How Can You Add to the Fillable Property in Laravel to Enable Mass Assignment?
In the dynamic world of web development, Laravel stands out as a powerful PHP framework that streamlines the process of building robust applications. One of its most essential features is the Eloquent ORM, which provides an elegant and expressive way to interact with your database. However, as developers harness the power of Eloquent for mass assignment, they must navigate the intricacies of the fillable property. Understanding how to effectively add attributes to the fillable array is crucial for ensuring that your application remains secure while maximizing its functionality. In this article, we will explore the significance of the fillable property in Laravel and guide you through the process of allowing mass assignment on your models.
Mass assignment is a powerful feature that allows developers to create or update multiple attributes of a model in a single operation, significantly enhancing productivity. However, it also introduces potential security risks if not managed properly. By design, Laravel requires you to explicitly define which attributes are mass assignable to prevent unintentional data manipulation. This is where the fillable property comes into play. By adding attributes to this array, you can control which fields can be mass assigned, ensuring that only the intended data is processed.
As we delve deeper into the topic, we will discuss best practices for managing the fillable property, the
Add To Fillable Property
In Laravel, mass assignment is a convenient way to create or update multiple attributes on a model in a single operation. However, to protect against mass assignment vulnerabilities, you must explicitly define which attributes are “fillable.” This is done by adding the attributes to the `$fillable` property of your model.
To allow mass assignment on a model, follow these steps:
- Open your model file, typically located in the `app/Models` directory.
- Define the `$fillable` property as an array containing the names of the attributes you wish to allow for mass assignment.
For example:
“`php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
protected $fillable = [‘title’, ‘content’, ‘author_id’];
}
“`
In this example, the `Post` model allows mass assignment for the `title`, `content`, and `author_id` attributes. This means you can create a new post or update an existing one using an associative array containing these keys.
Benefits of Using Fillable Property
Using the fillable property provides several advantages:
- Security: It prevents mass assignment vulnerabilities by ensuring only specified attributes can be mass assigned.
- Clarity: It makes your code clearer by explicitly stating which attributes are intended for mass assignment.
- Maintainability: It simplifies changes to your model attributes over time, as you can easily modify the fillable array.
Alternative: Guarded Property
In addition to `$fillable`, Laravel provides a `$guarded` property that serves the opposite purpose. Instead of specifying which attributes are mass assignable, you can define which attributes should not be mass assignable.
Example:
“`php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $guarded = [‘id’, ‘created_at’, ‘updated_at’];
}
“`
In this case, all attributes except `id`, `created_at`, and `updated_at` are mass assignable. This method can be useful when you have many attributes and only a few that should be protected.
Best Practices
When using the fillable property, consider the following best practices:
- Always define the fillable attributes explicitly to enhance security.
- Avoid using the guarded property unless necessary to prevent accidental mass assignment of sensitive fields.
- Regularly review and update the fillable attributes as your application evolves.
Property | Description | Use Case |
---|---|---|
$fillable | Array of attributes that are mass assignable. | When you want to specify allowed attributes. |
$guarded | Array of attributes that are not mass assignable. | When most attributes are allowed except a few. |
Adopting these practices will help ensure that your application remains secure while taking advantage of Laravel’s powerful mass assignment features.
Understanding the Fillable Property in Laravel
In Laravel, mass assignment is a feature that allows you to create or update multiple attributes on a model in a single statement. The `fillable` property on a model defines which attributes should be mass-assignable. This is crucial for preventing mass assignment vulnerabilities.
Defining the Fillable Property
To enable mass assignment for specific attributes in your model, you should define the `fillable` property within the model class. Here’s how you can do that:
“`php
class User extends Model
{
protected $fillable = [‘name’, ’email’, ‘password’];
}
“`
In this example, only the `name`, `email`, and `password` attributes can be mass-assigned. Any attempt to mass-assign attributes not listed in this array will be ignored.
Using the Fillable Property with Mass Assignment
When you want to create a new model instance or update an existing one, you can use the `create` or `update` methods. For example:
“`php
// Creating a new user
User::create([
‘name’ => ‘John Doe’,
’email’ => ‘[email protected]’,
‘password’ => bcrypt(‘secret’)
]);
“`
Since `name`, `email`, and `password` are in the `fillable` array, this operation will succeed. If you attempt to mass-assign an attribute like `role`, which is not in the `fillable` array, it will be ignored.
