How Can You Efficiently Find Text in a SQL Server Stored Procedure?
In the ever-evolving landscape of database management, SQL Server stands out as a robust platform for handling vast amounts of data. Among its many features, stored procedures play a pivotal role in enhancing performance and maintaining the integrity of database operations. However, as applications grow and evolve, the need to locate specific text or keywords within these stored procedures becomes increasingly crucial. Whether you’re troubleshooting, optimizing, or simply trying to understand legacy code, knowing how to efficiently find text within stored procedures can save you time and effort, allowing you to focus on what truly matters—delivering value through your data.
Navigating through the intricate web of stored procedures can often feel daunting, especially when searching for specific text or functionality. SQL Server provides various tools and techniques that can simplify this process, enabling developers and database administrators to quickly pinpoint relevant sections of code. From leveraging built-in system views to utilizing third-party tools, the methods available can significantly streamline your workflow and enhance your productivity.
Understanding how to effectively find text in stored procedures not only aids in code maintenance but also fosters better collaboration among team members. As developers share responsibilities and codebases evolve, having the ability to search for specific keywords can lead to quicker resolutions of issues and a deeper understanding of the underlying logic. In this article, we will explore the
Using SQL Server Management Studio (SSMS)
To find text within stored procedures in SQL Server Management Studio (SSMS), you can utilize the built-in search functionality. This is particularly useful when you need to locate specific keywords, variable names, or SQL commands within your stored procedures. Here’s how to do it:
- Open SSMS and connect to your database instance.
- In the Object Explorer, navigate to the database that contains the stored procedures.
- Right-click on the database and select “View” -> “Object Explorer Details.”
- In the Object Explorer Details window, you can filter the stored procedures by typing `proc` in the search box.
- Once the stored procedures are displayed, select the one you want to search within.
- Press `Ctrl + F` or go to the menu and select “Edit” -> “Find” to open the Find dialog.
- Enter the text you wish to find, and ensure that the “Current Project” or “Entire Solution” option is selected, depending on the scope of your search.
This method allows you to efficiently locate text within stored procedures and can be repeated for any other objects within the database.
Using T-SQL Queries
If you prefer a programmatic approach or need to search across multiple stored procedures, you can use T-SQL queries to search for text. The following query can be utilized to find specific text within the definitions of stored procedures:
“`sql
SELECT
p.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules AS m
INNER JOIN
sys.objects AS p ON m.object_id = p.object_id
WHERE
p.type = ‘P’ AND
m.definition LIKE ‘%YourSearchText%’
“`
Replace `YourSearchText` with the text you are searching for. This query will return the names of the stored procedures and their definitions that contain the specified text.
Searching in Multiple Objects
When you need to search not just in stored procedures but across multiple SQL objects, you can expand your T-SQL query as follows:
“`sql
SELECT
o.type_desc AS ObjectType,
o.name AS ObjectName,
m.definition AS ObjectDefinition
FROM
sys.sql_modules AS m
INNER JOIN
sys.objects AS o ON m.object_id = o.object_id
WHERE
m.definition LIKE ‘%YourSearchText%’
“`
This query will search through all SQL objects, including views, functions, and stored procedures, enabling you to find occurrences of the text across your entire database.
Object Type | Object Name | Object Definition |
---|---|---|
Procedure | spGetEmployees | CREATE PROCEDURE spGetEmployees AS … |
View | vwEmployeeDetails | SELECT * FROM Employees … |
Utilizing these methods will provide you with a comprehensive approach to finding text in stored procedures and other SQL objects within SQL Server, aiding in code maintenance and debugging efforts.
Identifying Text in Stored Procedures
To locate specific text or keywords within stored procedures in SQL Server, you can use several methods. The most common approach involves querying system views or using built-in functions. Below are the primary techniques to achieve this.
Using the sys.sql_modules View
The `sys.sql_modules` view contains a row for each object that is of type SQL Server module, which includes stored procedures. To find specific text, you can use a query like the following:
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%YourSearchText%’
“`
Replace `YourSearchText` with the text you are searching for. This query returns the names and definitions of stored procedures that contain the specified text.
Using the sys.objects View
You can also join `sys.objects` with `sys.sql_modules` to filter by type. This is particularly useful if you want to search only within stored procedures:
“`sql
SELECT
o.name AS ProcedureName,
m.definition
FROM
sys.objects o
JOIN
sys.sql_modules m ON o.object_id = m.object_id
WHERE
o.type = ‘P’ AND
m.definition LIKE ‘%YourSearchText%’
“`
This query filters the results to include only those objects of type ‘P’ (which stands for stored procedures).
