Is ‘Do Nothing Python’ the Key to Simplifying Your Coding Experience?
In the fast-paced world of programming, where efficiency and productivity reign supreme, the idea of “doing nothing” might seem counterintuitive, especially in a language as versatile as Python. However, the concept of “Do Nothing Python” invites us to explore a fascinating paradox: the power of simplicity and the art of minimalism in coding. This approach encourages developers to embrace the beauty of restraint, challenging the notion that more code always leads to better solutions. As we delve into this intriguing topic, we’ll uncover how doing less can sometimes yield more, fostering creativity and clarity in our coding practices.
Overview
At its core, “Do Nothing Python” emphasizes the importance of writing code that is clean, efficient, and easy to understand. By stripping away unnecessary complexity, developers can focus on the essential elements that drive functionality and maintainability. This minimalist philosophy not only enhances the readability of code but also encourages a deeper understanding of programming principles, allowing developers to make more informed decisions when crafting their applications.
Moreover, the practice of doing nothing in Python can lead to innovative solutions that prioritize user experience and performance. By recognizing when to step back and simplify, programmers can create applications that are not only effective but also elegant. As we explore this concept further, we will examine practical
Understanding the Do Nothing Pattern
The “Do Nothing” pattern in Python refers to a design approach where a function or method is defined but does not perform any operations. This can be useful in various scenarios, such as creating stubs for future implementations, testing, or as placeholders in complex systems.
When using the Do Nothing pattern, the function typically includes a `pass` statement, which is a null operation that serves as a syntactic placeholder. This allows the code to run without producing any output or side effects, making it a safe option during development.
Use Cases for the Do Nothing Pattern
The Do Nothing pattern can be applied in several contexts:
- Placeholder Functions: When outlining a program structure, developers can define functions that will be implemented later. This helps in maintaining a clear architecture.
- Event Handlers: In GUI applications, event handlers can be defined to manage events that do not require immediate action.
- Testing: During unit testing, a Do Nothing function can be used to isolate tests without triggering side effects.
- Interface Implementations: In object-oriented programming, classes may implement methods from an interface that are not relevant to their functionality, allowing them to compile without error.
Example of the Do Nothing Pattern
Here’s a simple example of how the Do Nothing pattern can be implemented in Python:
“`python
def do_nothing():
pass
“`
In this example, the `do_nothing` function does not perform any operations. It can be invoked without causing any disruption in the program flow.
Advantages and Disadvantages
The Do Nothing pattern has its pros and cons, which are important to consider:
Advantages | Disadvantages |
---|---|
Facilitates incremental development | Can lead to unused code if not properly managed |
Improves code readability by defining structure | Might confuse new developers regarding the function’s purpose |
Allows for easier testing and debugging | Risk of overusing can clutter the codebase |
By weighing these advantages and disadvantages, developers can make informed decisions about when and how to use the Do Nothing pattern effectively.
Understanding the Do Nothing Python
The term “Do Nothing Python” typically refers to a minimalist approach in Python programming, where a function or a piece of code is created but does not perform any action. This can be useful in various scenarios, such as placeholder functions, testing, or structural frameworks.
Use Cases for Do Nothing Functions
Incorporating a Do Nothing function can be beneficial in several contexts:
- Placeholder for Future Development: When planning a feature, developers can implement a Do Nothing function to outline the structure without actual functionality.
- Testing: During testing phases, Do Nothing functions can be used to simulate behavior without executing any logic.
- Callbacks: In event-driven programming, a Do Nothing function can serve as a default callback when no specific action is required.
Implementation Example
Here is a simple example of how a Do Nothing function can be implemented in Python:
“`python
def do_nothing():
pass
“`
In this example, the `do_nothing()` function is defined but contains the `pass` statement, which allows it to function without performing any operations.
Benefits of Using Do Nothing Functions
Utilizing Do Nothing functions can lead to several advantages:
- Code Clarity: It provides a clear structure for future development, indicating where functionality will be added later.
- Reduced Errors: By using a placeholder, it can prevent runtime errors that might arise from functions.
- Improved Readability: It makes the intention of the code clearer, especially in complex systems.
