How Can You Easily Input a List in Python?
### Introduction
In the world of programming, the ability to manage and manipulate data efficiently is paramount, and Python stands out as a versatile language that simplifies these tasks. One of the fundamental concepts every aspiring Python developer must master is the input of lists. Lists are a powerful data structure that allows you to store multiple items in a single variable, making them essential for a wide range of applications—from data analysis to machine learning. Whether you’re a beginner looking to grasp the basics or an experienced coder aiming to refine your skills, understanding how to input lists in Python is a crucial step on your coding journey.
As you delve into the intricacies of list input in Python, you’ll discover various methods to gather data from users, read from files, or even generate lists programmatically. Each approach offers unique advantages and can be tailored to fit the specific needs of your project. From simple user prompts to more complex data handling techniques, the ways to input a list are as diverse as the applications they serve.
Moreover, mastering list input not only enhances your coding proficiency but also empowers you to create more dynamic and interactive programs. With lists being a cornerstone of data manipulation in Python, understanding how to input them effectively will open doors to more advanced topics, such as data structures, algorithms, and beyond.
Using Built-in Functions to Input a List
Python provides several built-in functions to facilitate user input. The `input()` function is commonly used to accept user input as a string. To convert this string into a list, further manipulation is required. Here are some effective methods to achieve this:
- Using `split()` Method: This method breaks a string into a list based on a specified delimiter, which defaults to whitespace.
python
user_input = input(“Enter numbers separated by spaces: “)
number_list = user_input.split()
- Using List Comprehension: If the input needs to be transformed into a specific data type, such as integers, list comprehension can be utilized.
python
user_input = input(“Enter numbers separated by spaces: “)
number_list = [int(num) for num in user_input.split()]
Reading a List from a File
In some scenarios, data may be stored in a file. Python’s file handling capabilities allow you to read a list directly from a text file. The following steps illustrate how to accomplish this:
- Open the File: Use the `open()` function to access the file.
- Read Lines: Employ the `readlines()` method to retrieve lines as a list.
- Process Data: Clean and convert the data as necessary.
Here’s an example:
python
with open(‘data.txt’, ‘r’) as file:
number_list = [int(line.strip()) for line in file.readlines()]
This code reads each line from `data.txt`, strips any leading or trailing whitespace, and converts each line into an integer.
Creating Lists with User-defined Functions
For more complex input scenarios, defining a custom function can streamline the process. This is particularly useful when multiple inputs are required or if validation is needed.
python
def input_list(prompt):
user_input = input(prompt)
return [int(num) for num in user_input.split()]
my_list = input_list(“Enter integers separated by spaces: “)
This function prompts the user for input and returns a list of integers.
Comparison of Input Methods
The following table summarizes the different methods for inputting a list in Python:
Method | Usage | Example Code |
---|---|---|
Using `input()` and `split()` | Simple input from user | number_list = input().split() |
List Comprehension | Type conversion in one line | number_list = [int(num) for num in input().split()] |
File Input | Reading from a file | number_list = [int(line.strip()) for line in open('data.txt')] |
User-defined Function | Custom input handling | def input_list(prompt): ... |
Understanding these various methods will empower you to effectively gather input in list form, catering to different requirements and scenarios in your Python programs.
Creating a List in Python
To input a list in Python, you can create it using square brackets `[]`. Lists can contain elements of different data types, including integers, strings, and even other lists. Here are examples of how to define lists:
python
# A list of integers
int_list = [1, 2, 3, 4, 5]
# A list of strings
str_list = [“apple”, “banana”, “cherry”]
# A mixed list
mixed_list = [1, “apple”, 3.14, True]
Inputting Data into a List
You can gather user input and populate a list dynamically using the `input()` function within a loop. Here’s a step-by-step method to achieve this:
- Initialize an empty list.
- Use a loop to prompt the user for input.
