Why Am I Seeing ‘TypeError: ‘set’ Object Is Not Subscriptable’ and How Can I Fix It?
In the world of programming, encountering errors is an inevitable part of the journey. Among the myriad of error messages that can pop up during development, the `TypeError: ‘set’ object is not subscriptable` stands out as a common yet perplexing issue for many Python developers. This error serves as a reminder of the intricacies of data types and their behaviors within the Python language. As you delve into the world of sets, indexing, and the nuances of Python’s type system, you’ll uncover not only the cause of this error but also the best practices for avoiding it in the future.
Understanding why this error occurs requires a closer look at the fundamental characteristics of sets in Python. Unlike lists or tuples, sets are unordered collections that do not support indexing, slicing, or other sequence-like behavior. This means that attempting to access elements in a set using square brackets will lead to the infamous TypeError. As we explore the intricacies of this error, we will also discuss the broader implications it has on data manipulation and the importance of choosing the right data structures for your programming needs.
By the end of this article, you’ll have a clearer understanding of what it means for an object to be subscriptable, how to effectively work with sets, and strategies to
Understanding the Error
The error message `TypeError: ‘set’ object is not subscriptable` occurs in Python when an attempt is made to access an element of a set using indexing or slicing. Unlike lists or tuples, sets are unordered collections of unique elements, and therefore do not support indexing.
Key characteristics of sets include:
- Unordered: The elements in a set do not have a defined order.
- Unique: Each element in a set is unique; duplicates are not allowed.
- Mutable: Sets can be modified after their creation, allowing for the addition or removal of elements.
This error typically arises in scenarios where a programmer mistakenly treats a set like a list. Below are common situations leading to this error:
- Attempting to access a specific element using bracket notation, e.g., `my_set[0]`.
- Trying to slice a set with a colon, e.g., `my_set[1:3]`.
Examples of the Error
To illustrate the occurrence of this error, consider the following code snippets:
“`python
Example 1: Accessing an element incorrectly
my_set = {1, 2, 3, 4}
print(my_set[0]) Raises TypeError: ‘set’ object is not subscriptable
“`
“`python
Example 2: Slicing a set incorrectly
my_set = {1, 2, 3, 4}
print(my_set[1:3]) Raises TypeError: ‘set’ object is not subscriptable
“`
In both examples, the programmer attempts to access elements of a set as if it were a list, leading to the `TypeError`.
How to Access Set Elements
To work with elements in a set, one must use methods that are appropriate for set data structures. Here are some alternatives for accessing elements:
- Using a loop: Iterate through the set to access each element.
“`python
for element in my_set:
print(element) Prints each element in the set
“`
- Converting to a list: If indexing is necessary, convert the set to a list first.
“`python
my_list = list(my_set)
print(my_list[0]) Accesses the first element after conversion
“`
Best Practices
To avoid encountering the `TypeError: ‘set’ object is not subscriptable`, adhere to these best practices:
- Always remember that sets do not maintain any order.
- Use loops or comprehensions to handle sets.
- Convert to a list only when indexing is essential.
Data Type | Subscriptable | Example Access |
---|---|---|
List | Yes | my_list[0] |
Tuple | Yes | my_tuple[1] |
Set | No | my_set[0] (raises TypeError) |
By following these guidelines, developers can effectively manage sets in Python and avoid common pitfalls associated with data type misusage.
Understanding the TypeError
The error message `TypeError: ‘set’ object is not subscriptable` occurs when an attempt is made to access elements of a set using indexing or slicing, which is not allowed in Python. Sets are unordered collections of unique elements, and they do not support indexing like lists or tuples.
Common Causes of the Error
- Indexing a Set: Trying to retrieve an element using square brackets.
- Slicing a Set: Attempting to slice a set to obtain a subset of its elements.
Example
“`python
my_set = {1, 2, 3}
print(my_set[0]) This will raise TypeError
“`
In this example, accessing `my_set[0]` raises a `TypeError` because sets do not allow for direct indexing.
How to Access Set Elements
To work with individual elements within a set, utilize methods that are designed for this purpose:
- Iterate through the set: Use a loop to access each element.
- Convert to a list: If indexing is necessary, convert the set to a list first.
