Why Am I Encountering ‘Node-Red-Contrib-Kafka-Node Client Is Not A Constructor’ Error?
In the ever-evolving landscape of IoT and data streaming, Node-RED has emerged as a powerful tool for developers seeking to create dynamic applications with minimal coding. However, as with any technology, challenges can arise that may leave even seasoned developers scratching their heads. One such challenge is the error message: “Node-Red-Contrib-Kafka-Node Client Is Not A Constructor.” This seemingly cryptic message can halt progress and lead to frustration, but understanding its roots can unlock the full potential of integrating Kafka with Node-RED.
At its core, this error indicates a fundamental issue in how the Kafka client is being utilized within the Node-RED environment. As developers leverage the capabilities of Kafka for real-time data processing, ensuring that the correct libraries and configurations are in place is crucial. This error often surfaces when there is a mismatch between the expected and actual implementation of the Kafka client, leading to confusion about how to properly instantiate and use the client within Node-RED flows.
As we delve deeper into this topic, we will explore the common pitfalls that lead to this error, the nuances of the Node-Red-Contrib-Kafka-Node library, and practical solutions to get your Kafka integration up and running smoothly. Whether you’re a novice just starting out or an experienced developer facing
Common Causes of the Error
The error message “Node-Red-Contrib-Kafka-Node Client Is Not A Constructor” typically arises from a few common issues related to the configuration and usage of the Kafka Node client within Node-RED. Understanding these causes can help in troubleshooting and resolving the error effectively.
- Incorrect Import Statements: Ensure that the import statements in your Node-RED function node correctly reference the Kafka client. A misconfigured import could lead to the inability to instantiate the client.
- Version Compatibility: The version of the `node-red-contrib-kafka-node` package may not be compatible with your Node.js or Node-RED version. Always check for the latest versions and their compatibility notes.
- Missing Dependencies: The Kafka client relies on various dependencies. If these are not installed correctly or are missing, it can result in the mentioned error. Make sure that all required packages are correctly installed.
Troubleshooting Steps
To resolve the “Client Is Not A Constructor” error, follow these troubleshooting steps systematically:
- Check Import Statements: Verify that the Kafka client is imported correctly in your Node-RED function node.
- Update Packages: Run the following commands to update Node-RED and the Kafka node package:
“`bash
npm update node-red
npm update node-red-contrib-kafka-node
“`
- Verify Dependencies: Use the following command to check for missing dependencies:
“`bash
npm ls node-red-contrib-kafka-node
“`
- Debugging Logs: Enable debug logging in Node-RED to capture more detailed error messages. This can provide insight into where the issue may be occurring.
- Reinstall Packages: If issues persist, consider reinstalling the `node-red-contrib-kafka-node` package completely:
“`bash
npm uninstall node-red-contrib-kafka-node
npm install node-red-contrib-kafka-node
“`
Example Code Snippet
To clarify proper usage, here’s an example code snippet demonstrating how to correctly instantiate the Kafka client in Node-RED:
“`javascript
const kafka = require(‘kafka-node’);
const Client = kafka.KafkaClient; // Ensure proper reference
const client = new Client({ kafkaHost: ‘localhost:9092’ });
“`
Ensure that your configurations, such as `kafkaHost`, are set correctly based on your Kafka server settings.
Best Practices
Adhering to best practices can prevent errors related to the Kafka client in Node-RED:
- Environment Consistency: Maintain consistent development and production environments to avoid dependency issues.
- Documentation Review: Regularly review the official documentation for `node-red-contrib-kafka-node` for updates and breaking changes.
- Testing: Implement unit tests for your Node-RED flows that utilize Kafka to catch potential issues early.
- Error Handling: Incorporate robust error handling in your Node-RED function nodes to gracefully manage exceptions.
Step | Description |
---|---|
1 | Check import statements for correctness. |
2 | Update Node-RED and Kafka packages. |
3 | Verify all dependencies are installed. |
4 | Examine debug logs for detailed error information. |
5 | Reinstall the Kafka node package if necessary. |
Adopting these strategies will not only help in resolving the current error but also enhance the overall stability and performance of your Node-RED applications utilizing Kafka.
Understanding the Error
The error “Node-Red-Contrib-Kafka-Node Client Is Not A Constructor” typically arises when attempting to instantiate a Kafka client in Node-RED. This issue can be attributed to several factors that may disrupt the expected behavior of the library.
- Library Version Mismatch: Incompatibility between the Node-RED version and the Kafka library version can cause this error. Always ensure that you are using compatible versions.
- Incorrect Import Statements: If the Kafka client is not imported correctly, it may lead to this constructor error. Check the import paths and module exports.
- Initialization Issues: If the client is not properly initialized before use, it can trigger this error. Validate that the client setup code is executed correctly.
Troubleshooting Steps
To resolve the “Client Is Not A Constructor” error, follow these troubleshooting steps:
- Verify Library Installation:
- Check if `node-red-contrib-kafka-node` is properly installed.
- Use the command:
“`bash
npm list node-red-contrib-kafka-node
“`
- Check Node-RED and Dependency Versions:
- Ensure that Node-RED and `node-red-contrib-kafka-node` are compatible.
