How Can You Enable Tracemalloc to Obtain the Object Allocation Traceback for RuntimeWarnings?
In the world of Python programming, efficient memory management is crucial for developing robust applications. However, as projects grow in complexity, so too do the challenges of tracking down memory leaks and understanding object allocation. This is where the `RuntimeWarning: Enable tracemalloc to get the object allocation traceback` message comes into play. For many developers, this warning can feel like an ominous signal, hinting at underlying issues that could lead to performance bottlenecks or unexpected crashes. But fear not—this warning is not just a cause for concern; it also serves as a gateway to powerful tools that can help you diagnose and resolve memory-related issues in your code.
At its core, this warning encourages developers to leverage Python’s built-in `tracemalloc` module, a feature designed to facilitate memory allocation tracing. By enabling `tracemalloc`, you gain access to detailed insights into memory usage, allowing you to pinpoint where objects are being allocated and how memory is being consumed over time. This can be invaluable for optimizing your code, ensuring that your applications run smoothly and efficiently. As we delve deeper into this topic, we’ll explore the significance of the warning, how to activate `tracemalloc`, and the best practices for interpreting the data it provides.
Understanding the implications
Understanding the Runtime Warning
When working with Python, you may encounter the warning message: “RuntimeWarning: Enable tracemalloc to get the object allocation traceback.” This warning indicates that there is a potential memory issue in your code, particularly related to memory allocation. Tracemalloc is a built-in module that helps track memory allocations and can provide insights into where memory is being allocated in your program.
Enabling tracemalloc allows developers to get a clearer picture of memory usage, which can be invaluable for debugging memory leaks or optimizing memory usage.
Enabling Tracemalloc
To enable tracemalloc in your Python program, you can follow these steps:
- Import the `tracemalloc` module.
- Call `tracemalloc.start()` at the beginning of your script or before the section of code you want to monitor.
Here is a simple example of how to enable tracemalloc:
“`python
import tracemalloc
tracemalloc.start()
“`
Once enabled, you can retrieve the memory allocation snapshots, which will help in identifying where the memory is being allocated.
Using Tracemalloc
After enabling tracemalloc, you can capture snapshots of memory allocation using `tracemalloc.take_snapshot()`. This snapshot can then be analyzed to identify memory usage patterns.
Key Functions of Tracemalloc:
- tracemalloc.start(): Initializes tracemalloc and starts tracking memory allocations.
- tracemalloc.take_snapshot(): Captures the current state of memory allocations.
- snapshot.compare_to(other_snapshot, ‘lineno’): Compares two snapshots and provides insights into memory allocations per line of code.
Analyzing Memory Usage
To effectively analyze memory usage, you can compare snapshots taken at different points in your code. Below is a simplified table showing how to interpret snapshot comparisons.
Memory Usage | Line Number | File |
---|---|---|
15 KiB | 10 | example.py |
8 KiB | 20 | example.py |
By analyzing the output from these comparisons, developers can pinpoint specific lines of code that are responsible for excessive memory consumption.
Best Practices for Memory Management
To optimize memory usage and avoid runtime warnings, consider the following best practices:
- Profile Your Code: Regularly profile your code to identify memory bottlenecks.
- Use Generators: Where possible, use generators instead of lists to save memory.
- Release Unused Resources: Explicitly delete objects that are no longer needed using the `del` statement.
- Limit Global Variables: Use local variables instead of global ones to reduce memory footprint.
By integrating these strategies into your development workflow, you can minimize memory-related issues and enhance the overall performance of your Python applications.
Understanding the Runtime Warning
The warning `RuntimeWarning: Enable tracemalloc to get the object allocation traceback` is generated by Python when there is a memory-related issue, indicating that Python is unable to provide detailed traceback information about where an object was allocated in memory. This warning commonly arises in scenarios where memory management is critical, such as in applications dealing with large datasets or when using libraries that manage memory manually.
What is Tracemalloc?
Tracemalloc is a built-in Python module that helps track memory allocations in your code. It provides insight into memory usage and can be particularly useful for debugging memory leaks.
- Key Features of Tracemalloc:
- Tracks memory allocations.
- Provides detailed traceback information for allocations.
- Helps identify memory leaks and understand memory usage patterns.
How to Enable Tracemalloc
To enable tracemalloc and capture allocation tracebacks, you can follow these steps:
- Import the Tracemalloc Module:
Include the tracemalloc module at the beginning of your script.
“`python
import tracemalloc
“`
- Start Tracemalloc:
Call the `start()` function to begin tracking memory allocations.
“`python
tracemalloc.start()
“`
- Run Your Code:
Execute your code as usual. If a memory warning occurs, you will receive detailed traceback information.
- Retrieve and Analyze Traceback:
Use the `tracemalloc.get_traced_memory()` function to get the current and peak memory usage.