Common Scenarios for Do Nothing Functions
Here are some common scenarios where you might employ Do Nothing functions:
Scenario | Description |
---|---|
Event Handlers | Functions that need to be callable but do not require action. |
Default Arguments | When a function parameter must be passed but no action is needed. |
Interface Implementation | When defining interfaces where some methods are optional. |
Best Practices
When implementing Do Nothing functions, consider the following best practices:
- Naming Conventions: Use descriptive names that clarify the purpose of the function, such as `placeholder_function` or `no_action`.
- Documentation: Include comments or docstrings to explain why the function exists and its intended future use.
- Avoid Overuse: While useful, excessive reliance on Do Nothing functions can lead to confusion if not clearly documented or if the codebase becomes cluttered.
Incorporating Do Nothing functions effectively allows for flexible development practices and code organization. By understanding their utility and best practices, developers can enhance the maintainability and clarity of their code.
Understanding the Implications of Do Nothing Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Do Nothing’ Python approach can be a double-edged sword. While it simplifies the code by removing unnecessary operations, it may lead to performance issues if not carefully managed. Developers must balance simplicity with efficiency to ensure optimal application performance.”
Michael Chen (Lead Data Scientist, Analytics Hub). “In data processing workflows, employing a ‘Do Nothing’ strategy can be beneficial for maintaining data integrity. However, it is crucial to assess the context in which this approach is applied, as it can inadvertently lead to data stagnation if updates are not systematically implemented.”
Sarah Thompson (Python Programming Instructor, Code Academy). “Teaching the ‘Do Nothing’ concept in Python is essential for beginners. It helps them understand the importance of code readability and maintenance. However, students must also learn when to implement more complex logic to avoid pitfalls associated with overly simplistic coding practices.”
Frequently Asked Questions (FAQs)
What is the purpose of the `do nothing` function in Python?
The `do nothing` function, often represented as `pass`, serves as a placeholder in Python code. It allows the programmer to define a block of code syntactically without executing any operation, which is useful in scenarios like defining empty functions or control structures.
How can I create a `do nothing` function in Python?
You can create a `do nothing` function by defining a function that contains only the `pass` statement. For example:
“`python
def do_nothing():
pass
“`
When should I use a `do nothing` function?
A `do nothing` function is typically used during development to outline the structure of code without implementing functionality. It can also be useful in situations where a function is required syntactically but no action is needed at that moment.
Can `do nothing` functions affect performance in Python?
`Do nothing` functions have negligible impact on performance since they do not perform any operations. However, excessive use of such functions can lead to less readable code, making it harder to maintain.
Is there a difference between using `pass` and leaving a function body empty?
Yes, leaving a function body empty will raise a `SyntaxError` in Python. Using `pass` explicitly indicates that the function is intentionally left without implementation, making the code syntactically correct.
Are there alternatives to using a `do nothing` function in Python?
Alternatives include using comments to indicate future implementation or raising exceptions to signal that a function is not yet implemented. However, these alternatives serve different purposes and should be used accordingly based on the context.
The concept of “Do Nothing” in Python refers to the use of the `pass` statement, which serves as a placeholder for future code. It allows developers to define a block of code syntactically without implementing any functionality. This is particularly useful in scenarios where a function, loop, or conditional statement is required but the actual implementation will be added later. The `pass` statement is essential for maintaining code structure and readability, especially during the development phase when certain functionalities are still under consideration.
Additionally, “Do Nothing” can also be interpreted in the context of optimizing code execution. In certain situations, it may be beneficial to explicitly define a no-operation (no-op) function or statement to improve clarity or performance. This approach can help avoid unnecessary complexity in the codebase, making it easier for developers to understand the intended logic without executing any operations. By using such constructs judiciously, developers can create cleaner, more maintainable code.
In summary, the “Do Nothing” concept in Python is a valuable tool for developers, enabling them to maintain code structure while planning future implementations. It emphasizes the importance of clarity and organization in programming, allowing for smoother transitions from development to deployment. Understanding and effectively utilizing the `pass` statement
Author Profile
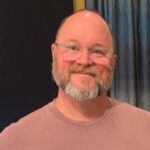
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?