Best Practices for Managing Fillable Attributes
When managing the `fillable` property, consider the following best practices:
- Limit Attributes: Only include attributes that are essential for mass assignment to minimize risk.
- Use Guarded: Alternatively, you can use the `guarded` property to specify attributes that should not be mass-assignable. This is particularly useful when you have a large number of attributes.
“`php
protected $guarded = [‘id’, ‘created_at’, ‘updated_at’];
“`
- Regular Reviews: Regularly review your models to ensure that only necessary attributes are included in the `fillable` array.
Example of Fillable and Guarded Properties
Property | Description |
---|---|
`fillable` | Specifies which attributes are mass-assignable. |
`guarded` | Specifies which attributes are not mass-assignable. |
Handling Relationships with Fillable
When dealing with relationships, ensure that you handle mass assignment appropriately. For instance, when creating related models, you may need to assign attributes to both the parent and child models.
Example of creating a post with a category:
“`php
$post = Post::create($request->only([‘title’, ‘body’]));
$post->category()->attach($request->input(‘category_id’));
“`
In this case, the `title` and `body` attributes must be in the `fillable` array of the `Post` model.
Conclusion on Fillable Property Management
Proper management of the `fillable` property is essential for maintaining security and functionality in Laravel applications. Regular updates and audits of the fillable attributes will help ensure that the application remains secure against mass assignment vulnerabilities.
Expert Insights on Mass Assignment in Laravel
Emily Tran (Senior Laravel Developer, CodeCraft Solutions). “Understanding how to effectively manage mass assignment in Laravel is crucial for maintaining application security. By adding fields to the fillable property, developers can precisely control which attributes are mass assignable, thus preventing unauthorized data manipulation.”
Michael Chen (Software Architect, DevOps Innovations). “The fillable property in Laravel is a powerful feature that allows developers to streamline data handling. By thoughtfully selecting which attributes to include, teams can enhance both the performance and security of their applications, minimizing the risk of mass assignment vulnerabilities.”
Sarah Patel (Laravel Consultant, Web Solutions Group). “When working with Laravel, it is essential to be deliberate about the attributes you add to the fillable array. This practice not only simplifies the code but also reinforces the principle of least privilege, ensuring that only the intended data is processed during mass assignment operations.”
Frequently Asked Questions (FAQs)
What is the fillable property in Laravel?
The fillable property in Laravel is an array that specifies which attributes of a model can be mass-assigned. This is a security feature that helps prevent mass assignment vulnerabilities.
How do I add attributes to the fillable property in Laravel?
To add attributes to the fillable property, you need to define the `$fillable` array in your Eloquent model class and include the attributes you wish to allow for mass assignment.
Can I use the guarded property instead of fillable in Laravel?
Yes, you can use the guarded property, which is the inverse of fillable. By defining the `$guarded` array, you specify which attributes should not be mass-assignable, allowing all others by default.
What happens if I do not define the fillable property?
If you do not define the fillable property, Laravel will prevent mass assignment for all attributes, which can lead to errors when attempting to create or update models using mass assignment.
Is it possible to dynamically add attributes to the fillable property?
No, the fillable property must be defined as a static array within the model class. However, you can manipulate the array in your code if necessary, but this is not recommended for maintaining clarity and security.
What are the best practices for using the fillable property in Laravel?
Best practices include explicitly defining only the attributes that should be mass-assignable, avoiding the use of wildcards, and regularly reviewing the fillable array to ensure it aligns with your application’s security requirements.
In Laravel, the fillable property is a crucial aspect of the Eloquent ORM that allows developers to specify which attributes of a model can be mass assigned. By defining the fillable property, developers can protect their application from mass assignment vulnerabilities, ensuring that only the intended attributes can be populated in bulk. This feature is essential for maintaining data integrity and security within an application.
To add attributes to the fillable property, developers simply need to include the desired fields in the `$fillable` array within their model class. This straightforward process enhances the model’s flexibility, allowing for efficient data handling and manipulation. By leveraging this functionality, developers can streamline the process of creating or updating records, making it easier to work with user input or bulk data operations.
understanding how to effectively use the fillable property in Laravel is vital for any developer working with Eloquent. It not only safeguards against potential security risks but also improves the overall efficiency of data management within an application. By carefully selecting which attributes to include in the fillable array, developers can create robust and secure applications that handle user data responsibly.
Author Profile
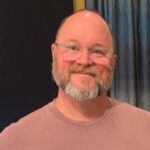
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?