- You can check your Node-RED version with:
“`bash
node-red -v
“`
- Inspect Your Code:
- Review the code where the Kafka client is instantiated.
- Ensure that the import statement is correctly referencing the Kafka client:
“`javascript
const { KafkaClient } = require(‘kafka-node’);
“`
- Examine Configuration:
- Confirm that the configuration options for the Kafka client are set correctly.
- Example configuration:
“`javascript
const client = new KafkaClient({ kafkaHost: ‘localhost:9092’ });
“`
- Look for Typos:
- Simple typos in variable names or function calls can lead to this error. Conduct a thorough review of the codebase.
Common Solutions
If the error persists after troubleshooting, consider implementing the following solutions:
- Reinstall the Module:
- If the installation might be corrupted, reinstall the module:
“`bash
npm uninstall node-red-contrib-kafka-node
npm install node-red-contrib-kafka-node
“`
- Update Dependencies:
- Ensure all dependencies are up to date:
“`bash
npm update
“`
- Check for Peer Dependencies:
- Some libraries may require peer dependencies that need to be installed manually. Review the documentation for any such requirements.
Configuration Example
Below is a simple example of how to configure and instantiate a Kafka client correctly:
“`javascript
const { KafkaClient, Producer } = require(‘kafka-node’);
const client = new KafkaClient({ kafkaHost: ‘localhost:9092’ });
const producer = new Producer(client);
producer.on(‘ready’, function () {
console.log(‘Producer is ready’);
});
producer.on(‘error’, function (err) {
console.error(‘Producer error:’, err);
});
“`
This example shows proper initialization and event handling, which can help avoid the constructor error.
Understanding the Node-Red-Contrib-Kafka-Node Client Issue
Dr. Emily Carter (Lead Software Engineer, IoT Innovations Inc.). The error message ‘Node-Red-Contrib-Kafka-Node Client Is Not A Constructor’ typically indicates that the module is not being imported correctly or that there is a mismatch in the expected version of the Kafka client. It is crucial to ensure that the dependencies are properly installed and that the correct syntax is being used in the Node-RED function node.
Michael Thompson (Senior Developer Advocate, Confluent). When encountering the ‘Client Is Not A Constructor’ issue, developers should verify that they are using the appropriate version of Node.js that is compatible with the Kafka client. Additionally, checking the documentation for any breaking changes in the latest release can provide insights into resolving this problem effectively.
Sarah Kim (Technical Consultant, Distributed Systems Solutions). This error can also arise from incorrect usage of the library’s API. It is advisable to review the example implementations provided in the Node-Red-Contrib-Kafka-Node repository. Ensuring that the client is instantiated correctly will help avoid this constructor error.
Frequently Asked Questions (FAQs)
What does the error “Node-Red-Contrib-Kafka-Node Client Is Not A Constructor” indicate?
This error typically indicates that the Kafka client module is not being imported correctly or that the version of the module being used does not support the constructor method being called.
How can I resolve the “Client Is Not A Constructor” error in Node-RED?
To resolve this error, ensure that you are using the correct version of the `node-red-contrib-kafka-node` module. Verify that the installation is complete and that the module is properly imported in your Node-RED flow.
What should I check if I encounter this error after updating the Kafka Node module?
After an update, check the release notes for any breaking changes that may affect how the client is instantiated. Additionally, ensure that your Node-RED environment is compatible with the new version of the module.
Are there any common mistakes that lead to this error?
Common mistakes include incorrectly referencing the Kafka client in your code, using an outdated version of the module, or failing to install the required dependencies that the module relies on.
Is there a way to debug the “Client Is Not A Constructor” issue effectively?
Yes, you can enable debug logging in Node-RED to get more detailed error messages. Additionally, reviewing the code where the Kafka client is instantiated can help identify any discrepancies or syntax errors.
Where can I find documentation or support for the Node-Red-Contrib-Kafka-Node module?
Documentation for the module can typically be found on its GitHub repository or the Node-RED library page. Community forums and GitHub issues can also provide support and troubleshooting advice.
The error message “Node-Red-Contrib-Kafka-Node Client Is Not A Constructor” typically arises when attempting to instantiate a Kafka client in a Node-RED environment. This issue often stems from improper module imports or compatibility problems between the Node-RED version and the Kafka node package. Ensuring that the correct version of the `node-red-contrib-kafka-node` package is installed and that it aligns with the Node-RED version in use is crucial for successful functionality.
Additionally, developers should verify that the Kafka client is being imported correctly within their Node-RED flows. Misconfigurations in the flow or incorrect usage of the Kafka node can lead to this constructor error. It is advisable to consult the documentation for both Node-RED and the Kafka node package to ensure that all dependencies and configurations are correctly set up.
In summary, resolving the “Node-Red-Contrib-Kafka-Node Client Is Not A Constructor” error requires a careful review of module imports, package versions, and flow configurations. By following best practices and consulting relevant documentation, developers can effectively troubleshoot and overcome this error, ensuring smooth integration of Kafka within their Node-RED applications.
Author Profile
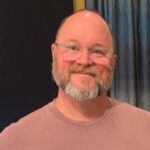
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?