Why Am I Seeing ‘No Slf4J Providers Were Found’ Error and How Can I Fix It?
In the world of Java development, logging is an essential component that helps developers track application behavior, troubleshoot issues, and maintain overall system health. However, encountering the error message “No Slf4J Providers Were Found” can be a frustrating roadblock for many. This cryptic notification often leaves developers puzzled, as it signifies a disconnect between the Simple Logging Facade for Java (SLF4J) and the underlying logging framework that should be providing the necessary functionality. Understanding the implications of this message is crucial for any developer aiming to implement effective logging in their applications.
The SLF4J framework serves as a bridge, allowing developers to plug in various logging implementations without altering their code. When you see the “No Slf4J Providers Were Found” error, it typically indicates that the SLF4J API is present in your project, but the corresponding logging implementation is missing or not properly configured. This oversight can lead to a lack of logging output, making it difficult to diagnose problems or monitor application performance.
In the following sections, we will delve deeper into the causes of this error, explore common scenarios that lead to its occurrence, and provide actionable solutions to ensure that your logging setup is robust and effective. Whether you’re a seasoned developer or just starting
No Slf4J Providers Were Found
When encountering the error message “No Slf4J Providers Were Found,” it indicates that the SLF4J (Simple Logging Facade for Java) framework is unable to locate a compatible logging implementation at runtime. This can lead to application logging issues, as SLF4J serves as a façade for various logging frameworks.
The primary reasons for this error typically include:
- Absence of a logging backend on the classpath.
- Presence of multiple SLF4J bindings, causing a conflict.
- Misconfiguration of the classpath in the project.
To resolve this issue, follow these steps:
- Check for SLF4J Bindings: Ensure that there is at least one SLF4J binding present in your project. Common bindings include:
- `slf4j-log4j12`
- `slf4j-simple`
- `slf4j-jdk14`
- Verify Classpath Configuration: Make sure the dependencies are correctly added to your build configuration (e.g., Maven, Gradle). Here’s an example of how to add a dependency in Maven:
“`xml
“`
- Remove Conflicting Bindings: If multiple SLF4J bindings are present, remove the unnecessary ones. This can be done by inspecting your dependencies for duplicates.
Here’s a simple table summarizing common SLF4J bindings and their usage:
Binding | Description | Usage Context |
---|---|---|
slf4j-log4j12 | Binding for Log4j 1.x | Legacy applications using Log4j |
slf4j-simple | Simple implementation for quick logging | Lightweight applications or testing |
slf4j-logback | Binding for Logback, a modern logging framework | Newer applications with advanced logging needs |
After ensuring that the appropriate SLF4J bindings are in place and there are no conflicts, restart your application. The error should be resolved, allowing your application to log messages correctly.
In addition to the above, verify your project structure and ensure that your build tool’s configuration file does not inadvertently exclude any necessary logging libraries. If using an IDE, ensure that the project is refreshed and dependencies are reloaded to reflect any changes made.
Understanding the `No Slf4J Providers Were Found` Error
The error message `No Slf4J Providers Were Found` indicates that the Simple Logging Facade for Java (SLF4J) is unable to locate any compatible logging implementation in your classpath. This is a common issue when setting up Java applications that use SLF4J for logging.
Common Causes
Several factors can lead to this error:
- Missing SLF4J Binding: The most prevalent cause is the absence of a binding library that connects SLF4J to an actual logging framework.
- Classpath Issues: The SLF4J implementation may be present in the project but not included in the runtime classpath.
- Version Mismatch: A mismatch between the SLF4J API version and the binding version can also trigger this error.
- Dependency Conflicts: Conflicting dependencies in your project can lead to SLF4J not recognizing the available logging implementations.
How to Resolve the Error
To effectively resolve the `No Slf4J Providers Were Found` error, follow these steps:
- Add SLF4J Binding:
- Ensure you have a compatible SLF4J binding in your project. Common bindings include:
- `slf4j-log4j12`
- `slf4j-simple`
- `slf4j-jdk14`
- If using Maven, include the relevant dependency in your `pom.xml`:
“`xml
“`
- Verify Classpath:
- Ensure that your build system (Maven, Gradle, etc.) is correctly including the SLF4J API and its binding in the runtime classpath.
- Check for Version Compatibility:
- Make sure that the versions of SLF4J API and the binding you are using are compatible. Refer to the SLF4J [version compatibility matrix](http://www.slf4j.org/faq.htmlcompatibility).
