How Do You Create a New Line in Python?
### Introduction
In the world of programming, clarity and organization are paramount, especially when it comes to displaying output. One of the simplest yet most effective ways to enhance readability in your Python scripts is by mastering the art of creating new lines. Whether you’re crafting a user-friendly interface, logging information, or simply printing messages to the console, knowing how to effectively manage line breaks can significantly improve the presentation of your data. In this article, we’ll explore the various methods to insert new lines in Python, empowering you to write cleaner and more intuitive code.
Creating new lines in Python is not just a matter of aesthetics; it’s about structuring your output in a way that makes sense to the user. From the basic print function to more advanced string manipulation techniques, Python offers a variety of tools that allow you to control how your text is displayed. Understanding these options will not only help you format your output but also enhance the overall user experience of your applications.
As we delve deeper into this topic, we will examine different approaches to inserting new lines, including the use of escape characters and string methods. Whether you are a beginner looking to grasp the fundamentals or an experienced coder seeking to refine your skills, this guide will provide you with the insights needed to effectively manage new lines in your Python
Using the Print Function
In Python, the simplest way to create a new line in your output is by using the `print()` function. By default, `print()` adds a newline character at the end of the output. You can explicitly indicate a new line by including `\n` within the string.
Example:
python
print(“Hello, World!”)
print(“Welcome to Python programming.\nEnjoy your coding!”)
This will output:
Hello, World!
Welcome to Python programming.
Enjoy your coding!
String Concatenation
Another method to introduce new lines is through string concatenation, which allows you to combine multiple strings, including newline characters. This can be particularly useful when constructing complex output.
Example:
python
line1 = “First line.”
line2 = “Second line.”
output = line1 + “\n” + line2
print(output)
Output:
First line.
Second line.
Using Triple Quotes for Multiline Strings
Python supports multiline strings, which can be created using triple quotes (`”’` or `”””`). This method allows you to write strings that span multiple lines directly in your code, maintaining the structure visually.
Example:
python
multiline_string = “””This is the first line.
This is the second line.
This is the third line.”””
print(multiline_string)
Output:
This is the first line.
This is the second line.
This is the third line.
Writing to Files with New Lines
When writing to files, you can also include new lines using the same techniques. The newline character `\n` can be included in the strings you write to a file.
Example:
python
with open(‘output.txt’, ‘w’) as file:
file.write(“Line one.\nLine two.\nLine three.”)
This will create a file named `output.txt` with the following content:
Line one.
Line two.
Line three.
Table of Common Methods for New Lines
Below is a comparison table of common methods to create new lines in Python.
Method | Description | Example |
---|---|---|
Print Function | Automatically adds a new line after each print. | print(“Hello\nWorld”) |
String Concatenation | Combines strings with newline characters. | str1 + “\n” + str2 |
Triple Quotes | Allows multiline strings directly in code. | “””Line 1\nLine 2″”” |
File Writing | Inserts new lines when writing to files. | file.write(“Line 1\nLine 2”) |
Each of these methods provides a way to manage new lines effectively in your Python programs, allowing for clear and organized output.
Using the Print Function
In Python, the simplest way to create a new line in output is by using the `print()` function. By default, `print()` adds a newline character at the end of the output. However, you can also manually specify new lines within the string.
- Basic usage of `print()`:
python
print(“Hello, World!”)
This will output:
Hello, World!
- Adding a new line:
python
print(“Hello, World!\nWelcome to Python!”)
The output will be:
Hello, World!
Welcome to Python!
Using Escape Characters
New lines can be introduced in strings using escape characters. The newline character `\n` is commonly used within strings to indicate where a new line should begin.
- Example:
python
message = “This is the first line.\nThis is the second line.”
print(message)
Output:
This is the first line.
This is the second line.
- Other escape characters include:
- `\t` for a tab space
- `\\` for a backslash
Using Triple Quotes for Multi-line Strings
Python allows the use of triple quotes (either single or double) to create multi-line strings. This method is particularly useful for longer text blocks.
