What Does ‘Return’ Mean in Python: Key Insights and Common Questions Answered
In the world of programming, Python stands out as a versatile and user-friendly language, making it a favorite among both beginners and seasoned developers. One of the fundamental concepts that underpins Python’s functionality is the notion of “return.” For those diving into the depths of Python programming, understanding what return means is essential for mastering how functions operate and how data flows within your code. This article will unravel the significance of the return statement, illuminating its role in enhancing the efficiency and clarity of your programming endeavors.
At its core, the return statement serves as a pivotal mechanism for functions, allowing them to output values back to the part of the program that called them. This simple yet powerful feature enables developers to encapsulate logic within functions, promoting code reusability and organization. When a function executes a return statement, it effectively hands off a result, which can then be utilized elsewhere in the program, thus streamlining processes and facilitating complex computations.
Moreover, the return statement is not just about sending values back; it also plays a critical role in controlling the flow of execution within a program. By determining when a function should cease its operations and provide an output, return statements help manage the overall structure and behavior of code. As we delve deeper into the intricacies of return in Python, we
Understanding the Return Statement
The `return` statement in Python is a fundamental component of functions, serving to exit the function and pass back a value to the caller. When a function is called, it executes its block of code, and when the `return` statement is encountered, it not only terminates the function but also sends a specified value back to the point where the function was called.
Consider the following points regarding the `return` statement:
- The `return` statement can return any type of Python object, including integers, strings, lists, dictionaries, or even other functions.
- If a function does not explicitly return a value using `return`, it implicitly returns `None`.
- A function can have multiple `return` statements, but only one will be executed during a single call to the function.
Syntax of the Return Statement
The syntax of the `return` statement is straightforward:
“`python
return [expression]
“`
Here, `expression` is optional. If provided, it is evaluated, and its value is returned. If omitted, the function returns `None`.
Examples of Return in Python
To illustrate how the `return` statement works, consider the following examples:
“`python
def add(a, b):
return a + b
result = add(5, 3)
print(result) Output: 8
“`
In this example, the `add` function takes two parameters, adds them together, and returns the result. The returned value is then stored in the variable `result`.
Another example with multiple return paths:
“`python
def check_number(num):
if num > 0:
return “Positive”
elif num < 0:
return "Negative"
else:
return "Zero"
status = check_number(-5)
print(status) Output: Negative
```
In this case, the function `check_number` returns different strings based on the value of `num`.
Return Values: A Detailed Breakdown
The following table summarizes the behavior of the `return` statement in different scenarios:
Scenario | Return Statement | Returned Value |
---|---|---|
Returning a value | return 10 | 10 |
Returning a string | return “Hello” | “Hello” |
No return statement | None | None |
Returning multiple values | return 1, 2, 3 | (1, 2, 3) |
In the last row of the table, note that returning multiple values from a function actually returns a tuple. This can be unpacked into individual variables:
“`python
x, y, z = return_multiple_values()
“`
Returning from Nested Functions
The `return` statement can also be used within nested functions. The returned value from an inner function can be passed back to the outer function. Here’s an example:
“`python
def outer_function():
def inner_function():
return “Hello from inner”
result = inner_function()
return result
message = outer_function()
print(message) Output: Hello from inner
“`
In this case, `inner_function` returns a string, which is then returned by `outer_function`, demonstrating how `return` works across different levels of function calls.
The `return` statement is a powerful tool in Python, allowing developers to manage the flow of data and control the output of functions effectively.
Understanding the Return Statement
The `return` statement in Python is a crucial element in function definitions. It is used to exit a function and optionally pass an expression back to the caller. This allows functions to produce output that can be utilized elsewhere in the program.
When a function is called, the execution of the function begins, and when a `return` statement is encountered, the function stops executing. The value provided in the `return` statement is sent back to the point where the function was called.
Syntax of Return Statement
The basic syntax of the return statement is as follows:
“`python
def function_name(parameters):
function body
return expression
“`
Characteristics of Return
- Single Return Value: A function can return only one value. However, this value can be a collection, such as a list or tuple, allowing multiple values to be grouped together.
- Optional Return: If no `return` statement is specified, the function will return `None` by default.
- Immediate Termination: The `return` statement immediately terminates the function, meaning that any code following a `return` statement within the same function will not be executed.
