How Can You Run a Python Script From Another Python Script?
In the world of programming, efficiency and modularity are paramount. As projects grow in complexity, the ability to run a Python script from another Python script becomes not just a convenience, but a necessity. Whether you’re looking to streamline your code, reuse functions across different scripts, or manage large applications, understanding how to effectively orchestrate multiple Python scripts can elevate your coding skills and enhance your workflow. In this article, we’ll explore the various methods available for executing one Python script from another, unlocking the potential for more organized and maintainable code.
When you need to leverage the functionality of an existing script without duplicating code, Python offers several straightforward approaches to achieve this. From using the built-in `import` statement to executing scripts as subprocesses, each method comes with its own advantages and use cases. By mastering these techniques, you can create a more modular codebase that can be easily updated and expanded, allowing for greater flexibility in your programming endeavors.
Moreover, the ability to run scripts from one another opens the door to more advanced programming paradigms, such as creating command-line interfaces or automating tasks. This not only makes your code more efficient but also enhances collaboration among team members, as different scripts can be developed independently and integrated seamlessly. Join us as we delve
Using the `subprocess` Module
The `subprocess` module is a powerful tool in Python that allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. This is one of the most common methods for executing a Python script from another Python script.
To run a Python script using `subprocess`, you would typically use the `subprocess.run()` function. Here is a basic example:
“`python
import subprocess
result = subprocess.run([‘python’, ‘script.py’], capture_output=True, text=True)
print(result.stdout)
“`
In this example, `script.py` is the name of the Python file you want to execute. The `capture_output=True` argument captures the standard output and error, while `text=True` ensures the output is returned as a string.
Using the `exec` Function
The `exec` function can be used to execute Python code dynamically. If you want to run a script from another script, you can read the script’s content and execute it in the current namespace.
“`python
with open(‘script.py’) as file:
exec(file.read())
“`
This method is less common for running entire scripts due to its limitations and potential security risks, especially if the content of the script is not controlled.
Importing as a Module
Another approach to run a Python script from another script is to import it as a module. This method allows for better modularization and reuse of code.
Assuming you have a Python script named `script.py`, you can import it as follows:
“`python
import script
“`
If `script.py` contains functions or classes, you can call them directly:
“`python
script.some_function()
“`
This method requires that the script you are importing follows proper Python module conventions.
Comparison Table
Method | Use Case | Advantages | Disadvantages |
---|---|---|---|
subprocess | Run external scripts | Control over inputs/outputs | More overhead |
exec | Execute dynamic code | Simple execution | Security risks, limited scope |
Import | Reuse code in modules | Encapsulation and organization | Requires proper module structure |
Considerations for Execution
When deciding which method to use for running a Python script from another script, consider the following:
- Performance: `subprocess` may introduce more overhead than importing a module.
- Reusability: Importing allows for better organization and reuse of code.
- Security: Using `exec` can pose security risks if the script content is not controlled.
- Output Handling: If you need to capture outputs and errors, `subprocess` is the best choice.
By understanding these methods and considerations, you can choose the most appropriate approach for your specific use case in Python programming.
Methods to Run a Python Script from Another Python Script
Running a Python script from another Python script can be achieved through several methods, each with its own use cases and benefits. Below are the most common approaches:
Using the Import Statement
The simplest way to run a script is to import it as a module. This allows you to access its functions and classes directly.
- How to Import:
“`python
import script_name
“`
- Example:
“`python
main_script.py
import helper_script
helper_script.some_function()
“`
This method is effective when you need to call specific functions or classes from the imported script.
Using the exec() Function
The `exec()` function can execute Python code dynamically. This is particularly useful for running code stored in a string or a file.
- Example:
“`python
with open(‘script_name.py’) as file:
exec(file.read())
“`
While `exec()` provides flexibility, it can introduce security risks if handling untrusted input.
Using the Subprocess Module
For running a script as a separate process, the `subprocess` module is the most robust choice. This method allows you to execute shell commands and scripts and handle their input and output effectively.
- Basic Usage:
“`python
import subprocess
subprocess.run([‘python’, ‘script_name.py’])
“`
- Advantages:
- Runs the script in a separate process.
- Captures output and errors.
- Example with Output:
“`python
result = subprocess.run([‘python’, ‘script_name.py’], capture_output=True, text=True)
print(result.stdout)
“`
Using the os.system() Method
Another straightforward approach is using `os.system()`, which executes a command in the shell.
