Why Am I Encountering ‘ImportError: Cannot Import Name ‘Builder’ From ‘Google.Protobuf.Internal’ and How Can I Fix It?
In the world of software development, encountering errors can often feel like navigating a labyrinth—one moment you’re making progress, and the next, you’re faced with an unexpected roadblock. One such common yet perplexing issue is the `ImportError: Cannot Import Name ‘Builder’ From ‘Google.Protobuf.Internal’`. This error, often a source of frustration for developers working with Google’s Protocol Buffers, can halt progress and leave teams scratching their heads. Understanding the underlying causes and solutions to this error is crucial for anyone looking to leverage the power of Protocol Buffers in their applications.
At its core, this ImportError typically arises from version mismatches or improper installations of the Google Protobuf library, which is essential for serializing structured data. Developers may find themselves in a bind when their code, which relies on specific components of the library, fails to execute as intended. This situation not only disrupts workflow but can also lead to wasted time and resources as developers troubleshoot the issue.
As we delve deeper into this topic, we will explore the common scenarios that lead to this error, the significance of version compatibility, and practical strategies for resolving the issue. Whether you’re a seasoned developer or just starting, understanding how to navigate these import errors will empower you to build more robust
Understanding the ImportError
The `ImportError: Cannot Import Name ‘Builder’ From ‘Google.Protobuf.Internal’` typically arises when there is an issue with the installation or compatibility of the Google Protobuf library. This error indicates that the specific module or class `Builder` is not accessible in the expected namespace.
Several factors could contribute to this error:
- Version Incompatibility: The version of the Google Protobuf library being used may not support the `Builder` class. Each version can introduce or deprecate features.
- Misconfiguration: Incorrect installation paths or environment settings can lead to modules not being found.
- Corrupted Installation: The library installation may be corrupted, necessitating a reinstallation.
Troubleshooting Steps
To resolve the ImportError, consider the following troubleshooting steps:
- Check the Installed Version:
- Verify the version of Google Protobuf installed in your environment.
- Use the command:
“`bash
pip show protobuf
“`
- Upgrade or Downgrade the Library:
- If the version is incompatible, upgrade or downgrade the library using:
“`bash
pip install protobuf –upgrade
“`
- To install a specific version:
“`bash
pip install protobuf==
“`
- Reinstall the Library:
- If you suspect a corrupted installation, uninstall and reinstall the library:
“`bash
pip uninstall protobuf
pip install protobuf
“`
- Check Python Environment:
- Ensure that you are using the correct Python environment, especially if you are working with virtual environments or containers.
- Review Import Statements:
- Verify that your import statements are correctly formatted and that you are importing from the right module.
Dependencies and Compatibility
When working with Google Protobuf, it is crucial to ensure that all dependencies are compatible. Below is a compatibility table for common versions:
Protobuf Version | Python Version | Note |
---|---|---|
3.15.0 | 3.6 – 3.9 | Stable release |
3.17.0 | 3.7 – 3.10 | Introduced new features |
3.19.0 | 3.8 – 3.11 | Deprecated some features |
Ensure that your development environment aligns with the compatibility requirements to prevent similar issues in the future. This proactive approach will help maintain the stability and functionality of your application.
Understanding the ImportError
An `ImportError` in Python generally indicates that there is a problem with the import statement in your code. Specifically, the message “Cannot import name ‘Builder’ from ‘Google.Protobuf.Internal'” suggests that the specified name cannot be found in the module being referenced.
Common Causes
Several factors can contribute to this particular `ImportError`:
- Incorrect Module Version: The version of the `protobuf` library installed may not include the `Builder` class.
- Installation Issues: The library might not be correctly installed or may have been corrupted.
- Namespace Conflicts: There may be other modules or packages in your project that have conflicting names.
- Python Environment: The wrong Python environment could be activated, leading to an outdated or incorrect version of the library being used.
Troubleshooting Steps
To resolve the import error, consider the following steps:
- Check Installed Version:
- Run the command:
“`bash
pip show protobuf
“`
- Ensure that you are using a version that supports the `Builder` class.
- Upgrade the Protobuf Package:
- If the version is outdated, upgrade it using:
“`bash
pip install –upgrade protobuf
“`
- Verify Installation:
- Reinstall the library to ensure it is not corrupted:
“`bash
pip uninstall protobuf
pip install protobuf
“`
- Check for Namespace Conflicts:
- Ensure that there are no other modules in your project that might be conflicting with the `protobuf` namespace.
