How Can You Resolve the OSError: [Errno 24] Too Many Open Files in Python?
If you’re a Python developer, you may have encountered the frustrating `OSError: [Errno 24] Too Many Open Files` error at some point in your coding journey. This cryptic message can halt your progress, leaving you scratching your head and searching for answers. As applications become increasingly complex and data-driven, managing file handles efficiently is more crucial than ever. Understanding the underlying causes of this error not only helps you troubleshoot effectively but also empowers you to write more robust and scalable code.
In essence, this error arises when your application exceeds the limit of file descriptors that the operating system allows. Each time a file is opened, a file descriptor is allocated, and if these descriptors are not properly managed, you can quickly find yourself hitting the ceiling. This issue is particularly common in scenarios involving multiple file operations, such as reading from or writing to numerous files simultaneously, or when using libraries that handle files in the background without proper cleanup.
Moreover, the implications of this error extend beyond mere annoyance; they can lead to performance bottlenecks and application crashes. By delving into the reasons behind the `OSError: [Errno 24]`, we can uncover best practices for managing resources effectively, as well as strategies for optimizing file handling in Python. Whether
Understanding the Error
The `OSError: [Errno 24] Too Many Open Files` in Python typically indicates that a program has exceeded the limit on the number of file descriptors it can have open simultaneously. This limit is set by the operating system and can vary based on the system configuration and user settings.
File descriptors are used by the operating system to manage open files, network connections, and other I/O resources. Each open file or socket consumes a file descriptor. When a Python application does not properly close these resources, it can lead to exhaustion of the available descriptors.
Common Causes
Several issues can lead to this error:
- Improper File Handling: Failing to close files after opening them, especially in loops or long-running processes.
- Excessive Resource Allocation: Opening too many files simultaneously without managing their lifecycle.
- Third-party Libraries: Some libraries may not properly manage file descriptors, leading to leaks.
- System Limitations: Default limits on file descriptors can be too low for certain applications.
Checking Current Limits
You can check the current file descriptor limits on a Unix-based system using the following command in the terminal:
“`bash
ulimit -n
“`
This command will return the maximum number of open files allowed for your user session.
Increasing the Limit
If your application requires more file descriptors than the current limit allows, you can increase this limit temporarily or permanently.
- Temporary Increase: Use `ulimit` in the terminal before starting your Python application.
“`bash
ulimit -n 4096
“`
- Permanent Increase: Modify the system configuration files (e.g., `/etc/security/limits.conf`) to set the limits for specific users or groups. Add the following lines:
“`
username soft nofile 4096
username hard nofile 8192
“`
Replace `username` with the actual user account name.
Best Practices for File Handling
To prevent `OSError: [Errno 24] Too Many Open Files`, consider the following best practices:
- Use Context Managers: Leverage the `with` statement for file operations. This ensures files are automatically closed after their block of code is executed.
“`python
with open(‘file.txt’, ‘r’) as f:
data = f.read()
“`
- Limit Simultaneous Open Files: Use a queue or a pool to manage concurrent file operations and ensure that only a manageable number of files are open at any given time.
- Explicitly Close Files: If not using context managers, ensure files are closed using `f.close()` after operations.
Example of Managing Open Files
Here is an example that demonstrates managing multiple file operations using a context manager:
“`python
file_paths = [‘file1.txt’, ‘file2.txt’, ‘file3.txt’]
for path in file_paths:
with open(path, ‘r’) as f:
process_file(f)
“`
This approach minimizes the risk of hitting the open files limit by ensuring each file is closed immediately after it is processed.
Resource Management Summary
Maintaining an efficient resource management strategy is crucial for avoiding `OSError: [Errno 24] Too Many Open Files`. Below is a summary table of techniques and their benefits:
Technique | Benefits |
---|---|
Context Managers | Automatic resource management, reduces leaks |
File Pools | Controlled concurrency, limited open files |
Explicit Closure | Ensures files are closed when done |
Understanding the Error
The `OSError: [Errno 24] Too Many Open Files` indicates that a process has exceeded the limit of file descriptors that can be opened simultaneously. Each operating system imposes a limit on the number of files that can be open at any given time, which varies based on system settings and user permissions.
Common Causes
Several scenarios can lead to this error:
- File Leaks: Failing to close file handles properly after usage can accumulate open files.
- High Concurrency: Applications that open many files simultaneously, such as web servers or data processing scripts, can hit the limit quickly.
- Misconfiguration: Default limits set by the operating system may be too low for specific applications or workloads.
Identifying the Limit
To check the current limit of open files on your system, you can use the following commands:
- Linux/MacOS:
“`bash
ulimit -n
“`
- Windows:
Windows does not have a direct equivalent to `ulimit`, but you can check the maximum number of handles per process using tools like Process Explorer.