Iteration Example
“`python
for item in my_set:
print(item)
“`
Conversion Example
“`python
my_list = list(my_set)
print(my_list[0]) Accessing the first element after conversion
“`
Alternative Data Structures
If you require indexing capabilities, consider using other data structures that support subscripting:
Data Structure | Description |
---|---|
List | Ordered collection that allows duplicates. |
Tuple | Ordered, immutable collection that allows duplicates. |
Dictionary | Unordered collection of key-value pairs. |
Each of these structures has its own use cases and should be chosen based on the needs of your application.
Best Practices to Avoid the Error
To prevent encountering this error in your code, follow these guidelines:
- Understand Data Types: Familiarize yourself with the characteristics of sets and their limitations.
- Use Appropriate Structures: Choose the right data structure based on your requirements for indexing and order.
- Error Handling: Implement error handling using try-except blocks to gracefully manage unexpected type errors.
Example of Error Handling
“`python
try:
print(my_set[0])
except TypeError:
print(“Cannot access elements of a set using indexing.”)
“`
By implementing these practices, you can effectively avoid the `TypeError: ‘set’ object is not subscriptable` and ensure that your code runs smoothly.
Understanding the ‘set’ Object Subscriptability Error in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘TypeError: ‘set’ object is not subscriptable’ occurs because sets in Python are unordered collections of unique elements. Unlike lists or tuples, sets do not support indexing, which leads to this common mistake among developers who attempt to access elements using square brackets.”
James Liu (Python Developer and Educator, CodeMaster Academy). “To resolve the ‘set’ object is not subscriptable error, developers should consider converting the set to a list or tuple if they need to access elements by index. This can be achieved using the built-in list() or tuple() functions, allowing for indexed access without encountering this error.”
Dr. Sarah Thompson (Data Scientist, Analytics Hub). “It’s crucial for programmers to understand the data structures they are working with. Sets are designed for membership testing and eliminating duplicates, not for ordered access. Recognizing the intended use of a set can prevent such errors and lead to more efficient code design.”
Frequently Asked Questions (FAQs)
What does the error ‘TypeError: ‘set’ object is not subscriptable’ mean?
This error indicates that you are attempting to access an element of a set using indexing or slicing, which is not allowed in Python. Sets are unordered collections and do not support indexing.
How can I resolve the ‘TypeError: ‘set’ object is not subscriptable’ error?
To resolve this error, you can convert the set to a list or tuple using the `list()` or `tuple()` functions, respectively, which do support indexing. For example, `list(my_set)[0]` retrieves the first element.
Can I access elements of a set directly using a loop?
Yes, you can iterate over a set using a loop. For example, you can use a `for` loop to access each element without needing to use indexing.
What are the common scenarios that lead to this error?
Common scenarios include mistakenly trying to access a set element with an index, such as `my_set[0]`, or when using a set in a context that expects a subscriptable object, like a list or dictionary.
Is it possible to convert a set to a list while maintaining order?
Sets are inherently unordered, so converting a set to a list will not maintain any specific order. If order is important, consider using a list or an `OrderedDict` from the `collections` module instead.
What should I do if I need to check membership in a set?
You can check for membership in a set using the `in` keyword. For example, `if element in my_set:` will efficiently determine if the element exists in the set without the need for indexing.
The error message “TypeError: ‘set’ object is not subscriptable” typically arises in Python when a programmer attempts to access elements of a set using indexing or slicing. Unlike lists or tuples, sets are unordered collections of unique elements, which means they do not support indexing. This fundamental characteristic of sets is crucial for developers to understand to avoid common pitfalls in their code.
To resolve this error, developers should consider the intended operation on the set. If the goal is to retrieve a specific element, it is advisable to convert the set to a list or tuple first, using functions like `list()` or `tuple()`. Alternatively, if the operation involves checking membership or iterating through the set, using methods such as `in` or a loop would be more appropriate, as these are designed to work with the nature of sets.
In summary, the “TypeError: ‘set’ object is not subscriptable” serves as a reminder of the distinct characteristics of data structures in Python. Understanding these differences is essential for writing efficient and error-free code. By adhering to the correct methods for manipulating sets, developers can enhance their programming skills and avoid similar errors in the future.
Author Profile
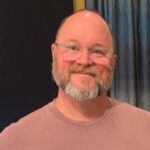
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?