins or varying configurations.
Common Issues and Troubleshooting
When importing from another directory, several issues may arise:
Issue | Solution |
---|---|
Module not found error | Check the path and ensure it is correct. |
ImportError on package | Ensure `__init__.py` exists in the directory. |
Conflicting module names | Use absolute imports to avoid ambiguity. |
Circular imports | Refactor code to prevent circular dependencies. |
By addressing these common issues, you can streamline the process of importing Python files from different directories.
Expert Insights on Importing Python Files from Different Directories
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When importing Python files from another directory, it is crucial to ensure that the target directory is included in the Python path. This can be achieved by modifying the `sys.path` list or using relative imports effectively. Understanding the directory structure is essential for maintaining clean and efficient code.”
Michael Chen (Python Developer Advocate, CodeCraft). “One common practice for importing files across directories is to use the `__init__.py` file to make a directory a package. This allows for cleaner imports and better organization of your codebase. Always consider the implications of circular imports when structuring your modules.”
Sarah Thompson (Lead Data Scientist, Data Solutions Group). “In data science projects, managing file imports from various directories can become complex. Utilizing virtual environments and maintaining a consistent project structure can simplify the import process, ensuring that all dependencies are correctly resolved without conflicts.”
Frequently Asked Questions (FAQs)
How can I import a Python file from a different directory?
To import a Python file from another directory, you can modify the `sys.path` list to include the directory containing the file. Use the following code:
“`python
import sys
sys.path.append(‘/path/to/directory’)
import your_module
“`
What is the purpose of the `sys.path` list in Python?
The `sys.path` list in Python contains the directories that the interpreter searches for modules to import. By appending a directory to `sys.path`, you instruct Python to look in that directory when attempting to import modules.
Can I use relative paths to import a Python file from another directory?
Yes, you can use relative paths to import a Python file. However, you need to ensure that the relative path is correctly specified based on the current working directory of your script. Use `from .. import your_module` for relative imports within packages.
What are the potential issues with importing files from different directories?
Potential issues include module name conflicts, difficulty in maintaining code readability, and challenges in managing dependencies. It is advisable to structure your project using packages to avoid these problems.
Is there a way to permanently add a directory to Python’s import path?
Yes, you can permanently add a directory to Python’s import path by setting the `PYTHONPATH` environment variable in your operating system. This allows Python to recognize the specified directory every time it runs.
What is the best practice for organizing Python modules across directories?
The best practice is to organize modules into packages using directories with `__init__.py` files. This structure enhances code maintainability and clarity, allowing for easier imports and better project organization.
importing a Python file from another directory is a common task that can be accomplished through several methods. The most straightforward approach involves using the `sys` module to append the target directory to the system path, allowing Python to locate and import the desired file. Alternatively, utilizing the `os` module can also facilitate the addition of directories to the path, providing a flexible way to manage imports across different project structures.
Another effective method is to structure your project as a package by including an `__init__.py` file in the target directory. This approach not only organizes the code better but also allows for relative imports, making it easier to maintain and scale the project. Additionally, using the `importlib` module offers a dynamic way to import modules, which can be particularly useful in scenarios where the module name is not known until runtime.
Key takeaways from this discussion include the importance of understanding Python’s module search path and the various methods available for importing files from different directories. By leveraging these techniques, developers can enhance code organization, improve maintainability, and ensure that their projects remain scalable as they grow. Ultimately, knowing how to effectively manage imports is a fundamental skill for any Python programmer aiming for efficient coding practices.
Author Profile
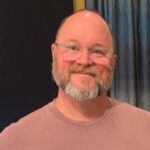
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?