Increasing the Limit
To resolve the issue, consider increasing the limit of open files:
- Linux:
- Temporarily increase the limit using:
“`bash
ulimit -n [new_limit]
“`
- For a permanent change, edit `/etc/security/limits.conf` and add:
“`
username soft nofile [new_limit]
username hard nofile [new_limit]
“`
- MacOS:
Edit the `/etc/sysctl.conf` file to include:
“`
kern.maxfiles=20480
kern.maxfilesperproc=20480
“`
- Windows:
Windows generally handles file descriptors differently; however, ensure your application does not exceed its handle limits.
Best Practices to Avoid the Error
Implementing best practices can help prevent hitting the open files limit:
- Ensure Proper Closure: Always close file handles using `with` statements or explicitly calling `.close()`.
- Limit Concurrent Access: Use connection pooling or limit the number of concurrent file accesses in your application.
- Monitor Resource Usage: Regularly check the number of open files during application runtime to identify potential leaks early.
Example Code Snippet
Here is a sample Python code snippet demonstrating proper file handling:
“`python
def read_file(file_path):
with open(file_path, ‘r’) as file:
data = file.read()
return data
“`
This approach ensures that the file is automatically closed after reading, minimizing the risk of exceeding the open file limit.
Tools for Monitoring
Utilize tools to monitor file usage and detect leaks:
Tool | Description |
---|---|
`lsof` | Lists open files and processes using them. |
`fuser` | Identifies processes using files or sockets. |
Resource Monitor | Windows built-in tool for tracking resource usage. |
By employing these strategies, you can effectively manage and mitigate the risk of encountering `OSError: [Errno 24] Too Many Open Files`.
Understanding the Implications of Oserror: [Errno 24] Too Many Open Files in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Oserror: [Errno 24] Too Many Open Files’ typically indicates that a Python application has exceeded the limit of file descriptors that can be opened simultaneously. This often occurs in applications that handle numerous file operations without properly closing file handles, leading to resource exhaustion.”
James Liu (Systems Architect, Cloud Solutions Group). “To mitigate the ‘Too Many Open Files’ error, developers should implement proper resource management techniques, such as using context managers in Python. This approach ensures that files are automatically closed after their usage, thus preventing the accumulation of open file descriptors.”
Sarah Thompson (DevOps Specialist, Agile Tech Services). “Monitoring and adjusting the system’s file descriptor limits can also be crucial. By using commands like ‘ulimit’ in Unix-based systems, developers can increase the maximum number of open files, thereby providing a temporary solution to the problem while addressing underlying code inefficiencies.”
Frequently Asked Questions (FAQs)
What does the error “Oserror: [Errno 24] Too Many Open Files” mean in Python?
This error indicates that your Python application has exceeded the limit of open file descriptors allowed by the operating system. Each file, socket, or pipe opened by the application counts against this limit.
What causes the “Too Many Open Files” error in Python applications?
The error can occur due to not properly closing files after opening them, excessive concurrent file operations, or reaching the system-wide limit for open files set by the operating system.
How can I check the current limit of open files in my system?
You can check the current limit by using the command `ulimit -n` in a Unix-based terminal. This command will display the maximum number of file descriptors that can be opened by a single process.
How can I increase the limit of open files in a Unix-based system?
To increase the limit, you can use the command `ulimit -n
What are some best practices to avoid the “Too Many Open Files” error in Python?
Best practices include ensuring that all files are closed after use, using context managers (the `with` statement) for file operations, and monitoring the number of open files during application runtime.
Can this error occur in other programming languages besides Python?
Yes, the “Too Many Open Files” error can occur in any programming language that interacts with the operating system’s file descriptor limit, including languages like Java, C, and Ruby.
The error message “OSError: [Errno 24] Too Many Open Files” in Python typically indicates that a program has exceeded the limit of file descriptors that can be opened simultaneously. This limit is enforced by the operating system and can vary based on system configuration. When a Python application attempts to open more files than the allowed limit, it will trigger this error, leading to potential disruptions in the application’s functionality.
To address this issue, developers can take several approaches. First, it is essential to ensure that files are properly closed after their use to free up file descriptors. Utilizing context managers (the `with` statement) in Python can facilitate this process by automatically closing files when they are no longer needed. Additionally, developers can monitor the number of open files during the application’s execution to identify potential leaks or inefficiencies in resource management.
Another strategy involves increasing the file descriptor limit at the system level. This can be achieved by modifying system configurations, such as the `/etc/security/limits.conf` file on Unix-like systems. However, this should be done with caution, as excessively high limits can lead to other performance issues. Ultimately, a combination of proper file handling practices and system-level adjustments can help mitigate the “Too Many Open Files
Author Profile
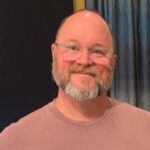
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?