Searching for Text in All Database Objects
If you want to search across all database objects, including views and functions, you can modify the previous query:
“`sql
SELECT
o.type_desc AS ObjectType,
o.name AS ObjectName,
m.definition
FROM
sys.objects o
JOIN
sys.sql_modules m ON o.object_id = m.object_id
WHERE
m.definition LIKE ‘%YourSearchText%’
“`
This will return all objects that contain the specified text, along with their types.
Using the sp_helptext Stored Procedure
For a specific stored procedure, you can use `sp_helptext` to display the text of the procedure. This method is useful for manual inspection:
“`sql
EXEC sp_helptext ‘YourProcedureName’
“`
This will output the full definition of the specified stored procedure, allowing you to visually inspect it for your text.
Automation with PowerShell
For more advanced searching or automation, PowerShell can be used to script the search across multiple stored procedures. Here’s a simple example:
“`powershell
$connectionString = “Server=YourServer;Database=YourDatabase;Integrated Security=True;”
$query = “SELECT OBJECT_NAME(object_id), definition FROM sys.sql_modules WHERE definition LIKE ‘%YourSearchText%'”
Invoke-Sqlcmd -ConnectionString $connectionString -Query $query
“`
This script connects to your SQL Server and executes the SQL query, returning results in a more automated fashion.
Considerations and Best Practices
When searching for text in stored procedures, consider the following:
- Performance: Searching large databases can be resource-intensive. Limit your search scope if possible.
- Text Variations: Be aware of variations in text (e.g., casing, spacing) that might affect your search results.
- Backup: Always ensure you have backups before performing extensive searches or modifications to database objects.
By employing these methods, you can effectively find and manage text within stored procedures in SQL Server.
Expert Insights on Finding Text in SQL Server Stored Procedures
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Finding specific text within stored procedures in SQL Server can be crucial for maintaining code quality and ensuring compliance. Utilizing the `sys.sql_modules` system view allows developers to search for text efficiently, ensuring that they can quickly identify dependencies and potential issues within their database code.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “I recommend using the `OBJECT_DEFINITION` function in conjunction with `LIKE` queries to pinpoint specific text in stored procedures. This method not only enhances the search process but also improves performance by reducing the need to manually sift through each procedure.”
Linda Zhang (Database Consultant, OptimizeDB). “For organizations dealing with large databases, implementing a script that iterates through all stored procedures and searches for the desired text can save significant time. This approach allows for batch processing and can be automated to run at regular intervals, ensuring that any changes to the procedures are monitored effectively.”
Frequently Asked Questions (FAQs)
How can I search for a specific text within stored procedures in SQL Server?
You can use the `sys.sql_modules` system view along with the `OBJECT_DEFINITION` function. For example:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%YourSearchText%’;
“`
Is there a way to find all stored procedures containing a certain keyword?
Yes, you can utilize the same query structure as above, replacing `YourSearchText` with the desired keyword. This will return all stored procedures that include that keyword in their definition.
Can I search for text in both stored procedures and functions simultaneously?
Yes, you can search in both stored procedures and functions by querying the `sys.sql_modules` view without filtering by type. This will include all objects that contain the specified text.
What SQL Server versions support the method to find text in stored procedures?
The method using `sys.sql_modules` and `OBJECT_DEFINITION` is supported in SQL Server 2005 and later versions.
Are there any performance considerations when searching for text in large databases?
Yes, searching through large databases may impact performance. It is advisable to run such queries during off-peak hours and to limit the search scope if possible.
Can I automate the process of finding text in stored procedures?
Yes, you can create a stored procedure or a SQL Server Agent job that executes the search query periodically, allowing for automated monitoring of stored procedure definitions.
In SQL Server, finding specific text within stored procedures is a crucial task for database administrators and developers. This process can be essential for various reasons, including code maintenance, debugging, and ensuring compliance with coding standards. SQL Server provides several methods to search for text in stored procedures, including system catalog views, dynamic management views, and the use of built-in functions. Utilizing these tools effectively can streamline the process of identifying and modifying stored procedures that contain particular keywords or phrases.
One of the most commonly used methods to find text in stored procedures is querying the `sys.sql_modules` system view. This view contains definitions of all SQL Server objects, including stored procedures. By executing a simple SQL query that filters on the `definition` column, users can quickly locate specific text within their stored procedures. Additionally, the `OBJECT_DEFINITION` function can be employed to retrieve the definition of a specific object, allowing for targeted searches.
Another valuable approach involves leveraging SQL Server Management Studio (SSMS) features, such as the “Find in Files” option. This feature enables users to search across multiple stored procedures and other database objects simultaneously, enhancing efficiency in locating the desired text. Furthermore, understanding the implications of modifying stored procedures is important, as changes
Author Profile
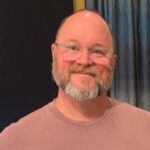
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?