Examples
Here are a few examples demonstrating the use of the return statement:
“`python
def add(a, b):
return a + b
result = add(3, 5) result is now 8
“`
“`python
def get_data():
return [1, 2, 3, 4]
data = get_data() data is now [1, 2, 3, 4]
“`
Multiple Return Values
Python supports returning multiple values packed in a tuple. This is achieved simply by separating the values with commas:
“`python
def divide(x, y):
if y == 0:
return None, “Error: Division by zero”
return x / y, “Success”
result, message = divide(10, 2) result is 5.0, message is “Success”
“`
Return in Recursive Functions
In recursive functions, the `return` statement is used to send the result of the recursive call back to the previous invocation of the function.
“`python
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n – 1)
result = factorial(5) result is 120
“`
Return vs Print
It’s important to distinguish between `return` and `print`. The `print` function outputs data to the console, whereas `return` sends data back to the caller:
- Return: Provides output to the calling context, useful for further processing.
- Print: Displays output directly to the console, primarily for user interaction or debugging.
Feature | Return | |
---|---|---|
Purpose | Send value back to caller | Display value on console |
Output Type | Value | None |
Usage Context | Function definitions | Debugging, user messages |
Conclusion
Understanding the `return` statement is essential for effective programming in Python. It allows for the creation of functions that not only perform actions but also provide valuable outputs for further computation or display.
Understanding the Role of Return in Python Programming
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “The ‘return’ statement in Python is crucial for defining the output of a function. It allows developers to send back a value to the caller, enabling modular programming and code reusability. Without ‘return’, functions would not be able to convey results effectively.”
Michael Torres (Python Developer Advocate, CodeCraft). “In Python, the ‘return’ keyword not only exits a function but also specifies what value is sent back. This behavior is fundamental for creating functions that can perform calculations or transformations and then provide results, which is essential for building dynamic applications.”
Sarah Patel (Lead Educator, Python Programming Academy). “Understanding how ‘return’ works in Python is vital for beginners. It distinguishes between procedures that perform actions and functions that compute values. Mastering ‘return’ is a stepping stone toward writing effective and efficient Python code.”
Frequently Asked Questions (FAQs)
What does the `return` statement do in Python?
The `return` statement in Python is used to exit a function and send a value back to the caller. It effectively terminates the function’s execution and can return a single value, multiple values as a tuple, or no value at all.
Can a function have multiple return statements in Python?
Yes, a function can have multiple return statements. The execution will stop at the first `return` statement encountered, and the function will return the specified value immediately.
What happens if a function does not have a return statement?
If a function does not contain a return statement, it implicitly returns `None`. This indicates that the function completed its execution without returning any specific value.
How do you return multiple values from a function in Python?
To return multiple values from a function in Python, you can separate the values with commas in the `return` statement. Python will return these values as a tuple, which can be unpacked when the function is called.
Is it possible to return a function from another function in Python?
Yes, it is possible to return a function from another function in Python. This is commonly done in higher-order functions, allowing for the creation of closures or decorators.
What is the difference between `return` and `print` in Python?
The `return` statement sends a value back to the caller of a function, while `print` outputs a value to the console. `return` affects the function’s output, whereas `print` is primarily used for displaying information to the user.
The keyword “return” in Python serves a fundamental role in defining the output of a function. When a function is executed, the return statement allows the function to send back a value to the caller. This value can be of any data type, including integers, strings, lists, or even other functions. The use of return is essential for creating reusable code, as it enables functions to produce results that can be utilized in various parts of a program.
Moreover, the return statement also influences the flow of execution within a program. Once a return statement is encountered, the function terminates immediately, and control is passed back to the point where the function was called. This behavior is crucial for managing the program’s logic and ensuring that functions perform their intended tasks without unnecessary processing after the desired output has been achieved.
In summary, understanding the return keyword is vital for effective Python programming. It not only facilitates the output of function results but also helps in structuring code for better readability and maintainability. By leveraging the return statement appropriately, developers can create functions that are both efficient and easy to understand, ultimately leading to more robust software development practices.
Author Profile
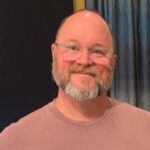
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?