Why Am I Encountering the ‘Type Object Is Not Subscriptable’ Error in Python?
In the world of programming, encountering errors is an inevitable part of the development process. One such error that often leaves developers scratching their heads is the infamous “Type’ object is not subscriptable” message. This cryptic phrase can halt your code in its tracks, leaving you to decipher its meaning and implications. Whether you are a seasoned programmer or a newcomer to the coding landscape, understanding this error is crucial for debugging and enhancing your coding skills.
At its core, the “Type’ object is not subscriptable” error typically arises in Python when you attempt to access an element of a non-subscriptable object, such as a class or a data type, as if it were a list or a dictionary. This misstep can occur in various scenarios, from simple typos to more complex misunderstandings of how data types function within your code. As you delve deeper into this topic, you’ll discover the common pitfalls that lead to this error and the best practices for avoiding it in your own projects.
In the following sections, we will explore the underlying causes of this error, provide illustrative examples, and offer practical solutions to help you navigate through the confusion. By the end of this article, you’ll be equipped with the knowledge to tackle this error head-on,
Understanding the TypeError
The error message `Type’ object is not subscriptable` typically occurs in Python when you try to index or slice an object that is not designed to be accessed in this manner. This situation often arises with built-in types or classes that do not support the subscript operation (i.e., using square brackets `[]`).
Common scenarios where this error can occur include:
- Attempting to access an element of a class instance that does not implement the `__getitem__` method.
- Using a type instead of an instance of that type.
- Forgetting to instantiate a list or dictionary before trying to access its elements.
For instance, consider the following code snippet:
“`python
class MyClass:
pass
obj = MyClass
value = obj[0] This will raise TypeError
“`
In this example, `obj` is a class, and attempting to use subscript notation on it generates the `TypeError`.
Common Causes of the Error
Identifying the root cause of the `Type’ object is not subscriptable` error is essential for debugging. Here are some prevalent causes:
- Using Class Names Instead of Instances: If you mistakenly use a class name without instantiating it, you will encounter this error. Ensure that you create an object of the class before trying to access its attributes or methods.
- Incorrectly Structured Data Types: When working with complex data structures, ensure that you are accessing them correctly. For example, if you expect a list but accidentally pass a type, you will trigger the error.
- Function Return Values: Sometimes, functions return types instead of instances. Always check the return type of a function if you suspect an issue.
How to Fix the Error
To resolve the `Type’ object is not subscriptable` error, follow these steps:
- Check Object Types: Use the `type()` function to verify the object type before subscripting.
- Instantiate Classes: Ensure that any class you are trying to use has been instantiated.
- Debugging Output: Utilize print statements or logging to trace variable values and types leading up to the error.
- Refactor Code: If necessary, refactor your code to ensure that you are working with the correct object types.
Here’s an example of correcting the previous code:
“`python
class MyClass:
def __init__(self):
self.data = [1, 2, 3]
obj = MyClass() Properly instantiate the class
value = obj.data[0] Now this works
“`
Best Practices
To minimize the chances of encountering the `Type’ object is not subscriptable` error, adhere to the following best practices:
- Use Type Annotations: Implement type hints to clarify what types are expected in your functions.
- Implement `__getitem__`: For custom classes where indexing is desirable, implement the `__getitem__` method.
- Unit Testing: Write tests that cover various scenarios to catch errors early in the development process.
- Code Reviews: Engage in peer reviews to ensure object types are correctly used throughout your code.
Cause | Solution |
---|---|
Using class instead of an instance | Instantiate the class before subscripting |
Accessing a non-iterable type | Check the type before access |
Incorrect function return type | Verify the return type of functions |
Understanding the Error: ‘Type’ Object Is Not Subscriptable
The error message `’Type’ object is not subscriptable` typically arises in Python when attempting to index or slice a type that is not designed to be subscripted. This situation often stems from a misunderstanding of data types and their properties.
Common Causes
- Using a Type Instead of an Instance:
- Attempting to access an element from a class or a built-in type directly instead of its instance.
- Example:
“`python
class MyClass:
pass
instance = MyClass
value = instance[0] Raises TypeError
“`
- Misusing Built-in Functions:
- Trying to subscript a built-in type (like `int`, `str`, or `list`) instead of an instance of that type.