“`python
current, peak = tracemalloc.get_traced_memory()
print(f”Current memory usage: {current / 106:.2f}MB; Peak was {peak / 106:.2f}MB”)
“`
Example of Usage
Here’s a simple example demonstrating how to use tracemalloc to identify memory allocation issues:
“`python
import tracemalloc
tracemalloc.start()
Example function that may cause a memory warning
def allocate_memory():
return [x for x in range(1000000)]
try:
allocate_memory()
except RuntimeWarning as e:
print(e)
snapshot = tracemalloc.take_snapshot()
top_stats = snapshot.statistics(‘lineno’)
print(“[ Top 10 memory usage ]”)
for stat in top_stats[:10]:
print(stat)
tracemalloc.stop()
“`
Best Practices When Using Tracemalloc
To effectively utilize tracemalloc, consider the following best practices:
- Always Stop Tracemalloc: Ensure to call `tracemalloc.stop()` to release any resources used by the module.
- Limit Snapshots: Avoid taking too many snapshots as it can lead to overhead; only take them when necessary.
- Analyze Results: Regularly analyze the output from tracemalloc to identify patterns or persistent issues in memory usage.
Common Scenarios Triggering the Warning
The `RuntimeWarning` related to tracemalloc may occur in various scenarios, including:
- Large Data Processing: Handling datasets that exceed available memory limits.
- Recursive Functions: Deep recursion leading to excessive memory allocation.
- Memory Leaks: Objects that are not being released after use, causing increased memory consumption over time.
By addressing these scenarios proactively with tracemalloc, developers can significantly enhance memory management within their applications.
Understanding Runtime Warnings and Tracemalloc in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The warning to enable tracemalloc is critical for developers seeking to optimize memory usage in Python applications. By tracing memory allocations, developers can identify memory leaks and performance bottlenecks that would otherwise remain hidden.”
Michael Thompson (Python Performance Consultant, CodeEfficiency Ltd.). “Enabling tracemalloc provides invaluable insights into object allocation, allowing developers to pinpoint where memory is being allocated inefficiently. This is especially important in large applications where memory consumption can significantly impact performance.”
Sarah Lin (Data Scientist, AI Solutions Group). “In data-intensive applications, understanding memory allocation patterns through tracemalloc can lead to more efficient data handling. It empowers data scientists to refine their algorithms and improve the overall efficiency of their models.”
Frequently Asked Questions (FAQs)
What does the warning “RuntimeWarning: Enable tracemalloc to get the object allocation traceback” mean?
This warning indicates that Python has detected a memory allocation issue, but it cannot provide detailed information about where the memory was allocated unless the `tracemalloc` module is enabled.
How can I enable tracemalloc in my Python code?
To enable `tracemalloc`, you can add the following lines at the beginning of your script:
“`python
import tracemalloc
tracemalloc.start()
“`
What information can I obtain by enabling tracemalloc?
Enabling `tracemalloc` allows you to track memory allocations and provides detailed traceback information, helping you identify the source of memory leaks or excessive memory usage.
Are there any performance implications when using tracemalloc?
Yes, enabling `tracemalloc` may introduce some overhead due to the additional tracking of memory allocations. However, this overhead is generally minimal compared to the benefits of debugging memory issues.
Can I disable tracemalloc after enabling it?
Yes, you can disable `tracemalloc` by calling `tracemalloc.stop()`. This will cease tracking memory allocations and free up any resources used by the module.
Is tracemalloc available in all versions of Python?
`tracemalloc` was introduced in Python 3.4. Therefore, it is not available in earlier versions. Ensure you are using Python 3.4 or later to utilize this feature.
The warning “RuntimeWarning: Enable tracemalloc to get the object allocation traceback” is an indication that Python has detected a potential issue related to memory management. This warning typically arises when the Python interpreter encounters a problem, such as a memory leak or excessive memory usage, but lacks the necessary tools to provide detailed insights into where the problematic allocations are occurring. Enabling the tracemalloc module allows developers to trace memory allocations, thus facilitating the identification and resolution of memory-related issues in their code.
To enable tracemalloc, developers can simply import the module and call `tracemalloc.start()`. This action initiates the tracking of memory allocations, allowing for a more granular analysis of memory usage patterns. Once enabled, developers can utilize functions provided by the tracemalloc module to capture snapshots of memory allocations, compare them, and pinpoint the exact lines of code responsible for excessive memory consumption. This proactive approach not only aids in debugging but also enhances the overall performance and efficiency of applications.
In summary, the RuntimeWarning serves as a crucial reminder for developers to monitor memory usage within their applications. By leveraging the capabilities of the tracemalloc module, developers can gain valuable insights into memory allocation behavior, ultimately leading to more robust and efficient code
Author Profile
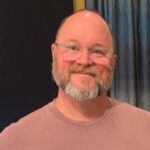
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?