- Resolve Dependency Conflicts:
- Use tools like Maven’s `dependency:tree` or Gradle’s dependency insight to identify and resolve any conflicts in your dependencies.
Example of Correct Configuration
Here’s an example of a correct Maven configuration that includes SLF4J with Logback, a common logging backend:
“`xml
“`
Testing Your Configuration
After adjusting your configuration, you should test to confirm that SLF4J is correctly recognizing the logging implementation. You can do this by adding a simple logging statement in your application:
“`java
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class LoggingExample {
private static final Logger logger = LoggerFactory.getLogger(LoggingExample.class);
public static void main(String[] args) {
logger.info(“SLF4J is configured correctly.”);
}
}
“`
If you see the log output as expected, your configuration is correct, and the error should be resolved.
Understanding the Absence of SLF4J Providers
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘No SLF4J Providers Were Found’ typically indicates that the SLF4J API is present in the classpath, but there is no corresponding logging implementation available. Developers must ensure that a compatible SLF4J binding, such as Logback or Log4j, is included in their project dependencies to resolve this issue.”
Mark Thompson (Java Development Consultant, CodeCraft Solutions). “In many cases, this error arises during the build process due to missing dependencies in the project configuration. It is crucial to review the build tool configuration, whether Maven or Gradle, to confirm that the necessary SLF4J provider is correctly declared and resolved.”
Susan Lee (Lead Software Architect, Global Tech Services). “Understanding the logging framework’s architecture is essential. SLF4J serves as a facade, and without a concrete logging implementation, applications will fail to log messages. Developers should not only include the SLF4J API but also ensure that the appropriate logging backend is configured to avoid runtime errors.”
Frequently Asked Questions (FAQs)
What does the error “No Slf4J Providers Were Found” mean?
This error indicates that the SLF4J (Simple Logging Facade for Java) framework cannot find a suitable logging implementation in the classpath. SLF4J serves as a facade for various logging frameworks, and without a concrete provider, logging functionality will be unavailable.
How can I resolve the “No Slf4J Providers Were Found” error?
To resolve this error, ensure that you include a compatible SLF4J binding in your project’s dependencies. Common bindings include SLF4J with Logback, Log4j, or java.util.logging. Verify that the chosen binding is correctly added to your build configuration.
What are some common SLF4J providers I can use?
Common SLF4J providers include Logback, Log4j 2, and JDK logging. Each of these implementations offers different features and configurations, allowing developers to choose one that best fits their project’s requirements.
Can I use multiple SLF4J providers simultaneously?
It is not advisable to use multiple SLF4J providers simultaneously, as this can lead to conflicts and unpredictable behavior. Always ensure that only one binding is present in the classpath to maintain consistent logging behavior.
How can I check if my SLF4J provider is correctly configured?
You can check your SLF4J provider configuration by reviewing your project’s dependencies and ensuring that the appropriate SLF4J binding is included. Additionally, running your application should not produce the “No Slf4J Providers Were Found” error if configured correctly.
What should I do if I have added a provider but still see the error?
If you have added a provider but still encounter the error, check for potential issues such as version mismatches, classpath conflicts, or missing dependencies. Ensure that your build tool is correctly resolving and including the necessary libraries.
The issue of “No Slf4J Providers Were Found” typically arises in Java applications that utilize the Simple Logging Facade for Java (SLF4J) framework. This message indicates that the SLF4J API is present in the classpath, but there are no compatible logging implementations available. Consequently, the application is unable to perform logging operations, which can hinder debugging and monitoring efforts.
To resolve this issue, developers should ensure that a compatible SLF4J binding is included in their project dependencies. Common bindings include Logback, Log4j, and java.util.logging. It is essential to check the project’s build configuration, such as Maven or Gradle files, to confirm that the appropriate logging implementation is declared. Additionally, verifying that there are no conflicting SLF4J versions in the classpath can prevent further complications.
In summary, addressing the “No Slf4J Providers Were Found” warning is crucial for maintaining effective logging practices in Java applications. By ensuring that a suitable SLF4J binding is present and correctly configured, developers can enhance their application’s reliability and ease of troubleshooting. This proactive approach not only improves the development experience but also contributes to the overall quality of the software produced.
Author Profile
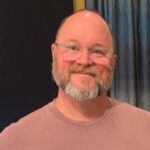
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?