- Example:
“`python
number = int
first_digit = number[0] Raises TypeError
“`
- Reassigning Variables:
- Overwriting a variable that was originally a subscriptable type (like a list or string) with a non-subscriptable type.
- Example:
“`python
my_list = [1, 2, 3]
my_list = str Now my_list is a type, not a list
element = my_list[0] Raises TypeError
“`
How to Resolve the Error
To address this error, follow these steps:
- Verify Variable Types:
- Ensure that the variable you are trying to subscript is indeed an instance of a subscriptable type.
- Check for Reassignments:
- Look for any lines of code that may have reassigned your variable to a non-subscriptable type.
- Use Correct Instances:
- Always ensure you are working with an instance of a class or a built-in type.
Examples of Correct Usage
Here are some examples that demonstrate proper use of subscriptable types:
Example Code | Explanation |
---|---|
`my_list = [1, 2, 3]` | A list is created. |
`element = my_list[0]` | Correctly accesses the first element (1). |
`my_string = “hello”` | A string is created. |
`char = my_string[1]` | Correctly accesses the second character (‘e’). |
Debugging Tips
- Use Print Statements:
- Print the type of the variable before subscript to identify its nature:
“`python
print(type(my_variable))
“`
- Check Tracebacks:
- Pay close attention to the traceback provided by Python, as it will point to the exact line where the error occurs.
By understanding the context and reasons behind the `’Type’ object is not subscriptable` error, you can effectively diagnose and correct the underlying issues in your Python code.
Understanding the ‘Type’ Object Is Not Subscriptable Error in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “The ‘Type’ object is not subscriptable error typically arises when a developer attempts to access elements of a type object as if it were a list or a dictionary. It is crucial to ensure that the variable being accessed is indeed a data structure that supports indexing.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “This error often indicates a misunderstanding of Python’s type system. Developers should familiarize themselves with the difference between types and instances, as trying to subscript a type directly can lead to confusion and bugs in the code.”
Linda Garcia (Python Educator, LearnPythonNow). “To resolve the ‘Type’ object is not subscriptable error, one should review the code to identify where a type is being incorrectly referenced. Utilizing type hints and static type checkers can significantly reduce the occurrence of such errors.”
Frequently Asked Questions (FAQs)
What does the error ‘Type’ object is not subscriptable’ mean?
This error indicates that you are trying to access an element of a type object as if it were a subscriptable collection, such as a list or dictionary. Type objects in Python cannot be indexed or sliced.
What causes the ‘Type’ object is not subscriptable error?
This error typically occurs when you mistakenly attempt to index a type rather than an instance of that type. For example, using `MyClass[0]` instead of creating an instance of `MyClass` first.
How can I fix the ‘Type’ object is not subscriptable error?
To resolve this error, ensure that you are accessing an instance of a class or a data structure that supports subscripting. Verify that you are not trying to index a class itself.
Can this error occur with built-in types?
Yes, this error can occur with built-in types if you mistakenly reference the type itself instead of an instance. For example, using `list[0]` instead of creating a list instance.
Are there any common scenarios where this error appears?
Common scenarios include trying to access elements of a class directly, using type names instead of instances, or misusing decorators that return type objects rather than instances.
How can I debug this error effectively?
To debug this error, check the line number indicated in the traceback, review the variable types involved, and ensure that you are working with instances of classes or collections that can be subscripted.
The error message “Type’ object is not subscriptable” typically arises in Python programming when an attempt is made to access an element of a type object as if it were a subscriptable collection, such as a list or dictionary. This often occurs when developers mistakenly use a class name instead of an instance of that class. Understanding the distinction between types and instances is crucial for avoiding this common pitfall.
In many cases, this error can be traced back to a misunderstanding of how to properly instantiate objects or access their attributes. For instance, using the class name directly to attempt to index into it, rather than creating an instance of that class first, leads to this error. Developers should ensure they are working with instances of objects when attempting to access their properties or methods.
To prevent encountering the “Type’ object is not subscriptable” error, it is advisable to review the code for instances where class names are mistakenly used in place of object instances. Additionally, implementing thorough debugging practices and utilizing type hints can help clarify the intended usage of types and instances. By adhering to these practices, programmers can enhance their code’s reliability and reduce the likelihood of encountering such errors in the future.
Author Profile
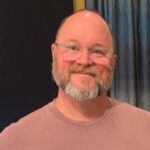
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?