- Example:
“`python
import os
os.system(‘python script_name.py’)
“`
This method is less flexible than `subprocess`, as it does not capture output or handle errors directly.
Using the Runpy Module
The `runpy` module allows you to locate and run Python programs using their module name or path.
- Example:
“`python
import runpy
runpy.run_path(‘script_name.py’)
“`
This method can be useful for executing scripts without needing to import them as modules.
Comparison Table of Methods
Method | Use Case | Output Capture | Process Isolation |
---|---|---|---|
Import | Calling functions/classes | No | No |
exec() | Executing dynamic code | No | No |
Subprocess | Running scripts independently | Yes | Yes |
os.system() | Simple command execution | No | No |
Runpy | Running scripts by path | No | No |
Each method has its specific advantages, and the choice depends on the requirements of the application and the desired level of control over the execution environment.
Expert Insights on Running Python Scripts from Another Python Script
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Running Python scripts from another Python script is a powerful technique that enhances modularity and reusability in code. By utilizing the `subprocess` module, developers can execute external scripts seamlessly, allowing for better organization of complex projects.”
Michael Chen (Lead Python Developer, Data Solutions Corp.). “When orchestrating multiple Python scripts, it’s crucial to manage dependencies and execution order carefully. Using the `import` statement for internal scripts or `os.system()` for external scripts can streamline workflows, but understanding the context of each method is essential to avoid performance issues.”
Sarah Patel (Python Instructor, Code Academy). “Teaching students to run scripts from one another is fundamental in Python education. It not only demonstrates the importance of code structure but also prepares them for real-world applications where scripts often need to interact. Encouraging the use of functions and modules can lead to more maintainable code.”
Frequently Asked Questions (FAQs)
How can I run a Python script from another Python script?
You can run a Python script from another script by using the `import` statement or the `subprocess` module. The `import` statement allows you to call functions and classes directly, while `subprocess.run()` can execute the script as a separate process.
What is the difference between using `import` and `subprocess`?
Using `import` allows you to access functions and variables defined in the imported script, maintaining the same interpreter session. In contrast, `subprocess` runs the script in a new process, which isolates it from the main script, making it suitable for executing scripts that require separate execution contexts.
Can I pass arguments to a Python script when using `subprocess`?
Yes, you can pass arguments to a Python script using `subprocess.run()` by including them in the list passed to the function. For example, `subprocess.run([‘python’, ‘script.py’, ‘arg1’, ‘arg2’])` will execute `script.py` with `arg1` and `arg2` as command-line arguments.
Is it possible to capture the output of a script executed with `subprocess`?
Yes, you can capture the output of a script executed with `subprocess` by setting the `stdout` parameter to `subprocess.PIPE`. This allows you to access the output programmatically after the script has finished executing.
What should I do if the script I want to run is in a different directory?
You can specify the full path to the script when using `subprocess.run()`. Alternatively, you can change the current working directory using `os.chdir()` before executing the script, or use relative paths based on the current script’s location.
Are there any performance considerations when running scripts from another script?
Yes, using `subprocess` can introduce overhead due to process creation and inter-process communication. If performance is critical, consider using `import` to call functions directly, which avoids the overhead of starting a new process.
In summary, running a Python script from another Python script is a common practice that can enhance modularity and code reusability. This can be achieved through various methods, including using the `import` statement, the `subprocess` module, and the `exec()` function. Each method has its own advantages and use cases, allowing developers to choose the most appropriate approach based on their specific requirements.
The `import` statement is often the preferred method for executing functions or classes defined in another script, as it allows for better organization of code and enables the use of namespaces. On the other hand, the `subprocess` module provides a way to execute external scripts and capture their output, which is particularly useful for integrating scripts that may not be part of the same codebase. The `exec()` function, while powerful, should be used with caution due to potential security risks associated with executing arbitrary code.
Ultimately, understanding the various methods to run scripts from one another empowers developers to create more efficient and maintainable code. By leveraging these techniques, programmers can streamline their workflows, facilitate collaboration, and enhance the overall functionality of their applications. As such, mastering these approaches is essential for anyone looking to optimize their use of Python in complex projects
Author Profile
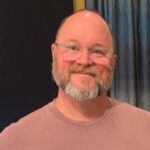
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?