- Example:
python
multi_line_string = “””This is the first line.
This is the second line.
This is the third line.”””
print(multi_line_string)
Output:
This is the first line.
This is the second line.
This is the third line.
Joining Strings with New Lines
You can also join multiple strings with new line characters using the `join()` method, which provides a clean way to concatenate strings with a specified separator.
- Example:
python
lines = [“Line 1”, “Line 2”, “Line 3”]
output = “\n”.join(lines)
print(output)
Output:
Line 1
Line 2
Line 3
Writing to Files with New Lines
When writing to files, new lines can be incorporated using the same newline character or through the `write()` function.
- Example:
python
with open(‘output.txt’, ‘w’) as file:
file.write(“First line.\nSecond line.\nThird line.”)
This will create a file `output.txt` with the following content:
First line.
Second line.
Third line.
Using these methods allows for flexible control over text formatting and output in Python, making it easier to manage how information is displayed or stored.
Expert Insights on Creating New Lines in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “In Python, the most common way to create a new line is by using the newline character, represented as ‘\n’. This character can be included in strings to format output effectively, ensuring readability and organization in your code.”
Michael Thompson (Lead Instructor, Python Programming Academy). “When working with multi-line strings in Python, the triple quotes (”’ or “””) provide an excellent way to include new lines without explicitly using the newline character. This feature enhances code clarity, especially for documentation or long text outputs.”
Sarah Lee (Data Scientist, Tech Innovations Inc.). “For developers dealing with file operations, using the newline character is crucial when writing to files. By specifying ‘\n’ in your write methods, you can ensure that each entry is properly separated, which is vital for data integrity and ease of analysis.”
Frequently Asked Questions (FAQs)
How do I create a new line in a Python string?
You can create a new line in a Python string by using the newline character `\n`. For example, `print(“Hello\nWorld”)` will output:
Hello
World
Can I use triple quotes to create multi-line strings in Python?
Yes, triple quotes (either `”’` or `”””`) allow you to create multi-line strings without needing to use newline characters explicitly. For instance:
python
text = “””This is line one.
This is line two.”””
print(text)
What function can I use to print multiple lines in Python?
You can use the `print()` function, which automatically adds a new line after each call. To print multiple lines, simply call `print()` multiple times, or use `\n` within a single print statement.
Is there a way to suppress the automatic newline in the print function?
Yes, you can suppress the automatic newline by using the `end` parameter in the `print()` function. For example, `print(“Hello”, end=””)` will not add a newline after “Hello”.
How can I read a file line by line in Python?
You can read a file line by line using a `for` loop. For example:
python
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line)
This will print each line in the file, including any new lines.
What is the difference between `\n` and `os.linesep` in Python?
The `\n` character represents a new line in Unix-based systems, while `os.linesep` is a platform-independent way to get the appropriate new line character(s) for the operating system. Use `os.linesep` for cross-platform compatibility.
In Python, creating a new line can be accomplished in several ways, primarily using the newline character `\n`. This character can be included within strings to indicate where a line break should occur. Additionally, the `print()` function in Python automatically adds a new line after each call unless specified otherwise. This behavior allows for easy formatting of output in console applications.
Another method to create new lines is by using triple quotes for multi-line strings. This approach is particularly useful for longer text blocks, as it preserves the formatting and includes line breaks as they appear in the code. Utilizing these techniques effectively can enhance the readability and structure of the output in Python programs.
In summary, understanding how to implement new lines in Python is essential for effective text formatting. By leveraging the newline character, the `print()` function, and multi-line strings, developers can create clear and organized outputs. Mastery of these methods will contribute to improved coding practices and better user experiences in Python applications.
Author Profile
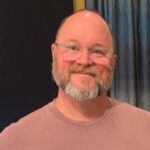
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?