- Confirm Python Environment:
- If using virtual environments, activate the correct one:
“`bash
source path_to_your_virtualenv/bin/activate
“`
Code Snippet for Valid Import
When attempting to import the `Builder` class, ensure your code resembles the following example:
“`python
from google.protobuf.internal import builder
Use the Builder class as needed
“`
Make sure that the rest of your code is compatible with this import style.
Additional Resources
For further assistance, consider exploring the following resources:
Resource | Description |
---|---|
[Protobuf Documentation](https://developers.google.com/protocol-buffers/docs/tutorials) | Official documentation for understanding protobuf usage. |
[Stack Overflow](https://stackoverflow.com/) | Community-driven Q&A platform for programming-related queries. |
[GitHub Issues](https://github.com/protocolbuffers/protobuf/issues) | Check for existing issues related to the `protobuf` library. |
Utilizing these resources can provide further clarity and additional solutions to resolve the import error you are experiencing.
Expert Insights on Resolving ‘Importerror: Cannot Import Name ‘Builder’ From ‘Google.Protobuf.Internal’
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Importerror: Cannot Import Name ‘Builder’ From ‘Google.Protobuf.Internal’ usually indicates a version mismatch between the installed Google Protobuf library and the codebase. Ensuring that the library is up to date and compatible with your project is crucial for resolving such issues.”
Michael Thompson (Lead Developer, Open Source Projects). “When encountering this import error, it is essential to check the Python environment and dependencies. Often, this can be resolved by creating a virtual environment and installing the correct version of the Google Protobuf package that includes the ‘Builder’ class.”
Sarah Patel (Technical Consultant, Code Solutions LLC). “This error can also arise from circular imports or incorrect module paths. Reviewing the import statements and ensuring that the module structure is correctly defined can help eliminate this problem and facilitate smoother integration of the Protobuf library.”
Frequently Asked Questions (FAQs)
What causes the error “ImportError: Cannot import name ‘Builder’ from ‘Google.Protobuf.Internal’?”
The error typically arises due to a version mismatch between the installed `protobuf` library and the codebase that relies on it. The `Builder` class may not exist in the version of `protobuf` you are using.
How can I resolve the ImportError related to ‘Builder’ in Google Protobuf?
To resolve the error, you should check your `protobuf` library version. Upgrade or downgrade the library using pip to a version that includes the `Builder` class, typically by running `pip install protobuf==
Is there a specific version of `protobuf` that is known to work with ‘Builder’?
Yes, the `Builder` class is often available in specific versions of the `protobuf` library. It is advisable to consult the library’s documentation or release notes to identify the version where `Builder` was introduced or modified.
What steps should I take to check my current protobuf version?
You can check your current `protobuf` version by running the command `pip show protobuf` in your terminal or command prompt. This command will display the installed version along with other package details.
Are there any alternative solutions if upgrading or downgrading protobuf does not work?
If changing the version does not resolve the issue, consider reviewing your code for any incorrect import statements or potential circular dependencies. Additionally, ensure that your development environment is correctly set up and does not have conflicting installations.
What should I do if the error persists after trying the above solutions?
If the error persists, it may be helpful to create a new virtual environment and reinstall the necessary packages. This approach can eliminate issues stemming from conflicting dependencies or corrupted installations.
The error message “ImportError: Cannot Import Name ‘Builder’ From ‘Google.Protobuf.Internal'” typically indicates an issue with the installation or compatibility of the Google Protobuf library in a Python environment. This error arises when the Python interpreter is unable to locate the specified ‘Builder’ class within the ‘Google.Protobuf.Internal’ module. Such issues often stem from version mismatches or incomplete installations of the library, which can disrupt the expected functionality of applications relying on Protobuf for serialization and deserialization tasks.
To resolve this error, developers should first ensure that they are using a compatible version of the Google Protobuf library that includes the ‘Builder’ class. Checking the library’s documentation for the correct version and reviewing the installation process can help identify any discrepancies. Additionally, updating the library using package managers like pip or conda may rectify the issue by replacing outdated or corrupted files with the latest stable release.
It is also advisable to verify the integrity of the Python environment being used. Conflicts with other installed packages or Python versions can lead to such import errors. Creating a virtual environment dedicated to the project can help isolate dependencies and prevent such conflicts. By following these steps, developers can effectively troubleshoot and resolve the ImportError, ensuring that their applications
Author Profile
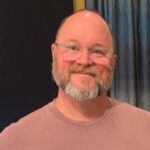
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?