- Append each input to the list.
python
# Initialize an empty list
user_list = []
# Number of elements to input
num_elements = int(input(“How many elements do you want to add to the list? “))
# Collect input
for _ in range(num_elements):
element = input(“Enter an element: “)
user_list.append(element)
print(user_list)
Using List Comprehensions
Python supports list comprehensions, which provide a concise way to create lists. You can use them to generate a list based on existing iterables or conditions. For example, to create a list of squares of numbers:
python
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Reading Input from a String
If you have a string of values separated by commas, you can convert it into a list using the `split()` method. Here’s how:
python
input_string = input(“Enter elements separated by commas: “)
element_list = input_string.split(‘,’)
element_list = [elem.strip() for elem in element_list] # Removes any extra whitespace
print(element_list)
Appending and Extending Lists
To add elements to an existing list, you can use the `append()` method to add a single element or the `extend()` method to add multiple elements from another iterable.
Method | Description | Example |
---|---|---|
`append()` | Adds a single element to the end of the list | `my_list.append(4)` |
`extend()` | Adds elements from another iterable | `my_list.extend([5, 6])` |
python
my_list = [1, 2, 3]
my_list.append(4) # my_list is now [1, 2, 3, 4]
my_list.extend([5, 6]) # my_list is now [1, 2, 3, 4, 5, 6]
Multi-dimensional Lists
Lists can also be multi-dimensional, allowing for the creation of matrices or grids. You can define a two-dimensional list as follows:
python
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
You can access elements using indices:
python
element = matrix[1][2] # Accesses the element 6
In Python, the flexibility of lists allows for a variety of input methods and manipulations, making it a powerful tool for data storage and management.
Expert Insights on Inputting Lists in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When inputting a list in Python, utilizing the built-in `input()` function is essential. It allows for dynamic data entry, which can then be processed into a list format using methods such as `split()`. This approach is particularly effective for handling user-generated data.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “To input a list in Python, one must consider the data type and structure required. Using list comprehensions alongside the `input()` function can streamline the process, allowing for concise and efficient list creation from user input.”
Jessica Lin (Data Scientist, Analytics Hub). “Incorporating error handling while inputting a list in Python is crucial. Implementing try-except blocks ensures that the program can gracefully handle invalid inputs, thus maintaining robustness in data collection and processing.”
Frequently Asked Questions (FAQs)
How do I create a list in Python?
You can create a list in Python by enclosing a comma-separated sequence of values within square brackets. For example: `my_list = [1, 2, 3, ‘four’]`.
What types of elements can a Python list contain?
A Python list can contain elements of different data types, including integers, floats, strings, and even other lists or objects.
How can I add elements to an existing list in Python?
You can add elements to an existing list using the `append()` method to add a single element or the `extend()` method to add multiple elements. For example: `my_list.append(5)` or `my_list.extend([6, 7])`.
How do I access elements in a Python list?
You can access elements in a Python list by using their index, which starts at 0. For example, `my_list[0]` retrieves the first element, while `my_list[-1]` retrieves the last element.
What is the difference between a list and a tuple in Python?
A list is mutable, meaning you can modify it after creation, while a tuple is immutable, meaning it cannot be changed. Lists use square brackets `[]`, while tuples use parentheses `()`.
How can I input a list from user input in Python?
You can input a list from user input by using the `input()` function and then converting the input string into a list. For example: `user_input = input(“Enter numbers separated by commas: “)` followed by `my_list = user_input.split(‘,’)`.
In Python, inputting a list can be accomplished through various methods, each catering to different use cases and user preferences. One of the most straightforward ways is by using the built-in `input()` function, which allows users to enter a string that can then be processed into a list. By utilizing the `split()` method, users can easily convert a space-separated string into a list of elements. Alternatively, users may opt for list comprehensions or the `map()` function to create lists from user input in a more structured manner.
Another effective approach involves using the `eval()` function, which can interpret a string as a Python expression, allowing for the direct input of a list in its literal form. However, caution is advised with this method due to potential security risks associated with executing arbitrary code. For more complex scenarios, such as reading lists from files or external sources, Python provides robust libraries like `csv` or `json`, which facilitate the input of structured data into lists.
Key takeaways include the importance of understanding the context in which a list is being inputted, as this will influence the choice of method. Additionally, users should be mindful of data validation and error handling to ensure that the inputted data meets the expected
Author Profile
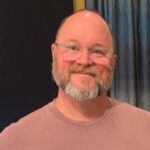
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?